Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Xml / System / Xml / XPath / Internal / precedingsibling.cs / 1 / precedingsibling.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.Collections.Generic; // This class can be rewritten much more efficient. // Algorithm could be like one for FollowingSibling: // - Build InputArrays: pares (first, sentinel) // -- Cash all input nodes as sentinel // -- Add firts node of its parent for each input node. // -- Sort these pares by first nodes. // - Advance algorithm will look like: // -- For each row in InputArays we will output first node + all its following nodes which are < sentinel // -- Before outputing each node in row #I we will check that it is < first node in row #I+1 // --- if true we actualy output it // --- if false, we hold with row #I and apply this algorith starting for row #I+1 // --- when we done with #I+1 we continue with row #I internal class PreSiblingQuery : CacheAxisQuery { public PreSiblingQuery(Query qyInput, string name, string prefix, XPathNodeType typeTest) : base (qyInput, name, prefix, typeTest) {} protected PreSiblingQuery(PreSiblingQuery other) : base(other) {} private bool NotVisited(XPathNavigator nav, ListparentStk){ XPathNavigator nav1 = nav.Clone(); nav1.MoveToParent(); for (int i = 0; i < parentStk.Count; i++) { if (nav1.IsSamePosition(parentStk[i])) { return false; } } parentStk.Add(nav1); return true; } public override object Evaluate(XPathNodeIterator context) { base.Evaluate(context); // Fill up base.outputBuffer List parentStk = new List (); Stack inputStk = new Stack (); while ((currentNode = qyInput.Advance()) != null) { inputStk.Push(currentNode.Clone()); } while (inputStk.Count != 0) { XPathNavigator input = inputStk.Pop(); if (input.NodeType == XPathNodeType.Attribute || input.NodeType == XPathNodeType.Namespace) { continue; } if (NotVisited(input, parentStk)) { XPathNavigator prev = input.Clone(); if (prev.MoveToParent()) { bool test = prev.MoveToFirstChild(); Debug.Assert(test, "We just moved to parent, how we can not have first child?"); while (!prev.IsSamePosition(input)) { if (matches(prev)) { Insert(outputBuffer, prev); } if (!prev.MoveToNext()) { Debug.Fail("We managed to miss sentinel node (input)"); break; } } } } } return this; } public override XPathNodeIterator Clone() { return new PreSiblingQuery(this); } public override QueryProps Properties { get { return base.Properties | QueryProps.Reverse; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.Collections.Generic; // This class can be rewritten much more efficient. // Algorithm could be like one for FollowingSibling: // - Build InputArrays: pares (first, sentinel) // -- Cash all input nodes as sentinel // -- Add firts node of its parent for each input node. // -- Sort these pares by first nodes. // - Advance algorithm will look like: // -- For each row in InputArays we will output first node + all its following nodes which are < sentinel // -- Before outputing each node in row #I we will check that it is < first node in row #I+1 // --- if true we actualy output it // --- if false, we hold with row #I and apply this algorith starting for row #I+1 // --- when we done with #I+1 we continue with row #I internal class PreSiblingQuery : CacheAxisQuery { public PreSiblingQuery(Query qyInput, string name, string prefix, XPathNodeType typeTest) : base (qyInput, name, prefix, typeTest) {} protected PreSiblingQuery(PreSiblingQuery other) : base(other) {} private bool NotVisited(XPathNavigator nav, ListparentStk){ XPathNavigator nav1 = nav.Clone(); nav1.MoveToParent(); for (int i = 0; i < parentStk.Count; i++) { if (nav1.IsSamePosition(parentStk[i])) { return