Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Metadata / MetadataArtifactLoaderCompositeResource.cs / 1305376 / MetadataArtifactLoaderCompositeResource.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Collections; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Text; using System.Xml; using System.Data.Mapping; using System.IO; using System.Data.Entity; using System.Security; using System.Security.Permissions; using System.Collections.ObjectModel; namespace System.Data.Metadata.Edm { ////// This class represents a collection of resources to be loaded from one /// or more assemblies. /// internal class MetadataArtifactLoaderCompositeResource : MetadataArtifactLoader { ////// The list of metadata artifacts encapsulated by the composite. /// private readonly ReadOnlyCollection_children; private readonly string _originalPath; /// /// This constructor expects to get the paths that have potential to turn into multiple /// artifacts like /// /// res://*/foo.csdl -- could be multiple assemblies /// res://MyAssembly/ -- could be multiple artifacts in the one assembly /// /// /// The path to the (collection of) resources /// The global registry of URIs /// internal MetadataArtifactLoaderCompositeResource(string originalPath, string assemblyName, string resourceName, ICollectionuriRegistry, MetadataArtifactAssemblyResolver resolver) { Debug.Assert(resolver != null); _originalPath = originalPath; _children = LoadResources(assemblyName, resourceName, uriRegistry, resolver).AsReadOnly(); } public override string Path { get { return _originalPath; } } public override bool IsComposite { get { return true; } } public override void CollectFilePermissionPaths(List paths, DataSpace spaceToGet) { foreach (var loader in _children) { loader.CollectFilePermissionPaths(paths, spaceToGet); } } /// /// Get paths to artifacts for a specific DataSpace, in the original, unexpanded /// form. /// ///An assembly can embed any kind of artifact as a resource, so we simply /// ignore the parameter and return the original assembly name in the URI. /// The DataSpace for the artifacts of interest ///A List of strings identifying paths to all artifacts for a specific DataSpace public override ListGetOriginalPaths(DataSpace spaceToGet) { return GetOriginalPaths(); } /// /// Get paths to artifacts for a specific DataSpace. /// /// The DataSpace for the artifacts of interest ///A List of strings identifying paths to all artifacts for a specific DataSpace public override ListGetPaths(DataSpace spaceToGet) { List list = new List (); foreach (MetadataArtifactLoaderResource resource in _children) { list.AddRange(resource.GetPaths(spaceToGet)); } return list; } /// /// Get paths to all artifacts /// ///A List of strings identifying paths to all resources public override ListGetPaths() { List list = new List (); foreach (MetadataArtifactLoaderResource resource in _children) { list.AddRange(resource.GetPaths()); } return list; } /// /// Aggregates all resource streams from the _children collection /// ///A List of XmlReader objects; cannot be null public override ListGetReaders(Dictionary sourceDictionary) { List list = new List (); foreach (MetadataArtifactLoaderResource resource in _children) { list.AddRange(resource.GetReaders(sourceDictionary)); } return list; } /// /// Get XmlReaders for a specific DataSpace. /// /// The DataSpace corresponding to the requested artifacts ///A List of XmlReader objects public override ListCreateReaders(DataSpace spaceToGet) { List list = new List (); foreach (MetadataArtifactLoaderResource resource in _children) { list.AddRange(resource.CreateReaders(spaceToGet)); } return list; } /// /// Load all resources from the assembly/assemblies identified in the resource path. /// /// The global registry of URIs /// ///private static List LoadResources(string assemblyName, string resourceName, ICollection uriRegistry, MetadataArtifactAssemblyResolver resolver) { Debug.Assert(resolver != null); List loaders = new List (); Debug.Assert(!string.IsNullOrEmpty(assemblyName)); if (assemblyName == MetadataArtifactLoader.wildcard) { foreach (Assembly assembly in resolver.GetWildcardAssemblies()) { if (AssemblyContainsResource(assembly, ref