Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / ManagedLibraries / Remoting / Channels / CORE / BasicAsyncResult.cs / 1305376 / BasicAsyncResult.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** File: BasicAsyncResult.cs ** ** Purpose: Base class for async result implementations. ** ** Date: Oct 21, 2001 ** ===========================================================*/ using System; using System.Threading; namespace System.Runtime.Remoting.Channels { internal class BasicAsyncResult : IAsyncResult { private AsyncCallback _asyncCallback; private Object _asyncState; private Object _returnValue; private Exception _exception; private bool _bIsComplete; private ManualResetEvent _manualResetEvent; internal BasicAsyncResult(AsyncCallback callback, Object state) { _asyncCallback = callback; _asyncState = state; } // BasicAsyncResult public Object AsyncState { get { return _asyncState; } } // AsyncState public WaitHandle AsyncWaitHandle { get { bool bSavedIsComplete = _bIsComplete; if (_manualResetEvent == null) { lock (this) { if (_manualResetEvent == null) { _manualResetEvent = new ManualResetEvent(bSavedIsComplete); } } } if (!bSavedIsComplete && _bIsComplete) _manualResetEvent.Set(); return (WaitHandle)_manualResetEvent; } } // AsyncWaitHandle public bool CompletedSynchronously { get { return false; } } // CompletedSynchronously public bool IsCompleted { get { return _bIsComplete; } } // IsCompleted internal Exception Exception { get { return _exception; } } internal void SetComplete(Object returnValue, Exception exception) { _returnValue = returnValue; _exception = exception; CleanupOnComplete(); _bIsComplete = true; try { if (_manualResetEvent != null) _manualResetEvent.Set(); } catch (Exception e) { if (_exception == null) _exception = e; } // invoke the callback if (_asyncCallback != null) _asyncCallback(this); } // SetComplete internal virtual void CleanupOnComplete() { } } // BasicAsyncResult } // namespace System.Runtime.Remoting.Channels // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** File: BasicAsyncResult.cs ** ** Purpose: Base class for async result implementations. ** ** Date: Oct 21, 2001 ** ===========================================================*/ using System; using System.Threading; namespace System.Runtime.Remoting.Channels { internal class BasicAsyncResult : IAsyncResult { private AsyncCallback _asyncCallback; private Object _asyncState; private Object _returnValue; private Exception _exception; private bool _bIsComplete; private ManualResetEvent _manualResetEvent; internal BasicAsyncResult(AsyncCallback callback, Object state) { _asyncCallback = callback; _asyncState = state; } // BasicAsyncResult public Object AsyncState { get { return _asyncState; } } // AsyncState public WaitHandle AsyncWaitHandle { get { bool bSavedIsComplete = _bIsComplete; if (_manualResetEvent == null) { lock (this) { if (_manualResetEvent == null) { _manualResetEvent = new ManualResetEvent(bSavedIsComplete); } } } if (!bSavedIsComplete && _bIsComplete) _manualResetEvent.Set(); return (WaitHandle)_manualResetEvent; } } // AsyncWaitHandle public bool CompletedSynchronously { get { return false; } } // CompletedSynchronously