Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Map / Update / Internal / PropagatorResult.cs / 2 / PropagatorResult.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Metadata.Edm; using System.Collections.Generic; using System.Globalization; using System.Diagnostics; using System.Data.Common.Utils; using System.Data.Objects; using System.Collections; using System.Data.Common; using System.Text; namespace System.Data.Mapping.Update.Internal { ////// requires: for structural types, member values are ordinally aligned with the members of the /// structural type. /// /// Stores a 'row' (or element within a row) being propagated through the update pipeline, including /// markup information and metadata. Internally, we maintain several different classes so that we only /// store the necessary state. /// /// - StructuralValue (complex types, entities, and association end keys): type and member values, /// one version for modified structural values and one version for unmodified structural values /// (a structural type is modified if its _type_ is changed, not its values /// - SimpleValue (scalar value): flags to describe the state of the value (is it a concurrency value, /// is it modified) and the value itself /// - ServerGenSimpleValue: adds back-prop information to the above (record and position in record /// so that we can set the value on back-prop) /// - KeyValue: the originating IEntityStateEntry also travels with keys. These entries are used purely for /// error reporting. We send them with keys so that every row containing an entity (which must also /// contain the key) has enough context to recover the state entry. /// ////// Not all memebers of a PropagatorResult are available for all specializations. For instance, GetSimpleValue /// is available only on simple types /// internal abstract class PropagatorResult { #region Constructors // private constructor: only nested classes may derive from propagator result private PropagatorResult() { } #endregion #region Fields internal const long NullIdentifier = -1; internal const int NullOrdinal = -1; #endregion #region Properties ////// Gets a value indicating whether this result is null. /// internal abstract bool IsNull { get; } ////// Gets a value indicating whether this is a simple (scalar) or complex /// structural) result. /// internal abstract bool IsSimple { get; } ////// Gets flags describing the behaviors for this element. /// internal virtual PropagatorFlags PropagatorFlags { get { return PropagatorFlags.NoFlags; } } ////// Gets all state entries from which this result originated. Only set for key /// values (to ensure every row knows all of its source entries) /// internal virtual IEntityStateEntry StateEntry { get { return null; } } ////// Gets record from which this result originated. Only set for server generated /// results (where the record needs to be synchronized). /// internal virtual CurrentValueRecord Record { get { return null; } } ////// Gets structural type for non simple results. Only available for entity and complex type /// results. /// internal virtual StructuralType StructuralType { get { return null; } } ////// Gets the ordinal within the originating record for this result. Only set /// for server generated results (otherwise, returns -1) /// internal virtual int RecordOrdinal { get { return NullOrdinal; } } ////// Gets the identifier for this entry if it is a server-gen key value (otherwise /// returns -1) /// internal virtual long Identifier { get { return NullIdentifier; } } #endregion #region Methods ////// Returns simple value stored in this result. Only valid when /// ///is /// true. /// Concrete value. internal virtual object GetSimpleValue() { throw EntityUtil.InternalError(EntityUtil.InternalErrorCode.UpdatePipelineResultRequestInvalid, 0, "PropagatorResult.GetSimpleValue"); } ////// Returns nested value. Only valid when /// Ordinal of value to return (ordinal based on type definition) ///is false. /// Nested result. internal virtual PropagatorResult GetMemberValue(int ordinal) { throw EntityUtil.InternalError(EntityUtil.InternalErrorCode.UpdatePipelineResultRequestInvalid, 0, "PropagatorResult.GetMemberValue"); } ////// Returns nested value. Only valid when /// Member for which to return a value ///is false. /// Nested result. internal PropagatorResult GetMemberValue(EdmMember member) { int ordinal = TypeHelpers.GetAllStructuralMembers(this.StructuralType).IndexOf(member); return