Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / Configuration / WebConfigurationManager.cs / 1 / WebConfigurationManager.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Collections; using System.Collections.Specialized; using System.Configuration; using System.Security; using System.Security.Permissions; using System.Web.Hosting; [AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal)] public static class WebConfigurationManager { public static NameValueCollection AppSettings { get { return ConfigurationManager.AppSettings; } } public static ConnectionStringSettingsCollection ConnectionStrings { get { return ConfigurationManager.ConnectionStrings; } } public static object GetSection(string sectionName) { if (HttpConfigurationSystem.UseHttpConfigurationSystem) { return HttpConfigurationSystem.GetSection(sectionName); } else { return ConfigurationManager.GetSection(sectionName); } } public static object GetSection(string sectionName, string path) { if (HttpConfigurationSystem.UseHttpConfigurationSystem) { return HttpConfigurationSystem.GetSection(sectionName, path); } else { throw new InvalidOperationException(SR.GetString(SR.Config_GetSectionWithPathArgInvalid)); } } public static object GetWebApplicationSection(string sectionName) { if (HttpConfigurationSystem.UseHttpConfigurationSystem) { return HttpConfigurationSystem.GetApplicationSection(sectionName); } else { return ConfigurationManager.GetSection(sectionName); } } // // ************************************************* // ** Static Management Functions to edit config ** // ************************************************* // private static Configuration OpenWebConfigurationImpl( WebLevel webLevel, ConfigurationFileMap fileMap, string path, string site, string locationSubPath, string server, string userName, string password, IntPtr userToken) { // In the hosted case, we allow app relative (~/....). Otherwise, it must be absolute VirtualPath virtualPath; if (HostingEnvironment.IsHosted) { virtualPath = VirtualPath.CreateNonRelativeAllowNull(path); } else { virtualPath = VirtualPath.CreateAbsoluteAllowNull(path); } return WebConfigurationHost.OpenConfiguration(webLevel, fileMap, virtualPath, site, locationSubPath, server, userName, password, userToken); } // // API extra notes: // // OpenWebConfiguration(null) // - Open root web.config // // OpenWebConfiguration("/fxtest"); // - If calling from a hosted app (e.g. an ASP.NET page), it will open web.config at // virtual path "/fxtest" in the current running application (which gives the site) // - If calling from a non-hosted app (e.g. console app), it will do the same thing // except it will use the default web site. // // OpenWebConfiguration("/", "1", "fxtest") // - Open web.config at the root of site "1" and get the config that applies to location "fxtest" // // // OpenMachineConfiguration // public static Configuration OpenMachineConfiguration() { return OpenWebConfigurationImpl(WebLevel.Machine, null, null, null, null, null, null, null, IntPtr.Zero); } public static Configuration OpenMachineConfiguration(string locationSubPath) { return OpenWebConfigurationImpl(WebLevel.Machine, null, null, null, locationSubPath, null, null, null, IntPtr.Zero); } public static Configuration OpenMachineConfiguration(string locationSubPath, string server) { return OpenWebConfigurationImpl(WebLevel.Machine, null, null, null, locationSubPath, server, null, null, IntPtr.Zero); } public static Configuration OpenMachineConfiguration(string locationSubPath, string server, string userName, string password) { return OpenWebConfigurationImpl(WebLevel.Machine, null, null, null, locationSubPath, server, userName, password, IntPtr.Zero); } [SecurityPermission(SecurityAction.Demand, Unrestricted = true)] public static Configuration OpenMachineConfiguration(string locationSubPath, string server, IntPtr userToken) { return