Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Security / WSSecurityOneDotOneReceiveSecurityHeader.cs / 1 / WSSecurityOneDotOneReceiveSecurityHeader.cs
//---------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Security { using System.IO; using System.ServiceModel.Channels; using System.ServiceModel; using System.ServiceModel.Description; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.IdentityModel.Tokens; using System.Security.Cryptography; using System.ServiceModel.Security.Tokens; using System.Text; using System.Xml; using System.ServiceModel.Diagnostics; class WSSecurityOneDotOneReceiveSecurityHeader : WSSecurityOneDotZeroReceiveSecurityHeader { public WSSecurityOneDotOneReceiveSecurityHeader(Message message, string actor, bool mustUnderstand, bool relay, SecurityStandardsManager standardsManager, SecurityAlgorithmSuite algorithmSuite, int headerIndex, MessageDirection direction) : base(message, actor, mustUnderstand, relay, standardsManager, algorithmSuite, headerIndex, direction) { } protected override DecryptedHeader DecryptHeader(XmlDictionaryReader reader, WrappedKeySecurityToken wrappedKeyToken) { EncryptedHeaderXml headerXml = new EncryptedHeaderXml(this.Version); headerXml.SecurityTokenSerializer = this.StandardsManager.SecurityTokenSerializer; headerXml.ReadFrom(reader, MaxReceivedMessageSize); // The Encrypted Headers MustUnderstand, Relay and Actor attributes should match the // Security Headers value. if (headerXml.MustUnderstand != this.MustUnderstand) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new MessageSecurityException(SR.GetString(SR.EncryptedHeaderAttributeMismatch, XD.MessageDictionary.MustUnderstand.Value, headerXml.MustUnderstand, this.MustUnderstand))); if (headerXml.Relay != this.Relay) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new MessageSecurityException(SR.GetString(SR.EncryptedHeaderAttributeMismatch, XD.Message12Dictionary.Relay.Value, headerXml.Relay, this.Relay))); if (headerXml.Actor != this.Actor) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new MessageSecurityException(SR.GetString(SR.EncryptedHeaderAttributeMismatch, this.Version.Envelope.DictionaryActor, headerXml.Actor, this.Actor))); SecurityToken token; if (wrappedKeyToken == null) { token = ResolveKeyIdentifier(headerXml.KeyIdentifier, this.CombinedPrimaryTokenResolver, false); } else { token = wrappedKeyToken; } RecordEncryptionToken(token); using (SymmetricAlgorithm algorithm = CreateDecryptionAlgorithm(token, headerXml.EncryptionMethod, this.AlgorithmSuite)) { headerXml.SetUpDecryption(algorithm); return new DecryptedHeader( headerXml.GetDecryptedBuffer(), this.SecurityVerifiedMessage.GetEnvelopeAttributes(), this.SecurityVerifiedMessage.GetHeaderAttributes(), this.Version, this.StandardsManager.IdManager, this.ReaderQuotas); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
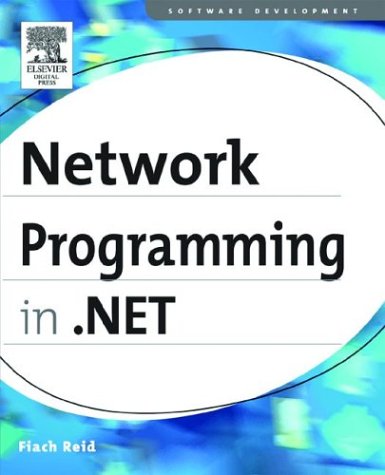
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityDataSourceWrapperCollection.cs
- PositiveTimeSpanValidator.cs
- XPathItem.cs
- SerializationEventsCache.cs
- AssociationSetEnd.cs
- FacetValueContainer.cs
- UIElementParaClient.cs
- ComponentResourceManager.cs
- ThreadExceptionDialog.cs
- ButtonColumn.cs
- RectAnimation.cs
- WebBrowsableAttribute.cs
- ScopedKnownTypes.cs
- DataBinding.cs
- Int32KeyFrameCollection.cs
- XmlSortKey.cs
- CaseExpr.cs
- XmlDocumentType.cs
- DataRecordInternal.cs
- SelectorItemAutomationPeer.cs
- DockPattern.cs
- Light.cs
- Environment.cs
- OpCellTreeNode.cs
- RegisteredScript.cs
- ZipIOExtraFieldPaddingElement.cs
- ContextMarshalException.cs
- DisplayNameAttribute.cs
- TrackingProfileDeserializationException.cs
- SrgsRule.cs
- EdgeProfileValidation.cs
- SegmentInfo.cs
- CommandManager.cs
- ListViewUpdateEventArgs.cs
- DataGridParentRows.cs
- ExplicitDiscriminatorMap.cs
- ChunkedMemoryStream.cs
- TextElementEnumerator.cs
- TextEffectResolver.cs
- ControlValuePropertyAttribute.cs
- StateChangeEvent.cs
- ReadOnlyCollection.cs
- AssemblyContextControlItem.cs
- InterleavedZipPartStream.cs
- ImageMap.cs
- RuleInfoComparer.cs
- Mapping.cs
- InboundActivityHelper.cs
- OperatingSystemVersionCheck.cs
- ReachPageContentSerializerAsync.cs
- NativeRecognizer.cs
- AtomParser.cs
- SoapObjectReader.cs
- WebPartRestoreVerb.cs
- NewArrayExpression.cs
- HtmlUtf8RawTextWriter.cs
- EasingKeyFrames.cs
- IdentitySection.cs
- DeferredSelectedIndexReference.cs
- GridProviderWrapper.cs
- EncoderParameters.cs
- EventMemberCodeDomSerializer.cs
- PathStreamGeometryContext.cs
- Assert.cs
- SpellCheck.cs
- DataGridItemEventArgs.cs
- AuthenticationConfig.cs
- BehaviorEditorPart.cs
- MetadataResolver.cs
- FtpCachePolicyElement.cs
- Vector3DCollection.cs
- ToolStripContentPanel.cs
- ActivityCompletionCallbackWrapper.cs
- WeakReferenceKey.cs
- GeneralTransform.cs
- EventDescriptorCollection.cs
- SrgsGrammar.cs
- DataSetFieldSchema.cs
- COMException.cs
- EntityStoreSchemaFilterEntry.cs
- InputProcessorProfilesLoader.cs
- UIAgentAsyncEndRequest.cs
- XmlLanguage.cs
- OutputScope.cs
- DataGridItemCollection.cs
- CqlGenerator.cs
- bidPrivateBase.cs
- CodeGeneratorOptions.cs
- OwnerDrawPropertyBag.cs
- CheckBoxField.cs
- XmlSchema.cs
- ListViewSelectEventArgs.cs
- ExternalCalls.cs
- RunInstallerAttribute.cs
- XmlWrappingReader.cs
- SettingsPropertyIsReadOnlyException.cs
- DiagnosticTrace.cs
- ResourceProperty.cs
- ListSourceHelper.cs
- _AutoWebProxyScriptEngine.cs