GetMemberValue(ordinal); } ////// Returns all structural values. Only valid when ///is false. /// Values of all structural members. internal virtual PropagatorResult[] GetMemberValues() { throw EntityUtil.InternalError(EntityUtil.InternalErrorCode.UpdatePipelineResultRequestInvalid, 0, "PropagatorResult.GetMembersValues"); } ////// Produces a replica of this propagator result with different flags. /// /// New flags for the result. ///This result with the given flags. internal abstract PropagatorResult ReplicateResultWithNewFlags(PropagatorFlags flags); ////// Copies this result replacing its value. Used for cast. Requires a simple result. /// /// New value for result ///Copy of this result with new value. internal virtual PropagatorResult ReplicateResultWithNewValue(object value) { throw EntityUtil.InternalError(EntityUtil.InternalErrorCode.UpdatePipelineResultRequestInvalid, 0, "PropagatorResult.ReplicateResultWithNewValue"); } #if DEBUG public override string ToString() { StringBuilder builder = new StringBuilder(); if (PropagatorFlags.NoFlags != PropagatorFlags) { builder.Append(PropagatorFlags.ToString()).Append(":"); } if (NullIdentifier != Identifier) { builder.Append("id").Append(Identifier.ToString(CultureInfo.InvariantCulture)).Append(":"); } if (NullOrdinal != RecordOrdinal) { builder.Append("ord").Append(RecordOrdinal.ToString(CultureInfo.InvariantCulture)).Append(":"); } if (IsSimple) { builder.AppendFormat(CultureInfo.InvariantCulture, "{0}", GetSimpleValue()); } else { if (!Helper.IsRowType(StructuralType)) { builder.Append(StructuralType.Name).Append(":"); } builder.Append("{"); bool first = true; foreach (KeyValuePairmemberValue in Helper.PairEnumerations( TypeHelpers.GetAllStructuralMembers(this.StructuralType), GetMemberValues())) { if (first) { first = false; } else { builder.Append(", "); } builder.Append(memberValue.Key.Name).Append("=").Append(memberValue.Value.ToString()); } builder.Append("}"); } return builder.ToString(); } #endif #endregion #region Nested types and factory methods internal static PropagatorResult CreateSimpleValue(PropagatorFlags flags, object value) { return new SimpleValue(flags, value); } private class SimpleValue : PropagatorResult { internal SimpleValue(PropagatorFlags flags, object value) { m_flags = flags; m_value = value ?? DBNull.Value; } private readonly PropagatorFlags m_flags; protected readonly object m_value; internal override PropagatorFlags PropagatorFlags { get { return m_flags; } } internal override bool IsSimple { get { return true; } } internal override bool IsNull { get { return DBNull.Value == m_value; } } internal override object GetSimpleValue() { return m_value; } internal override PropagatorResult ReplicateResultWithNewFlags(PropagatorFlags flags) { return new SimpleValue(flags, m_value); } internal override PropagatorResult ReplicateResultWithNewValue(object value) { return new SimpleValue(PropagatorFlags, value); } } internal static PropagatorResult CreateServerGenSimpleValue(PropagatorFlags flags, object value, CurrentValueRecord record, int recordOrdinal) { return new ServerGenSimpleValue(flags, value, record, recordOrdinal); } private class ServerGenSimpleValue : SimpleValue { internal ServerGenSimpleValue(PropagatorFlags flags, object value, CurrentValueRecord record, int recordOrdinal) : base(flags, value) { Debug.Assert(null != record); m_record = record; m_recordOrdinal = recordOrdinal; } private readonly CurrentValueRecord m_record; private readonly int m_recordOrdinal; internal override CurrentValueRecord Record { get { return m_record; } } internal override int RecordOrdinal { get { return m_recordOrdinal; } } internal override PropagatorResult ReplicateResultWithNewFlags(PropagatorFlags flags) { return new ServerGenSimpleValue(flags, m_value, Record, RecordOrdinal); } internal override PropagatorResult ReplicateResultWithNewValue(object value) { return new ServerGenSimpleValue(PropagatorFlags, value, Record, RecordOrdinal); } } internal static PropagatorResult CreateKeyValue(PropagatorFlags flags, object value, IEntityStateEntry stateEntry, long identifier) { return new KeyValue(flags, value, stateEntry, identifier); } private class KeyValue : SimpleValue { internal KeyValue(PropagatorFlags flags, object value, IEntityStateEntry stateEntry, long identifier) : base(flags, value) { Debug.Assert(null != stateEntry); m_stateEntry = stateEntry; m_identifier = identifier; } private readonly IEntityStateEntry m_stateEntry; private readonly long m_identifier; internal override IEntityStateEntry StateEntry { get { return m_stateEntry; } } internal override long Identifier { get { return m_identifier; } } internal override CurrentValueRecord Record { get { // delegate to the state entry, which also has the record return m_stateEntry.CurrentValues; } } internal override PropagatorResult ReplicateResultWithNewFlags(PropagatorFlags flags) { return new KeyValue(flags, m_value, StateEntry, Identifier); } internal override PropagatorResult ReplicateResultWithNewValue(object value) { return new KeyValue(PropagatorFlags, value, StateEntry, Identifier); } } internal static PropagatorResult CreateServerGenKeyValue(PropagatorFlags flags, object value, IEntityStateEntry stateEntry, long identifier, int recordOrdinal) { return new ServerGenKeyValue(flags, value, stateEntry, identifier, recordOrdinal); } private class ServerGenKeyValue : KeyValue { internal ServerGenKeyValue(PropagatorFlags flags, object value, IEntityStateEntry stateEntry, long identifier, int recordOrdinal) : base(flags, value, stateEntry, identifier) { m_recordOrdinal = recordOrdinal; } private readonly int m_recordOrdinal; internal override int RecordOrdinal { get { return m_recordOrdinal; } } internal override PropagatorResult ReplicateResultWithNewFlags(PropagatorFlags flags) { return new ServerGenKeyValue(flags, m_value, StateEntry, Identifier, RecordOrdinal); } internal override PropagatorResult ReplicateResultWithNewValue(object value) { return new ServerGenKeyValue(PropagatorFlags, value, StateEntry, Identifier, RecordOrdinal); } } internal static PropagatorResult CreateStructuralValue(PropagatorResult[] values, StructuralType structuralType, bool isModified) { if (isModified) { return new StructuralValue(values, structuralType); } else { return new UnmodifiedStructuralValue(values, structuralType); } } private class StructuralValue : PropagatorResult { internal StructuralValue(PropagatorResult[] values, StructuralType structuralType) { Debug.Assert(null != structuralType); Debug.Assert(null != values); Debug.Assert(values.Length == TypeHelpers.GetAllStructuralMembers(structuralType).Count); m_values = values; m_structuralType = structuralType; } private readonly PropagatorResult[] m_values; private readonly StructuralType m_structuralType; internal override bool IsSimple { get { return false; } } internal override bool IsNull { get { return false; } } internal override StructuralType StructuralType { get { return m_structuralType; } } internal override PropagatorResult GetMemberValue(int ordinal) { return m_values[ordinal]; } internal override PropagatorResult[] GetMemberValues() { return m_values; } internal override PropagatorResult ReplicateResultWithNewFlags(PropagatorFlags flags) { throw EntityUtil.InternalError(EntityUtil.InternalErrorCode.UpdatePipelineResultRequestInvalid, 0, "StructuralValue.ReplicateResultWithNewFlags"); } } private class UnmodifiedStructuralValue : StructuralValue { internal UnmodifiedStructuralValue(PropagatorResult[] values, StructuralType structuralType) : base (values, structuralType) { } internal override PropagatorFlags PropagatorFlags { get { return PropagatorFlags.Preserve; } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Metadata.Edm; using System.Collections.Generic; using System.Globalization; using System.Diagnostics; using System.Data.Common.Utils; using System.Data.Objects; using System.Collections; using System.Data.Common; using System.Text; namespace System.Data.Mapping.Update.Internal { ////// requires: for structural types, member values are ordinally aligned with the members of the /// structural type. /// /// Stores a 'row' (or element within a row) being propagated through the update pipeline, including /// markup information and metadata. Internally, we maintain several different classes so that we only /// store the necessary state. /// /// - StructuralValue (complex types, entities, and association end keys): type and member values, /// one version for modified structural values and one version for unmodified structural values /// (a structural type is modified if its _type_ is changed, not its values /// - SimpleValue (scalar value): flags to describe the state of the value (is it a concurrency value, /// is it modified) and the value itself /// - ServerGenSimpleValue: adds