Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / clr / src / BCL / System / Security / Cryptography / SymmetricAlgorithm.cs / 1 / SymmetricAlgorithm.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // SymmetricAlgorithm.cs // namespace System.Security.Cryptography { [System.Runtime.InteropServices.ComVisible(true)] public abstract class SymmetricAlgorithm : IDisposable { protected int BlockSizeValue; protected int FeedbackSizeValue; protected byte[] IVValue; protected byte[] KeyValue; protected KeySizes[] LegalBlockSizesValue; protected KeySizes[] LegalKeySizesValue; protected int KeySizeValue; protected CipherMode ModeValue; protected PaddingMode PaddingValue; // // protected constructors // protected SymmetricAlgorithm() { // Default to cipher block chaining (CipherMode.CBC) and // PKCS-style padding (pad n bytes with value n) ModeValue = CipherMode.CBC; PaddingValue = PaddingMode.PKCS7; } // SymmetricAlgorithm implements IDisposable ///void IDisposable.Dispose() { Dispose(true); GC.SuppressFinalize(this); } public void Clear() { ((IDisposable) this).Dispose(); } protected virtual void Dispose(bool disposing) { if (disposing) { // Note: we always want to zeroize the sensitive key material if (KeyValue != null) { Array.Clear(KeyValue, 0, KeyValue.Length); KeyValue = null; } if (IVValue != null) { Array.Clear(IVValue, 0, IVValue.Length); IVValue = null; } } } // // public properties // public virtual int BlockSize { get { return BlockSizeValue; } set { int i; int j; for (i=0; i BlockSizeValue || (value % 8) != 0) throw new CryptographicException(Environment.GetResourceString("Cryptography_InvalidFeedbackSize")); FeedbackSizeValue = value; } } public virtual byte[] IV { get { if (IVValue == null) GenerateIV(); return (byte[]) IVValue.Clone(); } set { if (value == null) throw new ArgumentNullException("value"); if (value.Length != BlockSizeValue / 8) throw new CryptographicException(Environment.GetResourceString("Cryptography_InvalidIVSize")); IVValue = (byte[]) value.Clone(); } } public virtual byte[] Key { get { if (KeyValue == null) GenerateKey(); return (byte[]) KeyValue.Clone(); } set { if (value == null) throw new ArgumentNullException("value"); if (!ValidKeySize(value.Length * 8)) throw new CryptographicException(Environment.GetResourceString("Cryptography_InvalidKeySize")); // must convert bytes to bits KeyValue = (byte[]) value.Clone(); KeySizeValue = value.Length * 8; } } public virtual KeySizes[] LegalBlockSizes { get { return (KeySizes[]) LegalBlockSizesValue.Clone(); } } public virtual KeySizes[] LegalKeySizes { get { return (KeySizes[]) LegalKeySizesValue.Clone(); } } public virtual int KeySize { get { return KeySizeValue; } set { if (!ValidKeySize(value)) throw new CryptographicException(Environment.GetResourceString("Cryptography_InvalidKeySize")); KeySizeValue = value; KeyValue = null; } } public virtual CipherMode Mode { get { return ModeValue; } set { if ((value < CipherMode.CBC) || (CipherMode.CFB < value)) throw new CryptographicException(Environment.GetResourceString("Cryptography_InvalidCipherMode")); ModeValue = value; } } public virtual PaddingMode Padding { get { return PaddingValue; } set { if ((value < PaddingMode.None) || (PaddingMode.ISO10126 < value)) throw new CryptographicException(Environment.GetResourceString("Cryptography_InvalidPaddingMode")); PaddingValue = value; } } // // public methods // // The following method takes a bit length input and returns whether that length is a valid size // according to LegalKeySizes public bool ValidKeySize(int bitLength) { KeySizes[] validSizes = this.LegalKeySizes; int i,j; if (validSizes == null) return false; for (i=0; i< validSizes.Length; i++) { if (validSizes[i].SkipSize == 0) { if (validSizes[i].MinSize == bitLength) { // assume MinSize = MaxSize return true; } } else { for (j = validSizes[i].MinSize; j<= validSizes[i].MaxSize; j += validSizes[i].SkipSize) { if (j == bitLength) { return