Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / XmlUtils / System / Xml / Xsl / QIL / QilName.cs / 1 / QilName.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Diagnostics; namespace System.Xml.Xsl.Qil { ////// View over a Qil name literal. /// ////// Don't construct QIL nodes directly; instead, use the internal class QilName : QilLiteral { private string local; private string uri; private string prefix; //----------------------------------------------- // Constructor //----------------------------------------------- ///QilFactory . ////// Construct a new node /// public QilName(QilNodeType nodeType, string local, string uri, string prefix) : base(nodeType, null) { LocalName = local; NamespaceUri = uri; Prefix = prefix; Value = this; } //----------------------------------------------- // QilName methods //----------------------------------------------- public string LocalName { get { return this.local; } set { this.local = value; } } public string NamespaceUri { get { return this.uri; } set { this.uri = value; } } public string Prefix { get { return this.prefix; } set { this.prefix = value; } } ////// Build the qualified name in the form prefix:local /// public string QualifiedName { get { if (this.prefix.Length == 0) { return this.local; } else { return this.prefix + ':' + this.local; } } } ////// Override GetHashCode() so that the QilName can be used as a key in the hashtable. /// ///Does not compare their prefixes (if any). public override int GetHashCode() { return this.local.GetHashCode(); } ////// Override Equals() so that the QilName can be used as a key in the hashtable. /// ///Does not compare their prefixes (if any). public override bool Equals(object other) { QilName name = other as QilName; if (name == null) return false; return this.local == name.local && this.uri == name.uri; } ////// Return the QilName in this format: "{namespace}prefix:local-name". /// If the namespace is empty, return the QilName in this truncated format: "local-name". /// If the prefix is empty, return the QilName in this truncated format: "{namespace}local-name". /// public override string ToString() { if (prefix.Length == 0) { if (uri.Length == 0) return local; return string.Concat("{", uri, "}", local); } return string.Concat("{", uri, "}", prefix, ":", local); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Diagnostics; namespace System.Xml.Xsl.Qil { ////// View over a Qil name literal. /// ////// Don't construct QIL nodes directly; instead, use the internal class QilName : QilLiteral { private string local; private string uri; private string prefix; //----------------------------------------------- // Constructor //----------------------------------------------- ///QilFactory . ////// Construct a new node /// public QilName(QilNodeType nodeType, string local, string uri, string prefix) : base(nodeType, null) { LocalName = local; NamespaceUri = uri; Prefix = prefix; Value = this; } //----------------------------------------------- // QilName methods //----------------------------------------------- public string LocalName { get { return this.local; } set { this.local = value; } } public string NamespaceUri { get { return this.uri; } set { this.uri = value; } } public string Prefix { get { return this.prefix; } set { this.prefix = value; } } ////// Build the qualified name in the form prefix:local /// public string QualifiedName { get { if (this.prefix.Length == 0) { return this.local; } else { return this.prefix + ':' + this.local; } } } ////// Override GetHashCode() so that the QilName