Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Extensions / Compilation / WCFModel / dataSvcMapFileLoader.cs / 1305376 / dataSvcMapFileLoader.cs
//------------------------------------------------------------------------------ //// Copyright (C) Microsoft Corporation. All Rights Reserved. // //----------------------------------------------------------------------------- // // Note: Unlike most files in this directory, the code in this file is *not* // shared with wizard\vsdesigner\designer\microsoft\vsdesigner\WCFModel. // // The code under ndp\fx\src\xsp\System\Web\Extensions\Compilation\XmlSerializer might have to be regerenated when // the format of the svcmap file is changed, or class structure has been changed in this directory. Please follow the HowTo file // under Compilation directory to see how to regerenate that code. // using System; using System.Collections.Generic; using System.Text; #if WEB_EXTENSIONS_CODE using System.Web.Resources; #else using Microsoft.VSDesigner.Resources.Microsoft.VSDesigner; #endif namespace System.Web.Compilation.WCFModel { internal class DataSvcMapFileLoader : AbstractDataSvcMapFileLoader { private string mapFilePath; public DataSvcMapFileLoader(string mapFilePath) { this.mapFilePath = mapFilePath; } ////// Given a name of a metadata file, returns the full expected path to the file. /// /// ///private string GetMetadataFileFullPath(string name) { // Should be in the same directory as the .svcmap file. return IO.Path.Combine(IO.Path.GetDirectoryName(mapFilePath), name); } /// /// Get a TextReader for the .svcmap file /// ///protected override System.IO.TextReader GetMapFileReader() { return IO.File.OpenText(mapFilePath); } /// /// Read the contents of the given metadata file /// /// The filename (without path) of the metadata file. ///protected override byte[] ReadMetadataFile(string name) { return IO.File.ReadAllBytes(GetMetadataFileFullPath(name)); } /// /// Get access to a byte array that contain the contents of the given extension /// file /// /// /// Name of the extension file. Could be a path relative to the svcmap file location /// or the name of an item in a metadata storage. /// ///protected override byte[] ReadExtensionFile(string name) { return IO.File.ReadAllBytes(GetMetadataFileFullPath(name)); } /// /// Demand-create an XmlSerializer for the SvcMap file... /// protected override System.Xml.Serialization.XmlSerializer Serializer { get { if (serializer == null) { // We have our own pre-generated XML serializer for the .datasvcmap file. serializer = new System.Web.Compilation.XmlSerializerDataSvc.DataSvcMapFileSerializer(); } return serializer; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (C) Microsoft Corporation. All Rights Reserved. // //----------------------------------------------------------------------------- // // Note: Unlike most files in this directory, the code in this file is *not* // shared with wizard\vsdesigner\designer\microsoft\vsdesigner\WCFModel. // // The code under ndp\fx\src\xsp\System\Web\Extensions\Compilation\XmlSerializer might have to be regerenated when // the format of the svcmap file is changed, or class structure has been changed in this directory. Please follow the HowTo file // under Compilation directory to see how to regerenate that code. // using System; using System.Collections.Generic; using System.Text; #if WEB_EXTENSIONS_CODE using System.Web.Resources; #else using Microsoft.VSDesigner.Resources.Microsoft.VSDesigner; #endif namespace System.Web.Compilation.WCFModel { internal class DataSvcMapFileLoader : AbstractDataSvcMapFileLoader { private string mapFilePath; public DataSvcMapFileLoader(string mapFilePath) { this.mapFilePath = mapFilePath; } ////// Given a name of a metadata file, returns the full expected path to the file. /// /// ///private