Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Extensions / UI / ScriptResourceMapping.cs / 1305376 / ScriptResourceMapping.cs
namespace System.Web.UI { using System; using System.Collections.Concurrent; using System.Globalization; using System.Reflection; using System.Web.Resources; using System.Web.Util; public class ScriptResourceMapping : IScriptResourceMapping { private ConcurrentDictionary, ScriptResourceDefinition> _definitions = new ConcurrentDictionary , ScriptResourceDefinition>(); public void AddDefinition(string name, ScriptResourceDefinition definition) { AddDefinition(name, null, definition); } public void AddDefinition(string name, Assembly assembly, ScriptResourceDefinition definition) { // dictionary indexer will update the value if it already exists if (String.IsNullOrEmpty(name)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "name"); } if (definition == null) { throw new ArgumentNullException("definition"); } if (String.IsNullOrEmpty(definition.ResourceName) && String.IsNullOrEmpty(definition.Path)) { throw new ArgumentException(AtlasWeb.ScriptResourceDefinition_NameAndPathCannotBeEmpty, "definition"); } EnsureAbsoluteOrAppRelative(definition.Path); EnsureAbsoluteOrAppRelative(definition.DebugPath); EnsureAbsoluteOrAppRelative(definition.CdnPath); EnsureAbsoluteOrAppRelative(definition.CdnDebugPath); _definitions[new Tuple (name, assembly)] = definition; } public void Clear() { _definitions.Clear(); } private void EnsureAbsoluteOrAppRelative(string path) { if (!String.IsNullOrEmpty(path) && !UrlPath.IsAppRelativePath(path) && // ~/foo.. !UrlPath.IsRooted(path) && // /foo !Uri.IsWellFormedUriString(path, UriKind.Absolute)) { // http://... throw new InvalidOperationException( String.Format(CultureInfo.InvariantCulture, AtlasWeb.ScriptResourceDefinition_InvalidPath, path)); } } public ScriptResourceDefinition GetDefinition(string name, Assembly assembly) { if (String.IsNullOrEmpty(name)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "name"); } ScriptResourceDefinition definition; _definitions.TryGetValue(new Tuple (name, assembly), out definition); return definition; } public ScriptResourceDefinition GetDefinition(ScriptReference scriptReference) { if (scriptReference == null) { throw new ArgumentNullException("scriptReference"); } string name = scriptReference.Name; Assembly assembly = null; ScriptResourceDefinition definition = null; if (!String.IsNullOrEmpty(name)) { assembly = scriptReference.GetAssembly(); if ((assembly != null) && AssemblyCache.IsAjaxFrameworkAssembly(assembly)) { assembly = null; } definition = ScriptManager.ScriptResourceMapping.GetDefinition(name, assembly); } return definition; } public ScriptResourceDefinition RemoveDefinition(string name, Assembly assembly) { if (String.IsNullOrEmpty(name)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "name"); } ScriptResourceDefinition definition; _definitions.TryRemove(new Tuple (name, assembly), out definition); return definition; } #region IScriptResourceMapping Members IScriptResourceDefinition IScriptResourceMapping.GetDefinition(string name, Assembly assembly) { return GetDefinition(name, assembly); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Web.UI { using System; using System.Collections.Concurrent; using System.Globalization; using System.Reflection; using System.Web.Resources; using System.Web.Util; public class ScriptResourceMapping : IScriptResourceMapping { private ConcurrentDictionary , ScriptResourceDefinition> _definitions = new ConcurrentDictionary , ScriptResourceDefinition>(); public void AddDefinition(string name, ScriptResourceDefinition definition) { AddDefinition(name, null, definition); } public void AddDefinition(string name, Assembly assembly, ScriptResourceDefinition definition) { // dictionary indexer will update the value if it already exists if (String.IsNullOrEmpty(name)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "name"); } if (definition == null) { throw new ArgumentNullException("definition"); } if (String.IsNullOrEmpty(definition.ResourceName) && String.IsNullOrEmpty(definition.Path)) { throw new ArgumentException(AtlasWeb.ScriptResourceDefinition_NameAndPathCannotBeEmpty, "definition"); } EnsureAbsoluteOrAppRelative(definition.Path); EnsureAbsoluteOrAppRelative(definition.DebugPath); EnsureAbsoluteOrAppRelative(definition.CdnPath); EnsureAbsoluteOrAppRelative(definition.CdnDebugPath); _definitions[new