Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CommonUI / System / Drawing / Advanced / PointF.cs / 1 / PointF.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /*************************************************************************\ * * Copyright (c) 1998-1999, Microsoft Corp. All Rights Reserved. * * Module Name: * * PointF.cs * * Abstract: * * Floating-point coordinate point class * * Revision History: * * 12/14/1998 davidx * Created it. * \**************************************************************************/ namespace System.Drawing { using System.Diagnostics; using System.Drawing; using System.ComponentModel; using System; using System.Diagnostics.CodeAnalysis; using System.Globalization; /** * Represents a point in 2D coordinate space * (float precision floating-point coordinates) */ ////// /// Represents an ordered pair of x and y coordinates that /// define a point in a two-dimensional plane. /// [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public struct PointF { ////// /// public static readonly PointF Empty = new PointF(); private float x; private float y; /** * Create a new Point object at the given location */ ////// Creates a new instance of the ///class /// with member data left uninitialized. /// /// /// public PointF(float x, float y) { this.x = x; this.y = y; } ////// Initializes a new instance of the ///class /// with the specified coordinates. /// /// /// [Browsable(false)] public bool IsEmpty { get { return x == 0f && y == 0f; } } ////// Gets a value indicating whether this ///is empty. /// /// /// public float X { get { return x; } set { x = value; } } ////// Gets the x-coordinate of this ///. /// /// /// public float Y { get { return y; } set { y = value; } } ////// Gets the y-coordinate of this ///. /// /// /// public static PointF operator +(PointF pt, Size sz) { return Add(pt, sz); } ////// Translates a ///by a given . /// /// /// public static PointF operator -(PointF pt, Size sz) { return Subtract(pt, sz); } ////// Translates a ///by the negative of a given . /// /// public static PointF operator +(PointF pt, SizeF sz) { return Add(pt, sz); } ////// Translates a ///by a given . /// /// public static PointF operator -(PointF pt, SizeF sz) { return Subtract(pt, sz); } ////// Translates a ///by the negative of a given . /// /// /// public static bool operator ==(PointF left, PointF right) { return left.X == right.X && left.Y == right.Y; } ////// Compares two ///objects. The result specifies /// whether the values of the and properties of the two /// objects are equal. /// /// /// public static bool operator !=(PointF left, PointF right) { return !(left == right); } ////// Compares two ///objects. The result specifies whether the values /// of the or properties of the two /// /// objects are unequal. /// /// public static PointF Add(PointF pt, Size sz) { return new PointF(pt.X + sz.Width, pt.Y + sz.Height); } ////// Translates a ///by a given . /// /// public static PointF Subtract(PointF pt, Size sz) { return new PointF(pt.X - sz.Width, pt.Y - sz.Height); } ////// Translates a ///by the negative of a given . /// /// public static PointF Add(PointF pt, SizeF sz){ return new PointF(pt.X + sz.Width, pt.Y + sz.Height); } ////// Translates a ///by a given . /// /// public static PointF Subtract(PointF pt, SizeF sz){ return new PointF(pt.X - sz.Width, pt.Y - sz.Height); } ////// Translates a ///by the negative of a given . /// /// /// public override bool Equals(object obj) { if (!