Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / DataGridCellInfo.cs / 1305600 / DataGridCellInfo.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; namespace System.Windows.Controls { ////// Defines a structure that identifies a specific cell based on row and column data. /// public struct DataGridCellInfo { ////// Identifies a cell at the column within the row for the specified item. /// /// The item who's row contains the cell. /// The column of the cell within the row. ////// This constructor will not tie the DataGridCellInfo to any particular /// DataGrid. /// public DataGridCellInfo(object item, DataGridColumn column) { if (column == null) { throw new ArgumentNullException("column"); } _item = item; _column = column; _owner = null; } ////// Creates a structure that identifies the specific cell container. /// /// /// A reference to a cell. /// This structure does not maintain a strong reference to the cell. /// Changes to the cell will not affect this structure. /// ////// This constructor will tie the DataGridCellInfo to the specific /// DataGrid that owns the cell container. /// public DataGridCellInfo(DataGridCell cell) { if (cell == null) { throw new ArgumentNullException("cell"); } _item = cell.RowDataItem; _column = cell.Column; _owner = new WeakReference(cell.DataGridOwner); } internal DataGridCellInfo(object item, DataGridColumn column, DataGrid owner) { Debug.Assert(item != null, "item should not be null."); Debug.Assert(column != null, "column should not be null."); Debug.Assert(owner != null, "owner should not be null."); _item = item; _column = column; _owner = new WeakReference(owner); } ////// Used to create an unset DataGridCellInfo. /// internal DataGridCellInfo(object item) { Debug.Assert(item == DependencyProperty.UnsetValue, "This should only be used to make an Unset CellInfo."); _item = item; _column = null; _owner = null; } ////// This is used strictly to create the partial CellInfos. /// ////// This is being kept private so that it is explicit that the /// caller expects invalid data. /// private DataGridCellInfo(DataGrid owner, DataGridColumn column, object item) { Debug.Assert(owner != null, "owner should not be null."); _item = item; _column = column; _owner = new WeakReference(owner); } ////// This is used by CurrentCell if there isn't a valid CurrentItem or CurrentColumn. /// internal static DataGridCellInfo CreatePossiblyPartialCellInfo(object item, DataGridColumn column, DataGrid owner) { Debug.Assert(owner != null, "owner should not be null."); if ((item == null) && (column == null)) { return Unset; } else { return new DataGridCellInfo(owner, column, (item == null) ? DependencyProperty.UnsetValue : item); } } ////// The item who's row contains the cell. /// public object Item { get { return _item; } } ////// The column of the cell within the row. /// public DataGridColumn Column { get { return _column; } } ////// Whether the two objects are equal. /// public override bool Equals(object obj) { if (obj is DataGridCellInfo) { return EqualsImpl((DataGridCellInfo)obj); } return false; } ////// Returns whether the two values are equal. /// public static bool operator ==(DataGridCellInfo cell1, DataGridCellInfo cell2) { return cell1.EqualsImpl(cell2); } ////// Returns whether the two values are not equal. /// public static bool operator !=(DataGridCellInfo cell1, DataGridCellInfo cell2) { return !cell1.EqualsImpl(cell2); } internal bool EqualsImpl(DataGridCellInfo cell) { return (cell._item == _item) && (cell._column == _column) && (cell.Owner == Owner); } ////// Returns a hash code for the structure. /// public override int GetHashCode() { return ((_item == null) ? 0 : _item.GetHashCode()) ^ ((_column == null) ? 