resourceName)) { LoadResourcesFromAssembly(assembly, resourceName, uriRegistry, loaders, resolver); } } } else { Assembly assembly = ResolveAssemblyName(assemblyName, resolver); LoadResourcesFromAssembly(assembly, resourceName, uriRegistry, loaders, resolver); } if (resourceName != null && loaders.Count == 0) { // they were asking for a specific resource name, and we didn't find it throw EntityUtil.Metadata(System.Data.Entity.Strings.UnableToLoadResource); } return loaders; } private static bool AssemblyContainsResource(Assembly assembly, ref string resourceName) { if (resourceName == null) { return true; } string[] allresources = GetManifestResourceNamesForAssembly(assembly); foreach (string current in allresources) { if (string.Equals(resourceName, current, StringComparison.OrdinalIgnoreCase)) { resourceName = current; return true; } } return false; } private static void LoadResourcesFromAssembly(Assembly assembly, string resourceName, ICollection uriRegistry, List loaders, MetadataArtifactAssemblyResolver resolver) { if (resourceName == null) { LoadAllResourcesFromAssembly(assembly, uriRegistry, loaders, resolver); } else if (AssemblyContainsResource(assembly, ref resourceName)) { CreateAndAddSingleResourceLoader(assembly, resourceName, uriRegistry, loaders); } else { throw EntityUtil.Metadata(System.Data.Entity.Strings.UnableToLoadResource); } } private static void LoadAllResourcesFromAssembly(Assembly assembly, ICollection uriRegistry, List loaders, MetadataArtifactAssemblyResolver resolver) { Debug.Assert(assembly != null); string[] allresources = GetManifestResourceNamesForAssembly(assembly); foreach (string resourceName in allresources) { CreateAndAddSingleResourceLoader(assembly, resourceName, uriRegistry, loaders); } } private static void CreateAndAddSingleResourceLoader(Assembly assembly, string resourceName, ICollection uriRegistry, List loaders) { Debug.Assert(resourceName != null); Debug.Assert(assembly != null); string resourceUri = CreateResPath(assembly, resourceName); if (!uriRegistry.Contains(resourceUri)) { loaders.Add(new MetadataArtifactLoaderResource(assembly, resourceName, uriRegistry)); } } internal static string CreateResPath(Assembly assembly, string resourceName) { string resourceUri = string.Format(CultureInfo.InvariantCulture, "{0}{1}{2}{3}", resPathPrefix, assembly.FullName, resPathSeparator, resourceName); return resourceUri; } internal static string[] GetManifestResourceNamesForAssembly(Assembly assembly) { Debug.Assert(assembly != null); return !assembly.IsDynamic ? assembly.GetManifestResourceNames() : new string[0]; } /// /// Load all resources from a specific assembly. /// /// The full name identifying the assembly to /// load resources from /// The global registry of URIs /// delegate for resolve the assembly private static Assembly ResolveAssemblyName(string assemblyName, MetadataArtifactAssemblyResolver resolver) { Debug.Assert(resolver != null); AssemblyName referenceName = new AssemblyName(assemblyName); Assembly assembly; if(!resolver.TryResolveAssemblyReference(referenceName, out assembly)) { throw new FileNotFoundException(Strings.UnableToResolveAssembly(assemblyName)); } return assembly; } internal static MetadataArtifactLoader CreateResourceLoader(string path, ExtensionCheck extensionCheck, string validExtension, ICollectionuriRegistry, MetadataArtifactAssemblyResolver resolver) { Debug.Assert(path != null); Debug.Assert(MetadataArtifactLoader.PathStartsWithResPrefix(path)); // if the supplied path ends with a separator, or contains only one // segment (i.e., the name of an assembly, or the wildcard character), // create a composite loader that can extract resources from one or // more assemblies // bool createCompositeResLoader = false; string assemblyName = null; string resourceName = null; ParseResourcePath(path, out assemblyName, out resourceName); createCompositeResLoader = (assemblyName != null) && (resourceName == null || assemblyName.Trim() == wildcard); ValidateExtension(extensionCheck, validExtension, resourceName); if (createCompositeResLoader) { return new MetadataArtifactLoaderCompositeResource(path, assemblyName, resourceName, uriRegistry, resolver); } Debug.Assert(!string.IsNullOrEmpty(resourceName), "we