false; } } parentStk.Add(nav1); return true; } public override object Evaluate(XPathNodeIterator context) { base.Evaluate(context); // Fill up base.outputBuffer List parentStk = new List (); Stack inputStk = new Stack (); while ((currentNode = qyInput.Advance()) != null) { inputStk.Push(currentNode.Clone()); } while (inputStk.Count != 0) { XPathNavigator input = inputStk.Pop(); if (input.NodeType == XPathNodeType.Attribute || input.NodeType == XPathNodeType.Namespace) { continue; } if (NotVisited(input, parentStk)) { XPathNavigator prev = input.Clone(); if (prev.MoveToParent()) { bool test = prev.MoveToFirstChild(); Debug.Assert(test, "We just moved to parent, how we can not have first child?"); while (!prev.IsSamePosition(input)) { if (matches(prev)) { Insert(outputBuffer, prev); } if (!prev.MoveToNext()) { Debug.Fail("We managed to miss sentinel node (input)"); break; } } } } } return this; } public override XPathNodeIterator Clone() { return new PreSiblingQuery(this); } public override QueryProps Properties { get { return base.Properties | QueryProps.Reverse; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
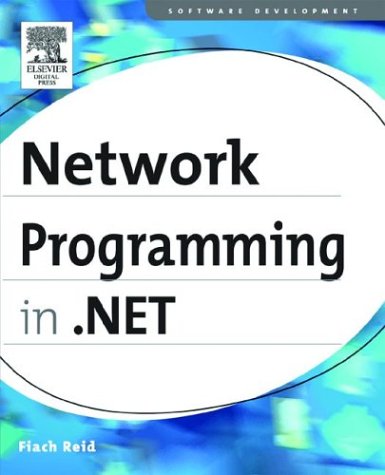
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DetailsViewPageEventArgs.cs
- DocumentEventArgs.cs
- JsonCollectionDataContract.cs
- SchemaImporterExtensionsSection.cs
- ExtensionFile.cs
- controlskin.cs
- XamlFigureLengthSerializer.cs
- ColorMap.cs
- ProfileSettingsCollection.cs
- AsmxEndpointPickerExtension.cs
- ImportStoreException.cs
- QuadraticEase.cs
- ValidationRule.cs
- XD.cs
- MessageEncoder.cs
- WriteFileContext.cs
- ApplicationDirectoryMembershipCondition.cs
- AutomationEvent.cs
- CodeTypeReference.cs
- WebPartDisplayMode.cs
- TemplateBindingExpression.cs
- XmlSchemaAnnotation.cs
- SizeIndependentAnimationStorage.cs
- ZipFileInfoCollection.cs
- EventNotify.cs
- ConnectionPoolManager.cs
- DataRecordInternal.cs
- StatusInfoItem.cs
- AutomationPropertyInfo.cs
- WebPart.cs
- KeyboardNavigation.cs
- XamlReaderHelper.cs
- ContentTextAutomationPeer.cs
- VisualBasicDesignerHelper.cs
- JapaneseLunisolarCalendar.cs
- KnownAssembliesSet.cs
- ToolboxItemSnapLineBehavior.cs
- SortDescription.cs
- XPathPatternBuilder.cs
- TextTreePropertyUndoUnit.cs
- ChtmlLinkAdapter.cs
- GridEntryCollection.cs
- FrameworkTemplate.cs
- ChannelServices.cs
- ContentHostHelper.cs
- CharConverter.cs
- EtwProvider.cs
- NamespaceMapping.cs
- Evaluator.cs
- UnsupportedPolicyOptionsException.cs
- _LocalDataStore.cs
- DbUpdateCommandTree.cs
- AdornerDecorator.cs
- PlacementWorkspace.cs
- LayoutEvent.cs
- LinqDataSourceHelper.cs
- Native.cs
- StringConverter.cs
- XmlSchemaRedefine.cs
- _Win32.cs
- GeometryModel3D.cs
- IsolatedStoragePermission.cs
- KeysConverter.cs
- HttpServerVarsCollection.cs
- OverrideMode.cs
- ProviderMetadata.cs
- SByteConverter.cs
- TypefaceMap.cs
- ResourcesBuildProvider.cs
- PrefixQName.cs
- XamlSerializerUtil.cs
- WorkflowRuntimeSection.cs
- CodeParameterDeclarationExpression.cs
- RemoteWebConfigurationHost.cs
- SqlDependencyUtils.cs
- UIElement.cs
- assemblycache.cs
- FileSystemEventArgs.cs
- Base64Decoder.cs
- SynchronizationHandlesCodeDomSerializer.cs
- WebFaultException.cs
- TextUtf8RawTextWriter.cs
- DesigntimeLicenseContextSerializer.cs
- WebResourceUtil.cs
- WebPartEditVerb.cs
- OdbcError.cs
- SetIterators.cs
- TypeDelegator.cs
- GeneralTransform2DTo3DTo2D.cs
- AnnotationObservableCollection.cs
- TdsParser.cs
- DataAdapter.cs
- IndexingContentUnit.cs
- SchemaTableOptionalColumn.cs
- Msmq4SubqueuePoisonHandler.cs
- ElapsedEventArgs.cs
- FrameworkElement.cs
- MenuItem.cs
- WorkflowMarkupElementEventArgs.cs
- GenericUriParser.cs