true; } } } } return false; } static public SymmetricAlgorithm Create() { // use the crypto config system to return an instance of // the default SymmetricAlgorithm on this machine return Create("System.Security.Cryptography.SymmetricAlgorithm"); } static public SymmetricAlgorithm Create(String algName) { return (SymmetricAlgorithm) CryptoConfig.CreateFromName(algName); } public virtual ICryptoTransform CreateEncryptor() { return CreateEncryptor(Key, IV); } public abstract ICryptoTransform CreateEncryptor(byte[] rgbKey, byte[] rgbIV); public virtual ICryptoTransform CreateDecryptor() { return CreateDecryptor(Key, IV); } public abstract ICryptoTransform CreateDecryptor(byte[] rgbKey, byte[] rgbIV); public abstract void GenerateKey(); public abstract void GenerateIV(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // SymmetricAlgorithm.cs // namespace System.Security.Cryptography { [System.Runtime.InteropServices.ComVisible(true)] public abstract class SymmetricAlgorithm : IDisposable { protected int BlockSizeValue; protected int FeedbackSizeValue; protected byte[] IVValue; protected byte[] KeyValue; protected KeySizes[] LegalBlockSizesValue; protected KeySizes[] LegalKeySizesValue; protected int KeySizeValue; protected CipherMode ModeValue; protected PaddingMode PaddingValue; // // protected constructors // protected SymmetricAlgorithm() { // Default to cipher block chaining (CipherMode.CBC) and // PKCS-style padding (pad n bytes with value n) ModeValue = CipherMode.CBC; PaddingValue = PaddingMode.PKCS7; } // SymmetricAlgorithm implements IDisposable /// void IDisposable.Dispose() { Dispose(true); GC.SuppressFinalize(this); } public void Clear() { ((IDisposable) this).Dispose(); } protected virtual void Dispose(bool disposing) { if (disposing) { // Note: we always want to zeroize the sensitive key material if (KeyValue != null) { Array.Clear(KeyValue, 0, KeyValue.Length); KeyValue = null; } if (IVValue != null) { Array.Clear(IVValue, 0, IVValue.Length); IVValue = null; } } } // // public properties // public virtual int BlockSize { get { return BlockSizeValue; } set { int i; int j; for (i=0; i BlockSizeValue || (value % 8) != 0) throw new CryptographicException(Environment.GetResourceString("Cryptography_InvalidFeedbackSize")); FeedbackSizeValue = value; } } public virtual byte[] IV { get { if (IVValue == null) GenerateIV(); return (byte[]) IVValue.Clone(); } set { if (value == null) throw new ArgumentNullException("value"); if (value.Length != BlockSizeValue / 8) throw new CryptographicException(Environment.GetResourceString("Cryptography_InvalidIVSize")); IVValue = (byte[]) value.Clone(); } } public virtual byte[] Key { get { if (KeyValue == null) GenerateKey(); return (byte[]) KeyValue.Clone(); } set { if (value == null) throw new ArgumentNullException("value"); if (!ValidKeySize(value.Length * 8)) throw new CryptographicException(Environment.GetResourceString("Cryptography_InvalidKeySize")); // must convert bytes to bits KeyValue = (byte[]) value.Clone(); KeySizeValue = value.Length * 8; } } public virtual KeySizes[] LegalBlockSizes { get { return (KeySizes[]) LegalBlockSizesValue.Clone(); } } public virtual KeySizes[] LegalKeySizes { get { return (KeySizes[]) LegalKeySizesValue.Clone(); } } public virtual int KeySize { get { return KeySizeValue; } set { if (!ValidKeySize(value)) throw new CryptographicException(Environment.GetResourceString("Cryptography_InvalidKeySize")); KeySizeValue = value; KeyValue = null; } } public virtual CipherMode Mode { get { return ModeValue; } set { if ((value < CipherMode.CBC) || (CipherMode.CFB < value)) throw new CryptographicException(Environment.GetResourceString("Cryptography_InvalidCipherMode")); ModeValue = value; } } public virtual PaddingMode Padding { get { return PaddingValue; } set { if ((value < PaddingMode.None) || (PaddingMode.ISO10126 < value)) throw new