back-prop information to the above (record and position in record /// so that we can set the value on back-prop) /// - KeyValue: the originating IEntityStateEntry also travels with keys. These entries are used purely for /// error reporting. We send them with keys so that every row containing an entity (which must also /// contain the key) has enough context to recover the state entry. /// ////// Not all memebers of a PropagatorResult are available for all specializations. For instance, GetSimpleValue /// is available only on simple types /// internal abstract class PropagatorResult { #region Constructors // private constructor: only nested classes may derive from propagator result private PropagatorResult() { } #endregion #region Fields internal const long NullIdentifier = -1; internal const int NullOrdinal = -1; #endregion #region Properties ////// Gets a value indicating whether this result is null. /// internal abstract bool IsNull { get; } ////// Gets a value indicating whether this is a simple (scalar) or complex /// structural) result. /// internal abstract bool IsSimple { get; } ////// Gets flags describing the behaviors for this element. /// internal virtual PropagatorFlags PropagatorFlags { get { return PropagatorFlags.NoFlags; } } ////// Gets all state entries from which this result originated. Only set for key /// values (to ensure every row knows all of its source entries) /// internal virtual IEntityStateEntry StateEntry { get { return null; } } ////// Gets record from which this result originated. Only set for server generated /// results (where the record needs to be synchronized). /// internal virtual CurrentValueRecord Record { get { return null; } } ////// Gets structural type for non simple results. Only available for entity and complex type /// results. /// internal virtual StructuralType StructuralType { get { return null; } } ////// Gets the ordinal within the originating record for this result. Only set /// for server generated results (otherwise, returns -1) /// internal virtual int RecordOrdinal { get { return NullOrdinal; } } ////// Gets the identifier for this entry if it is a server-gen key value (otherwise /// returns -1) /// internal virtual long Identifier { get { return NullIdentifier; } } #endregion #region Methods ////// Returns simple value stored in this result. Only valid when /// ///is /// true. /// Concrete value. internal virtual object GetSimpleValue() { throw EntityUtil.InternalError(EntityUtil.InternalErrorCode.UpdatePipelineResultRequestInvalid, 0, "PropagatorResult.GetSimpleValue"); } ////// Returns nested value. Only valid when /// Ordinal of value to return (ordinal based on type definition) ///is false. /// Nested result. internal virtual PropagatorResult GetMemberValue(int ordinal) { throw EntityUtil.InternalError(EntityUtil.InternalErrorCode.UpdatePipelineResultRequestInvalid, 0, "PropagatorResult.GetMemberValue"); } ////// Returns nested value. Only valid when /// Member for which to return a value ///is false. /// Nested result. internal PropagatorResult GetMemberValue(EdmMember member) { int ordinal = TypeHelpers.GetAllStructuralMembers(this.StructuralType).IndexOf(member); return GetMemberValue(ordinal); } ////// Returns all structural values. Only valid when ///is false. /// Values of all structural members. internal virtual PropagatorResult[] GetMemberValues() { throw EntityUtil.InternalError(EntityUtil.InternalErrorCode.UpdatePipelineResultRequestInvalid, 0, "PropagatorResult.GetMembersValues"); } ////// Produces a replica of this propagator result with different flags. /// /// New flags for the result. ///This result with the given flags. internal abstract PropagatorResult ReplicateResultWithNewFlags(PropagatorFlags flags); ////// Copies this result replacing its value. Used for cast. Requires a simple result. /// /// New value for result ///Copy of this result with new value. internal virtual PropagatorResult ReplicateResultWithNewValue(object value) { throw EntityUtil.InternalError(EntityUtil.InternalErrorCode.UpdatePipelineResultRequestInvalid, 0, "PropagatorResult.ReplicateResultWithNewValue"); } #if DEBUG public override string ToString() { StringBuilder builder = new StringBuilder(); if (PropagatorFlags.NoFlags != PropagatorFlags) { builder.Append(PropagatorFlags.ToString()).Append(":"); } if (NullIdentifier != Identifier) { builder.Append("id").Append(Identifier.ToString(CultureInfo.InvariantCulture)).Append(":"); } if (NullOrdinal != RecordOrdinal) { builder.Append("ord").Append(RecordOrdinal.ToString(CultureInfo.InvariantCulture)).Append(":"); } if (IsSimple) { builder.AppendFormat(CultureInfo.InvariantCulture, "{0}", GetSimpleValue()); } else { if (!Helper.IsRowType(StructuralType)) { builder.Append(StructuralType.Name).Append(":"); } builder.Append("{"); bool first = true; foreach (KeyValuePairmemberValue in Helper.PairEnumerations( TypeHelpers.GetAllStructuralMembers(this.StructuralType), GetMemberValues())) { if (first) { first = false; } else { builder.Append(", "); } builder.Append(memberValue.Key.Name).Append("=").Append(memberValue.Value.ToString()); } builder.Append("}"); } return builder.ToString(); } #endif #endregion #region Nested types and factory methods internal static PropagatorResult CreateSimpleValue(PropagatorFlags flags, object value) { return new SimpleValue(flags, value); } private class SimpleValue : PropagatorResult { internal SimpleValue(PropagatorFlags flags, object value) { m_flags = flags; m_value = value ?? DBNull.Value; } private readonly PropagatorFlags m_flags; protected readonly object m_value; internal override PropagatorFlags PropagatorFlags { get { return m_flags; } } internal override bool IsSimple { get { return true; } } internal override bool IsNull { get { return DBNull.Value == m_value; } } internal override object GetSimpleValue() { return m_value; } internal override PropagatorResult ReplicateResultWithNewFlags(PropagatorFlags flags) { return new SimpleValue(flags, m_value); } internal override PropagatorResult ReplicateResultWithNewValue(object value) { return new SimpleValue(PropagatorFlags, value); } } internal static PropagatorResult CreateServerGenSimpleValue(PropagatorFlags flags, object value, CurrentValueRecord record, int recordOrdinal) { return new ServerGenSimpleValue(flags, value, record, recordOrdinal); } private class ServerGenSimpleValue : SimpleValue { internal ServerGenSimpleValue(PropagatorFlags flags, object value, CurrentValueRecord record, int recordOrdinal) : base(flags, value) { Debug.Assert(null != record); m_record = record; m_recordOrdinal = recordOrdinal; } private readonly CurrentValueRecord m_record; private readonly int m_recordOrdinal; internal override CurrentValueRecord Record { get { return m_record; } } internal override int RecordOrdinal { get { return m_recordOrdinal; } } internal override PropagatorResult ReplicateResultWithNewFlags(PropagatorFlags flags) { return new ServerGenSimpleValue(flags, m_value, Record, RecordOrdinal); } internal override PropagatorResult ReplicateResultWithNewValue(object value) { return new ServerGenSimpleValue(PropagatorFlags, value, Record, RecordOrdinal); } } internal static PropagatorResult CreateKeyValue(PropagatorFlags flags, object value, IEntityStateEntry stateEntry, long identifier) { return new KeyValue(flags, value, stateEntry, identifier); } private class KeyValue : SimpleValue { internal KeyValue(PropagatorFlags flags, object value, IEntityStateEntry stateEntry, long identifier) : base(flags, value) { Debug.Assert(null != stateEntry); m_stateEntry = stateEntry; m_identifier = identifier; } private readonly IEntityStateEntry m_stateEntry; private readonly long m_identifier; internal override IEntityStateEntry StateEntry { get { return m_stateEntry; } } internal override long Identifier { get { return m_identifier; } } internal override CurrentValueRecord Record { get { // delegate to the state entry, which also has the record return m_stateEntry.CurrentValues; } } internal override PropagatorResult ReplicateResultWithNewFlags(PropagatorFlags flags) { return new KeyValue(flags, m_value, StateEntry, Identifier); } internal override PropagatorResult ReplicateResultWithNewValue(object value) { return new KeyValue(PropagatorFlags, value, StateEntry, Identifier); } } internal static PropagatorResult CreateServerGenKeyValue(PropagatorFlags flags, object value, IEntityStateEntry stateEntry, long identifier, int recordOrdinal) { return new ServerGenKeyValue(flags, value, stateEntry, identifier, recordOrdinal); } private class ServerGenKeyValue : KeyValue { internal ServerGenKeyValue(PropagatorFlags flags, object value, IEntityStateEntry stateEntry, long identifier, int recordOrdinal) : base(flags, value, stateEntry, identifier) { m_recordOrdinal = recordOrdinal; } private readonly int m_recordOrdinal; internal