Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / clr / src / BCL / System / Diagnostics / LogSwitch.cs / 1 / LogSwitch.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== namespace System.Diagnostics { using System; using System.IO; using System.Collections; [Serializable()] internal class LogSwitch { // ! WARNING ! // If any fields are added/deleted/modified, perform the // same in the EE code (debugdebugger.cpp) internal String strName; internal String strDescription; private LogSwitch ParentSwitch; private LogSwitch[] ChildSwitch; internal LoggingLevels iLevel; internal LoggingLevels iOldLevel; private int iNumChildren; private int iChildArraySize; // ! END WARNING ! private LogSwitch () { } // Constructs a LogSwitch. A LogSwitch is used to categorize log messages. // // All switches (except for the global LogSwitch) have a parent LogSwitch. // public LogSwitch(String name, String description, LogSwitch parent) { if ((name != null) && (parent != null) ) { if (name.Length == 0) throw new ArgumentOutOfRangeException ("Name", Environment.GetResourceString("Argument_StringZeroLength")); strName = name; strDescription = description; iLevel = LoggingLevels.ErrorLevel; iOldLevel = iLevel; // update the parent switch to reflect this child switch parent.AddChildSwitch (this); ParentSwitch = parent; ChildSwitch = null; iNumChildren = 0; iChildArraySize = 0; Log.m_Hashtable.Add (strName, this); // Call into the EE to let it know about the creation of // this switch Log.AddLogSwitch (this); // update switch count Log.iNumOfSwitches++; } else throw new ArgumentNullException ((name==null ? "name" : "parent")); } internal LogSwitch(String name, String description) { strName = name; strDescription = description; iLevel = LoggingLevels.ErrorLevel; iOldLevel = iLevel; ParentSwitch = null; ChildSwitch = null; iNumChildren = 0; iChildArraySize = 0; Log.m_Hashtable.Add (strName, this); // Call into the EE to let it know about the creation of // this switch Log.AddLogSwitch (this); // update switch count Log.iNumOfSwitches++; } // Get property returns the name of the switch public virtual String Name { get { return strName;} } // Get property returns the description of the switch public virtual String Description { get {return strDescription;} } // Get property returns the parent of the switch public virtual LogSwitch Parent { get { return ParentSwitch; } } // Property to Get/Set the level of log messages which are "on" for the switch. // public virtual LoggingLevels MinimumLevel { get { return iLevel; } set { iLevel = value; iOldLevel = value; String strParentName = ParentSwitch!=null ? ParentSwitch.Name : ""; if (Debugger.IsAttached) Log.ModifyLogSwitch ((int)iLevel, strName, strParentName); Log.InvokeLogSwitchLevelHandlers (this, iLevel); } } // Checks if the given level is "on" for this switch or one of its parents. // public virtual bool CheckLevel(LoggingLevels level) { if (iLevel > level) { // recurse through the list till parent is hit. if (this.ParentSwitch == null) return false; else return this.ParentSwitch.CheckLevel (level); } else return true; } // Returns a switch with the particular name, if any. Returns null if no // such switch exists. public static LogSwitch GetSwitch(String name) { return (LogSwitch) Log.m_Hashtable[name]; } private void AddChildSwitch (LogSwitch child) { if (iChildArraySize <= iNumChildren) { int iIncreasedSize; if (iChildArraySize == 0) iIncreasedSize = 10; else iIncreasedSize = (iChildArraySize * 3) / 2; // increase child array size in chunks of 4 LogSwitch[] newChildSwitchArray = new LogSwitch [iIncreasedSize]; // copy the old array objects into the new one. if (iNumChildren > 0) Array.Copy(ChildSwitch, newChildSwitchArray, iNumChildren); iChildArraySize = iIncreasedSize; ChildSwitch = newChildSwitchArray; } ChildSwitch [iNumChildren++] = child; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
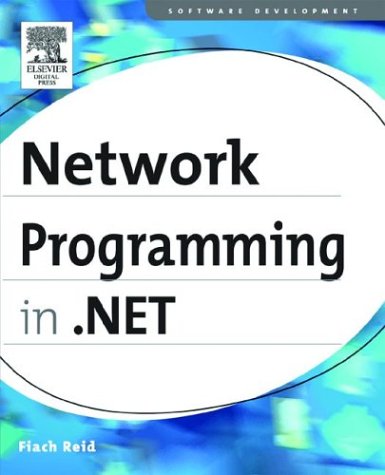
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TableDetailsRow.cs
- SoapAttributeAttribute.cs
- ToolStripSettings.cs
- COM2IProvidePropertyBuilderHandler.cs
- VisualBasic.cs
- NativeRightsManagementAPIsStructures.cs
- ActivityWithResultValueSerializer.cs
- DependencyPropertyAttribute.cs
- DispatcherEventArgs.cs
- AssemblyContextControlItem.cs
- Condition.cs
- BlockUIContainer.cs
- DragAssistanceManager.cs
- _NtlmClient.cs
- ItemCollectionEditor.cs
- ServiceEndpoint.cs
- ListItemDetailViewAttribute.cs
- DoubleStorage.cs
- SHA256Managed.cs
- XmlSerializerFactory.cs
- QilFunction.cs
- ComponentChangedEvent.cs
- DateTimeOffsetAdapter.cs
- Atom10FormatterFactory.cs
- ZipIOLocalFileHeader.cs
- XPathParser.cs
- XPathNavigator.cs
- MediaTimeline.cs
- RuntimeHandles.cs
- WebPartZoneBase.cs
- SchemaSetCompiler.cs
- Timer.cs
- TemplateBuilder.cs
- SimpleFileLog.cs
- Marshal.cs
- Menu.cs
- FacetDescription.cs
- TypedTableBase.cs
- Filter.cs
- LayoutTableCell.cs
- QueryCacheManager.cs
- EdmEntityTypeAttribute.cs
- BitmapMetadata.cs
- PlatformNotSupportedException.cs
- InputScopeAttribute.cs
- OleDbRowUpdatingEvent.cs
- MemberAssignment.cs
- TreeViewImageIndexConverter.cs
- Odbc32.cs
- RIPEMD160Managed.cs
- DependencyPropertyValueSerializer.cs
- DbParameterCollection.cs
- BroadcastEventHelper.cs
- WinHttpWebProxyFinder.cs
- ObjectContextServiceProvider.cs
- ExecutionEngineException.cs
- Overlapped.cs
- SqlTriggerAttribute.cs
- MemberMaps.cs
- RawStylusInputCustomDataList.cs
- TextReader.cs
- TextChange.cs
- SecurityTokenSerializer.cs
- StrokeIntersection.cs
- CompilationRelaxations.cs
- DispatcherProcessingDisabled.cs
- StylusPointPropertyId.cs
- HierarchicalDataTemplate.cs
- ContextStaticAttribute.cs
- DataGridComboBoxColumn.cs
- Error.cs
- FilterFactory.cs
- DataGridViewSortCompareEventArgs.cs
- ComEventsMethod.cs
- TextFormatter.cs
- RsaSecurityToken.cs
- InfoCardKeyedHashAlgorithm.cs
- SpotLight.cs
- _ListenerResponseStream.cs
- TextTreeRootNode.cs
- RectIndependentAnimationStorage.cs
- DBPropSet.cs
- ProtectedConfiguration.cs
- LazyInitializer.cs
- DataRow.cs
- DefaultExpressionVisitor.cs
- RepeatBehavior.cs
- BaseCAMarshaler.cs
- RoutedUICommand.cs
- GroupBox.cs
- DataControlPagerLinkButton.cs
- ToolStripContainerDesigner.cs
- ProvideValueServiceProvider.cs
- QilLoop.cs
- SerializableAttribute.cs
- Label.cs
- DataRecord.cs
- XmlUTF8TextWriter.cs
- ScriptManager.cs
- CompositeKey.cs