Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / XmlUtils / System / Xml / Xsl / XsltOld / CallTemplateAction.cs / 1 / CallTemplateAction.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; internal class CallTemplateAction : ContainerAction { private const int ProcessedChildren = 2; private const int ProcessedTemplate = 3; private XmlQualifiedName name; internal override void Compile(Compiler compiler) { CompileAttributes(compiler); CheckRequiredAttribute(compiler, this.name, Keywords.s_Name); CompileContent(compiler); } internal override bool CompileAttribute(Compiler compiler) { string name = compiler.Input.LocalName; string value = compiler.Input.Value; if (Keywords.Equals(name, compiler.Atoms.Name)) { Debug.Assert(this.name == null); this.name = compiler.CreateXPathQName(value); } else { return false; } return true; } private void CompileContent(Compiler compiler) { NavigatorInput input = compiler.Input; if (compiler.Recurse()) { do { switch(input.NodeType) { case XPathNodeType.Element: compiler.PushNamespaceScope(); string nspace = input.NamespaceURI; string name = input.LocalName; if (Keywords.Equals(nspace, input.Atoms.XsltNamespace) && Keywords.Equals(name, input.Atoms.WithParam)) { WithParamAction par = compiler.CreateWithParamAction(); CheckDuplicateParams(par.Name); AddAction(par); } else { throw compiler.UnexpectedKeyword(); } compiler.PopScope(); break; case XPathNodeType.Comment: case XPathNodeType.ProcessingInstruction: case XPathNodeType.Whitespace: case XPathNodeType.SignificantWhitespace: break; default: throw XsltException.Create(Res.Xslt_InvalidContents, Keywords.s_CallTemplate); } } while(compiler.Advance()); compiler.ToParent(); } } internal override void Execute(Processor processor, ActionFrame frame) { Debug.Assert(processor != null && frame != null); switch(frame.State) { case Initialized : processor.ResetParams(); if (this.containedActions != null && this.containedActions.Count > 0) { processor.PushActionFrame(frame); frame.State = ProcessedChildren; break; } goto case ProcessedChildren; case ProcessedChildren: TemplateAction action = processor.Stylesheet.FindTemplate(this.name); if (action != null) { frame.State = ProcessedTemplate; processor.PushActionFrame(action, frame.NodeSet); break; } else { throw XsltException.Create(Res.Xslt_InvalidCallTemplate, this.name.ToString()); } case ProcessedTemplate: frame.Finished(); break; default: Debug.Fail("Invalid CallTemplateAction execution state"); break; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; internal class CallTemplateAction : ContainerAction { private const int ProcessedChildren = 2; private const int ProcessedTemplate = 3; private XmlQualifiedName name; internal override void Compile(Compiler compiler) { CompileAttributes(compiler); CheckRequiredAttribute(compiler, this.name, Keywords.s_Name); CompileContent(compiler); } internal override bool CompileAttribute(Compiler compiler) { string name = compiler.Input.LocalName; string value = compiler.Input.Value; if (Keywords.Equals(name, compiler.Atoms.Name)) { Debug.Assert(this.name == null); this.name = compiler.CreateXPathQName(value); } else { return false; } return true; } private void CompileContent(Compiler compiler) { NavigatorInput input = compiler.Input; if (compiler.Recurse()) { do { switch(input.NodeType) { case XPathNodeType.Element: compiler.PushNamespaceScope(); string nspace = input.NamespaceURI; string name = input.LocalName; if (Keywords.Equals(nspace, input.Atoms.XsltNamespace) && Keywords.Equals(name, input.Atoms.WithParam)) { WithParamAction par = compiler.CreateWithParamAction(); CheckDuplicateParams(par.Name); AddAction(par); } else { throw