Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Markup / XamlBrushSerializer.cs / 1 / XamlBrushSerializer.cs
//---------------------------------------------------------------------------- // // File: XamlBrushSerializer.cs // // Description: // XamlSerializer used to persist Brush objects in Baml // // Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.IO; using System.Xml; using MS.Utility; using MS.Internal; #if PBTCOMPILER using System.Reflection; namespace MS.Internal.Markup #else using System.Windows; using System.Windows.Media; namespace System.Windows.Markup #endif { ////// XamlBrushSerializer is used to persist a Brush in Baml files /// ////// The brush serializer currently only handles solid color brushes. Other types of /// are not persisted in a custom binary format. /// internal class XamlBrushSerializer : XamlSerializer { #region Construction ////// Constructor for XamlBrushSerializer /// ////// This constructor will be used under /// the following two scenarios /// 1. Convert a string to a custom binary representation stored in BAML /// 2. Convert a custom binary representation back into a Brush /// public XamlBrushSerializer() { } #endregion Construction #region Conversions ////// Convert a string into a compact binary representation and write it out /// to the passed BinaryWriter. /// ////// This is called ONLY from the Parser and is not a general public method. /// This currently only works for SolidColorBrushes that are identified /// by a known color name (eg - "Green" ) /// public override bool ConvertStringToCustomBinary ( BinaryWriter writer, // Writer into the baml stream string stringValue) // String to convert { #if !PBTCOMPILER return SolidColorBrush.SerializeOn(writer, stringValue.Trim()); #else return SerializeOn(writer, stringValue.Trim()); #endif } #if !PBTCOMPILER ////// Convert a compact binary representation of a Brush into and instance /// of Brush. The reader must be left pointing immediately after the object /// data in the underlying stream. /// ////// This is called ONLY from the Parser and is not a general public method. /// public override object ConvertCustomBinaryToObject( BinaryReader reader) { // ********* VERY IMPORTANT NOTE ***************** // If this method is changed, then BamlPropertyCustomRecord.GetCustomValue() needs // to be correspondingly changed as well // ********* VERY IMPORTANT NOTE ***************** return SolidColorBrush.DeserializeFrom(reader); } #else private static bool SerializeOn(BinaryWriter writer, string stringValue) { if (writer == null) { throw new ArgumentNullException("writer"); } KnownColor knownColor = KnownColors.ColorStringToKnownColor(stringValue); if (knownColor != KnownColor.UnknownColor) { // Serialize values of the type "Red", "Blue" and other names writer.Write((byte)SerializationBrushType.KnownSolidColor); writer.Write((uint)knownColor); return true; } else { // Serialize values of the type "#F00", "#0000FF" and other hex color values. // We don't have a good way to check if this is valid without running the // converter at this point, so just store the string if it has at least a // minimum length of 4. stringValue = stringValue.Trim(); if (stringValue.Length > 3) { writer.Write((byte)SerializationBrushType.OtherColor); writer.Write(stringValue); return true; } } return false; } // This enum is used to identify brush types for deserialization in the // ConvertCustomBinaryToObject method. internal enum SerializationBrushType : byte { Unknown = 0, KnownSolidColor = 1, OtherColor = 2, } #endif #endregion Conversions } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: XamlBrushSerializer.cs // // Description: // XamlSerializer used to persist Brush objects in Baml // // Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.IO; using System.Xml; using MS.Utility; using MS.Internal; #if PBTCOMPILER using System.Reflection; namespace MS.Internal.Markup #else using System.Windows; using System.Windows.Media; namespace System.Windows.Markup #endif { ////// XamlBrushSerializer is used to persist a Brush in Baml files /// ////// The brush serializer currently only handles solid color brushes. Other types of /// are not persisted in a custom binary format. /// internal class XamlBrushSerializer : XamlSerializer { #region Construction ////// Constructor for XamlBrushSerializer /// ////// This constructor will be used under /// the following two scenarios /// 1. Convert a string to a custom binary representation stored in BAML /// 2. Convert a custom binary representation back into a Brush /// public XamlBrushSerializer() { } #endregion Construction #region Conversions ////// Convert a string into a compact binary representation and write it out /// to the passed BinaryWriter. /// ////// This is called ONLY