Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Documents / TextServicesDisplayAttribute.cs / 1 / TextServicesDisplayAttribute.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: TextServicesDisplayAttribute // // History: // 08/01/2003 : [....] - Ported from dotnet tree. // //--------------------------------------------------------------------------- using System.Runtime.InteropServices; using System.Windows.Threading; using System.Collections; using System.Diagnostics; using System.Windows.Media; using System.Windows.Input; using System.Windows.Documents; using MS.Win32; using System; namespace System.Windows.Documents { //----------------------------------------------------- // // TextServicesDisplayAttribute class // //----------------------------------------------------- ////// The helper class to wrap TF_DISPLAYATTRIBUTE. /// internal class TextServicesDisplayAttribute { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- internal TextServicesDisplayAttribute(UnsafeNativeMethods.TF_DISPLAYATTRIBUTE attr) { _attr = attr; } //------------------------------------------------------ // // Internal Method // //------------------------------------------------------ ////// Check if this is empty. /// internal bool IsEmptyAttribute() { if (_attr.crText.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE || _attr.crBk.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE || _attr.crLine.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE || _attr.lsStyle != UnsafeNativeMethods.TF_DA_LINESTYLE.TF_LS_NONE) return false; return true; } ////// Apply the display attribute to to the given range. /// internal void Apply(ITextPointer start, ITextPointer end) { // // #if NOT_YET if (_attr.crText.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE) { } if (_attr.crBk.type != UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_NONE) { } if (_attr.lsStyle != UnsafeNativeMethods.TF_DA_LINESTYLE.TF_LS_NONE) { } #endif } ////// Convert TF_DA_COLOR to Color. /// internal static Color GetColor(UnsafeNativeMethods.TF_DA_COLOR dacolor) { if (dacolor.type == UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_SYSCOLOR) { return GetSystemColor(dacolor.indexOrColorRef); } else if (dacolor.type == UnsafeNativeMethods.TF_DA_COLORTYPE.TF_CT_COLORREF) { int color = dacolor.indexOrColorRef; uint argb = (uint)FromWin32Value(color); return Color.FromArgb((byte)((argb&0xff000000)>>24), (byte)((argb&0x00ff0000)>>16),(byte)((argb&0x0000ff00)>>8), (byte)(argb&0x000000ff)); } return Color.FromArgb(0xff,0,0,0); } ////// Line color of the composition line draw /// internal Color LineColor { get { return GetColor(_attr.crLine); } } ////// Line style of the composition line draw /// internal UnsafeNativeMethods.TF_DA_LINESTYLE LineStyle { get { return _attr.lsStyle; } } ////// Line bold of the composition line draw /// internal bool IsBoldLine { get { return _attr.fBoldLine; } } /** * Shift count and bit mask for A, R, G, B components */ private const int AlphaShift = 24; private const int RedShift = 16; private const int GreenShift = 8; private const int BlueShift = 0; private const int Win32RedShift = 0; private const int Win32GreenShift = 8; private const int Win32BlueShift = 16; private static int Encode(int alpha, int red, int green, int blue) { return red << RedShift | green << GreenShift | blue << BlueShift | alpha << AlphaShift; } private static int FromWin32Value(int value) { return Encode(255, (value >> Win32RedShift) & 0xFF, (value >> Win32GreenShift) & 0xFF, (value >> Win32BlueShift) & 0xFF); } ////// Query for system colors. /// /// Same parameter as Win32's GetSysColor ///The system color. private static Color GetSystemColor(int index) { uint argb; int color = SafeNativeMethods.GetSysColor(index); argb = (uint)FromWin32Value(color); return Color.FromArgb((byte)((argb&0xff000000)>>24), (byte)((argb&0x00ff0000)>>16),(byte)((argb&0x0000ff00)>>8), (byte)(argb&0x000000ff)); } //----------------------------------------------------- // // Private Field // //------------------------------------------------------ private UnsafeNativeMethods.TF_DISPLAYATTRIBUTE _attr; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
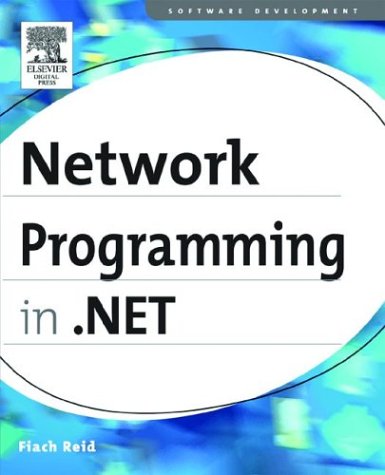
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AncestorChangedEventArgs.cs
- AssemblyCollection.cs
- FontSource.cs
- HierarchicalDataSourceControl.cs
- SQLChars.cs
- HandleCollector.cs
- ConfigurationValue.cs
- RelationshipEndCollection.cs
- COM2FontConverter.cs
- RichTextBox.cs
- TextEditorDragDrop.cs
- ElementsClipboardData.cs
- ExtendedTransformFactory.cs
- WebPartHeaderCloseVerb.cs
- ConstraintEnumerator.cs
- DictationGrammar.cs
- SerialReceived.cs
- ComboBox.cs
- FontNameConverter.cs
- DecoderFallbackWithFailureFlag.cs
- TakeOrSkipQueryOperator.cs
- __Error.cs
- GregorianCalendarHelper.cs
- WorkflowView.cs
- QuaternionAnimationUsingKeyFrames.cs
- RegistryDataKey.cs
- FieldToken.cs
- CodeEventReferenceExpression.cs
- WindowsScrollBarBits.cs
- HostDesigntimeLicenseContext.cs
- Int32KeyFrameCollection.cs
- PreservationFileReader.cs
- PlatformNotSupportedException.cs
- CorrelationToken.cs
- BasicHttpSecurityElement.cs
- LineProperties.cs
- HttpValueCollection.cs
- TaskSchedulerException.cs
- XmlSchemaSimpleTypeRestriction.cs
- PermissionAttributes.cs
- SqlParameterCollection.cs
- BindToObject.cs
- Int16Animation.cs
- ResourceFallbackManager.cs
- MarkupCompilePass2.cs
- regiisutil.cs
- HtmlElement.cs
- UIntPtr.cs
- XmlMessageFormatter.cs
- ServiceDebugBehavior.cs
- DataServiceQueryOfT.cs
- ImageBrush.cs
- Listbox.cs
- HMACSHA256.cs
- ResourcePermissionBase.cs
- TrailingSpaceComparer.cs
- LoadWorkflowByKeyAsyncResult.cs
- ChangeToolStripParentVerb.cs
- ProfilePropertySettingsCollection.cs
- PeerCustomResolverSettings.cs
- SubpageParaClient.cs
- CodeMemberEvent.cs
- DataTableCollection.cs
- TypeTypeConverter.cs
- HandlerFactoryWrapper.cs
- DataBindingCollection.cs
- ButtonBaseAdapter.cs
- TagPrefixInfo.cs
- XmlSchemaObjectTable.cs
- NumberFunctions.cs
- TextLineResult.cs
- IconHelper.cs
- Executor.cs
- ContentFileHelper.cs
- WebRequestModuleElement.cs
- SQLUtility.cs
- CroppedBitmap.cs
- TreePrinter.cs
- AddInEnvironment.cs
- PolyQuadraticBezierSegmentFigureLogic.cs
- GrammarBuilder.cs
- Size3D.cs
- DbProviderSpecificTypePropertyAttribute.cs
- TextRunCache.cs
- RequestBringIntoViewEventArgs.cs
- StoreAnnotationsMap.cs
- PerformanceCounterNameAttribute.cs
- DbConnectionClosed.cs
- XmlValidatingReader.cs
- Triplet.cs
- TimeSpanValidator.cs
- ReferenceService.cs
- MdiWindowListStrip.cs
- StronglyTypedResourceBuilder.cs
- recordstate.cs
- IRCollection.cs
- DispatcherFrame.cs
- RotationValidation.cs
- Viewport3DVisual.cs
- Quad.cs