0 : _column.GetHashCode()); } ////// Whether the structure holds valid information. /// public bool IsValid { get { return ArePropertyValuesValid #if STRICT_VALIDATION && ColumnInDataGrid && ItemInDataGrid #endif ; } } ////// Assumes that if the owner matches, then the column and item fields are valid. /// internal bool IsValidForDataGrid(DataGrid dataGrid) { DataGrid owner = Owner; return (ArePropertyValuesValid && (owner == dataGrid)) || (owner == null); } private bool ArePropertyValuesValid { get { return (_item != DependencyProperty.UnsetValue) && (_column != null); } } #if STRICT_VALIDATION private bool ColumnInDataGrid { get { DataGrid owner = Owner; if (owner != null) { return owner.Columns.Contains(_column); } return true; } } private bool ItemInDataGrid { get { DataGrid owner = Owner; if (owner != null) { owner.Items.Contains(_item); } return true; } } #endif ////// Used for default values. /// internal static DataGridCellInfo Unset { get { return new DataGridCellInfo(DependencyProperty.UnsetValue); } } private DataGrid Owner { get { if (_owner != null) { return (DataGrid)_owner.Target; } return null; } } private object _item; private DataGridColumn _column; private WeakReference _owner; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; namespace System.Windows.Controls { ////// Defines a structure that identifies a specific cell based on row and column data. /// public struct DataGridCellInfo { ////// Identifies a cell at the column within the row for the specified item. /// /// The item who's row contains the cell. /// The column of the cell within the row. ////// This constructor will not tie the DataGridCellInfo to any particular /// DataGrid. /// public DataGridCellInfo(object item, DataGridColumn column) { if (column == null) { throw new ArgumentNullException("column"); } _item = item; _column = column; _owner = null; } ////// Creates a structure that identifies the specific cell container. /// /// /// A reference to a cell. /// This structure does not maintain a strong reference to the cell. /// Changes to the cell will not affect this structure. /// ////// This constructor will tie the DataGridCellInfo to the specific /// DataGrid that owns the cell container. /// public DataGridCellInfo(DataGridCell cell) { if (cell == null) { throw new ArgumentNullException("cell"); } _item = cell.RowDataItem; _column = cell.Column; _owner = new WeakReference(cell.DataGridOwner); } internal DataGridCellInfo(object item, DataGridColumn column, DataGrid owner) { Debug.Assert(item != null, "item should not be null."); Debug.Assert(column != null, "column should not be null."); Debug.Assert(owner != null, "owner should not be null."); _item = item; _column = column; _owner = new WeakReference(owner); } ////// Used to create an unset DataGridCellInfo. /// internal DataGridCellInfo(object item) { Debug.Assert(item == DependencyProperty.UnsetValue, "This should only be used to make an Unset CellInfo."); _item = item; _column = null; _owner = null; } ////// This is used strictly to create the partial CellInfos. /// ////// This is being kept private so that it is explicit that the /// caller expects invalid data. /// private DataGridCellInfo(DataGrid owner, DataGridColumn column, object item) { Debug.Assert(owner != null, "owner should not be null."); _item = item; _column = column; _owner = new WeakReference(owner); } ////// This is used by CurrentCell if there isn't a valid CurrentItem or CurrentColumn. /// internal static DataGridCellInfo CreatePossiblyPartialCellInfo(object item, DataGridColumn column, DataGrid owner) { Debug.Assert(owner != null, "owner should not be null."); if ((item == null) && (column == null)) { return Unset; } else { return new DataGridCellInfo(owner, column, (item == null) ? DependencyProperty.UnsetValue : item); } } ////// The item who's row contains the cell. /// public object Item { get { return _item; } } ////// The column of the cell within the row. /// public DataGridColumn Column { get { return _column; } } ////// Whether the two objects are equal. /// public override bool Equals(object obj) { if (obj is DataGridCellInfo) { return EqualsImpl((DataGridCellInfo)obj); } return false; } ////// Returns whether the two values are equal. /// public static bool operator ==(DataGridCellInfo cell1, DataGridCellInfo cell2) { return cell1.EqualsImpl(cell2); } ////// Returns whether the two values are not equal. /// public static bool operator !