Tuple (name, assembly)] = definition; } public void Clear() { _definitions.Clear(); } private void EnsureAbsoluteOrAppRelative(string path) { if (!String.IsNullOrEmpty(path) && !UrlPath.IsAppRelativePath(path) && // ~/foo.. !UrlPath.IsRooted(path) && // /foo !Uri.IsWellFormedUriString(path, UriKind.Absolute)) { // http://... throw new InvalidOperationException( String.Format(CultureInfo.InvariantCulture, AtlasWeb.ScriptResourceDefinition_InvalidPath, path)); } } public ScriptResourceDefinition GetDefinition(string name, Assembly assembly) { if (String.IsNullOrEmpty(name)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "name"); } ScriptResourceDefinition definition; _definitions.TryGetValue(new Tuple (name, assembly), out definition); return definition; } public ScriptResourceDefinition GetDefinition(ScriptReference scriptReference) { if (scriptReference == null) { throw new ArgumentNullException("scriptReference"); } string name = scriptReference.Name; Assembly assembly = null; ScriptResourceDefinition definition = null; if (!String.IsNullOrEmpty(name)) { assembly = scriptReference.GetAssembly(); if ((assembly != null) && AssemblyCache.IsAjaxFrameworkAssembly(assembly)) { assembly = null; } definition = ScriptManager.ScriptResourceMapping.GetDefinition(name, assembly); } return definition; } public ScriptResourceDefinition RemoveDefinition(string name, Assembly assembly) { if (String.IsNullOrEmpty(name)) { throw new ArgumentException(AtlasWeb.Common_NullOrEmpty, "name"); } ScriptResourceDefinition definition; _definitions.TryRemove(new Tuple (name, assembly), out definition); return definition; } #region IScriptResourceMapping Members IScriptResourceDefinition IScriptResourceMapping.GetDefinition(string name, Assembly assembly) { return GetDefinition(name, assembly); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
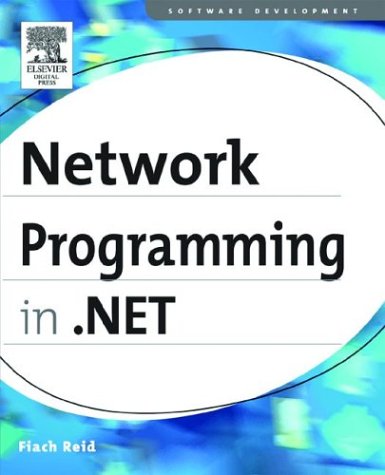
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DefaultAsyncDataDispatcher.cs
- SizeFConverter.cs
- DeflateEmulationStream.cs
- UserUseLicenseDictionaryLoader.cs
- NGCSerializationManagerAsync.cs
- BitmapEffectDrawing.cs
- Emitter.cs
- XmlWellformedWriterHelpers.cs
- ReadOnlyCollection.cs
- CharacterMetricsDictionary.cs
- HTMLTextWriter.cs
- Int16AnimationUsingKeyFrames.cs
- DelegatedStream.cs
- TextEncodedRawTextWriter.cs
- WindowsListViewItemCheckBox.cs
- activationcontext.cs
- SystemIPAddressInformation.cs
- DirtyTextRange.cs
- HuffmanTree.cs
- EncoderExceptionFallback.cs
- linebase.cs
- DashStyle.cs
- UserNamePasswordServiceCredential.cs
- ContainerControl.cs
- ImportContext.cs
- PropertyOrder.cs
- ListBase.cs
- _NTAuthentication.cs
- mda.cs
- Tokenizer.cs
- DataTableTypeConverter.cs
- DomainConstraint.cs
- UrlMappingsSection.cs
- METAHEADER.cs
- WorkflowElementDialog.cs
- SubclassTypeValidator.cs
- BuildDependencySet.cs
- UIElementPropertyUndoUnit.cs
- WindowClosedEventArgs.cs
- TraceEventCache.cs
- DataGridColumn.cs
- XsltSettings.cs
- DataRecordInternal.cs
- HighlightVisual.cs
- SHA384.cs
- DataGridCellInfo.cs
- InternalPolicyElement.cs
- ProfileSettings.cs
- DataListItemCollection.cs
- EventBuilder.cs
- TemplatedAdorner.cs
- RepeaterDesigner.cs
- SqlWorkflowInstanceStoreLock.cs
- BaseTemplateParser.cs
- BinaryWriter.cs
- DocumentViewerBaseAutomationPeer.cs
- FigureParagraph.cs
- HttpWebResponse.cs
- ADMembershipUser.cs
- PackageDigitalSignatureManager.cs
- WindowInteractionStateTracker.cs
- XmlComplianceUtil.cs
- RunInstallerAttribute.cs
- ExpressionBindingCollection.cs
- PrintPageEvent.cs
- SizeAnimationBase.cs
- BitVector32.cs
- DataContractAttribute.cs
- MethodCallTranslator.cs
- XmlSchemaCollection.cs
- TraceUtils.cs
- ResourceCodeDomSerializer.cs
- Int32Storage.cs
- HttpModule.cs
- SecurityUtils.cs
- WebPartAuthorizationEventArgs.cs
- CodeNamespaceImportCollection.cs
- MaterialCollection.cs
- RemotingSurrogateSelector.cs
- Padding.cs
- CheckBox.cs
- PipelineModuleStepContainer.cs
- ToolStripItemCollection.cs
- Journaling.cs
- DiscoveryVersion.cs
- EditBehavior.cs
- RegistrySecurity.cs
- DiscoveryServiceExtension.cs
- DeliveryStrategy.cs
- HttpHandlersInstallComponent.cs
- EntityDataSourceContextDisposingEventArgs.cs
- ServiceEndpoint.cs
- WorkflowServiceNamespace.cs
- TableRow.cs
- AdapterDictionary.cs
- OracleTransaction.cs
- TextRunCache.cs
- SystemIcmpV6Statistics.cs
- FrameworkElementAutomationPeer.cs
- ping.cs