Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Documents / DocumentReferenceCollection.cs / 1 / DocumentReferenceCollection.cs
//---------------------------------------------------------------------------- //// Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // // // Description: // Implements the DocumentReferenceCollection as holder for a collection // of DocumentReference // // History: // 05/07/2004 - [....] ([....]) - Created. // // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using System; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; //===================================================================== ////// DocumentReferenceCollection is an ordered collection of DocumentReference /// [CLSCompliant(false)] public sealed class DocumentReferenceCollection : IEnumerable, INotifyCollectionChanged { //------------------------------------------------------------------- // // Connstructors // //---------------------------------------------------------------------- #region Constructors internal DocumentReferenceCollection() { } #endregion Constructors //------------------------------------------------------------------- // // Public Methods // //---------------------------------------------------------------------- #region Public Methods #region IEnumerable /// /// /// public IEnumeratorGetEnumerator() { return _InternalList.GetEnumerator(); } System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } #endregion IEnumerable /// /// /// public void Add(DocumentReference item) { int count = _InternalList.Count; _InternalList.Add(item); OnCollectionChanged(NotifyCollectionChangedAction.Add, item, count); } ////// Passes in document reference array to be copied /// public void CopyTo(DocumentReference[] array, int arrayIndex) { _InternalList.CopyTo(array, arrayIndex); } #endregion Public Methods #region Public Properties ////// Count of Document References in collection /// public int Count { get { return _InternalList.Count; } } ////// /// public DocumentReference this[int index] { get { return _InternalList[index]; } } #endregion Public Properties //-------------------------------------------------------------------- // // Public Events // //--------------------------------------------------------------------- #region Public Event ////// Occurs when the collection changes, either by adding or removing an item. /// ////// see public event NotifyCollectionChangedEventHandler CollectionChanged; #endregion Public Event //-------------------------------------------------------------------- // // private Properties // //--------------------------------------------------------------------- #region Private Properties // Aggregated IList private IList/// _InternalList { get { if (_internalList == null) { _internalList = new List (); } return _internalList; } } #endregion Private Properties #region Private Methods //------------------------------------------------------------------- // // Private Methods // //--------------------------------------------------------------------- // fire CollectionChanged event to any listeners private void OnCollectionChanged(NotifyCollectionChangedAction action, object item, int index) { if (CollectionChanged != null) { NotifyCollectionChangedEventArgs args; args = new NotifyCollectionChangedEventArgs(action, item, index); CollectionChanged(this, args); } } #endregion Private Methods //-------------------------------------------------------------------- // // Private Fields // //--------------------------------------------------------------------- #region Private Fields private List _internalList; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
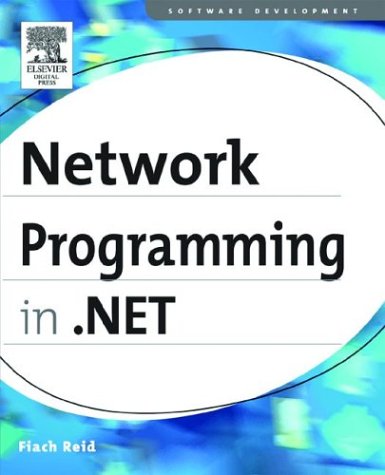
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MetadataItem.cs
- PointCollection.cs
- ParameterCollection.cs
- TextControl.cs
- PriorityQueue.cs
- FrugalMap.cs
- ChildTable.cs
- DataGridViewControlCollection.cs
- UnauthorizedWebPart.cs
- PublishLicense.cs
- AttributeEmitter.cs
- DesignerSerializerAttribute.cs
- WindowsAuthenticationModule.cs
- Schema.cs
- IntSecurity.cs
- FormViewInsertEventArgs.cs
- SiteMembershipCondition.cs
- _SingleItemRequestCache.cs
- DescendentsWalkerBase.cs
- GZipDecoder.cs
- Completion.cs
- MethodRental.cs
- DataTableClearEvent.cs
- CombinedGeometry.cs
- DeferredTextReference.cs
- Point3DIndependentAnimationStorage.cs
- VideoDrawing.cs
- SoapRpcServiceAttribute.cs
- ObjectItemConventionAssemblyLoader.cs
- XPathNode.cs
- ControlHelper.cs
- NotCondition.cs
- WebPartDeleteVerb.cs
- HttpMethodConstraint.cs
- PropVariant.cs
- XPathSingletonIterator.cs
- CallTemplateAction.cs
- TrailingSpaceComparer.cs
- CaseInsensitiveComparer.cs
- XmlSerializerAssemblyAttribute.cs
- AnonymousIdentificationSection.cs
- ScaleTransform.cs
- ShaderRenderModeValidation.cs
- DataListCommandEventArgs.cs
- ToolBarTray.cs
- SmiMetaDataProperty.cs
- SizeChangedEventArgs.cs
- ContentElement.cs
- SqlBinder.cs
- FullTextBreakpoint.cs
- OleStrCAMarshaler.cs
- CustomDictionarySources.cs
- UnsafeNativeMethodsMilCoreApi.cs
- DocumentViewer.cs
- CryptographicAttribute.cs
- FrameDimension.cs
- SourceFilter.cs
- TextTreeTextNode.cs
- ParsedAttributeCollection.cs
- MessageSecurityOverTcp.cs
- Size3DConverter.cs
- OperatorExpressions.cs
- base64Transforms.cs
- RepeatButtonAutomationPeer.cs
- Directory.cs
- AuthenticatingEventArgs.cs
- CountdownEvent.cs
- MimeReflector.cs
- ConstructorBuilder.cs
- _ProxyChain.cs
- ConfigPathUtility.cs
- UpdatePanel.cs
- BookmarkUndoUnit.cs
- ISCIIEncoding.cs
- Base64Stream.cs
- TextPattern.cs
- AuthorizationRuleCollection.cs
- SqlClientWrapperSmiStream.cs
- SrgsDocument.cs
- DesignerSerializationOptionsAttribute.cs
- EventRoute.cs
- ManagementObjectCollection.cs
- RadialGradientBrush.cs
- WebPartTransformerAttribute.cs
- ContentValidator.cs
- ResXFileRef.cs
- EntryWrittenEventArgs.cs
- HotSpotCollectionEditor.cs
- Attributes.cs
- _Connection.cs
- OletxTransactionFormatter.cs
- MoveSizeWinEventHandler.cs
- ScopelessEnumAttribute.cs
- RoutedEvent.cs
- XsltFunctions.cs
- elementinformation.cs
- ExpressionEditor.cs
- BaseTreeIterator.cs
- WindowsGraphicsWrapper.cs
- WebHostUnsafeNativeMethods.cs