Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Security / Tokens / BinarySecretSecurityToken.cs / 1 / BinarySecretSecurityToken.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Security.Tokens { using System.Collections; using System.ServiceModel; using System.Collections.Generic; using System.Collections.ObjectModel; using System.IO; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.IdentityModel.Tokens; using System.Security.Cryptography; using System.Text; using System.Xml; public class BinarySecretSecurityToken : SecurityToken { string id; DateTime effectiveTime; byte[] key; ReadOnlyCollectionsecurityKeys; public BinarySecretSecurityToken(int keySizeInBits) : this(SecurityUniqueId.Create().Value, keySizeInBits) { } public BinarySecretSecurityToken(string id, int keySizeInBits) : this(id, keySizeInBits, true) { } public BinarySecretSecurityToken(byte[] key) : this(SecurityUniqueId.Create().Value, key) { } public BinarySecretSecurityToken(string id, byte[] key) : this(id, key, true) { } protected BinarySecretSecurityToken(string id, int keySizeInBits, bool allowCrypto) { if (keySizeInBits <= 0 || keySizeInBits >= 512) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("keySizeInBits", SR.GetString(SR.ValueMustBeInRange, 0, 512))); } if ((keySizeInBits % 8) != 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("keySizeInBits", SR.GetString(SR.KeyLengthMustBeMultipleOfEight, keySizeInBits))); } this.id = id; this.effectiveTime = DateTime.UtcNow; this.key = new byte[keySizeInBits / 8]; CryptoHelper.FillRandomBytes(this.key); if (allowCrypto) { this.securityKeys = SecurityUtils.CreateSymmetricSecurityKeys(this.key); } else { this.securityKeys = EmptyReadOnlyCollection .Instance; } } protected BinarySecretSecurityToken(string id, byte[] key, bool allowCrypto) { if (key == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("key"); this.id = id; this.effectiveTime = DateTime.UtcNow; this.key = new byte[key.Length]; Buffer.BlockCopy(key, 0, this.key, 0, key.Length); if (allowCrypto) { this.securityKeys = SecurityUtils.CreateSymmetricSecurityKeys(this.key); } else { this.securityKeys = EmptyReadOnlyCollection .Instance; } } public override string Id { get { return this.id; } } public override DateTime ValidFrom { get { return this.effectiveTime; } } public override DateTime ValidTo { // Never expire get { return DateTime.MaxValue; } } public int KeySize { get { return (this.key.Length * 8); } } public override ReadOnlyCollection SecurityKeys { get { return this.securityKeys; } } public byte[] GetKeyBytes() { return SecurityUtils.CloneBuffer(this.key); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
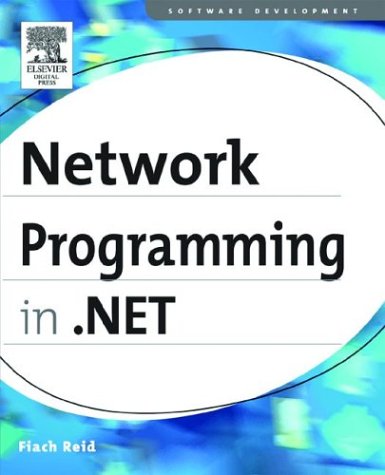
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WeakReferenceKey.cs
- SqlVisitor.cs
- TraceContext.cs
- CachedCompositeFamily.cs
- ProcessHost.cs
- ClientBuildManagerCallback.cs
- TemplatePartAttribute.cs
- JapaneseLunisolarCalendar.cs
- XamlRtfConverter.cs
- CommandLibraryHelper.cs
- WebPartDisplayModeCollection.cs
- NoPersistHandle.cs
- EntityDataSourceDataSelection.cs
- FieldAccessException.cs
- OdbcErrorCollection.cs
- InboundActivityHelper.cs
- DefaultAssemblyResolver.cs
- GridErrorDlg.cs
- ServiceDurableInstance.cs
- DispatcherTimer.cs
- ParseHttpDate.cs
- EntityDesignerUtils.cs
- parserscommon.cs
- ObjectReaderCompiler.cs
- AnnotationHighlightLayer.cs
- HScrollBar.cs
- UnknownBitmapEncoder.cs
- ModelTypeConverter.cs
- safemediahandle.cs
- RIPEMD160.cs
- NativeMethodsOther.cs
- CommandDevice.cs
- TreeNodeCollection.cs
- MouseButtonEventArgs.cs
- KeyFrames.cs
- BindStream.cs
- PeerNameRegistration.cs
- CodeArrayIndexerExpression.cs
- NegationPusher.cs
- _ProxyChain.cs
- SpellCheck.cs
- Wildcard.cs
- MemberDescriptor.cs
- BooleanStorage.cs
- CodeGenerationManager.cs
- SafeEventHandle.cs
- DataConnectionHelper.cs
- EntityDataSourceChangedEventArgs.cs
- Win32.cs
- TraversalRequest.cs
- SqlReorderer.cs
- XmlReflectionImporter.cs
- ManifestResourceInfo.cs
- UInt32.cs
- SharedConnectionListener.cs
- sqlstateclientmanager.cs
- InputProcessorProfiles.cs
- XmlDictionaryReader.cs
- TrackingStringDictionary.cs
- DataGridViewColumnStateChangedEventArgs.cs
- ControlValuePropertyAttribute.cs
- DataPagerFieldCollection.cs
- HostingPreferredMapPath.cs
- XmlSchemaAnnotation.cs
- XmlDataSourceNodeDescriptor.cs
- ValueSerializerAttribute.cs
- Closure.cs
- RenderTargetBitmap.cs
- FormatVersion.cs
- TableLayout.cs
- SQLBoolean.cs
- ThreadSafeMessageFilterTable.cs
- SafeRightsManagementPubHandle.cs
- StatusBarItemAutomationPeer.cs
- UriSection.cs
- TraceUtils.cs
- Double.cs
- SqlCommandBuilder.cs
- ProcessModule.cs
- DodSequenceMerge.cs
- FormsAuthenticationUser.cs
- DispatcherOperation.cs
- MetadataSerializer.cs
- IndexerNameAttribute.cs
- OdbcConnectionPoolProviderInfo.cs
- NameSpaceExtractor.cs
- SqlNotificationEventArgs.cs
- TypographyProperties.cs
- DataListItem.cs
- ServiceOperation.cs
- ClientFormsIdentity.cs
- RoleGroupCollectionEditor.cs
- TimelineGroup.cs
- GradientStopCollection.cs
- SqlCommandSet.cs
- DetailsViewPageEventArgs.cs
- ItemsPanelTemplate.cs
- OutputCacheSection.cs
- Path.cs
- TransformDescriptor.cs