OpenWebConfigurationImpl(WebLevel.Machine, null, null, null, locationSubPath, server, null, null, userToken); } public static Configuration OpenMappedMachineConfiguration(ConfigurationFileMap fileMap) { return OpenWebConfigurationImpl(WebLevel.Machine, fileMap, null, null, null, null, null, null, IntPtr.Zero); } public static Configuration OpenMappedMachineConfiguration(ConfigurationFileMap fileMap, string locationSubPath) { return OpenWebConfigurationImpl(WebLevel.Machine, fileMap, null, null, locationSubPath, null, null, null, IntPtr.Zero); } // // OpenWebConfiguration // public static Configuration OpenWebConfiguration(string path) { return OpenWebConfigurationImpl(WebLevel.Path, null, path, null, null, null, null, null, IntPtr.Zero); } public static Configuration OpenWebConfiguration(string path, string site) { return OpenWebConfigurationImpl(WebLevel.Path, null, path, site, null, null, null, null, IntPtr.Zero); } public static Configuration OpenWebConfiguration(string path, string site, string locationSubPath) { return OpenWebConfigurationImpl(WebLevel.Path, null, path, site, locationSubPath, null, null, null, IntPtr.Zero); } public static Configuration OpenWebConfiguration(string path, string site, string locationSubPath, string server) { return OpenWebConfigurationImpl(WebLevel.Path, null, path, site, locationSubPath, server, null, null, IntPtr.Zero); } public static Configuration OpenWebConfiguration(string path, string site, string locationSubPath, string server, string userName, string password) { return OpenWebConfigurationImpl(WebLevel.Path, null, path, site, locationSubPath, server, userName, password, IntPtr.Zero); } [SecurityPermission(SecurityAction.Demand, Unrestricted = true)] public static Configuration OpenWebConfiguration(string path, string site, string locationSubPath, string server, IntPtr userToken) { return OpenWebConfigurationImpl(WebLevel.Path, null, path, site, locationSubPath, server, null, null, userToken); } public static Configuration OpenMappedWebConfiguration(WebConfigurationFileMap fileMap, string path) { return OpenWebConfigurationImpl(WebLevel.Path, fileMap, path, null, null, null, null, null, IntPtr.Zero); } public static Configuration OpenMappedWebConfiguration(WebConfigurationFileMap fileMap, string path, string site) { return OpenWebConfigurationImpl(WebLevel.Path, fileMap, path, site, null, null, null, null, IntPtr.Zero); } public static Configuration OpenMappedWebConfiguration(WebConfigurationFileMap fileMap, string path, string site, string locationSubPath) { return OpenWebConfigurationImpl(WebLevel.Path, fileMap, path, site, locationSubPath, null, null, null, IntPtr.Zero); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Collections; using System.Collections.Specialized; using System.Configuration; using System.Security; using System.Security.Permissions; using System.Web.Hosting; [AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal)] public static class WebConfigurationManager { public static NameValueCollection AppSettings { get { return ConfigurationManager.AppSettings; } } public static ConnectionStringSettingsCollection ConnectionStrings { get { return ConfigurationManager.ConnectionStrings; } } public static object GetSection(string sectionName) { if (HttpConfigurationSystem.UseHttpConfigurationSystem) { return HttpConfigurationSystem.GetSection(sectionName); } else { return ConfigurationManager.GetSection(sectionName); } } public static object GetSection(string sectionName, string path) { if (HttpConfigurationSystem.UseHttpConfigurationSystem) { return HttpConfigurationSystem.GetSection(sectionName, path); } else { throw new InvalidOperationException(SR.GetString(SR.Config_GetSectionWithPathArgInvalid)); } } public static object GetWebApplicationSection(string sectionName) { if (HttpConfigurationSystem.UseHttpConfigurationSystem) { return