should not get here is the resourceName is null"); Assembly assembly = ResolveAssemblyName(assemblyName, resolver); return new MetadataArtifactLoaderResource(assembly, resourceName, uriRegistry); } private static void ValidateExtension(ExtensionCheck extensionCheck, string validExtension, string resourceName) { if (resourceName == null) { return; } // the supplied path represents a single resource // switch (extensionCheck) { case ExtensionCheck.Specific: MetadataArtifactLoader.CheckArtifactExtension(resourceName, validExtension); break; case ExtensionCheck.All: if (!MetadataArtifactLoader.IsValidArtifact(resourceName)) { throw EntityUtil.Metadata(Strings.InvalidMetadataPath); } break; } } /// /// Splits the supplied path into the assembly portion and the resource /// part (if any) /// /// The resource path to parse ///An array of (two) strings containing the assembly name /// and the resource name private static void ParseResourcePath(string path, out string assemblyName, out string resourceName) { // Extract the components from the path int prefixLength = MetadataArtifactLoader.resPathPrefix.Length; string[] result = path.Substring(prefixLength).Split( new string[] { MetadataArtifactLoader.resPathSeparator, MetadataArtifactLoader.altPathSeparator }, StringSplitOptions.RemoveEmptyEntries ); if (result.Length == 0 || result.Length > 2) { throw EntityUtil.Metadata(Strings.InvalidMetadataPath); } if (result.Length >= 1) { assemblyName = result[0]; } else { assemblyName = null; } if (result.Length == 2) { resourceName = result[1]; } else { resourceName = null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
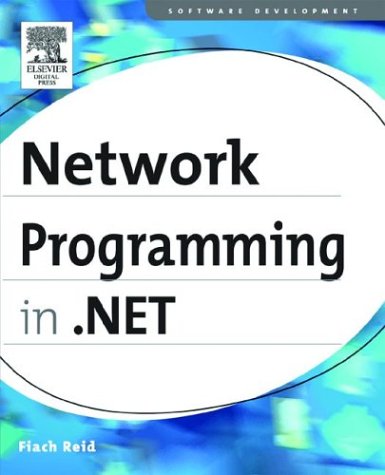
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RoutedEvent.cs
- SqlDataSourceView.cs
- ConnectionConsumerAttribute.cs
- PathGradientBrush.cs
- TableLayoutCellPaintEventArgs.cs
- ScriptReference.cs
- CatalogPart.cs
- StyleBamlRecordReader.cs
- HybridDictionary.cs
- LightweightCodeGenerator.cs
- TagPrefixCollection.cs
- CoTaskMemHandle.cs
- EnvelopedPkcs7.cs
- CreateRefExpr.cs
- CompilationUtil.cs
- FragmentNavigationEventArgs.cs
- DBSchemaRow.cs
- TextShapeableCharacters.cs
- TextServicesCompartmentContext.cs
- FragmentNavigationEventArgs.cs
- TcpChannelHelper.cs
- InstanceDescriptor.cs
- OleAutBinder.cs
- ToolBarPanel.cs
- CallSiteOps.cs
- ReadWriteSpinLock.cs
- CompilationLock.cs
- TableLayoutSettings.cs
- CodeVariableDeclarationStatement.cs
- ChangeBlockUndoRecord.cs
- QueryConverter.cs
- RepeaterItem.cs
- DataViewSettingCollection.cs
- HyperLinkStyle.cs
- PermissionAttributes.cs
- DbConnectionPoolGroupProviderInfo.cs
- AuthenticationException.cs
- ModelItemCollection.cs
- ListItemsCollectionEditor.cs
- SupportingTokenChannel.cs
- CompositeScriptReference.cs
- DataGridViewRowConverter.cs
- ObjectIDGenerator.cs
- VariantWrapper.cs
- RepeaterItemEventArgs.cs
- SecondaryViewProvider.cs
- WebServiceErrorEvent.cs
- util.cs
- AmbiguousMatchException.cs
- DiscoveryUtility.cs
- ExportFileRequest.cs
- MemberCollection.cs
- RuleSetDialog.Designer.cs
- FlowSwitchDesigner.xaml.cs
- CodeDirectionExpression.cs
- ConsumerConnectionPointCollection.cs
- HuffmanTree.cs
- BinaryReader.cs
- XmlSchemaObjectTable.cs
- Single.cs
- TreeNode.cs
- TextServicesDisplayAttribute.cs
- EncoderReplacementFallback.cs
- StreamBodyWriter.cs
- MethodRental.cs
- HtmlInputButton.cs
- MimeMapping.cs
- CompositeControlDesigner.cs
- TemplatingOptionsDialog.cs
- CodeConditionStatement.cs
- SQLDateTimeStorage.cs
- AccessDataSource.cs
- ChannelBinding.cs
- PartEditor.cs
- ServiceControllerDesigner.cs
- BatchParser.cs
- JsonFormatWriterGenerator.cs
- MailWriter.cs
- TransformDescriptor.cs
- Flowchart.cs
- WebPartsSection.cs
- SqlServer2KCompatibilityCheck.cs
- CookieParameter.cs
- HeaderedItemsControl.cs
- DocumentViewerAutomationPeer.cs
- ElementsClipboardData.cs
- Translator.cs
- SerializableTypeCodeDomSerializer.cs
- DataTableCollection.cs
- ResourceIDHelper.cs
- CodeBlockBuilder.cs
- CqlQuery.cs
- RecordManager.cs
- Activator.cs
- SecurityElement.cs
- CodeParameterDeclarationExpressionCollection.cs
- ConfigurationSchemaErrors.cs
- Crc32.cs
- XmlQualifiedNameTest.cs
- Package.cs