Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / DataServiceQueryException.cs / 1 / DataServiceQueryException.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Exception class for query requests. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; ////// The exception that is thrown when an error occurs while processing a batch request or /// during SaveChanges. /// #if !ASTORIA_LIGHT [Serializable] #endif [System.Diagnostics.DebuggerDisplay("{Message}")] public sealed class DataServiceQueryException : InvalidOperationException { #region Private fields. ///Actual response object. #if !ASTORIA_LIGHT [NonSerialized] #endif private readonly QueryOperationResponse response; #endregion Private fields. #region Constructors. ////// Creates a new instance of DataServiceQueryException. /// public DataServiceQueryException() : base(Strings.DataServiceException_GeneralError) { } ////// Creates a new instance of DataServiceQueryException. /// /// error message for this exception. public DataServiceQueryException(string message) : base(message) { } ////// Creates a new instance of DataServiceQueryException. /// /// error message for this exception. /// Exception that caused this exception to be thrown. public DataServiceQueryException(string message, Exception innerException) : base(message, innerException) { } ////// Creates a new instance of DataServiceQueryException. /// /// error message for this exception. /// Exception that caused this exception to be thrown. /// response object for this exception. public DataServiceQueryException(string message, Exception innerException, QueryOperationResponse response) : base(message, innerException) { this.response = response; } #if !ASTORIA_LIGHT #pragma warning disable 0628 ////// Initializes a new instance of the DataServiceQueryException class from the /// specified SerializationInfo and StreamingContext instances. /// /// /// A SerializationInfo containing the information required to serialize /// the new DataServiceQueryException. /// /// A StreamingContext containing the source of the serialized stream /// associated with the new DataServiceQueryException. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1047", Justification = "Follows serialization info pattern.")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1032", Justification = "Follows serialization info pattern.")] protected DataServiceQueryException(System.Runtime.Serialization.SerializationInfo info, System.Runtime.Serialization.StreamingContext context) : base(info, context) { } #pragma warning restore 0628 #endif #endregion Constructors. #region Public properties. ///Error code to be used in payloads. public QueryOperationResponse Response { get { return this.response; } } #endregion Public properties. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Exception class for query requests. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; ////// The exception that is thrown when an error occurs while processing a batch request or /// during SaveChanges. /// #if !ASTORIA_LIGHT [Serializable] #endif [System.Diagnostics.DebuggerDisplay("{Message}")] public sealed class DataServiceQueryException : InvalidOperationException { #region Private fields. ///Actual response object. #if !ASTORIA_LIGHT [NonSerialized] #endif private readonly QueryOperationResponse response; #endregion Private fields. #region Constructors. ////// Creates a new instance of DataServiceQueryException. /// public DataServiceQueryException() : base(Strings.DataServiceException_GeneralError) { } ////// Creates a new instance of DataServiceQueryException. /// /// error message for this exception. public DataServiceQueryException(string message) : base(message) { } ////// Creates a new instance of DataServiceQueryException. /// /// error message for this exception. /// Exception that caused this exception to be thrown. public DataServiceQueryException(string message, Exception innerException) : base(message, innerException) { } ////// Creates