Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Sys / System / Configuration / SettingsBase.cs / 1305376 / SettingsBase.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Collections.Specialized; using System.Runtime.Serialization; using System.Configuration.Provider; using System.Collections; using System.ComponentModel; //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public abstract class SettingsBase { protected SettingsBase() { _PropertyValues = new SettingsPropertyValueCollection(); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public virtual object this[string propertyName] { get { if (IsSynchronized) { lock (this) { return GetPropertyValueByName(propertyName); } } else { return GetPropertyValueByName(propertyName); } } set { if (IsSynchronized) { lock (this) { SetPropertyValueByName(propertyName, value); } } else { SetPropertyValueByName(propertyName, value); } } } private object GetPropertyValueByName(string propertyName) { if (Properties == null || _PropertyValues == null || Properties.Count == 0) throw new SettingsPropertyNotFoundException(SR.GetString(SR.SettingsPropertyNotFound, propertyName)); SettingsProperty pp = Properties[propertyName]; if (pp == null) throw new SettingsPropertyNotFoundException(SR.GetString(SR.SettingsPropertyNotFound, propertyName)); SettingsPropertyValue p = _PropertyValues[propertyName]; if (p == null) { GetPropertiesFromProvider(pp.Provider); p = _PropertyValues[propertyName]; if (p == null) throw new SettingsPropertyNotFoundException(SR.GetString(SR.SettingsPropertyNotFound, propertyName)); } return p.PropertyValue; } private void SetPropertyValueByName(string propertyName, object propertyValue) { if (Properties == null || _PropertyValues == null || Properties.Count == 0) throw new SettingsPropertyNotFoundException(SR.GetString(SR.SettingsPropertyNotFound, propertyName)); SettingsProperty pp = Properties[propertyName]; if (pp == null) throw new SettingsPropertyNotFoundException(SR.GetString(SR.SettingsPropertyNotFound, propertyName)); if (pp.IsReadOnly) throw new SettingsPropertyIsReadOnlyException(SR.GetString(SR.SettingsPropertyReadOnly, propertyName)); if (propertyValue != null && !pp.PropertyType.IsInstanceOfType(propertyValue)) throw new SettingsPropertyWrongTypeException(SR.GetString(SR.SettingsPropertyWrongType, propertyName)); SettingsPropertyValue p = _PropertyValues[propertyName]; if (p == null) { GetPropertiesFromProvider(pp.Provider); p = _PropertyValues[propertyName]; if (p == null) throw new SettingsPropertyNotFoundException(SR.GetString(SR.SettingsPropertyNotFound, propertyName)); } p.PropertyValue = propertyValue; } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void Initialize( SettingsContext context, SettingsPropertyCollection properties, SettingsProviderCollection providers) { _Context = context; _Properties = properties; _Providers = providers; } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public virtual void Save() { if (IsSynchronized) { lock (this) { SaveCore(); } } else { SaveCore(); } } private void SaveCore() { if (Properties == null || _PropertyValues == null || Properties.Count == 0) return; foreach(SettingsProvider prov in Providers) { SettingsPropertyValueCollection ppcv = new SettingsPropertyValueCollection(); foreach (SettingsPropertyValue pp in PropertyValues) { if (pp.Property.Provider == prov) { ppcv.Add(pp); } } if (ppcv.Count > 0) { prov.SetPropertyValues(Context, ppcv); } } foreach (SettingsPropertyValue pp in PropertyValues) pp.IsDirty = false; } virtual public SettingsPropertyCollection Properties { get { return _Properties; }} virtual public SettingsProviderCollection Providers { get { return _Providers; }} virtual public SettingsPropertyValueCollection PropertyValues { get { return _PropertyValues; } } virtual public SettingsContext Context { get { return _Context; } } private void GetPropertiesFromProvider(SettingsProvider provider) { SettingsPropertyCollection ppc = new SettingsPropertyCollection(); foreach (SettingsProperty