Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / Scheduling / SpoolingTaskBase.cs / 1305376 / SpoolingTaskBase.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // SpoolingTaskBase.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Threading; using System.Diagnostics.Contracts; namespace System.Linq.Parallel { ////// A spooling task handles marshaling data from a producer to a consumer. It simply /// takes data from a producer and hands it off to a consumer. This class is the base /// class from which other concrete spooling tasks derive, encapsulating some common /// logic (such as capturing exceptions). /// internal abstract class SpoolingTaskBase : QueryTask { //------------------------------------------------------------------------------------ // Constructs a new spooling task. // // Arguments: // taskIndex - the unique index of this task // protected SpoolingTaskBase(int taskIndex, QueryTaskGroupState groupState) : base(taskIndex, groupState) { } //----------------------------------------------------------------------------------- // The implementation of the Work API just enumerates the producer's data, and // enqueues it into the consumer channel. Well, really, it just defers to extension // points (below) implemented by subclasses to do these things. // protected override void Work() { try { // Defer to the base class for the actual work. SpoolingWork(); } catch (Exception ex) { OperationCanceledException oce = ex as OperationCanceledException; if (oce != null && oce.CancellationToken == m_groupState.CancellationState.MergedCancellationToken && m_groupState.CancellationState.MergedCancellationToken.IsCancellationRequested) { //an expected internal cancellation has occurred. suppress this exception. } else { // TPL will catch and store the exception on the task object. We'll then later // turn around and wait on it, having the effect of propagating it. In the meantime, // we want to cooperative cancel all workers. m_groupState.CancellationState.InternalCancellationTokenSource.Cancel(); // And then repropagate to let TPL catch it. throw; } } finally { SpoolingFinally(); //dispose resources etc. } } //----------------------------------------------------------------------------------- // This method is responsible for enumerating results and enqueueing them to // the output channel(s) as appropriate. Each base class implements its own. // protected abstract void SpoolingWork(); //----------------------------------------------------------------------------------- // If the subclass needs to do something in the finally block of the main work loop, // it should override this and do it. Purely optional. // protected virtual void SpoolingFinally() { // (Intentionally left blank.) } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // SpoolingTaskBase.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Threading; using System.Diagnostics.Contracts; namespace System.Linq.Parallel { ////// A spooling task handles marshaling data from a producer to a consumer. It simply /// takes data from a producer and hands it off to a consumer. This class is the base /// class from which other concrete spooling tasks derive, encapsulating some common /// logic (such as capturing exceptions). /// internal abstract class SpoolingTaskBase : QueryTask { //------------------------------------------------------------------------------------ // Constructs a new spooling task. // // Arguments: // taskIndex - the unique index of this task // protected SpoolingTaskBase(int taskIndex, QueryTaskGroupState groupState) : base(taskIndex, groupState) { } //----------------------------------------------------------------------------------- // The implementation of the Work API just enumerates the producer's data, and // enqueues it into the consumer channel. Well, really, it just defers to extension // points (below) implemented by subclasses to do these things. // protected override void Work() { try { // Defer to the base class for the actual