can be used as a key in the hashtable. /// ///Does not compare their prefixes (if any). public override int GetHashCode() { return this.local.GetHashCode(); } ////// Override Equals() so that the QilName can be used as a key in the hashtable. /// ///Does not compare their prefixes (if any). public override bool Equals(object other) { QilName name = other as QilName; if (name == null) return false; return this.local == name.local && this.uri == name.uri; } ////// Return the QilName in this format: "{namespace}prefix:local-name". /// If the namespace is empty, return the QilName in this truncated format: "local-name". /// If the prefix is empty, return the QilName in this truncated format: "{namespace}local-name". /// public override string ToString() { if (prefix.Length == 0) { if (uri.Length == 0) return local; return string.Concat("{", uri, "}", local); } return string.Concat("{", uri, "}", prefix, ":", local); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
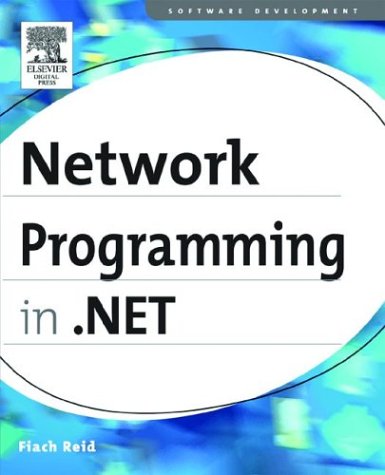
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ErrorEventArgs.cs
- ResourceDescriptionAttribute.cs
- EventMappingSettings.cs
- BamlRecordHelper.cs
- CodeCommentStatementCollection.cs
- _RequestCacheProtocol.cs
- MenuItem.cs
- XmlSchemaObjectCollection.cs
- GeometryConverter.cs
- FormViewUpdateEventArgs.cs
- ConnectionStringSettings.cs
- TextEditorThreadLocalStore.cs
- MultiPageTextView.cs
- ErrorStyle.cs
- EventLogger.cs
- OracleBinary.cs
- DbDataSourceEnumerator.cs
- MatrixConverter.cs
- AggregateException.cs
- DesignerWidgets.cs
- DbParameterCollectionHelper.cs
- XmlSerializerAssemblyAttribute.cs
- RectangleHotSpot.cs
- PartitionResolver.cs
- safex509handles.cs
- InvalidComObjectException.cs
- SettingsAttributes.cs
- SqlClientPermission.cs
- MediaTimeline.cs
- SelectorItemAutomationPeer.cs
- RegexNode.cs
- AdornerPresentationContext.cs
- CopyOnWriteList.cs
- MailBnfHelper.cs
- LinqDataSourceDisposeEventArgs.cs
- SHA512Managed.cs
- DtcInterfaces.cs
- EditorZone.cs
- PathGeometry.cs
- CacheEntry.cs
- ValidationRule.cs
- InfoCardService.cs
- HttpCookieCollection.cs
- SqlDataSourceSelectingEventArgs.cs
- SystemIPInterfaceProperties.cs
- StandardOleMarshalObject.cs
- SystemIcons.cs
- sqlstateclientmanager.cs
- ConfigXmlText.cs
- OleDbWrapper.cs
- URI.cs
- CssStyleCollection.cs
- PerspectiveCamera.cs
- WSFederationHttpSecurityMode.cs
- XhtmlTextWriter.cs
- precedingsibling.cs
- KeysConverter.cs
- TouchFrameEventArgs.cs
- SvcMapFileLoader.cs
- StyleModeStack.cs
- AxHost.cs
- SecUtil.cs
- CodeLinePragma.cs
- TraceHandlerErrorFormatter.cs
- LogicalExpressionEditor.cs
- FillBehavior.cs
- ObjectDataSourceSelectingEventArgs.cs
- HasCopySemanticsAttribute.cs
- ContentTextAutomationPeer.cs
- ValidatorCompatibilityHelper.cs
- HuffCodec.cs
- StyleXamlParser.cs
- MSHTMLHostUtil.cs
- BufferedGraphicsContext.cs
- ValidationHelper.cs
- DataRelationCollection.cs
- FullTextState.cs
- AlphabeticalEnumConverter.cs
- UTF32Encoding.cs
- DataTable.cs
- EventLogPermissionHolder.cs
- ToolStripRenderEventArgs.cs
- EntityConnectionStringBuilderItem.cs
- AsyncResult.cs
- DesignerOptionService.cs
- TreeNodeCollection.cs
- AbandonedMutexException.cs
- CompositeFontInfo.cs
- UnicastIPAddressInformationCollection.cs
- ObservableCollectionDefaultValueFactory.cs
- NativeWrapper.cs
- LicenseException.cs
- DataContext.cs
- ToolStripItemImageRenderEventArgs.cs
- HttpApplication.cs
- StoryFragments.cs
- IsolatedStorageFileStream.cs
- AvTrace.cs
- MobileSysDescriptionAttribute.cs
- TextServicesDisplayAttributePropertyRanges.cs