pp in Properties) { if (pp.Provider == provider) { ppc.Add(pp); } } if (ppc.Count > 0) { SettingsPropertyValueCollection ppcv = provider.GetPropertyValues(Context, ppc); foreach (SettingsPropertyValue p in ppcv) { if (_PropertyValues[p.Name] == null) _PropertyValues.Add(p); } } } public static SettingsBase Synchronized(SettingsBase settingsBase) { settingsBase._IsSynchronized = true; return settingsBase; } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// private SettingsPropertyCollection _Properties = null; private SettingsProviderCollection _Providers = null; private SettingsPropertyValueCollection _PropertyValues = null; private SettingsContext _Context = null; private bool _IsSynchronized = false; [Browsable(false)] public bool IsSynchronized { get { return _IsSynchronized; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Collections.Specialized; using System.Runtime.Serialization; using System.Configuration.Provider; using System.Collections; using System.ComponentModel; //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public abstract class SettingsBase { protected SettingsBase() { _PropertyValues = new SettingsPropertyValueCollection(); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public virtual object this[string propertyName] { get { if (IsSynchronized) { lock (this) { return GetPropertyValueByName(propertyName); } } else { return GetPropertyValueByName(propertyName); } } set { if (IsSynchronized) { lock (this) { SetPropertyValueByName(propertyName, value); } } else { SetPropertyValueByName(propertyName, value); } } } private object GetPropertyValueByName(string propertyName) { if (Properties == null || _PropertyValues == null || Properties.Count == 0) throw new SettingsPropertyNotFoundException(SR.GetString(SR.SettingsPropertyNotFound, propertyName)); SettingsProperty pp = Properties[propertyName]; if (pp == null) throw new SettingsPropertyNotFoundException(SR.GetString(SR.SettingsPropertyNotFound, propertyName)); SettingsPropertyValue p = _PropertyValues[propertyName]; if (p == null) { GetPropertiesFromProvider(pp.Provider); p = _PropertyValues[propertyName]; if (p == null) throw new SettingsPropertyNotFoundException(SR.GetString(SR.SettingsPropertyNotFound, propertyName)); } return p.PropertyValue; } private void SetPropertyValueByName(string propertyName, object propertyValue) { if (Properties == null || _PropertyValues == null || Properties.Count == 0) throw new SettingsPropertyNotFoundException(SR.GetString(SR.SettingsPropertyNotFound, propertyName)); SettingsProperty pp = Properties[propertyName]; if (pp == null) throw new SettingsPropertyNotFoundException(SR.GetString(SR.SettingsPropertyNotFound, propertyName)); if (pp.IsReadOnly) throw new SettingsPropertyIsReadOnlyException(SR.GetString(SR.SettingsPropertyReadOnly, propertyName)); if (propertyValue != null && !pp.PropertyType.IsInstanceOfType(propertyValue)) throw new SettingsPropertyWrongTypeException(SR.GetString(SR.SettingsPropertyWrongType, propertyName)); SettingsPropertyValue p = _PropertyValues[propertyName]; if (p == null) { GetPropertiesFromProvider(pp.Provider); p = _PropertyValues[propertyName]; if (p == null) throw new SettingsPropertyNotFoundException(SR.GetString(SR.SettingsPropertyNotFound, propertyName)); } p.PropertyValue = propertyValue; } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void Initialize( SettingsContext context, SettingsPropertyCollection properties, SettingsProviderCollection providers) { _Context = context; _Properties = properties; _Providers = providers; } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public virtual void Save() { if (IsSynchronized) { lock (this) { SaveCore(); } } else { SaveCore(); } } private void SaveCore() { if (Properties == null || _PropertyValues == null || Properties.Count == 0) return; foreach(SettingsProvider prov in Providers) { SettingsPropertyValueCollection ppcv = new SettingsPropertyValueCollection(); foreach (SettingsPropertyValue pp in PropertyValues) { if (pp.Property.Provider == prov) { ppcv.Add(pp); } } if (ppcv.Count > 0) { prov.SetPropertyValues(Context, ppcv); } } foreach (SettingsPropertyValue pp in PropertyValues) pp.IsDirty = false; } virtual public SettingsPropertyCollection Properties { get { return _Properties; }} virtual public SettingsProviderCollection Providers { get { return _Providers; }} virtual public SettingsPropertyValueCollection PropertyValues { get { return _PropertyValues; } } virtual public SettingsContext Context { get { return _Context; } } private void GetPropertiesFromProvider(SettingsProvider provider) { SettingsPropertyCollection ppc = new SettingsPropertyCollection(); foreach (SettingsProperty pp in Properties) { if (pp.Provider == provider) { ppc.Add(pp); } } if (ppc.Count > 0) { SettingsPropertyValueCollection ppcv = provider.GetPropertyValues(Context, ppc); foreach (SettingsPropertyValue p in ppcv) { if (_PropertyValues[p.Name] == null) _PropertyValues.Add(p); } } } public static SettingsBase Synchronized(SettingsBase settingsBase) { settingsBase._IsSynchronized = true; return settingsBase; } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// private SettingsPropertyCollection _Properties = null; private SettingsProviderCollection _Providers = null; private SettingsPropertyValueCollection _PropertyValues = null; private SettingsContext _Context = null; private bool _IsSynchronized = false; [Browsable(false)] public bool IsSynchronized { get { return _IsSynchronized; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
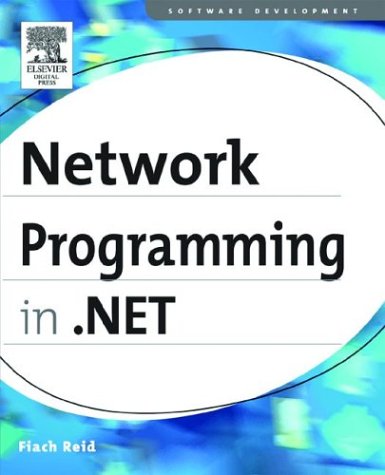
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartCatalogAddVerb.cs
- ReflectionPermission.cs
- Decimal.cs
- KeyboardNavigation.cs
- ResourceType.cs
- MsmqTransportSecurityElement.cs
- SecurityRuntime.cs
- OutputCacheModule.cs
- Message.cs
- Polyline.cs
- ScaleTransform3D.cs
- PeerOutputChannel.cs
- LabelDesigner.cs
- PageEventArgs.cs
- EffectiveValueEntry.cs
- StreamSecurityUpgradeAcceptorAsyncResult.cs
- InternalsVisibleToAttribute.cs
- KeyToListMap.cs
- KeyConstraint.cs
- StylusPointPropertyUnit.cs
- MarkupExtensionReturnTypeAttribute.cs
- _DomainName.cs
- KnownTypesHelper.cs
- FixUpCollection.cs
- PixelFormat.cs
- TileBrush.cs
- HttpsHostedTransportConfiguration.cs
- WindowsSecurityToken.cs
- DesignerCapabilities.cs
- WeakEventTable.cs
- AutomationPatternInfo.cs
- GroupJoinQueryOperator.cs
- FrugalMap.cs
- FragmentNavigationEventArgs.cs
- SvcMapFile.cs
- oledbmetadatacollectionnames.cs
- DataTableTypeConverter.cs
- AutomationPatternInfo.cs
- FrameworkContextData.cs
- EditorZoneBase.cs
- _FixedSizeReader.cs
- ConnectionConsumerAttribute.cs
- PersonalizationProviderCollection.cs
- BooleanToVisibilityConverter.cs
- TextDpi.cs
- AuthenticatingEventArgs.cs
- ToolStripSplitButton.cs
- OutOfMemoryException.cs
- ISCIIEncoding.cs
- ContentIterators.cs
- ObjectAnimationBase.cs
- Overlapped.cs
- ResourceExpression.cs
- PageCodeDomTreeGenerator.cs
- FactoryRecord.cs
- FontUnit.cs
- DynamicPropertyHolder.cs
- _RequestCacheProtocol.cs
- OdbcConnectionStringbuilder.cs
- PenThread.cs
- Error.cs
- VariantWrapper.cs
- CodeTypeReference.cs
- QilScopedVisitor.cs
- _SecureChannel.cs
- HttpWriter.cs
- EntityTypeBase.cs
- CopyAction.cs
- StrokeCollectionDefaultValueFactory.cs
- CqlGenerator.cs
- HasCopySemanticsAttribute.cs
- TypefaceMetricsCache.cs
- TranslateTransform3D.cs
- ping.cs
- TextClipboardData.cs
- CustomError.cs
- NullableBoolConverter.cs
- TypeNameParser.cs
- WorkItem.cs
- PackageDigitalSignature.cs
- SafeWaitHandle.cs
- HttpBindingExtension.cs
- NonSerializedAttribute.cs
- FontDialog.cs
- LoadItemsEventArgs.cs
- InputMethodStateTypeInfo.cs
- XamlPoint3DCollectionSerializer.cs
- DynamicValidatorEventArgs.cs
- TextBoxAutoCompleteSourceConverter.cs
- BuildProviderCollection.cs
- SQLStringStorage.cs
- MatrixAnimationBase.cs
- UnsafeNativeMethods.cs
- NotCondition.cs
- UrlAuthFailureHandler.cs
- Effect.cs
- DashStyle.cs
- SingleBodyParameterMessageFormatter.cs
- CaretElement.cs
- CatalogPart.cs