HttpConfigurationSystem.GetApplicationSection(sectionName); } else { return ConfigurationManager.GetSection(sectionName); } } // // ************************************************* // ** Static Management Functions to edit config ** // ************************************************* // private static Configuration OpenWebConfigurationImpl( WebLevel webLevel, ConfigurationFileMap fileMap, string path, string site, string locationSubPath, string server, string userName, string password, IntPtr userToken) { // In the hosted case, we allow app relative (~/....). Otherwise, it must be absolute VirtualPath virtualPath; if (HostingEnvironment.IsHosted) { virtualPath = VirtualPath.CreateNonRelativeAllowNull(path); } else { virtualPath = VirtualPath.CreateAbsoluteAllowNull(path); } return WebConfigurationHost.OpenConfiguration(webLevel, fileMap, virtualPath, site, locationSubPath, server, userName, password, userToken); } // // API extra notes: // // OpenWebConfiguration(null) // - Open root web.config // // OpenWebConfiguration("/fxtest"); // - If calling from a hosted app (e.g. an ASP.NET page), it will open web.config at // virtual path "/fxtest" in the current running application (which gives the site) // - If calling from a non-hosted app (e.g. console app), it will do the same thing // except it will use the default web site. // // OpenWebConfiguration("/", "1", "fxtest") // - Open web.config at the root of site "1" and get the config that applies to location "fxtest" // // // OpenMachineConfiguration // public static Configuration OpenMachineConfiguration() { return OpenWebConfigurationImpl(WebLevel.Machine, null, null, null, null, null, null, null, IntPtr.Zero); } public static Configuration OpenMachineConfiguration(string locationSubPath) { return OpenWebConfigurationImpl(WebLevel.Machine, null, null, null, locationSubPath, null, null, null, IntPtr.Zero); } public static Configuration OpenMachineConfiguration(string locationSubPath, string server) { return OpenWebConfigurationImpl(WebLevel.Machine, null, null, null, locationSubPath, server, null, null, IntPtr.Zero); } public static Configuration OpenMachineConfiguration(string locationSubPath, string server, string userName, string password) { return OpenWebConfigurationImpl(WebLevel.Machine, null, null, null, locationSubPath, server, userName, password, IntPtr.Zero); } [SecurityPermission(SecurityAction.Demand, Unrestricted = true)] public static Configuration OpenMachineConfiguration(string locationSubPath, string server, IntPtr userToken) { return OpenWebConfigurationImpl(WebLevel.Machine, null, null, null, locationSubPath, server, null, null, userToken); } public static Configuration OpenMappedMachineConfiguration(ConfigurationFileMap fileMap) { return OpenWebConfigurationImpl(WebLevel.Machine, fileMap, null, null, null, null, null, null, IntPtr.Zero); } public static Configuration OpenMappedMachineConfiguration(ConfigurationFileMap fileMap, string locationSubPath) { return OpenWebConfigurationImpl(WebLevel.Machine, fileMap, null, null, locationSubPath, null, null, null, IntPtr.Zero); } // // OpenWebConfiguration // public static Configuration OpenWebConfiguration(string path) { return OpenWebConfigurationImpl(WebLevel.Path, null, path, null, null, null, null, null, IntPtr.Zero); } public static Configuration OpenWebConfiguration(string path, string site) { return OpenWebConfigurationImpl(WebLevel.Path, null, path, site, null, null, null, null, IntPtr.Zero); } public static Configuration OpenWebConfiguration(string path, string site, string locationSubPath) { return OpenWebConfigurationImpl(WebLevel.Path, null, path, site, locationSubPath, null, null, null, IntPtr.Zero); } public static Configuration OpenWebConfiguration(string path, string site, string locationSubPath, string server) { return