Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / Clusters / SafeNativeMethods.cs / 1 / SafeNativeMethods.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- using System; using System.Runtime.CompilerServices; using System.Runtime.ConstrainedExecution; using System.Runtime.InteropServices; using System.Security.AccessControl; using System.Security.Permissions; using System.Text; using Microsoft.Win32; namespace Microsoft.Transactions.Wsat.Clusters { abstract class SafeClusterHandle : SafeHandle { [SecurityPermission(SecurityAction.LinkDemand, UnmanagedCode = true)] internal SafeClusterHandle() : base(IntPtr.Zero, true) { } [SecurityPermission(SecurityAction.LinkDemand, UnmanagedCode = true)] internal SafeClusterHandle(IntPtr hcluster) : base(IntPtr.Zero, true) { SetHandle(hcluster); } public override bool IsInvalid { get { return IsClosed || handle == IntPtr.Zero; } } } class SafeHCluster : SafeClusterHandle { // MSDN remarks: This function always returns TRUE. [DllImport(SafeNativeMethods.ClusApi)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] static extern bool CloseCluster([In] IntPtr hCluster); protected override bool ReleaseHandle() { return CloseCluster(handle); } } class SafeHResource : SafeClusterHandle { [DllImport(SafeNativeMethods.ClusApi)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] static extern bool CloseClusterResource([In] IntPtr hResource); protected override bool ReleaseHandle() { return CloseClusterResource(handle); } } class SafeHClusEnum : SafeClusterHandle { [DllImport(SafeNativeMethods.ClusApi)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] static extern uint ClusterCloseEnum([In] IntPtr hEnum); protected override bool ReleaseHandle() { return ClusterCloseEnum(handle) == SafeNativeMethods.ERROR_SUCCESS; } } class SafeHKey : SafeClusterHandle { [DllImport(SafeNativeMethods.ClusApi)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] static extern int ClusterRegCloseKey([In] IntPtr hEnum); protected override bool ReleaseHandle() { return ClusterRegCloseKey(handle) == SafeNativeMethods.ERROR_SUCCESS; } } [Flags] enum ClusterEnum : uint { Node = 0x00000001, ResType = 0x00000002, Resource = 0x00000004, Group = 0x00000008, Network = 0x00000010, NetInterface = 0x00000020, InternalNetwork = 0x80000000 } enum ClusterResourceControlCode : uint { GetResourceType = 0x0100002d, //GetId = 0x01000039 } class SafeNativeMethods { public const string ClusApi = "clusapi.dll"; public const uint ERROR_SUCCESS = 0; public const uint ERROR_FILE_NOT_FOUND = 2; public const uint ERROR_MORE_DATA = 234; public const uint ERROR_NO_MORE_ITEMS = 259; [DllImport(ClusApi, SetLastError = true, CharSet = CharSet.Unicode)] public static extern SafeHCluster OpenCluster( [MarshalAs(UnmanagedType.LPWStr)] [In] string lpszClusterName); [DllImport(ClusApi, SetLastError = true, CharSet = CharSet.Unicode)] public static extern SafeHClusEnum ClusterOpenEnum( [In] SafeHCluster hCluster, [In] ClusterEnum dwType); [DllImport(ClusApi, CharSet = CharSet.Unicode)] public static extern uint ClusterEnum( [In] SafeHClusEnum hEnum, [In] uint dwIndex, [Out] out uint lpdwType, [Out] StringBuilder lpszName, [In, Out] ref uint lpcchName); [DllImport(ClusApi, CharSet = CharSet.Unicode)] public static extern uint ClusterResourceControl( [In] SafeHResource hResource, [In] IntPtr hHostNode, //HNODE hHostNode, never used [In] ClusterResourceControlCode dwControlCode, [In] IntPtr lpInBuffer, // LPVOID lpInBuffer, never used [In] uint cbInBufferSize, [In, Out, MarshalAs(UnmanagedType.LPArray)] byte[] buffer, [In] uint cbOutBufferSize, [In, Out] ref uint lpcbBytesReturned); [DllImport(ClusApi, SetLastError = true, CharSet = CharSet.Unicode)] public static extern SafeHResource OpenClusterResource( [In] SafeHCluster hCluster, [In, MarshalAs(UnmanagedType.LPWStr)] string lpszResourceName); [DllImport(ClusApi, SetLastError = true, CharSet = CharSet.Unicode)] public static extern bool GetClusterResourceNetworkName( [In] SafeHResource hResource, [Out] StringBuilder lpBuffer, [In, Out] ref uint nSize); [DllImport(ClusApi, SetLastError = true, CharSet = CharSet.Unicode)] public static extern SafeHKey GetClusterResourceKey( [In] SafeHResource hResource, [In] RegistryRights samDesired); [DllImport(ClusApi, CharSet = CharSet.Unicode)] public static extern int ClusterRegOpenKey( [In] SafeHKey hKey, [In, MarshalAs(UnmanagedType.LPWStr)] string lpszSubKey, [In] RegistryRights samDesired, [Out] out SafeHKey phkResult); [DllImport(ClusApi, CharSet = CharSet.Unicode)] public static extern int ClusterRegQueryValue( [In] SafeHKey hKey, [In, MarshalAs(UnmanagedType.LPWStr)] string lpszValueName, [Out] out RegistryValueKind lpdwValueType, [In, Out, MarshalAs(UnmanagedType.LPArray)] byte[] lpbData, [In, Out] ref uint lpcbData); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
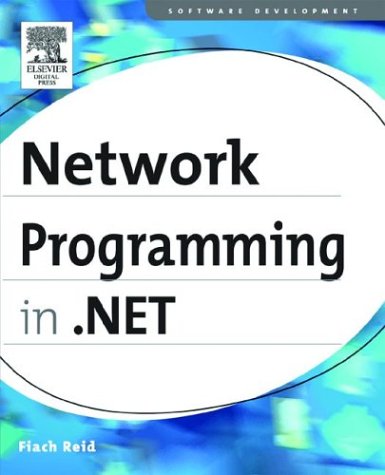
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ResourceDictionaryCollection.cs
- RegularExpressionValidator.cs
- ThreadBehavior.cs
- ClaimTypes.cs
- ScriptServiceAttribute.cs
- TypedColumnHandler.cs
- ScriptManager.cs
- TargetFrameworkAttribute.cs
- ConditionalExpression.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- IntMinMaxAggregationOperator.cs
- RegexStringValidatorAttribute.cs
- TextContainer.cs
- Expressions.cs
- DataPointer.cs
- SafeArchiveContext.cs
- PrintPreviewControl.cs
- SiteMapNodeItem.cs
- IntAverageAggregationOperator.cs
- Storyboard.cs
- Listbox.cs
- WebPartExportVerb.cs
- HttpCachePolicy.cs
- SortKey.cs
- FormatConvertedBitmap.cs
- CaseStatementProjectedSlot.cs
- RadioButtonFlatAdapter.cs
- IHttpResponseInternal.cs
- Expressions.cs
- future.cs
- DiscoveryClientElement.cs
- util.cs
- XmlSerializerSection.cs
- ImageSourceValueSerializer.cs
- Codec.cs
- AttributeCollection.cs
- PageMediaType.cs
- OrderPreservingPipeliningSpoolingTask.cs
- CaseKeyBox.ViewModel.cs
- DrawingGroup.cs
- ErrorInfoXmlDocument.cs
- BaseCAMarshaler.cs
- ObjectQuery_EntitySqlExtensions.cs
- Query.cs
- SafeBitVector32.cs
- LinkButton.cs
- SessionPageStateSection.cs
- NamespaceEmitter.cs
- WindowsIPAddress.cs
- UICuesEvent.cs
- PresentationAppDomainManager.cs
- SimpleTextLine.cs
- ByteConverter.cs
- AmbiguousMatchException.cs
- ServiceModelInstallComponent.cs
- CachingHintValidation.cs
- OletxVolatileEnlistment.cs
- COM2AboutBoxPropertyDescriptor.cs
- AspCompat.cs
- StaticTextPointer.cs
- SqlCacheDependencySection.cs
- SingleBodyParameterMessageFormatter.cs
- ColorTransformHelper.cs
- ProfileProvider.cs
- ContextBase.cs
- RectangleF.cs
- ExternalFile.cs
- DataGridCommandEventArgs.cs
- ApplicationGesture.cs
- BamlLocalizationDictionary.cs
- RadioButtonAutomationPeer.cs
- ModelUIElement3D.cs
- ConnectionsZone.cs
- XPathDocument.cs
- HtmlInputRadioButton.cs
- XmlMembersMapping.cs
- AmbientProperties.cs
- RecommendedAsConfigurableAttribute.cs
- ListControl.cs
- BookmarkTable.cs
- TypeInfo.cs
- GregorianCalendarHelper.cs
- CacheOutputQuery.cs
- MissingMethodException.cs
- Decoder.cs
- DebugView.cs
- BCLDebug.cs
- CommonXSendMessage.cs
- BufferAllocator.cs
- ProxyWebPartManagerDesigner.cs
- ProjectionAnalyzer.cs
- Cursor.cs
- FixedHighlight.cs
- PointLightBase.cs
- BinarySecretSecurityToken.cs
- TraceListeners.cs
- Section.cs
- HttpRuntime.cs
- BlockingCollection.cs
- MetadataCacheItem.cs