Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / ThreadSafeMessageFilterTable.cs / 1 / ThreadSafeMessageFilterTable.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.Threading; using System.ServiceModel; using System.ServiceModel.Channels; using System.Collections; using System.Collections.Generic; internal class ThreadSafeMessageFilterTable: IMessageFilterTable { MessageFilterTable table; object syncRoot; internal ThreadSafeMessageFilterTable() { this.table = new MessageFilterTable (); this.syncRoot = new object(); } internal object SyncRoot { get { return this.syncRoot; } } public int DefaultPriority { get { lock (this.syncRoot) { return this.table.DefaultPriority; } } set { lock (this.syncRoot) { this.table.DefaultPriority = value; } } } internal void Add(MessageFilter filter, FilterData data, int priority) { lock (this.syncRoot) { this.table.Add(filter, data, priority); } } // // IMessageFilterTable methods // public int Count { get { lock (this.syncRoot) { return this.table.Count; } } } public void Clear() { lock (this.syncRoot) { this.table.Clear(); } } public bool GetMatchingValue(Message message, out FilterData data) { lock (this.syncRoot) { return this.table.GetMatchingValue(message, out data); } } public bool GetMatchingValue(MessageBuffer buffer, out FilterData data) { lock (this.syncRoot) { return this.table.GetMatchingValue(buffer, out data); } } public bool GetMatchingValues(Message message, ICollection results) { lock (this.syncRoot) { return this.table.GetMatchingValues(message, results); } } public bool GetMatchingValues(MessageBuffer buffer, ICollection results) { lock (this.syncRoot) { return this.table.GetMatchingValues(buffer, results); } } public bool GetMatchingFilter(Message message, out MessageFilter filter) { lock (this.syncRoot) { return this.table.GetMatchingFilter(message, out filter); } } public bool GetMatchingFilter(MessageBuffer buffer, out MessageFilter filter) { lock (this.syncRoot) { return this.table.GetMatchingFilter(buffer, out filter); } } public bool GetMatchingFilters(Message message, ICollection results) { lock (this.syncRoot) { return this.table.GetMatchingFilters(message, results); } } public bool GetMatchingFilters(MessageBuffer buffer, ICollection results) { lock (this.syncRoot) { return this.table.GetMatchingFilters(buffer, results); } } // // IDictionary methods // public FilterData this[MessageFilter key] { get { lock (this.syncRoot) { return this.table[key]; } } set { lock (this.syncRoot) { this.table[key] = value; } } } public ICollection Keys { get { lock (this.syncRoot) { return this.table.Keys; } } } public ICollection Values { get { lock (this.syncRoot) { return this.table.Values; } } } public bool ContainsKey(MessageFilter key) { lock (this.syncRoot) { return this.table.ContainsKey(key); } } public void Add(MessageFilter key, FilterData value) { lock (this.syncRoot) { this.table.Add(key, value); } } public bool Remove(MessageFilter key) { lock (this.syncRoot) { return this.table.Remove(key); } } // // ICollection > methods // bool ICollection >.IsReadOnly { get { lock (this.syncRoot) { return ((ICollection >)this.table).IsReadOnly; } } } void ICollection >.Add(KeyValuePair item) { lock (this.syncRoot) { ((ICollection >)this.table).Add(item); } } bool ICollection >.Contains(KeyValuePair