compiler.UnexpectedKeyword(); } compiler.PopScope(); break; case XPathNodeType.Comment: case XPathNodeType.ProcessingInstruction: case XPathNodeType.Whitespace: case XPathNodeType.SignificantWhitespace: break; default: throw XsltException.Create(Res.Xslt_InvalidContents, Keywords.s_CallTemplate); } } while(compiler.Advance()); compiler.ToParent(); } } internal override void Execute(Processor processor, ActionFrame frame) { Debug.Assert(processor != null && frame != null); switch(frame.State) { case Initialized : processor.ResetParams(); if (this.containedActions != null && this.containedActions.Count > 0) { processor.PushActionFrame(frame); frame.State = ProcessedChildren; break; } goto case ProcessedChildren; case ProcessedChildren: TemplateAction action = processor.Stylesheet.FindTemplate(this.name); if (action != null) { frame.State = ProcessedTemplate; processor.PushActionFrame(action, frame.NodeSet); break; } else { throw XsltException.Create(Res.Xslt_InvalidCallTemplate, this.name.ToString()); } case ProcessedTemplate: frame.Finished(); break; default: Debug.Fail("Invalid CallTemplateAction execution state"); break; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
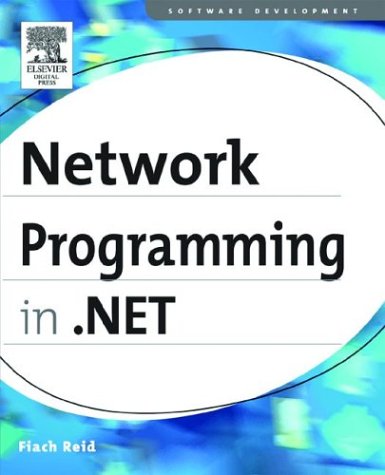
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FreezableDefaultValueFactory.cs
- _DynamicWinsockMethods.cs
- RelatedView.cs
- SoapHelper.cs
- Int32RectConverter.cs
- EnumMember.cs
- DataGridColumnHeadersPresenter.cs
- BrowserCapabilitiesCompiler.cs
- DeclaredTypeValidatorAttribute.cs
- ObjectManager.cs
- DetailsViewUpdatedEventArgs.cs
- SecurityPolicySection.cs
- PrtCap_Reader.cs
- WmlObjectListAdapter.cs
- TrustManager.cs
- DbConnectionFactory.cs
- CursorConverter.cs
- Perspective.cs
- SmtpLoginAuthenticationModule.cs
- RC2CryptoServiceProvider.cs
- CodeAccessPermission.cs
- WindowsNonControl.cs
- BitSet.cs
- EntityDataSourceQueryBuilder.cs
- BamlCollectionHolder.cs
- QuaternionAnimation.cs
- CultureData.cs
- InvokeMethod.cs
- BinaryHeap.cs
- FontEmbeddingManager.cs
- KeyValueInternalCollection.cs
- SmiEventStream.cs
- LogicalChannelCollection.cs
- FacetChecker.cs
- OperatingSystemVersionCheck.cs
- _OverlappedAsyncResult.cs
- BaseProcessor.cs
- CacheDependency.cs
- DataGridViewComboBoxColumnDesigner.cs
- FontDialog.cs
- ControlParser.cs
- LayoutTableCell.cs
- ActivationServices.cs
- PtsCache.cs
- CustomLineCap.cs
- CustomTypeDescriptor.cs
- DigitalSignature.cs
- FigureParaClient.cs
- BlockCollection.cs
- GestureRecognitionResult.cs
- XPathNodeIterator.cs
- EditingScope.cs
- Error.cs
- DataGridViewColumnDesignTimeVisibleAttribute.cs
- ToolStripPanelRenderEventArgs.cs
- Parser.cs
- GcHandle.cs
- CodeFieldReferenceExpression.cs
- EntityWithChangeTrackerStrategy.cs
- HtmlGenericControl.cs
- TraceSource.cs
- PropertyMetadata.cs
- DependencyObjectCodeDomSerializer.cs
- DateTimeConstantAttribute.cs
- SelectionRangeConverter.cs
- IsolationInterop.cs
- WebPartEditorApplyVerb.cs
- PeerPresenceInfo.cs
- MarshalByRefObject.cs
- SqlRowUpdatingEvent.cs
- ListViewCommandEventArgs.cs
- LabelEditEvent.cs
- GridEntryCollection.cs
- ServerType.cs
- ProxyWebPart.cs
- WhileDesigner.cs
- ArrayWithOffset.cs
- Soap.cs
- SerializationException.cs
- FontWeight.cs
- Component.cs
- SiteMapNodeItem.cs
- Timeline.cs
- ListBoxChrome.cs
- WindowInteropHelper.cs
- XmlUtil.cs
- FreezableDefaultValueFactory.cs
- OrderingQueryOperator.cs
- ScrollEvent.cs
- DataGridToolTip.cs
- PeerDefaultCustomResolverClient.cs
- ExpanderAutomationPeer.cs
- XmlText.cs
- XmlSerializableReader.cs
- NetTcpBindingElement.cs
- PageAdapter.cs
- ThaiBuddhistCalendar.cs
- TextEditorCharacters.cs
- safesecurityhelperavalon.cs
- DirectoryInfo.cs