override int RecordOrdinal { get { return m_recordOrdinal; } } internal override PropagatorResult ReplicateResultWithNewFlags(PropagatorFlags flags) { return new ServerGenKeyValue(flags, m_value, StateEntry, Identifier, RecordOrdinal); } internal override PropagatorResult ReplicateResultWithNewValue(object value) { return new ServerGenKeyValue(PropagatorFlags, value, StateEntry, Identifier, RecordOrdinal); } } internal static PropagatorResult CreateStructuralValue(PropagatorResult[] values, StructuralType structuralType, bool isModified) { if (isModified) { return new StructuralValue(values, structuralType); } else { return new UnmodifiedStructuralValue(values, structuralType); } } private class StructuralValue : PropagatorResult { internal StructuralValue(PropagatorResult[] values, StructuralType structuralType) { Debug.Assert(null != structuralType); Debug.Assert(null != values); Debug.Assert(values.Length == TypeHelpers.GetAllStructuralMembers(structuralType).Count); m_values = values; m_structuralType = structuralType; } private readonly PropagatorResult[] m_values; private readonly StructuralType m_structuralType; internal override bool IsSimple { get { return false; } } internal override bool IsNull { get { return false; } } internal override StructuralType StructuralType { get { return m_structuralType; } } internal override PropagatorResult GetMemberValue(int ordinal) { return m_values[ordinal]; } internal override PropagatorResult[] GetMemberValues() { return m_values; } internal override PropagatorResult ReplicateResultWithNewFlags(PropagatorFlags flags) { throw EntityUtil.InternalError(EntityUtil.InternalErrorCode.UpdatePipelineResultRequestInvalid, 0, "StructuralValue.ReplicateResultWithNewFlags"); } } private class UnmodifiedStructuralValue : StructuralValue { internal UnmodifiedStructuralValue(PropagatorResult[] values, StructuralType structuralType) : base (values, structuralType) { } internal override PropagatorFlags PropagatorFlags { get { return PropagatorFlags.Preserve; } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
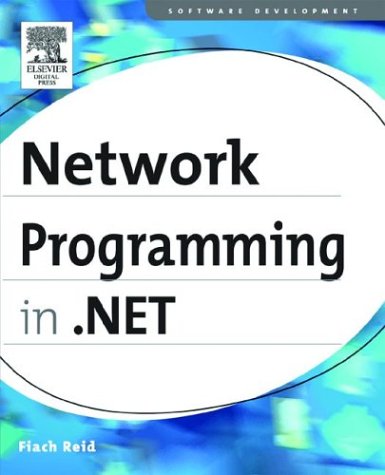
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Interlocked.cs
- ReadOnlyDictionary.cs
- SqlUnionizer.cs
- SqlExpressionNullability.cs
- XmlException.cs
- ApplicationGesture.cs
- ReadOnlyObservableCollection.cs
- CommandHelper.cs
- AvTrace.cs
- ManagementOptions.cs
- SymDocumentType.cs
- RC2.cs
- ControlParameter.cs
- ImportCatalogPart.cs
- PermissionRequestEvidence.cs
- MailMessageEventArgs.cs
- XhtmlTextWriter.cs
- Brush.cs
- RectValueSerializer.cs
- XmlSchemaObject.cs
- SqlMethodAttribute.cs
- RelationshipEndCollection.cs
- XmlDataSourceView.cs
- templategroup.cs
- SignedXmlDebugLog.cs
- SafeNativeMethods.cs
- WindowsSecurityToken.cs
- RuleSettings.cs
- ContentIterators.cs
- XmlUtil.cs
- Helpers.cs
- GuidelineSet.cs
- Padding.cs
- SqlException.cs
- IdentifierService.cs
- ILGenerator.cs
- ChannelDispatcherBase.cs
- QilNode.cs
- ScriptReference.cs
- IdentityValidationException.cs
- SoapException.cs
- FileDialog_Vista.cs
- DesignerTransaction.cs
- Popup.cs
- TableRow.cs
- KnownIds.cs
- XmlSchemaGroupRef.cs
- ConditionalAttribute.cs
- PaintEvent.cs
- ProcessHostMapPath.cs
- FilterRepeater.cs
- ConfigurationValue.cs
- EventMappingSettings.cs
- PhoneCallDesigner.cs
- DependencyPropertyChangedEventArgs.cs
- VisualStates.cs
- DbSource.cs
- OperandQuery.cs
- CompilationPass2TaskInternal.cs
- Metafile.cs
- PageCodeDomTreeGenerator.cs
- XmlArrayAttribute.cs
- CurrentChangedEventManager.cs
- SemanticResultValue.cs
- AuthorizationRuleCollection.cs
- StateBag.cs
- FileSystemWatcher.cs
- CommentEmitter.cs
- PlainXmlSerializer.cs
- GetIsBrowserClientRequest.cs
- DataGridViewImageCell.cs
- DLinqTableProvider.cs
- DataSourceControl.cs
- AsyncPostBackTrigger.cs
- PeerUnsafeNativeCryptMethods.cs
- AppDomainManager.cs
- SystemColors.cs
- ApplicationException.cs
- HttpCachePolicyElement.cs
- PlanCompilerUtil.cs
- ObjectContextServiceProvider.cs
- StringAnimationUsingKeyFrames.cs
- WeakHashtable.cs
- DTCTransactionManager.cs
- RC2.cs
- PrintDialogException.cs
- ValueTypeFieldReference.cs
- SymmetricAlgorithm.cs
- MobileErrorInfo.cs
- NullableDoubleAverageAggregationOperator.cs
- LinqDataSourceSelectEventArgs.cs
- ObjectListFieldsPage.cs
- EntityViewGenerator.cs
- Journal.cs
- PageCodeDomTreeGenerator.cs
- TabRenderer.cs
- TrustLevel.cs
- CustomGrammar.cs
- StopStoryboard.cs
- Wizard.cs