(obj is PointF)) return false; PointF comp = (PointF)obj; return comp.X == this.X && comp.Y == this.Y && comp.GetType().Equals(this.GetType()); } ///[To be supplied.] ////// /// public override int GetHashCode() { return base.GetHashCode(); } ///[To be supplied.] ///public override string ToString() { return string.Format(CultureInfo.CurrentCulture, "{{X={0}, Y={1}}}", x, y); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
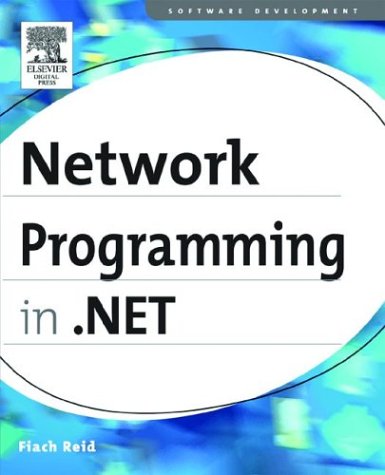
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemplatedEditableDesignerRegion.cs
- ValueExpressions.cs
- mediaeventargs.cs
- DbParameterHelper.cs
- HtmlLabelAdapter.cs
- CodeSubDirectoriesCollection.cs
- ArglessEventHandlerProxy.cs
- PointCollectionConverter.cs
- RelativeSource.cs
- SafeRegistryHandle.cs
- EventMappingSettingsCollection.cs
- CapabilitiesRule.cs
- TextBreakpoint.cs
- TreeNodeCollection.cs
- XsdDataContractExporter.cs
- SkinIDTypeConverter.cs
- AnnotationResourceCollection.cs
- ProtocolReflector.cs
- ApplicationFileCodeDomTreeGenerator.cs
- ReadOnlyHierarchicalDataSourceView.cs
- NameValueConfigurationElement.cs
- PerformanceCountersElement.cs
- ExpressionPrefixAttribute.cs
- EventData.cs
- GenericPrincipal.cs
- ProviderConnectionPoint.cs
- DataGridViewCellValidatingEventArgs.cs
- FilterElement.cs
- LocalizedNameDescriptionPair.cs
- SessionPageStateSection.cs
- RequestNavigateEventArgs.cs
- FormsAuthentication.cs
- AttachedAnnotationChangedEventArgs.cs
- XmlWellformedWriterHelpers.cs
- BaseTreeIterator.cs
- EntityDataSourceDataSelectionPanel.cs
- WsatServiceCertificate.cs
- GeneralTransform3D.cs
- DetailsViewDeletedEventArgs.cs
- SafeHandle.cs
- ConnectionPool.cs
- DatePickerTextBox.cs
- RotateTransform3D.cs
- PropertyDescriptorComparer.cs
- TextServicesCompartment.cs
- SctClaimDictionary.cs
- XmlSchemas.cs
- XmlTypeAttribute.cs
- NetSectionGroup.cs
- unsafenativemethodsother.cs
- DataGridViewColumnConverter.cs
- ExpiredSecurityTokenException.cs
- Clipboard.cs
- SchemaElementLookUpTable.cs
- Image.cs
- PageHandlerFactory.cs
- XmlSchemaAll.cs
- Aes.cs
- ExceptionValidationRule.cs
- CodeTypeOfExpression.cs
- HashHelper.cs
- Section.cs
- XmlTextReaderImpl.cs
- ExtractorMetadata.cs
- CodeBlockBuilder.cs
- PromptEventArgs.cs
- RankException.cs
- TypefaceCollection.cs
- MediaEntryAttribute.cs
- MD5CryptoServiceProvider.cs
- Input.cs
- LockCookie.cs
- ResourceExpression.cs
- SelectionBorderGlyph.cs
- UnionExpr.cs
- ChildTable.cs
- StorageEntityContainerMapping.cs
- NavigatorInput.cs
- IconConverter.cs
- WindowAutomationPeer.cs
- VisualBasicDesignerHelper.cs
- RequestTimeoutManager.cs
- DictionaryContent.cs
- NodeFunctions.cs
- WSFederationHttpBinding.cs
- PagesChangedEventArgs.cs
- UserNameSecurityTokenProvider.cs
- x509utils.cs
- ExpressionEditorAttribute.cs
- Translator.cs
- NamedElement.cs
- ToolStripDesignerAvailabilityAttribute.cs
- TextDecoration.cs
- Translator.cs
- FormViewInsertEventArgs.cs
- KnownBoxes.cs
- CompilationRelaxations.cs
- MenuAdapter.cs
- ProfileSection.cs
- LocalizableResourceBuilder.cs