from the Parser and is not a general public method. /// This currently only works for SolidColorBrushes that are identified /// by a known color name (eg - "Green" ) /// public override bool ConvertStringToCustomBinary ( BinaryWriter writer, // Writer into the baml stream string stringValue) // String to convert { #if !PBTCOMPILER return SolidColorBrush.SerializeOn(writer, stringValue.Trim()); #else return SerializeOn(writer, stringValue.Trim()); #endif } #if !PBTCOMPILER ////// Convert a compact binary representation of a Brush into and instance /// of Brush. The reader must be left pointing immediately after the object /// data in the underlying stream. /// ////// This is called ONLY from the Parser and is not a general public method. /// public override object ConvertCustomBinaryToObject( BinaryReader reader) { // ********* VERY IMPORTANT NOTE ***************** // If this method is changed, then BamlPropertyCustomRecord.GetCustomValue() needs // to be correspondingly changed as well // ********* VERY IMPORTANT NOTE ***************** return SolidColorBrush.DeserializeFrom(reader); } #else private static bool SerializeOn(BinaryWriter writer, string stringValue) { if (writer == null) { throw new ArgumentNullException("writer"); } KnownColor knownColor = KnownColors.ColorStringToKnownColor(stringValue); if (knownColor != KnownColor.UnknownColor) { // Serialize values of the type "Red", "Blue" and other names writer.Write((byte)SerializationBrushType.KnownSolidColor); writer.Write((uint)knownColor); return true; } else { // Serialize values of the type "#F00", "#0000FF" and other hex color values. // We don't have a good way to check if this is valid without running the // converter at this point, so just store the string if it has at least a // minimum length of 4. stringValue = stringValue.Trim(); if (stringValue.Length > 3) { writer.Write((byte)SerializationBrushType.OtherColor); writer.Write(stringValue); return true; } } return false; } // This enum is used to identify brush types for deserialization in the // ConvertCustomBinaryToObject method. internal enum SerializationBrushType : byte { Unknown = 0, KnownSolidColor = 1, OtherColor = 2, } #endif #endregion Conversions } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
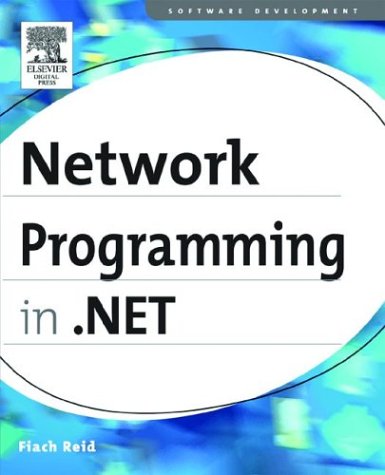
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SID.cs
- uribuilder.cs
- Parser.cs
- _AuthenticationState.cs
- EntryPointNotFoundException.cs
- XamlSerializationHelper.cs
- QueryOptionExpression.cs
- securitycriticaldataformultiplegetandset.cs
- ByteStream.cs
- TextServicesCompartmentContext.cs
- RolePrincipal.cs
- AsyncPostBackErrorEventArgs.cs
- tooltip.cs
- DataControlField.cs
- FormsAuthenticationUserCollection.cs
- GridSplitterAutomationPeer.cs
- ValidationHelpers.cs
- OutKeywords.cs
- WebPartEditorCancelVerb.cs
- DateTimePickerDesigner.cs
- X509CertificateChain.cs
- ImportCatalogPart.cs
- TitleStyle.cs
- Misc.cs
- URL.cs
- CalloutQueueItem.cs
- SimpleParser.cs
- MissingFieldException.cs
- XmlQualifiedName.cs
- WebPartExportVerb.cs
- SecurityTokenException.cs
- Crc32Helper.cs
- SettingsSection.cs
- AdRotator.cs
- MetadataArtifactLoaderCompositeResource.cs
- ResourcePool.cs
- WindowsListViewSubItem.cs
- CellIdBoolean.cs
- StylusTouchDevice.cs
- SelectionRangeConverter.cs
- DataBindingCollection.cs
- _ListenerRequestStream.cs
- Inline.cs
- DynamicRouteExpression.cs
- ProgressBar.cs
- MultiSelectRootGridEntry.cs
- WebPartHeaderCloseVerb.cs
- CompiledRegexRunnerFactory.cs
- CustomBindingElementCollection.cs
- Cursor.cs
- Privilege.cs
- XsltException.cs
- GeometryGroup.cs
- SafeEventLogWriteHandle.cs
- ConditionalAttribute.cs
- CompressEmulationStream.cs
- Ref.cs
- UnsafeNativeMethods.cs
- DetailsViewDeletedEventArgs.cs
- KeyEvent.cs
- OpCodes.cs
- DataPagerFieldCollection.cs
- ObjectDisposedException.cs
- MailMessage.cs
- GlyphInfoList.cs
- JournalEntryListConverter.cs
- GeometryHitTestParameters.cs
- ObjectResult.cs
- WebConfigurationManager.cs
- ComponentResourceManager.cs
- PointCollection.cs
- DesignerActionItemCollection.cs
- ModulesEntry.cs
- XmlSerializer.cs
- ReadOnlyTernaryTree.cs
- AppLevelCompilationSectionCache.cs
- SystemDropShadowChrome.cs
- RepeaterItemEventArgs.cs
- StrokeRenderer.cs
- SqlCacheDependency.cs
- ScrollPatternIdentifiers.cs
- XmlSchemaSimpleType.cs
- TreeViewItem.cs
- SqlDelegatedTransaction.cs
- RelationshipSet.cs
- SystemException.cs
- InitializingNewItemEventArgs.cs
- ConfigurationStrings.cs
- AssignDesigner.xaml.cs
- HtmlInputControl.cs
- UserControl.cs
- LoginView.cs
- StrongNameIdentityPermission.cs
- HttpValueCollection.cs
- VBCodeProvider.cs
- TemplateComponentConnector.cs
- FullTrustAssembly.cs
- ObjectDisposedException.cs
- HtmlUtf8RawTextWriter.cs
- DetailsViewModeEventArgs.cs