OpenWebConfigurationImpl(WebLevel.Path, null, path, site, locationSubPath, server, null, null, IntPtr.Zero); } public static Configuration OpenWebConfiguration(string path, string site, string locationSubPath, string server, string userName, string password) { return OpenWebConfigurationImpl(WebLevel.Path, null, path, site, locationSubPath, server, userName, password, IntPtr.Zero); } [SecurityPermission(SecurityAction.Demand, Unrestricted = true)] public static Configuration OpenWebConfiguration(string path, string site, string locationSubPath, string server, IntPtr userToken) { return OpenWebConfigurationImpl(WebLevel.Path, null, path, site, locationSubPath, server, null, null, userToken); } public static Configuration OpenMappedWebConfiguration(WebConfigurationFileMap fileMap, string path) { return OpenWebConfigurationImpl(WebLevel.Path, fileMap, path, null, null, null, null, null, IntPtr.Zero); } public static Configuration OpenMappedWebConfiguration(WebConfigurationFileMap fileMap, string path, string site) { return OpenWebConfigurationImpl(WebLevel.Path, fileMap, path, site, null, null, null, null, IntPtr.Zero); } public static Configuration OpenMappedWebConfiguration(WebConfigurationFileMap fileMap, string path, string site, string locationSubPath) { return OpenWebConfigurationImpl(WebLevel.Path, fileMap, path, site, locationSubPath, null, null, null, IntPtr.Zero); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
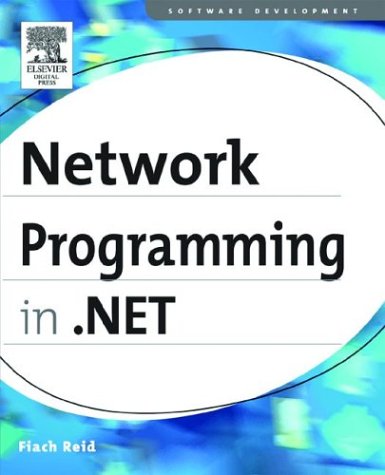
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MailWriter.cs
- SynchronizationContext.cs
- VSWCFServiceContractGenerator.cs
- Main.cs
- TrustSection.cs
- BasicExpandProvider.cs
- CodeParameterDeclarationExpression.cs
- KeyEvent.cs
- MetadataUtil.cs
- ValidationSummaryDesigner.cs
- WindowProviderWrapper.cs
- MenuItemCollection.cs
- hresults.cs
- IdnMapping.cs
- Deserializer.cs
- SupportsEventValidationAttribute.cs
- TypeRefElement.cs
- DetailsViewInsertEventArgs.cs
- SmtpClient.cs
- ReadContentAsBinaryHelper.cs
- FamilyTypeface.cs
- SecurityTokenException.cs
- BamlStream.cs
- ObjectHelper.cs
- StructuralType.cs
- InputQueue.cs
- PseudoWebRequest.cs
- SpecialFolderEnumConverter.cs
- CodeComment.cs
- ConstantProjectedSlot.cs
- IgnoreFileBuildProvider.cs
- XmlMembersMapping.cs
- TypefaceMetricsCache.cs
- DataGridViewRowPostPaintEventArgs.cs
- Pkcs7Signer.cs
- PatternMatcher.cs
- _FtpDataStream.cs
- AutomationPropertyChangedEventArgs.cs
- ParentQuery.cs
- _RequestCacheProtocol.cs
- ListControl.cs
- AuthenticationService.cs
- CodeCatchClause.cs
- TableMethodGenerator.cs
- LabelDesigner.cs
- GetPageCompletedEventArgs.cs
- ImmutableObjectAttribute.cs
- SkinBuilder.cs
- ProfileEventArgs.cs
- WebPartDisplayMode.cs
- RegexCaptureCollection.cs
- SQLMoney.cs
- UInt64.cs
- RequestContext.cs
- _SslSessionsCache.cs
- sqlmetadatafactory.cs
- BaseAppDomainProtocolHandler.cs
- DeclaredTypeValidatorAttribute.cs
- FrameworkPropertyMetadata.cs
- ThemeDictionaryExtension.cs
- CrossSiteScriptingValidation.cs
- ToolStripDropDownButton.cs
- Emitter.cs
- GetCardDetailsRequest.cs
- AdjustableArrowCap.cs
- SafeNativeMethods.cs
- StateWorkerRequest.cs
- ReleaseInstanceMode.cs
- ControlCachePolicy.cs
- DataSetMappper.cs
- MD5CryptoServiceProvider.cs
- PartManifestEntry.cs
- HttpHostedTransportConfiguration.cs
- DetailsViewUpdatedEventArgs.cs
- HandleCollector.cs
- ListQueryResults.cs
- CreateParams.cs
- DbConnectionStringCommon.cs
- URI.cs
- SoapTypeAttribute.cs
- ApplicationFileParser.cs
- TogglePattern.cs
- NodeFunctions.cs
- PageBuildProvider.cs
- Profiler.cs
- FloatMinMaxAggregationOperator.cs
- SelectorAutomationPeer.cs
- TracingConnection.cs
- XslCompiledTransform.cs
- TemplateControlBuildProvider.cs
- ObjectStorage.cs
- MenuItemCollectionEditor.cs
- ImportStoreException.cs
- OpCopier.cs
- CheckBoxRenderer.cs
- PersonalizationStateInfo.cs
- TextClipboardData.cs
- ApplicationDirectory.cs
- Color.cs
- Selection.cs