Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / Microsoft / Scripting / Ast / NewArrayExpression.cs / 1305376 / NewArrayExpression.cs
/* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Dynamic.Utils; using System.Runtime.CompilerServices; #if SILVERLIGHT using System.Core; #endif namespace System.Linq.Expressions { ////// Represents creating a new array and possibly initializing the elements of the new array. /// #if !SILVERLIGHT [DebuggerTypeProxy(typeof(Expression.NewArrayExpressionProxy))] #endif public class NewArrayExpression : Expression { private readonly ReadOnlyCollection_expressions; private readonly Type _type; internal NewArrayExpression(Type type, ReadOnlyCollection expressions) { _expressions = expressions; _type = type; } internal static NewArrayExpression Make(ExpressionType nodeType, Type type, ReadOnlyCollection expressions) { if (nodeType == ExpressionType.NewArrayInit) { return new NewArrayInitExpression(type, expressions); } else { return new NewArrayBoundsExpression(type, expressions); } } /// /// Gets the static type of the expression that this ///represents. (Inherited from .) /// The public sealed override Type Type { get { return _type; } } ///that represents the static type of the expression. /// Gets the bounds of the array if the value of the public ReadOnlyCollectionproperty is NewArrayBounds, or the values to initialize the elements of the new array if the value of the property is NewArrayInit. /// Expressions { get { return _expressions; } } /// /// Dispatches to the specific visit method for this node type. /// protected internal override Expression Accept(ExpressionVisitor visitor) { return visitor.VisitNewArray(this); } ////// Creates a new expression that is like this one, but using the /// supplied children. If all of the children are the same, it will /// return this expression. /// /// Theproperty of the result. /// This expression if no children changed, or an expression with the updated children. public NewArrayExpression Update(IEnumerableexpressions) { if (expressions == Expressions) { return this; } if (NodeType == ExpressionType.NewArrayInit) { return Expression.NewArrayInit(Type.GetElementType(), expressions); } return Expression.NewArrayBounds(Type.GetElementType(), expressions); } } internal sealed class NewArrayInitExpression : NewArrayExpression { internal NewArrayInitExpression(Type type, ReadOnlyCollection expressions) : base(type, expressions) { } /// /// Returns the node type of this ///. (Inherited from .) /// The public sealed override ExpressionType NodeType { get { return ExpressionType.NewArrayInit; } } } internal sealed class NewArrayBoundsExpression : NewArrayExpression { internal NewArrayBoundsExpression(Type type, ReadOnlyCollectionthat represents this expression. expressions) : base(type, expressions) { } /// /// Returns the node type of this ///. (Inherited from .) /// The public sealed override ExpressionType NodeType { get { return ExpressionType.NewArrayBounds; } } } public partial class Expression { #region NewArrayInit ///that represents this expression. /// Creates a new array expression of the specified type from the provided initializers. /// /// A Type that represents the element type of the array. /// The expressions used to create the array elements. ///An instance of the public static NewArrayExpression NewArrayInit(Type type, params Expression[] initializers) { return NewArrayInit(type, (IEnumerable. )initializers); } /// /// Creates a new array expression of the specified type from the provided initializers. /// /// A Type that represents the element type of the array. /// The expressions used to create the array elements. ///An instance of the public static NewArrayExpression NewArrayInit(Type type, IEnumerable. initializers) { ContractUtils.RequiresNotNull(type, "type"); ContractUtils.RequiresNotNull(initializers, "initializers"); if (type.Equals(typeof(void))) { throw Error.ArgumentCannotBeOfTypeVoid(); } ReadOnlyCollection initializerList = initializers.ToReadOnly(); Expression[] newList = null; for (int i = 0, n = initializerList.Count; i < n; i++) { Expression expr = initializerList[i]; RequiresCanRead(expr, "initializers"); if (!TypeUtils.AreReferenceAssignable(type, expr.Type)) { if (TypeUtils.IsSameOrSubclass(typeof(LambdaExpression), type) && type.IsAssignableFrom(expr.GetType())) { expr = Expression.Quote(expr); } else { throw Error.ExpressionTypeCannotInitializeArrayType(expr.Type, type); } if (newList == null) { newList = new Expression[initializerList.Count]; for (int j = 0; j < i; j++) { newList[j] = initializerList[j]; } } } if (newList != null) { newList[i] = expr; } } if (newList != null) { initializerList = new TrueReadOnlyCollection (newList); } return NewArrayExpression.Make(ExpressionType.NewArrayInit, type.MakeArrayType(), initializerList); } #endregion #region NewArrayBounds /// /// Creates a /// Athat represents creating an array that has a specified rank. /// that represents the element type of the array. /// An array that contains Expression objects to use to populate the Expressions collection. /// A public static NewArrayExpression NewArrayBounds(Type type, params Expression[] bounds) { return NewArrayBounds(type, (IEnumerablethat has the property equal to type and the property set to the specified value. )bounds); } /// /// Creates a /// Athat represents creating an array that has a specified rank. /// that represents the element type of the array. /// An IEnumerable{T} that contains Expression objects to use to populate the Expressions collection. /// A public static NewArrayExpression NewArrayBounds(Type type, IEnumerablethat has the property equal to type and the property set to the specified value. bounds) { ContractUtils.RequiresNotNull(type, "type"); ContractUtils.RequiresNotNull(bounds, "bounds"); if (type.Equals(typeof(void))) { throw Error.ArgumentCannotBeOfTypeVoid(); } ReadOnlyCollection boundsList = bounds.ToReadOnly(); int dimensions = boundsList.Count; if (dimensions <= 0) throw Error.BoundsCannotBeLessThanOne(); for (int i = 0; i < dimensions; i++) { Expression expr = boundsList[i]; RequiresCanRead(expr, "bounds"); if (!TypeUtils.IsInteger(expr.Type)) { throw Error.ArgumentMustBeInteger(); } } Type arrayType; if (dimensions == 1) { //To get a vector, need call Type.MakeArrayType(). //Type.MakeArrayType(1) gives a non-vector array, which will cause type check error. arrayType = type.MakeArrayType(); } else { arrayType = type.MakeArrayType(dimensions); } return NewArrayExpression.Make(ExpressionType.NewArrayBounds, arrayType, bounds.ToReadOnly()); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
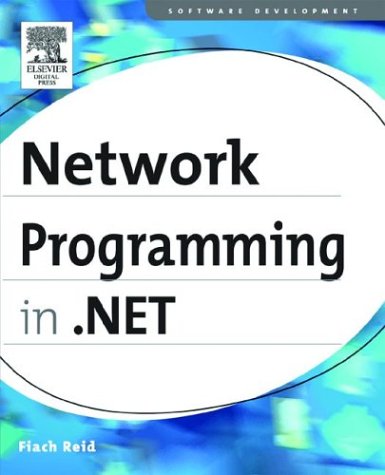
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DependencyPropertyDescriptor.cs
- SqlTypesSchemaImporter.cs
- EdmRelationshipNavigationPropertyAttribute.cs
- WindowsTab.cs
- CachedBitmap.cs
- ScriptMethodAttribute.cs
- DataSourceControlBuilder.cs
- SqlGenericUtil.cs
- RegexMatch.cs
- TabControlAutomationPeer.cs
- PageBuildProvider.cs
- ConfigurationPermission.cs
- StylusPlugInCollection.cs
- PromptStyle.cs
- SplineQuaternionKeyFrame.cs
- FactoryRecord.cs
- SQLDouble.cs
- ResourceReferenceExpressionConverter.cs
- ToolboxItemFilterAttribute.cs
- DatagramAdapter.cs
- ActionItem.cs
- QueryOperationResponseOfT.cs
- TableCellCollection.cs
- QueryStringHandler.cs
- TiffBitmapEncoder.cs
- NativeRightsManagementAPIsStructures.cs
- ServiceEndpointElementCollection.cs
- PropertyTabAttribute.cs
- PerspectiveCamera.cs
- Property.cs
- XmlSchemaValidator.cs
- CacheMemory.cs
- CapabilitiesPattern.cs
- ConfigurationSettings.cs
- _ListenerRequestStream.cs
- KeyTimeConverter.cs
- QueueNameHelper.cs
- XamlContextStack.cs
- CompilerParameters.cs
- SerializationUtilities.cs
- WindowsRegion.cs
- Point3DKeyFrameCollection.cs
- ItemsControlAutomationPeer.cs
- NameValuePermission.cs
- HTMLTagNameToTypeMapper.cs
- GetPageNumberCompletedEventArgs.cs
- ListViewDataItem.cs
- TreeNodeStyle.cs
- RawAppCommandInputReport.cs
- DesignColumnCollection.cs
- ValuePattern.cs
- DataGridViewToolTip.cs
- BookmarkNameHelper.cs
- NodeInfo.cs
- RemoveStoryboard.cs
- RootDesignerSerializerAttribute.cs
- RayMeshGeometry3DHitTestResult.cs
- CaseInsensitiveHashCodeProvider.cs
- BasePattern.cs
- SqlBulkCopyColumnMapping.cs
- CheckBoxBaseAdapter.cs
- SystemIPGlobalStatistics.cs
- QilName.cs
- DiscoveryClientDuplexChannel.cs
- FrugalList.cs
- TextContainerChangedEventArgs.cs
- GridPattern.cs
- AuthenticationServiceManager.cs
- EditorAttributeInfo.cs
- SqlCaseSimplifier.cs
- RadioButtonList.cs
- AppDomainUnloadedException.cs
- ImportContext.cs
- WebConfigurationHost.cs
- Int32CollectionConverter.cs
- CharEnumerator.cs
- OAVariantLib.cs
- ElementUtil.cs
- LookupBindingPropertiesAttribute.cs
- ValidationError.cs
- ScrollProperties.cs
- RuntimeCompatibilityAttribute.cs
- Int64KeyFrameCollection.cs
- TcpClientSocketManager.cs
- DataGridAddNewRow.cs
- MulticastOption.cs
- UnsafeNativeMethods.cs
- COMException.cs
- SspiSafeHandles.cs
- PrintPageEvent.cs
- MimeParameterWriter.cs
- PostBackTrigger.cs
- WebPartHeaderCloseVerb.cs
- KoreanCalendar.cs
- GeometryDrawing.cs
- HWStack.cs
- CookieProtection.cs
- AutomationPropertyInfo.cs
- parserscommon.cs
- HMACMD5.cs