string GetMetadataFileFullPath(string name) { // Should be in the same directory as the .svcmap file. return IO.Path.Combine(IO.Path.GetDirectoryName(mapFilePath), name); } /// /// Get a TextReader for the .svcmap file /// ///protected override System.IO.TextReader GetMapFileReader() { return IO.File.OpenText(mapFilePath); } /// /// Read the contents of the given metadata file /// /// The filename (without path) of the metadata file. ///protected override byte[] ReadMetadataFile(string name) { return IO.File.ReadAllBytes(GetMetadataFileFullPath(name)); } /// /// Get access to a byte array that contain the contents of the given extension /// file /// /// /// Name of the extension file. Could be a path relative to the svcmap file location /// or the name of an item in a metadata storage. /// ///protected override byte[] ReadExtensionFile(string name) { return IO.File.ReadAllBytes(GetMetadataFileFullPath(name)); } /// /// Demand-create an XmlSerializer for the SvcMap file... /// protected override System.Xml.Serialization.XmlSerializer Serializer { get { if (serializer == null) { // We have our own pre-generated XML serializer for the .datasvcmap file. serializer = new System.Web.Compilation.XmlSerializerDataSvc.DataSvcMapFileSerializer(); } return serializer; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
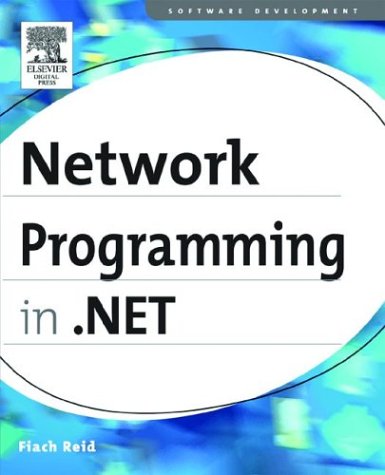
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SessionIDManager.cs
- XPathScanner.cs
- SqlInternalConnection.cs
- DbProviderConfigurationHandler.cs
- ConfigDefinitionUpdates.cs
- SingleObjectCollection.cs
- filewebrequest.cs
- Light.cs
- TextDecorationLocationValidation.cs
- Int64Storage.cs
- InvalidAsynchronousStateException.cs
- CacheSection.cs
- RowSpanVector.cs
- ReflectionServiceProvider.cs
- RadioButtonStandardAdapter.cs
- CTreeGenerator.cs
- TextTreeRootNode.cs
- ArrangedElementCollection.cs
- DataListItem.cs
- Timer.cs
- TextEvent.cs
- DataGridViewColumn.cs
- DataTemplateSelector.cs
- UIntPtr.cs
- DeviceSpecificChoice.cs
- SimpleHandlerBuildProvider.cs
- GroupItemAutomationPeer.cs
- BamlLocalizer.cs
- WebBaseEventKeyComparer.cs
- TextEditorMouse.cs
- GeometryGroup.cs
- DataSourceSerializationException.cs
- DataBindingExpressionBuilder.cs
- DeploymentSection.cs
- TransformConverter.cs
- XmlNodeReader.cs
- ColorConverter.cs
- FieldAccessException.cs
- Operand.cs
- WorkflowInstanceSuspendedRecord.cs
- RegexWriter.cs
- PageThemeParser.cs
- Vector3D.cs
- SetIterators.cs
- IItemContainerGenerator.cs
- HandleRef.cs
- TransformerInfoCollection.cs
- HitTestParameters.cs
- WindowsAuthenticationModule.cs
- Point3DAnimation.cs
- TextAction.cs
- CompilerScope.Storage.cs
- SizeAnimation.cs
- Region.cs
- WindowsSecurityToken.cs
- NotifyCollectionChangedEventArgs.cs
- Tokenizer.cs
- ToolboxComponentsCreatedEventArgs.cs
- LinearQuaternionKeyFrame.cs
- StreamWriter.cs
- Int64Storage.cs
- OverflowException.cs
- ScrollProviderWrapper.cs
- InfoCardTrace.cs
- Soap12ProtocolImporter.cs
- GenerateTemporaryTargetAssembly.cs
- WebScriptClientGenerator.cs
- SystemIcons.cs
- PrimitiveXmlSerializers.cs
- HwndSourceKeyboardInputSite.cs
- TextTreeRootNode.cs
- TiffBitmapDecoder.cs
- DocumentGridPage.cs
- SeekableReadStream.cs
- ParameterElement.cs
- FlowDocumentScrollViewerAutomationPeer.cs
- XmlNodeComparer.cs
- Win32PrintDialog.cs
- WinInet.cs
- TransportContext.cs
- MsmqAppDomainProtocolHandler.cs
- XPathMultyIterator.cs
- BindingMAnagerBase.cs
- Soap12ProtocolImporter.cs
- SchemaElementDecl.cs
- Overlapped.cs
- CaseInsensitiveComparer.cs
- WindowsFormsHelpers.cs
- Transform.cs
- SqlNode.cs
- nulltextcontainer.cs
- DoubleAnimationUsingKeyFrames.cs
- CorePropertiesFilter.cs
- uribuilder.cs
- VisemeEventArgs.cs
- ItemMap.cs
- EncryptedPackageFilter.cs
- TranslateTransform.cs
- TrackBarRenderer.cs
- MsmqIntegrationSecurity.cs