Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / clr / src / BCL / System / Diagnostics / Assert.cs / 1 / Assert.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== namespace System.Diagnostics { using System; using System.Security.Permissions; using System.IO; using System.Reflection; using System.Runtime.CompilerServices; // Class which handles code asserts. Asserts are used to explicitly protect // assumptions made in the code. In general if an assert fails, it indicates // a program bug so is immediately called to the attention of the user. // Only static data members, does not need to be marked with the serializable attribute internal static class Assert { private static AssertFilter[] ListOfFilters; private static int iNumOfFilters; private static int iFilterArraySize; static Assert() { Assert.AddFilter(new DefaultFilter()); } // AddFilter adds a new assert filter. This replaces the current // filter, unless the filter returns FailContinue. // public static void AddFilter(AssertFilter filter) { if (iFilterArraySize <= iNumOfFilters) { AssertFilter[] newFilterArray = new AssertFilter [iFilterArraySize+2]; if (iNumOfFilters > 0) Array.Copy(ListOfFilters, newFilterArray, iNumOfFilters); iFilterArraySize += 2; ListOfFilters = newFilterArray; } ListOfFilters [iNumOfFilters++] = filter; } // Called when an assertion is being made. // public static void Check(bool condition, String conditionString, String message) { if (!condition) { Fail (conditionString, message); } } public static void Fail(String conditionString, String message) { // get the stacktrace StackTrace st = new StackTrace(); // Run through the list of filters backwards (the last filter in the list // is the default filter. So we're guaranteed that there will be atleast // one filter to handle the assert. int iTemp = iNumOfFilters; while (iTemp > 0) { AssertFilters iResult = ListOfFilters [--iTemp].AssertFailure (conditionString, message, st); if (iResult == AssertFilters.FailDebug) { if (Debugger.IsAttached == true) Debugger.Break(); else { if (Debugger.Launch() == false) { throw new InvalidOperationException( Environment.GetResourceString("InvalidOperation_DebuggerLaunchFailed")); } } break; } else if (iResult == AssertFilters.FailTerminate) Environment.Exit(-1); else if (iResult == AssertFilters.FailIgnore) break; // If none of the above, it means that the Filter returned FailContinue. // So invoke the next filter. } } // Called when an assert happens. // [MethodImplAttribute(MethodImplOptions.InternalCall)] public extern static int ShowDefaultAssertDialog(String conditionString, String message); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== namespace System.Diagnostics { using System; using System.Security.Permissions; using System.IO; using System.Reflection; using System.Runtime.CompilerServices; // Class which handles code asserts. Asserts are used to explicitly protect // assumptions made in the code. In general if an assert fails, it indicates // a program bug so is immediately called to the attention of the user. // Only static data members, does not need to be marked with the serializable attribute internal static class Assert { private static AssertFilter[] ListOfFilters; private static int iNumOfFilters; private static int iFilterArraySize; static Assert() { Assert.AddFilter(new DefaultFilter()); } // AddFilter adds a new assert filter. This replaces the current // filter, unless the filter returns FailContinue. // public static void AddFilter(AssertFilter filter) { if (iFilterArraySize <= iNumOfFilters) { AssertFilter[] newFilterArray = new AssertFilter [iFilterArraySize+2]; if (iNumOfFilters > 0) Array.Copy(ListOfFilters, newFilterArray, iNumOfFilters); iFilterArraySize += 2; ListOfFilters = newFilterArray; } ListOfFilters [iNumOfFilters++] = filter; } // Called when an assertion is