Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / UIAutomation / UIAutomationClient / System / Windows / Automation / InvokePattern.cs / 1 / InvokePattern.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Client-side wrapper for Invoke Pattern // // History: // 06/23/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Represents objects that have a single, unambiguous, action associated with them. /// /// Examples of UI that implments this includes: /// Push buttons /// Hyperlinks /// Menu items /// Radio buttons /// Check boxes /// #if (INTERNAL_COMPILE) internal class InvokePattern: BasePattern #else public class InvokePattern: BasePattern #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private InvokePattern(AutomationElement el, SafePatternHandle hPattern) : base(el, hPattern) { _hPattern = hPattern; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///Invokable pattern public static readonly AutomationPattern Pattern = InvokePatternIdentifiers.Pattern; ///Event ID: Invoked - event used to watch for Invokable pattern Invoked events public static readonly AutomationEvent InvokedEvent = InvokePatternIdentifiers.InvokedEvent; #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ////// Request that the control initiate its action. /// Should return immediately without blocking. /// There is no way to determine what happened, when it happend, or whether /// anything happened at all. /// /// ////// This API does not work inside the secure execution environment. /// public void Invoke() { UiaCoreApi.InvokePattern_Invoke(_hPattern); } #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties // No properties #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal static object Wrap(AutomationElement el, SafePatternHandle hPattern, bool cached) { return new InvokePattern(el, hPattern); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private SafePatternHandle _hPattern; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // ///// // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Client-side wrapper for Invoke Pattern // // History: // 06/23/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Represents objects that have a single, unambiguous, action associated with them. /// /// Examples of UI that implments this includes: /// Push buttons /// Hyperlinks /// Menu items /// Radio buttons /// Check boxes /// #if (INTERNAL_COMPILE) internal class InvokePattern: BasePattern #else public class InvokePattern: BasePattern #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private InvokePattern(AutomationElement el, SafePatternHandle hPattern) : base(el, hPattern) { _hPattern = hPattern; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///Invokable pattern public static readonly AutomationPattern Pattern = InvokePatternIdentifiers.Pattern; ///Event ID: Invoked - event used to watch for Invokable pattern Invoked events public static readonly AutomationEvent InvokedEvent = InvokePatternIdentifiers.InvokedEvent; #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ////// Request that the control initiate its action. /// Should return immediately without blocking. /// There is no way to determine what happened, when it happend, or whether /// anything happened at all. /// /// ////// This API does not work inside the secure execution environment. /// public void Invoke() { UiaCoreApi.InvokePattern_Invoke(_hPattern); } #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties // No properties #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal static object Wrap(AutomationElement el, SafePatternHandle hPattern, bool cached) { return new InvokePattern(el, hPattern); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private SafePatternHandle _hPattern; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.///
Link Menu
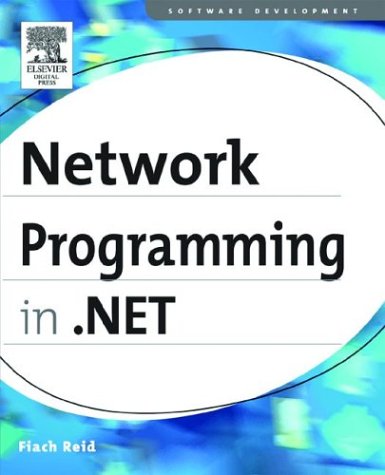
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaElement.cs
- UriTemplateHelpers.cs
- Util.cs
- BasicExpandProvider.cs
- WinInet.cs
- SplitterDesigner.cs
- ApplicationInfo.cs
- AmbientLight.cs
- WebPartActionVerb.cs
- DataBoundLiteralControl.cs
- ReadOnlyObservableCollection.cs
- ContainerParaClient.cs
- EmptyQuery.cs
- WSHttpBindingCollectionElement.cs
- WebPartDeleteVerb.cs
- ChooseAction.cs
- HwndKeyboardInputProvider.cs
- SByte.cs
- RowUpdatedEventArgs.cs
- NonSerializedAttribute.cs
- FileRecordSequenceCompletedAsyncResult.cs
- DataGridViewRowPostPaintEventArgs.cs
- XmlTextReader.cs
- ProviderBase.cs
- EtwTrackingBehaviorElement.cs
- MasterPageBuildProvider.cs
- HorizontalAlignConverter.cs
- CompilationLock.cs
- CdpEqualityComparer.cs
- Polygon.cs
- PathGeometry.cs
- BasicHttpBinding.cs
- PlatformNotSupportedException.cs
- OneOfElement.cs
- MobileComponentEditorPage.cs
- StringUtil.cs
- BmpBitmapEncoder.cs
- Selector.cs
- DataFormats.cs
- CompatibleIComparer.cs
- WebPartEditorOkVerb.cs
- InvalidCastException.cs
- LabelTarget.cs
- TemplatePropertyEntry.cs
- RegexReplacement.cs
- PropertyNames.cs
- SortQuery.cs
- FontWeight.cs
- DataRowComparer.cs
- ConnectionInterfaceCollection.cs
- CodeSnippetTypeMember.cs
- ReflectTypeDescriptionProvider.cs
- CustomActivityDesigner.cs
- QilGeneratorEnv.cs
- configsystem.cs
- MSAAEventDispatcher.cs
- TypeBuilder.cs
- SelectionItemPattern.cs
- DocumentSequence.cs
- Util.cs
- ConfigurationPermission.cs
- SiteMapDataSource.cs
- PropertyValue.cs
- Content.cs
- NameValuePair.cs
- BinaryMethodMessage.cs
- XmlSerializerFactory.cs
- EventQueueState.cs
- XmlSchemaValidationException.cs
- WebPartMovingEventArgs.cs
- KeyValuePair.cs
- XmlCustomFormatter.cs
- MultiAsyncResult.cs
- StaticFileHandler.cs
- AccessibilityApplicationManager.cs
- XhtmlBasicLabelAdapter.cs
- FileDialog.cs
- HMACRIPEMD160.cs
- JsonServiceDocumentSerializer.cs
- SqlException.cs
- Regex.cs
- LocalizableResourceBuilder.cs
- ServiceDeploymentInfo.cs
- ExtendedProtectionPolicy.cs
- MDIClient.cs
- RelationshipEnd.cs
- ConnectionPoolManager.cs
- ExpressionBuilderCollection.cs
- ObjectCloneHelper.cs
- CroppedBitmap.cs
- ToolStripMenuItem.cs
- _OverlappedAsyncResult.cs
- WebPartCancelEventArgs.cs
- ObjectConverter.cs
- PolygonHotSpot.cs
- EndpointBehaviorElementCollection.cs
- AccessKeyManager.cs
- SyndicationDeserializer.cs
- DesignerAttributeInfo.cs
- IList.cs