Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / textformatting / TextBreakpoint.cs / 1 / TextBreakpoint.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: TextBreakpoint.cs // // Contents: A specialized text line representing state of line up to the // point where line break may occur // // Spec: [....]/sites/Avalon/Specs/Text%20Formatting%20API.doc // // Created: 1-1-2005 [....] ([....]) // //----------------------------------------------------------------------- using System; using System.Security; using MS.Internal; using MS.Internal.PresentationCore; using MS.Internal.TextFormatting; namespace System.Windows.Media.TextFormatting { ////// A specialized text line representing state of line up to the point /// where line break may occur. Unlike the normal text line, breakpoint /// could not draw or performs hit-testing operation. It could only reflect /// formatting result back to the client. /// #if OPTIMALBREAK_API public abstract class TextBreakpoint : ITextMetrics, IDisposable #else [FriendAccessAllowed] // used by Framework internal abstract class TextBreakpoint : ITextMetrics, IDisposable #endif { ////// Clean up text breakpoint internal resource /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ////// Actual clean up code to be overridden by subclasses, by default it does nothing. /// /// flag indicates whether the clean up is thru disposal protected virtual void Dispose(bool disposing) {} ////// Client to acquire a state at the point where breakpoint is determined by line breaking process; /// can be null when the line ends by the ending of the paragraph. Client may pass this /// value back to TextFormatter as an input argument to TextFormatter.FormatParagraphBreakpoints when /// formatting the next set of breakpoints within the same paragraph. /// public abstract TextLineBreak GetTextLineBreak(); ////// Client to get the handle of the internal factors that are used to determine penalty of this breakpoint. /// ////// Calling this method means that the client will now manage the lifetime of this unmanaged resource themselves using unsafe penalty handler. /// We would make a correspondent call to notify our unmanaged wrapper to release them from duty of managing this /// resource. /// ////// Critical - as this method returns unmanaged resource to the client. /// [SecurityCritical] internal abstract SecurityCriticalDataForSetGetTextPenaltyResource(); /// /// Client to get a Boolean flag indicating whether the line is truncated in the /// middle of a word. This flag is set only when TextParagraphProperties.TextWrapping /// is set to TextWrapping.Wrap and a single word is longer than the formatting /// paragraph width. In such situation, TextFormatter truncates the line in the middle /// of the word to honor the desired behavior specified by TextWrapping.Wrap setting. /// public abstract bool IsTruncated { get; } #region ITextMetrics ////// Client to get the number of text source positions of this line /// public abstract int Length { get; } ////// Client to get the number of characters following the last character /// of the line that may trigger reformatting of the current line. /// public abstract int DependentLength { get; } ////// Client to get the number of newline characters at line end /// public abstract int NewlineLength { get; } ////// Client to get distance from paragraph start to line start /// public abstract double Start { get; } ////// Client to get the total width of this line /// public abstract double Width { get; } ////// Client to get the total width of this line including width of whitespace characters at the end of the