being made. // public static void Check(bool condition, String conditionString, String message) { if (!condition) { Fail (conditionString, message); } } public static void Fail(String conditionString, String message) { // get the stacktrace StackTrace st = new StackTrace(); // Run through the list of filters backwards (the last filter in the list // is the default filter. So we're guaranteed that there will be atleast // one filter to handle the assert. int iTemp = iNumOfFilters; while (iTemp > 0) { AssertFilters iResult = ListOfFilters [--iTemp].AssertFailure (conditionString, message, st); if (iResult == AssertFilters.FailDebug) { if (Debugger.IsAttached == true) Debugger.Break(); else { if (Debugger.Launch() == false) { throw new InvalidOperationException( Environment.GetResourceString("InvalidOperation_DebuggerLaunchFailed")); } } break; } else if (iResult == AssertFilters.FailTerminate) Environment.Exit(-1); else if (iResult == AssertFilters.FailIgnore) break; // If none of the above, it means that the Filter returned FailContinue. // So invoke the next filter. } } // Called when an assert happens. // [MethodImplAttribute(MethodImplOptions.InternalCall)] public extern static int ShowDefaultAssertDialog(String conditionString, String message); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
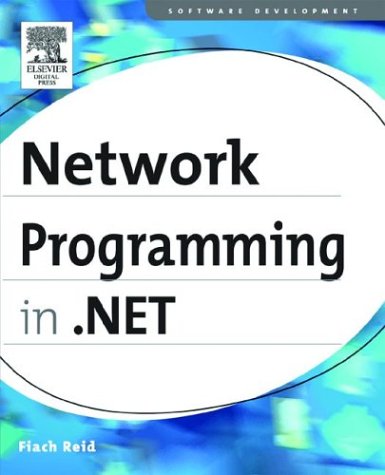
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataBinding.cs
- FormView.cs
- WebPartHelpVerb.cs
- CodeAttributeArgument.cs
- TextModifier.cs
- PassportIdentity.cs
- DataTable.cs
- XmlSchemas.cs
- DetailsViewRow.cs
- EtwTrace.cs
- SqlNotificationEventArgs.cs
- DispatcherFrame.cs
- WebCodeGenerator.cs
- TemplateControl.cs
- DebugView.cs
- StateDesigner.CommentLayoutGlyph.cs
- WindowsListViewItemCheckBox.cs
- RegistryConfigurationProvider.cs
- ExtentKey.cs
- _NestedSingleAsyncResult.cs
- Preprocessor.cs
- RoutedCommand.cs
- UInt32.cs
- ComponentManagerBroker.cs
- KeyPullup.cs
- ValidatedControlConverter.cs
- CustomAttributeSerializer.cs
- AnnotationComponentManager.cs
- QueryOperationResponseOfT.cs
- MultipleViewProviderWrapper.cs
- MouseGestureConverter.cs
- PolyBezierSegmentFigureLogic.cs
- ChildDocumentBlock.cs
- FileDialogPermission.cs
- FormClosingEvent.cs
- StringTraceRecord.cs
- ListViewItemEventArgs.cs
- FontSource.cs
- NullableIntAverageAggregationOperator.cs
- CodeIdentifier.cs
- PropertiesTab.cs
- SqlErrorCollection.cs
- TextSelection.cs
- SemaphoreFullException.cs
- CreateCardRequest.cs
- ConvertersCollection.cs
- FtpCachePolicyElement.cs
- GlyphElement.cs
- Point4DConverter.cs
- CompilerError.cs
- HtmlWindowCollection.cs
- MenuStrip.cs
- Module.cs
- ChildrenQuery.cs
- ReliabilityContractAttribute.cs
- ExpressionPrinter.cs
- ProfileServiceManager.cs
- NullableConverter.cs
- TrustManager.cs
- SamlAction.cs
- CodeTypeReferenceExpression.cs
- DataServiceException.cs
- PageTheme.cs
- XPathDocumentIterator.cs
- SqlCommandBuilder.cs
- Base64Encoder.cs
- ReadOnlyMetadataCollection.cs
- MultiAsyncResult.cs
- SessionKeyExpiredException.cs
- EffectiveValueEntry.cs
- Point3DAnimationBase.cs
- SqlUserDefinedAggregateAttribute.cs
- EventDescriptorCollection.cs
- DeploymentSectionCache.cs
- DetailsViewInsertedEventArgs.cs
- SupportingTokenParameters.cs
- AttachmentService.cs
- XmlSchemaComplexContentRestriction.cs
- SystemIcmpV4Statistics.cs
- XmlSchemaSimpleType.cs
- QueryResponse.cs
- UnitySerializationHolder.cs
- PeerCollaborationPermission.cs
- ClientType.cs
- MultiDataTrigger.cs
- HandleTable.cs
- ColumnMapProcessor.cs
- PropertyItem.cs
- PeerContact.cs
- ModelUtilities.cs
- CompilerLocalReference.cs
- StickyNote.cs
- TypeNameConverter.cs
- X509ThumbprintKeyIdentifierClause.cs
- MetadataArtifactLoader.cs
- ConsumerConnectionPointCollection.cs
- DataDocumentXPathNavigator.cs
- ModelVisual3D.cs
- MappingItemCollection.cs
- EventDescriptor.cs