work. SpoolingWork(); } catch (Exception ex) { OperationCanceledException oce = ex as OperationCanceledException; if (oce != null && oce.CancellationToken == m_groupState.CancellationState.MergedCancellationToken && m_groupState.CancellationState.MergedCancellationToken.IsCancellationRequested) { //an expected internal cancellation has occurred. suppress this exception. } else { // TPL will catch and store the exception on the task object. We'll then later // turn around and wait on it, having the effect of propagating it. In the meantime, // we want to cooperative cancel all workers. m_groupState.CancellationState.InternalCancellationTokenSource.Cancel(); // And then repropagate to let TPL catch it. throw; } } finally { SpoolingFinally(); //dispose resources etc. } } //----------------------------------------------------------------------------------- // This method is responsible for enumerating results and enqueueing them to // the output channel(s) as appropriate. Each base class implements its own. // protected abstract void SpoolingWork(); //----------------------------------------------------------------------------------- // If the subclass needs to do something in the finally block of the main work loop, // it should override this and do it. Purely optional. // protected virtual void SpoolingFinally() { // (Intentionally left blank.) } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
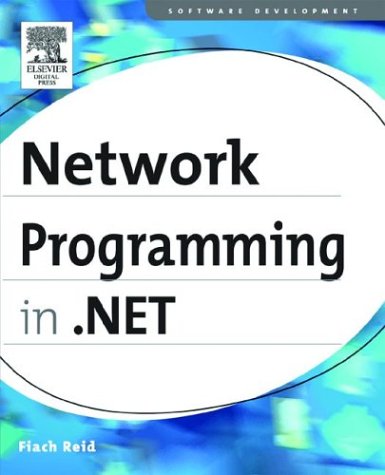
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypedColumnHandler.cs
- LabelAutomationPeer.cs
- ToolStripDropDownMenu.cs
- BamlCollectionHolder.cs
- DescriptionCreator.cs
- BulletedList.cs
- AssemblyEvidenceFactory.cs
- RoleManagerSection.cs
- DecoratedNameAttribute.cs
- ConfigurationSettings.cs
- PipelineDeploymentState.cs
- ButtonChrome.cs
- HighlightComponent.cs
- ServerValidateEventArgs.cs
- DataStreamFromComStream.cs
- KeyNotFoundException.cs
- ScriptServiceAttribute.cs
- ExecutionContext.cs
- JournalEntryStack.cs
- OledbConnectionStringbuilder.cs
- KeyNotFoundException.cs
- PropertyChangingEventArgs.cs
- DesignerCommandAdapter.cs
- CacheVirtualItemsEvent.cs
- CultureNotFoundException.cs
- ReferenceAssemblyAttribute.cs
- XmlQueryOutput.cs
- OdbcConnectionStringbuilder.cs
- GeneralTransform3DCollection.cs
- Normalizer.cs
- ResourceExpressionBuilder.cs
- ColumnWidthChangingEvent.cs
- DashStyle.cs
- PointF.cs
- DesignerAutoFormatStyle.cs
- CommonRemoteMemoryBlock.cs
- EtwTrace.cs
- X509WindowsSecurityToken.cs
- _RegBlobWebProxyDataBuilder.cs
- SelectManyQueryOperator.cs
- PointLightBase.cs
- SafeRightsManagementSessionHandle.cs
- relpropertyhelper.cs
- DesignerAttribute.cs
- XmlBinaryReaderSession.cs
- AbsoluteQuery.cs
- PackageRelationship.cs
- DependentTransaction.cs
- SecurityException.cs
- RawTextInputReport.cs
- ValueConversionAttribute.cs
- ArgumentElement.cs
- OleTxTransaction.cs
- XmlWrappingReader.cs
- ClientSponsor.cs
- PageCatalogPart.cs
- ConfigurationCollectionAttribute.cs
- WaitHandle.cs
- XmlElementList.cs
- SslStream.cs
- XmlSchemaGroupRef.cs
- FontWeights.cs
- ConstraintEnumerator.cs
- WindowsFont.cs
- StrokeDescriptor.cs
- Vector3DAnimationBase.cs
- AnnotationAdorner.cs
- WebControlParameterProxy.cs
- Process.cs
- SizeChangedInfo.cs
- SocketCache.cs
- DeferredTextReference.cs
- WebPartConnectionCollection.cs
- SecurityTokenAuthenticator.cs
- X509ChainElement.cs
- CodeDomDesignerLoader.cs
- DocumentSequenceHighlightLayer.cs
- DotNetATv1WindowsLogEntrySerializer.cs
- SiteOfOriginPart.cs
- HttpApplication.cs
- AsyncStreamReader.cs
- ListControl.cs
- Int16Animation.cs
- XmlObjectSerializerWriteContextComplex.cs
- GeneralTransformCollection.cs
- CharEnumerator.cs
- embossbitmapeffect.cs
- MulticastNotSupportedException.cs
- WindowsNonControl.cs
- WebPartConnectVerb.cs
- PropertyGroupDescription.cs
- Root.cs
- BaseDataListPage.cs
- WindowsAuthenticationModule.cs
- ManagementObject.cs
- BindingElementCollection.cs
- DynamicObject.cs
- DataTemplateKey.cs
- ContentValidator.cs
- ResourceLoader.cs