public bool IsCompleted { get { return _bIsComplete; } } // IsCompleted internal Exception Exception { get { return _exception; } } internal void SetComplete(Object returnValue, Exception exception) { _returnValue = returnValue; _exception = exception; CleanupOnComplete(); _bIsComplete = true; try { if (_manualResetEvent != null) _manualResetEvent.Set(); } catch (Exception e) { if (_exception == null) _exception = e; } // invoke the callback if (_asyncCallback != null) _asyncCallback(this); } // SetComplete internal virtual void CleanupOnComplete() { } } // BasicAsyncResult } // namespace System.Runtime.Remoting.Channels // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
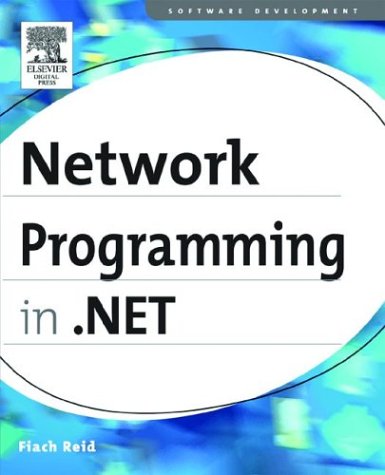
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemplateXamlTreeBuilder.cs
- CompatibleComparer.cs
- PersonalizationProviderCollection.cs
- Storyboard.cs
- ProcessHostFactoryHelper.cs
- TranslateTransform.cs
- EventLog.cs
- InternalsVisibleToAttribute.cs
- RightsManagementEncryptionTransform.cs
- ScrollBar.cs
- Attribute.cs
- DrawingGroup.cs
- SqlExpander.cs
- SchemaElementDecl.cs
- ManagementObjectCollection.cs
- ExpressionBuilder.cs
- EdmError.cs
- PkcsMisc.cs
- EncoderParameters.cs
- DoubleLink.cs
- CancellationTokenSource.cs
- ISFClipboardData.cs
- KnownIds.cs
- RestHandlerFactory.cs
- DirectoryNotFoundException.cs
- ParserExtension.cs
- recordstatescratchpad.cs
- ImageMetadata.cs
- Property.cs
- AbstractExpressions.cs
- DefinitionBase.cs
- XmlnsPrefixAttribute.cs
- Timer.cs
- ImpersonateTokenRef.cs
- EventSetter.cs
- BuildProvidersCompiler.cs
- SecUtil.cs
- TextServicesDisplayAttribute.cs
- StringToken.cs
- CFStream.cs
- SecurityBindingElementImporter.cs
- KeyedHashAlgorithm.cs
- ProfileProvider.cs
- GridLengthConverter.cs
- SchemaImporter.cs
- DetailsViewPagerRow.cs
- DiscardableAttribute.cs
- RepeaterItemCollection.cs
- SerializableAuthorizationContext.cs
- SqlCacheDependencySection.cs
- BamlRecordHelper.cs
- DoubleAnimationBase.cs
- MissingManifestResourceException.cs
- OracleCommandBuilder.cs
- FileStream.cs
- XmlSchemaAttributeGroupRef.cs
- NullPackagingPolicy.cs
- XmlSchemaValidator.cs
- VSWCFServiceContractGenerator.cs
- XmlUtf8RawTextWriter.cs
- RenderData.cs
- SmiSettersStream.cs
- UnsafeNativeMethods.cs
- PersonalizableAttribute.cs
- ScriptMethodAttribute.cs
- RequestUriProcessor.cs
- TdsParserSafeHandles.cs
- TraceUtils.cs
- TrackingDataItemValue.cs
- StringBlob.cs
- ProgressBarAutomationPeer.cs
- CharUnicodeInfo.cs
- XmlSchemaSimpleTypeUnion.cs
- VirtualPathUtility.cs
- BufferAllocator.cs
- StoreContentChangedEventArgs.cs
- FixedDSBuilder.cs
- PerspectiveCamera.cs
- XmlUnspecifiedAttribute.cs
- TrackBarRenderer.cs
- WindowProviderWrapper.cs
- FilteredAttributeCollection.cs
- LiteralSubsegment.cs
- ConstructorBuilder.cs
- ListBox.cs
- TableRowGroup.cs
- DataTable.cs
- WindowsListViewItemCheckBox.cs
- KnownColorTable.cs
- CollectionViewGroupInternal.cs
- SchemaAttDef.cs
- Grant.cs
- StylusPointPropertyUnit.cs
- MulticastNotSupportedException.cs
- FormsAuthenticationCredentials.cs
- MultiPropertyDescriptorGridEntry.cs
- XamlToRtfWriter.cs
- QueryOutputWriter.cs
- TextServicesDisplayAttribute.cs
- ProgressBar.cs