item) { lock (this.syncRoot) { return ((ICollection >)this.table).Contains(item); } } void ICollection >.CopyTo(KeyValuePair [] array, int arrayIndex) { lock (this.syncRoot) { ((ICollection >)this.table).CopyTo(array, arrayIndex); } } bool ICollection >.Remove(KeyValuePair item) { lock (this.syncRoot) { return ((ICollection >)this.table).Remove(item); } } IEnumerator IEnumerable.GetEnumerator() { lock (this.syncRoot) { return ((IEnumerable >)this).GetEnumerator(); } } IEnumerator > IEnumerable >.GetEnumerator() { lock (this.syncRoot) { return ((ICollection >)this.table).GetEnumerator(); } } public bool TryGetValue(MessageFilter filter, out FilterData data) { lock (this.syncRoot) { return this.table.TryGetValue(filter, out data); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
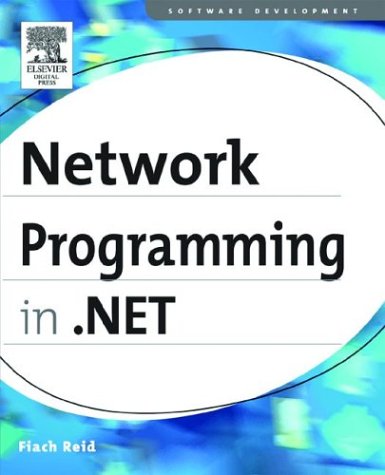
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaComplexType.cs
- ListBoxAutomationPeer.cs
- Wizard.cs
- XmlNodeChangedEventArgs.cs
- XmlFormatReaderGenerator.cs
- StrictAndMessageFilter.cs
- ExpressionVisitorHelpers.cs
- WizardForm.cs
- CryptoConfig.cs
- Block.cs
- LocatorPart.cs
- QueryOptionExpression.cs
- HScrollProperties.cs
- CalendarTable.cs
- InputLanguageSource.cs
- SchemaCollectionPreprocessor.cs
- LinqDataSourceValidationException.cs
- JsonServiceDocumentSerializer.cs
- BindingExpressionUncommonField.cs
- Bezier.cs
- SecUtil.cs
- Int16.cs
- PropertyGridEditorPart.cs
- contentDescriptor.cs
- ApplicationException.cs
- OdbcRowUpdatingEvent.cs
- Thumb.cs
- TextBoxAutoCompleteSourceConverter.cs
- DataSourceXmlElementAttribute.cs
- ManualResetEvent.cs
- WizardStepCollectionEditor.cs
- PageFunction.cs
- HatchBrush.cs
- ObjectDataSourceSelectingEventArgs.cs
- KeyValueConfigurationCollection.cs
- QilVisitor.cs
- NotCondition.cs
- configsystem.cs
- LogReservationCollection.cs
- Win32SafeHandles.cs
- webeventbuffer.cs
- CssClassPropertyAttribute.cs
- PointF.cs
- NetPeerTcpBindingElement.cs
- ReturnEventArgs.cs
- DataObjectSettingDataEventArgs.cs
- AdapterDictionary.cs
- HostedElements.cs
- OleDbPropertySetGuid.cs
- SqlTransaction.cs
- AssemblyAttributes.cs
- ContextMenuStrip.cs
- OleAutBinder.cs
- PackWebRequest.cs
- EditorAttribute.cs
- ViewBase.cs
- PatternMatcher.cs
- ProcessHostConfigUtils.cs
- ScriptServiceAttribute.cs
- TextEditorTyping.cs
- SafeNativeMethods.cs
- PlatformCulture.cs
- ProxyWebPartManager.cs
- TreeNodeBinding.cs
- elementinformation.cs
- CellNormalizer.cs
- DmlSqlGenerator.cs
- WindowsFormsSynchronizationContext.cs
- XmlSchemaImport.cs
- SmtpDigestAuthenticationModule.cs
- EventSinkActivityDesigner.cs
- ToolStripComboBox.cs
- CollectionType.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- ExpressionVisitor.cs
- ConfigurationStrings.cs
- SecurityPolicySection.cs
- IndentedWriter.cs
- ConfigXmlComment.cs
- ReflectionServiceProvider.cs
- ValidatingPropertiesEventArgs.cs
- DataGridViewLinkCell.cs
- PolicyException.cs
- HttpBrowserCapabilitiesWrapper.cs
- WebPartsSection.cs
- UTF7Encoding.cs
- UnsettableComboBox.cs
- AutomationInteropProvider.cs
- Annotation.cs
- XmlHierarchicalDataSourceView.cs
- OutputCacheSection.cs
- ListSourceHelper.cs
- WebPartEditorApplyVerb.cs
- DataError.cs
- Cast.cs
- ValueType.cs
- SqlDataSourceCommandEventArgs.cs
- ObservableCollection.cs
- SoapFault.cs
- ToolStripAdornerWindowService.cs