Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / Messaging / SupportingTokenChannel.cs / 1 / SupportingTokenChannel.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // Defines the SupportingTokenChannel, with the only interesting code in these parts using System; using System.Diagnostics; using System.ServiceModel; using System.ServiceModel.Description; using System.ServiceModel.Channels; using System.ServiceModel.Security; using Microsoft.Transactions.Wsat.Protocol; namespace Microsoft.Transactions.Wsat.Messaging { abstract class SupportingTokenChannel: ChannelBase where TChannel : IChannel { SupportingTokenSecurityTokenResolver tokenResolver; protected TChannel innerChannel; static SecurityStandardsManager securityStandardsManager; ProtocolVersion protocolVersion; static SecurityStandardsManager SecurityStandardsManager { get { if (securityStandardsManager == null) { securityStandardsManager = new SecurityStandardsManager(MessageSecurityVersion.Default, WSSecurityTokenSerializer.DefaultInstance); } return securityStandardsManager; } } public SupportingTokenChannel(ChannelManagerBase manager, TChannel innerChannel, SupportingTokenSecurityTokenResolver tokenResolver, ProtocolVersion protocolVersion) : base(manager) { this.innerChannel = innerChannel; this.tokenResolver = tokenResolver; this.protocolVersion = protocolVersion; } protected override void OnAbort() { this.innerChannel.Abort(); } protected override IAsyncResult OnBeginClose(TimeSpan timeout, AsyncCallback callback, object state) { return this.innerChannel.BeginClose(timeout, callback, state); } protected override IAsyncResult OnBeginOpen(TimeSpan timeout, AsyncCallback callback, object state) { return this.innerChannel.BeginOpen(timeout, callback, state); } protected override void OnClose(TimeSpan timeout) { this.innerChannel.Close(timeout); } protected override void OnEndClose(IAsyncResult result) { this.innerChannel.EndClose(result); } protected override void OnEndOpen(IAsyncResult result) { this.innerChannel.EndOpen(result); } protected override void OnOpen(TimeSpan timeout) { this.innerChannel.Open(timeout); } protected void OnReceive(Message message) { DebugTrace.TraceEnter(this, "OnReceive"); // This is the big payoff for all this SupportingToken* work if (message != null && !this.tokenResolver.FaultInSupportingToken(message)) { if (DebugTrace.Verbose) DebugTrace.Trace(TraceLevel.Verbose, "Failed to fault in SCT for supporting token signature"); // Send a fault Fault fault = Faults.Version(this.protocolVersion).InvalidParameters; if (message.Headers.MessageId != null) { if (DebugTrace.Verbose) DebugTrace.Trace(TraceLevel.Verbose, "Attempting to send {0} fault", fault.Code.Name); Message faultMessage = Library.CreateFaultMessage(message.Headers.MessageId, message.Version, fault); // Note that we already have a RelatesTo on the message, so there's no need // to call RequestReplyCorrelator.PrepareReply RequestReplyCorrelator.AddressReply(faultMessage, new RequestReplyCorrelator.ReplyToInfo(message)); SendSecurityHeader securityHeader = SecurityStandardsManager.CreateSendSecurityHeader( faultMessage, string.Empty, true, false, SecurityAlgorithmSuite.Default, MessageDirection.Output); securityHeader.RequireMessageProtection = false; securityHeader.AddTimestamp(SecurityProtocolFactory.defaultTimestampValidityDuration); faultMessage = securityHeader.SetupExecution(); TrySendFaultReply(faultMessage); } // Reject the message throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new CommunicationException(SR.GetString(SR.SupportingTokenSignatureExpected))); } DebugTrace.TraceLeave(this, "OnReceive"); } public override T GetProperty () { return this.innerChannel.GetProperty (); } protected abstract void TrySendFaultReply(Message faultMessage); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
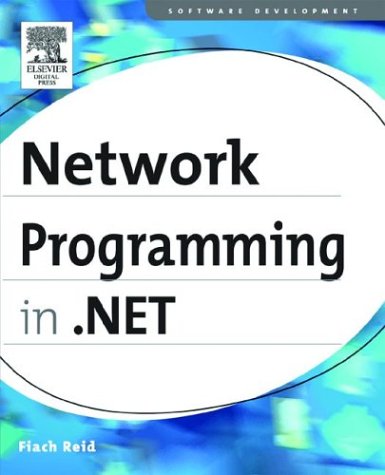
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XNodeNavigator.cs
- UnhandledExceptionEventArgs.cs
- TextElementEnumerator.cs
- FileSystemWatcher.cs
- SoapException.cs
- PackageProperties.cs
- WebPartTransformerAttribute.cs
- VectorKeyFrameCollection.cs
- Expressions.cs
- ToolBarTray.cs
- SoapAttributes.cs
- SmiXetterAccessMap.cs
- CodeObjectCreateExpression.cs
- MemoryRecordBuffer.cs
- Misc.cs
- EntitySqlQueryCacheKey.cs
- MultipleCopiesCollection.cs
- AncestorChangedEventArgs.cs
- ToolStripSplitStackLayout.cs
- HashHelper.cs
- CounterSample.cs
- NativeBuffer.cs
- EncodingTable.cs
- CngKeyBlobFormat.cs
- safex509handles.cs
- SortFieldComparer.cs
- HostingEnvironmentWrapper.cs
- KnownColorTable.cs
- StorageScalarPropertyMapping.cs
- HtmlMeta.cs
- BitHelper.cs
- FollowerQueueCreator.cs
- SystemUdpStatistics.cs
- SafeFindHandle.cs
- FileChangesMonitor.cs
- Component.cs
- PageCache.cs
- TemplateBindingExtensionConverter.cs
- ObjectTag.cs
- HttpProfileBase.cs
- PropertyNames.cs
- FixedSOMPage.cs
- FileSecurity.cs
- OverflowException.cs
- ClickablePoint.cs
- StringDictionary.cs
- FilterEventArgs.cs
- DetailsViewUpdateEventArgs.cs
- NameValuePermission.cs
- BamlBinaryReader.cs
- HttpCookie.cs
- AstTree.cs
- CodeMethodReturnStatement.cs
- GridView.cs
- Avt.cs
- HitTestParameters.cs
- StyleXamlParser.cs
- HtmlElementCollection.cs
- Sentence.cs
- InitializationEventAttribute.cs
- LinearKeyFrames.cs
- SystemBrushes.cs
- TraceSection.cs
- DisplayMemberTemplateSelector.cs
- BitmapPalettes.cs
- ViewSimplifier.cs
- StringUtil.cs
- EntityStoreSchemaGenerator.cs
- WebPartChrome.cs
- TriggerActionCollection.cs
- UpdateException.cs
- TimelineGroup.cs
- SQLDecimal.cs
- PluralizationServiceUtil.cs
- Native.cs
- AdapterUtil.cs
- StylusEventArgs.cs
- CodeArgumentReferenceExpression.cs
- DataSourceControl.cs
- HideDisabledControlAdapter.cs
- AdCreatedEventArgs.cs
- AutomationPatternInfo.cs
- ListViewHitTestInfo.cs
- WindowsGraphicsWrapper.cs
- UserNamePasswordValidator.cs
- AssemblyAttributes.cs
- ApplicationCommands.cs
- NetSectionGroup.cs
- ListSortDescriptionCollection.cs
- CommandBinding.cs
- PagePropertiesChangingEventArgs.cs
- TypeToken.cs
- XamlBrushSerializer.cs
- Identity.cs
- CSharpCodeProvider.cs
- CachedBitmap.cs
- ObsoleteAttribute.cs
- GeneratedCodeAttribute.cs
- AnnouncementEndpointElement.cs
- SettingsPropertyCollection.cs