=(DataGridCellInfo cell1, DataGridCellInfo cell2) { return !cell1.EqualsImpl(cell2); } internal bool EqualsImpl(DataGridCellInfo cell) { return (cell._item == _item) && (cell._column == _column) && (cell.Owner == Owner); } ////// Returns a hash code for the structure. /// public override int GetHashCode() { return ((_item == null) ? 0 : _item.GetHashCode()) ^ ((_column == null) ? 0 : _column.GetHashCode()); } ////// Whether the structure holds valid information. /// public bool IsValid { get { return ArePropertyValuesValid #if STRICT_VALIDATION && ColumnInDataGrid && ItemInDataGrid #endif ; } } ////// Assumes that if the owner matches, then the column and item fields are valid. /// internal bool IsValidForDataGrid(DataGrid dataGrid) { DataGrid owner = Owner; return (ArePropertyValuesValid && (owner == dataGrid)) || (owner == null); } private bool ArePropertyValuesValid { get { return (_item != DependencyProperty.UnsetValue) && (_column != null); } } #if STRICT_VALIDATION private bool ColumnInDataGrid { get { DataGrid owner = Owner; if (owner != null) { return owner.Columns.Contains(_column); } return true; } } private bool ItemInDataGrid { get { DataGrid owner = Owner; if (owner != null) { owner.Items.Contains(_item); } return true; } } #endif ////// Used for default values. /// internal static DataGridCellInfo Unset { get { return new DataGridCellInfo(DependencyProperty.UnsetValue); } } private DataGrid Owner { get { if (_owner != null) { return (DataGrid)_owner.Target; } return null; } } private object _item; private DataGridColumn _column; private WeakReference _owner; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
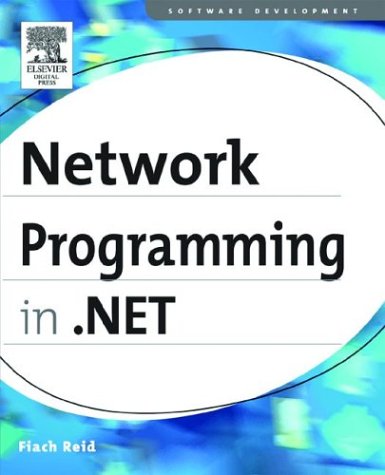
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartMinimizeVerb.cs
- DataViewSettingCollection.cs
- WebPartsSection.cs
- SpecialTypeDataContract.cs
- FontNamesConverter.cs
- UDPClient.cs
- PinnedBufferMemoryStream.cs
- Boolean.cs
- SecondaryViewProvider.cs
- ImportedNamespaceContextItem.cs
- ThreadStateException.cs
- dataSvcMapFileLoader.cs
- ActivityCodeDomSerializer.cs
- QilStrConcatenator.cs
- FixedFlowMap.cs
- DataPagerFieldItem.cs
- RefType.cs
- BackoffTimeoutHelper.cs
- StyleBamlRecordReader.cs
- ApplicationInterop.cs
- __Error.cs
- AsyncOperationManager.cs
- XmlRootAttribute.cs
- ClientSettingsStore.cs
- ObjectFullSpanRewriter.cs
- SharedPersonalizationStateInfo.cs
- SystemUnicastIPAddressInformation.cs
- DecoderFallbackWithFailureFlag.cs
- RepeaterItemEventArgs.cs
- ReceiveSecurityHeaderElementManager.cs
- ItemsPresenter.cs
- counter.cs
- WindowsUpDown.cs
- DrawItemEvent.cs
- WebZone.cs
- PropertyIDSet.cs
- ToolStripControlHost.cs
- VectorCollection.cs
- SessionIDManager.cs
- PropertyChangeTracker.cs
- GenericIdentity.cs
- translator.cs
- GridItemPattern.cs
- InvalidWMPVersionException.cs
- IntSecurity.cs
- KoreanCalendar.cs
- smtppermission.cs
- ToolStripSeparator.cs
- Utility.cs
- GridViewDeleteEventArgs.cs
- EnlistmentTraceIdentifier.cs
- NetworkCredential.cs
- XamlFilter.cs
- TextViewBase.cs
- TaskHelper.cs
- Int32AnimationBase.cs
- SessionPageStateSection.cs
- FontInfo.cs
- CodeArrayCreateExpression.cs
- MetadataPropertyvalue.cs
- ParseElementCollection.cs
- ToolStripItemEventArgs.cs
- ILGenerator.cs
- ImmutableDispatchRuntime.cs
- ServiceDescriptionReflector.cs
- HMACSHA512.cs
- DeclarativeCatalogPart.cs
- SessionPageStatePersister.cs
- ApplicationServicesHostFactory.cs
- InfoCardRSAOAEPKeyExchangeDeformatter.cs
- EmptyImpersonationContext.cs
- UnauthorizedWebPart.cs
- ProtectedConfigurationSection.cs
- SafeNativeMethods.cs
- HtmlInputButton.cs
- FileEnumerator.cs
- TextFragmentEngine.cs
- PropertyMapper.cs
- RadioButtonDesigner.cs
- InfoCardArgumentException.cs
- PackageDigitalSignatureManager.cs
- WebPartRestoreVerb.cs
- URLString.cs
- ImageDrawing.cs
- DoubleCollectionConverter.cs
- ObjectToken.cs
- WindowsListViewItemStartMenu.cs
- LinqDataSourceView.cs
- ByteAnimation.cs
- InertiaRotationBehavior.cs
- PeerNameRegistration.cs
- XmlTextAttribute.cs
- InputScopeConverter.cs
- CreateUserWizardStep.cs
- PropertyItem.cs
- ProfileSection.cs
- ISAPIRuntime.cs
- PixelFormatConverter.cs
- Identity.cs
- PlatformNotSupportedException.cs