a new instance of DataServiceQueryException. /// /// error message for this exception. /// Exception that caused this exception to be thrown. /// response object for this exception. public DataServiceQueryException(string message, Exception innerException, QueryOperationResponse response) : base(message, innerException) { this.response = response; } #if !ASTORIA_LIGHT #pragma warning disable 0628 ////// Initializes a new instance of the DataServiceQueryException class from the /// specified SerializationInfo and StreamingContext instances. /// /// /// A SerializationInfo containing the information required to serialize /// the new DataServiceQueryException. /// /// A StreamingContext containing the source of the serialized stream /// associated with the new DataServiceQueryException. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1047", Justification = "Follows serialization info pattern.")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1032", Justification = "Follows serialization info pattern.")] protected DataServiceQueryException(System.Runtime.Serialization.SerializationInfo info, System.Runtime.Serialization.StreamingContext context) : base(info, context) { } #pragma warning restore 0628 #endif #endregion Constructors. #region Public properties. ///Error code to be used in payloads. public QueryOperationResponse Response { get { return this.response; } } #endregion Public properties. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
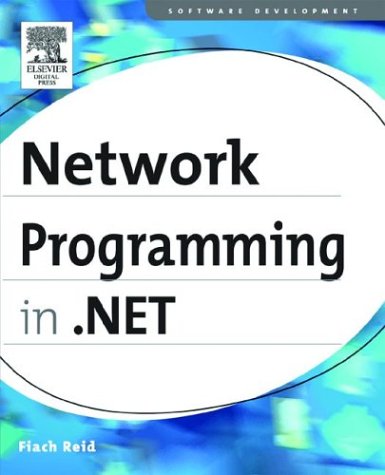
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ResourceContainer.cs
- TextEndOfSegment.cs
- QilTernary.cs
- DesignerRegionCollection.cs
- XslUrlEditor.cs
- TraceFilter.cs
- ThemeInfoAttribute.cs
- DateTimeStorage.cs
- KeyPressEvent.cs
- ClientScriptManagerWrapper.cs
- ScrollChangedEventArgs.cs
- DefaultTraceListener.cs
- AttachedPropertyMethodSelector.cs
- TdsParser.cs
- PermissionSet.cs
- InputLanguageManager.cs
- CannotUnloadAppDomainException.cs
- AtomServiceDocumentSerializer.cs
- Attachment.cs
- ManagementObject.cs
- DataTable.cs
- XmlChildNodes.cs
- QilScopedVisitor.cs
- ProviderConnectionPointCollection.cs
- DockingAttribute.cs
- RoleManagerSection.cs
- ControlCachePolicy.cs
- HtmlImage.cs
- SRDisplayNameAttribute.cs
- Line.cs
- DataServiceResponse.cs
- TableSectionStyle.cs
- ScriptHandlerFactory.cs
- ModuleConfigurationInfo.cs
- QuaternionAnimationBase.cs
- DataGridViewRowPostPaintEventArgs.cs
- SemaphoreSlim.cs
- DocumentsTrace.cs
- Size3DValueSerializer.cs
- TcpTransportBindingElement.cs
- BinaryConverter.cs
- QilVisitor.cs
- Attributes.cs
- BufferedReadStream.cs
- DataChangedEventManager.cs
- compensatingcollection.cs
- EventLogPermission.cs
- MaskInputRejectedEventArgs.cs
- RootBrowserWindow.cs
- SecurityManager.cs
- ProfileSection.cs
- SchemaType.cs
- DockAndAnchorLayout.cs
- LayoutSettings.cs
- SQLSingle.cs
- ErrorRuntimeConfig.cs
- PropertyMappingExceptionEventArgs.cs
- ListViewTableCell.cs
- ExtensibleClassFactory.cs
- HttpListenerContext.cs
- DeviceContexts.cs
- DelegateArgumentReference.cs
- KeyFrames.cs
- XmlTypeAttribute.cs
- EntityDataSourceReferenceGroup.cs
- ExceptionAggregator.cs
- UpdateCompiler.cs
- AssemblyResourceLoader.cs
- WhitespaceRule.cs
- ItemsPresenter.cs
- EntityContainerEmitter.cs
- TextLine.cs
- ErrorReporting.cs
- AssemblyCache.cs
- SqlTypeConverter.cs
- WebBrowserNavigatingEventHandler.cs
- BaseParagraph.cs
- RedirectionProxy.cs
- WindowsRebar.cs
- StorageEntitySetMapping.cs
- DuplicateWaitObjectException.cs
- EventSinkHelperWriter.cs
- TTSEngineTypes.cs
- CategoryGridEntry.cs
- StreamGeometry.cs
- LogLogRecordHeader.cs
- UriParserTemplates.cs
- StreamSecurityUpgradeProvider.cs
- ToolStripItemClickedEventArgs.cs
- WebBrowserEvent.cs
- WasEndpointConfigContainer.cs
- BuildProvider.cs
- MimeBasePart.cs
- HttpResponseInternalWrapper.cs
- Deflater.cs
- EditingScopeUndoUnit.cs
- ViewRendering.cs
- DATA_BLOB.cs
- DataGridViewCellStyle.cs
- ResourceManagerWrapper.cs