line. /// public abstract double WidthIncludingTrailingWhitespace { get; } ////// Client to get the height of the line /// public abstract double Height { get; } ////// Client to get the height of the text (or other content) in the line; this property may differ from the Height /// property if the client specified the line height /// public abstract double TextHeight { get; } ////// Client to get the distance from top to baseline of this text line /// public abstract double Baseline { get; } ////// Client to get the distance from the top of the text (or other content) to the baseline of this text line; /// this property may differ from the Baseline property if the client specified the line height /// public abstract double TextBaseline { get; } ////// Client to get the distance from the before edge of line height /// to the baseline of marker of the line if any. /// public abstract double MarkerBaseline { get; } ////// Client to get the overall height of the list items marker of the line if any. /// public abstract double MarkerHeight { get; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
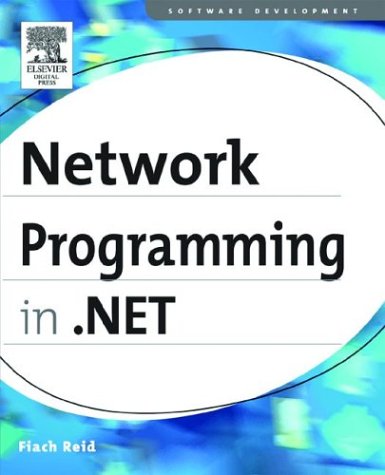
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WaitingCursor.cs
- _FixedSizeReader.cs
- DbParameterCollectionHelper.cs
- XmlReader.cs
- Helpers.cs
- TreeNode.cs
- ClientRolePrincipal.cs
- SendMailErrorEventArgs.cs
- InputLanguageCollection.cs
- ValuePattern.cs
- InvokeWebService.cs
- AxisAngleRotation3D.cs
- WebPartTransformerCollection.cs
- ScriptResourceMapping.cs
- LayoutExceptionEventArgs.cs
- HtmlTextBoxAdapter.cs
- PrinterUnitConvert.cs
- PasswordDeriveBytes.cs
- WasEndpointConfigContainer.cs
- InkPresenter.cs
- ProtocolsConfiguration.cs
- Vector3DKeyFrameCollection.cs
- CodeStatement.cs
- DbConnectionOptions.cs
- SqlRecordBuffer.cs
- ListViewCancelEventArgs.cs
- DBConnection.cs
- BasicHttpSecurityElement.cs
- EpmCustomContentDeSerializer.cs
- Comparer.cs
- Int16AnimationUsingKeyFrames.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- TaskHelper.cs
- SmtpFailedRecipientsException.cs
- ProviderUtil.cs
- AsymmetricAlgorithm.cs
- GPRECTF.cs
- ConsumerConnectionPoint.cs
- ScaleTransform.cs
- Expression.cs
- PlainXmlWriter.cs
- StringFunctions.cs
- AffineTransform3D.cs
- WsdlBuildProvider.cs
- ConnectionPointCookie.cs
- TypeInitializationException.cs
- XsdDuration.cs
- CorrelationManager.cs
- PointIndependentAnimationStorage.cs
- RegexReplacement.cs
- StorageModelBuildProvider.cs
- AnnotationDocumentPaginator.cs
- ResXFileRef.cs
- FillErrorEventArgs.cs
- RuntimeCompatibilityAttribute.cs
- WinEventQueueItem.cs
- LinqDataSourceHelper.cs
- XmlTextReaderImplHelpers.cs
- ServiceModelReg.cs
- HttpModuleAction.cs
- BamlLocalizabilityResolver.cs
- EmptyCollection.cs
- CheckBoxBaseAdapter.cs
- MatrixValueSerializer.cs
- InputMethodStateTypeInfo.cs
- DbConnectionStringCommon.cs
- DataBoundControl.cs
- Matrix3DStack.cs
- ParameterDataSourceExpression.cs
- SimpleWebHandlerParser.cs
- ObjectCache.cs
- NumberAction.cs
- RtfFormatStack.cs
- ObjectViewListener.cs
- StreamDocument.cs
- LocatorManager.cs
- Brush.cs
- NewArrayExpression.cs
- CustomValidator.cs
- DataTableReader.cs
- PlatformNotSupportedException.cs
- Table.cs
- DirectionalLight.cs
- ProcessHostServerConfig.cs
- MulticastDelegate.cs
- CubicEase.cs
- TableChangeProcessor.cs
- EndOfStreamException.cs
- ImageCodecInfo.cs
- ResetableIterator.cs
- CompositionTarget.cs
- PersonalizationAdministration.cs
- FlowLayout.cs
- SimpleApplicationHost.cs
- BindingCompleteEventArgs.cs
- DataMemberFieldConverter.cs
- JsonDeserializer.cs
- AttachmentCollection.cs
- Camera.cs
- SQLSingle.cs