CryptographicException(Environment.GetResourceString("Cryptography_InvalidPaddingMode")); PaddingValue = value; } } // // public methods // // The following method takes a bit length input and returns whether that length is a valid size // according to LegalKeySizes public bool ValidKeySize(int bitLength) { KeySizes[] validSizes = this.LegalKeySizes; int i,j; if (validSizes == null) return false; for (i=0; i< validSizes.Length; i++) { if (validSizes[i].SkipSize == 0) { if (validSizes[i].MinSize == bitLength) { // assume MinSize = MaxSize return true; } } else { for (j = validSizes[i].MinSize; j<= validSizes[i].MaxSize; j += validSizes[i].SkipSize) { if (j == bitLength) { return true; } } } } return false; } static public SymmetricAlgorithm Create() { // use the crypto config system to return an instance of // the default SymmetricAlgorithm on this machine return Create("System.Security.Cryptography.SymmetricAlgorithm"); } static public SymmetricAlgorithm Create(String algName) { return (SymmetricAlgorithm) CryptoConfig.CreateFromName(algName); } public virtual ICryptoTransform CreateEncryptor() { return CreateEncryptor(Key, IV); } public abstract ICryptoTransform CreateEncryptor(byte[] rgbKey, byte[] rgbIV); public virtual ICryptoTransform CreateDecryptor() { return CreateDecryptor(Key, IV); } public abstract ICryptoTransform CreateDecryptor(byte[] rgbKey, byte[] rgbIV); public abstract void GenerateKey(); public abstract void GenerateIV(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
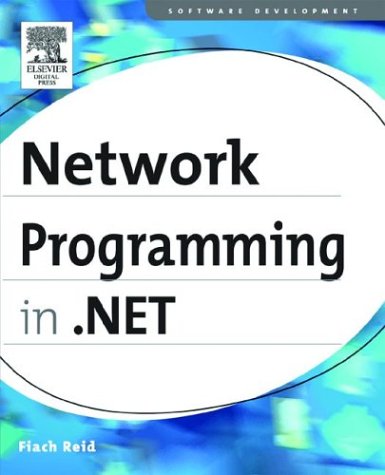
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SessionStateUtil.cs
- Crypto.cs
- ProcessHostServerConfig.cs
- SuppressMergeCheckAttribute.cs
- WebPartConnectionsCancelVerb.cs
- UICuesEvent.cs
- Group.cs
- SafeLocalMemHandle.cs
- RemotingAttributes.cs
- ControlUtil.cs
- DockingAttribute.cs
- DataGridDetailsPresenterAutomationPeer.cs
- ListView.cs
- InputLangChangeEvent.cs
- EditorBrowsableAttribute.cs
- PageCodeDomTreeGenerator.cs
- StylusEventArgs.cs
- IERequestCache.cs
- NativeMethodsCLR.cs
- TranslateTransform.cs
- DataControlFieldsEditor.cs
- SQLByte.cs
- DockPatternIdentifiers.cs
- SafePEFileHandle.cs
- AddInProcess.cs
- DataControlLinkButton.cs
- TextProperties.cs
- UIPropertyMetadata.cs
- GridViewColumn.cs
- PreProcessInputEventArgs.cs
- Logging.cs
- DBDataPermissionAttribute.cs
- WinEventQueueItem.cs
- TreeNodeSelectionProcessor.cs
- ComponentEditorForm.cs
- TypeResolvingOptionsAttribute.cs
- ClientRuntimeConfig.cs
- SoapSchemaExporter.cs
- PersonalizableAttribute.cs
- X509PeerCertificateAuthentication.cs
- TypeSemantics.cs
- MaterializeFromAtom.cs
- ObjectConverter.cs
- SmiRequestExecutor.cs
- IUnknownConstantAttribute.cs
- DefaultAuthorizationContext.cs
- PassportPrincipal.cs
- Label.cs
- AttributeParameterInfo.cs
- FontUnitConverter.cs
- PropertyPushdownHelper.cs
- DomainUpDown.cs
- TypeLibConverter.cs
- _UriSyntax.cs
- SecurityPolicyVersion.cs
- DataSourceControl.cs
- XmlAutoDetectWriter.cs
- FrugalList.cs
- UniqueIdentifierService.cs
- PropertyKey.cs
- SpeechSeg.cs
- TextEffectResolver.cs
- RelatedEnd.cs
- Selection.cs
- WebPartCatalogCloseVerb.cs
- ShapeTypeface.cs
- TreeView.cs
- SqlExpander.cs
- TextEditorParagraphs.cs
- TypographyProperties.cs
- DrawingGroupDrawingContext.cs
- ContainerControl.cs
- DataStreams.cs
- StylusLogic.cs
- Calendar.cs
- NativeDirectoryServicesQueryAPIs.cs
- CaseInsensitiveOrdinalStringComparer.cs
- CriticalFinalizerObject.cs
- TimelineGroup.cs
- EventWaitHandle.cs
- WebBrowser.cs
- BinaryMessageEncodingBindingElement.cs
- HttpSessionStateWrapper.cs
- LogEntryDeserializer.cs
- CompressStream.cs
- InstalledVoice.cs
- BuildProviderCollection.cs
- SafeNativeMethods.cs
- SendingRequestEventArgs.cs
- SmiEventSink_DeferedProcessing.cs
- BitmapEffectGeneralTransform.cs
- ColorMatrix.cs
- TraceLevelHelper.cs
- EntityDataSourceState.cs
- DateTimeConverter.cs
- RelatedView.cs
- CodeNamespace.cs
- BinHexDecoder.cs
- dsa.cs
- UnsafeNativeMethods.cs