Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / clr / src / BCL / System / Collections / Generic / DebugView.cs / 1 / DebugView.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** ** ** Purpose: DebugView class for generic collections ** ** =============================================================================*/ namespace System.Collections.Generic { using System; using System.Collections.ObjectModel; using System.Security.Permissions; using System.Diagnostics; // // VS IDE can't differentiate between types with the same name from different // assembly. So we need to use different names for collection debug view for // collections in mscorlib.dll and system.dll. // internal sealed class Mscorlib_CollectionDebugView{ private ICollection collection; public Mscorlib_CollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { T[] items = new T[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_DictionaryKeyCollectionDebugView { private ICollection collection; public Mscorlib_DictionaryKeyCollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public TKey[] Items { get { TKey[] items = new TKey[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_DictionaryValueCollectionDebugView { private ICollection collection; public Mscorlib_DictionaryValueCollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public TValue[] Items { get { TValue[] items = new TValue[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_DictionaryDebugView { private IDictionary dict; public Mscorlib_DictionaryDebugView(IDictionary dictionary) { if (dictionary == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.dictionary); this.dict = dictionary; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public KeyValuePair [] Items { get { KeyValuePair [] items = new KeyValuePair [dict.Count]; dict.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_KeyedCollectionDebugView { private KeyedCollection kc; public Mscorlib_KeyedCollectionDebugView(KeyedCollection keyedCollection) { if (keyedCollection == null) { throw new ArgumentNullException("keyedCollection"); } kc = keyedCollection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { T[] items = new T[kc.Count]; kc.CopyTo(items, 0); return items; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** ** ** Purpose: DebugView class for generic collections ** ** =============================================================================*/ namespace System.Collections.Generic { using System; using System.Collections.ObjectModel; using System.Security.Permissions; using System.Diagnostics; // // VS IDE can't differentiate between types with the same name from different // assembly. So we need to use different names for collection debug view for // collections in mscorlib.dll and system.dll. // internal sealed class Mscorlib_CollectionDebugView { private ICollection collection; public Mscorlib_CollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { T[] items = new T[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_DictionaryKeyCollectionDebugView { private ICollection collection; public Mscorlib_DictionaryKeyCollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public TKey[] Items { get { TKey[] items = new TKey[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_DictionaryValueCollectionDebugView { private ICollection collection; public Mscorlib_DictionaryValueCollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public TValue[] Items { get { TValue[] items = new TValue[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_DictionaryDebugView { private IDictionary dict; public Mscorlib_DictionaryDebugView(IDictionary dictionary) { if (dictionary == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.dictionary); this.dict = dictionary; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public KeyValuePair [] Items { get { KeyValuePair [] items = new KeyValuePair [dict.Count]; dict.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_KeyedCollectionDebugView { private KeyedCollection kc; public Mscorlib_KeyedCollectionDebugView(KeyedCollection keyedCollection) { if (keyedCollection == null) { throw new ArgumentNullException("keyedCollection"); } kc = keyedCollection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { T[] items = new T[kc.Count]; kc.CopyTo(items, 0); return items; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
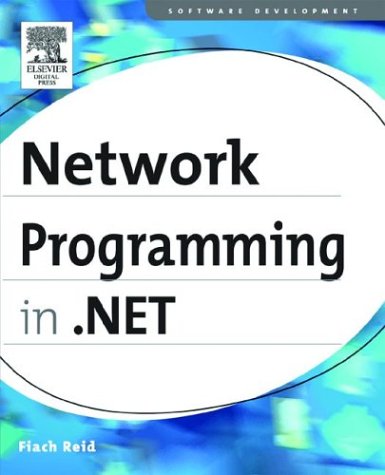
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlUnspecifiedAttribute.cs
- SQLDoubleStorage.cs
- UseManagedPresentationBindingElement.cs
- ExtendedProperty.cs
- ExpandSegmentCollection.cs
- FixedHyperLink.cs
- FullTrustAssembly.cs
- KnowledgeBase.cs
- Baml2006KnownTypes.cs
- BitmapEffectInputConnector.cs
- ReadOnlyDataSource.cs
- DetailsViewInsertEventArgs.cs
- EntityWrapper.cs
- ApplicationServicesHostFactory.cs
- ThreadStartException.cs
- TrackingServices.cs
- CollectionExtensions.cs
- ContextBase.cs
- FixedSchema.cs
- SpanIndex.cs
- SecurityCriticalDataForSet.cs
- WebPartVerbCollection.cs
- Monitor.cs
- UserControl.cs
- KeyValueConfigurationElement.cs
- XNodeValidator.cs
- TrustManager.cs
- TypedRowHandler.cs
- WebBrowser.cs
- DrawingVisualDrawingContext.cs
- HitTestDrawingContextWalker.cs
- ServiceHostingEnvironment.cs
- SqlWorkflowInstanceStoreLock.cs
- PagedDataSource.cs
- ParameterBuilder.cs
- HashFinalRequest.cs
- ArithmeticException.cs
- _BaseOverlappedAsyncResult.cs
- WebResourceAttribute.cs
- SinglePhaseEnlistment.cs
- FailedToStartupUIException.cs
- TextRunTypographyProperties.cs
- CornerRadiusConverter.cs
- UndoManager.cs
- SettingsBase.cs
- ObjectConverter.cs
- TaskFormBase.cs
- DetailsViewDeleteEventArgs.cs
- HttpServerVarsCollection.cs
- ResourcesBuildProvider.cs
- Privilege.cs
- Int32CAMarshaler.cs
- SecurityResources.cs
- UnaryNode.cs
- PropertyIDSet.cs
- XmlSchemaInfo.cs
- ChannelPool.cs
- CultureInfoConverter.cs
- ImageFormatConverter.cs
- ArgumentNullException.cs
- SortedList.cs
- AbsoluteQuery.cs
- DocumentPage.cs
- DesignerUtility.cs
- Zone.cs
- WinEventWrap.cs
- UnmanagedBitmapWrapper.cs
- UserPersonalizationStateInfo.cs
- ConnectionStringSettingsCollection.cs
- IDispatchConstantAttribute.cs
- InfoCardRequestException.cs
- EventLogEntry.cs
- IisTraceWebEventProvider.cs
- ListItemConverter.cs
- EntityContainerEntitySet.cs
- XmlElementElement.cs
- ArrayElementGridEntry.cs
- TextServicesCompartment.cs
- DataGridItemAttachedStorage.cs
- CacheChildrenQuery.cs
- DocumentReferenceCollection.cs
- RSAOAEPKeyExchangeFormatter.cs
- MoveSizeWinEventHandler.cs
- DataServiceQueryException.cs
- HtmlInputFile.cs
- httpapplicationstate.cs
- ReferenceEqualityComparer.cs
- TableLayoutSettings.cs
- DesignTimeTemplateParser.cs
- SqlClientFactory.cs
- DataControlPagerLinkButton.cs
- TemplateControlCodeDomTreeGenerator.cs
- ViewEvent.cs
- PrincipalPermission.cs
- SelectionEditingBehavior.cs
- Property.cs
- RelOps.cs
- InputScopeNameConverter.cs
- ContentType.cs
- CalendarItem.cs