Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Regex / System / Text / RegularExpressions / RegexCaptureCollection.cs / 1305376 / RegexCaptureCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- // The CaptureCollection lists the captured Capture numbers // contained in a compiled Regex. namespace System.Text.RegularExpressions { using System.Collections; /* * This collection returns the Captures for a group * in the order in which they were matched (left to right * or right to left). It is created by Group.Captures */ ////// #if !SILVERLIGHT [ Serializable() ] #endif public class CaptureCollection : ICollection { internal Group _group; internal int _capcount; internal Capture[] _captures; /* * Nonpublic constructor */ internal CaptureCollection(Group group) { _group = group; _capcount = _group._capcount; } /* * The object on which to synchronize */ ////// Represents a sequence of capture substrings. The object is used /// to return the set of captures done by a single capturing group. /// ////// public Object SyncRoot { get { return _group; } } /* * ICollection */ ///[To be supplied.] ////// public bool IsSynchronized { get { return false; } } /* * ICollection */ ///[To be supplied.] ////// public bool IsReadOnly { get { return true; } } /* * The number of captures for the group */ ///[To be supplied.] ////// public int Count { get { return _capcount; } } /* * The ith capture in the group */ ////// Returns the number of captures. /// ////// public Capture this[int i] { get { return GetCapture(i); } } /* * As required by ICollection */ ////// Provides a means of accessing a specific capture in the collection. /// ////// public void CopyTo(Array array, int arrayIndex) { if (array == null) throw new ArgumentNullException("array"); for (int i = arrayIndex, j = 0; j < Count; i++, j++) { array.SetValue(this[j], i); } } /* * As required by ICollection */ ////// Copies all the elements of the collection to the given array /// beginning at the given index. /// ////// public IEnumerator GetEnumerator() { return new CaptureEnumerator(this); } /* * Nonpublic code to return set of captures for the group */ internal Capture GetCapture(int i) { if (i == _capcount - 1 && i >= 0) return _group; if (i >= _capcount || i < 0) throw new ArgumentOutOfRangeException("i"); // first time a capture is accessed, compute them all if (_captures == null) { _captures = new Capture[_capcount]; for (int j = 0; j < _capcount - 1; j++) { _captures[j] = new Capture(_group._text, _group._caps[j * 2], _group._caps[j * 2 + 1]); } } return _captures[i]; } } /* * This non-public enumerator lists all the captures * Should it be public? */ #if !SILVERLIGHT [ Serializable() ] #endif internal class CaptureEnumerator : IEnumerator { internal CaptureCollection _rcc; internal int _curindex; /* * Nonpublic constructor */ internal CaptureEnumerator(CaptureCollection rcc) { _curindex = -1; _rcc = rcc; } /* * As required by IEnumerator */ public bool MoveNext() { int size = _rcc.Count; if (_curindex >= size) return false; _curindex++; return(_curindex < size); } /* * As required by IEnumerator */ public Object Current { get { return Capture;} } /* * Returns the current capture */ public Capture Capture { get { if (_curindex < 0 || _curindex >= _rcc.Count) throw new InvalidOperationException(SR.GetString(SR.EnumNotStarted)); return _rcc[_curindex]; } } /* * Reset to before the first item */ public void Reset() { _curindex = -1; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Provides an enumerator in the same order as Item[]. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- // The CaptureCollection lists the captured Capture numbers // contained in a compiled Regex. namespace System.Text.RegularExpressions { using System.Collections; /* * This collection returns the Captures for a group * in the order in which they were matched (left to right * or right to left). It is created by Group.Captures */ ////// #if !SILVERLIGHT [ Serializable() ] #endif public class CaptureCollection : ICollection { internal Group _group; internal int _capcount; internal Capture[] _captures; /* * Nonpublic constructor */ internal CaptureCollection(Group group) { _group = group; _capcount = _group._capcount; } /* * The object on which to synchronize */ ////// Represents a sequence of capture substrings. The object is used /// to return the set of captures done by a single capturing group. /// ////// public Object SyncRoot { get { return _group; } } /* * ICollection */ ///[To be supplied.] ////// public bool IsSynchronized { get { return false; } } /* * ICollection */ ///[To be supplied.] ////// public bool IsReadOnly { get { return true; } } /* * The number of captures for the group */ ///[To be supplied.] ////// public int Count { get { return _capcount; } } /* * The ith capture in the group */ ////// Returns the number of captures. /// ////// public Capture this[int i] { get { return GetCapture(i); } } /* * As required by ICollection */ ////// Provides a means of accessing a specific capture in the collection. /// ////// public void CopyTo(Array array, int arrayIndex) { if (array == null) throw new ArgumentNullException("array"); for (int i = arrayIndex, j = 0; j < Count; i++, j++) { array.SetValue(this[j], i); } } /* * As required by ICollection */ ////// Copies all the elements of the collection to the given array /// beginning at the given index. /// ////// public IEnumerator GetEnumerator() { return new CaptureEnumerator(this); } /* * Nonpublic code to return set of captures for the group */ internal Capture GetCapture(int i) { if (i == _capcount - 1 && i >= 0) return _group; if (i >= _capcount || i < 0) throw new ArgumentOutOfRangeException("i"); // first time a capture is accessed, compute them all if (_captures == null) { _captures = new Capture[_capcount]; for (int j = 0; j < _capcount - 1; j++) { _captures[j] = new Capture(_group._text, _group._caps[j * 2], _group._caps[j * 2 + 1]); } } return _captures[i]; } } /* * This non-public enumerator lists all the captures * Should it be public? */ #if !SILVERLIGHT [ Serializable() ] #endif internal class CaptureEnumerator : IEnumerator { internal CaptureCollection _rcc; internal int _curindex; /* * Nonpublic constructor */ internal CaptureEnumerator(CaptureCollection rcc) { _curindex = -1; _rcc = rcc; } /* * As required by IEnumerator */ public bool MoveNext() { int size = _rcc.Count; if (_curindex >= size) return false; _curindex++; return(_curindex < size); } /* * As required by IEnumerator */ public Object Current { get { return Capture;} } /* * Returns the current capture */ public Capture Capture { get { if (_curindex < 0 || _curindex >= _rcc.Count) throw new InvalidOperationException(SR.GetString(SR.EnumNotStarted)); return _rcc[_curindex]; } } /* * Reset to before the first item */ public void Reset() { _curindex = -1; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Provides an enumerator in the same order as Item[]. /// ///
Link Menu
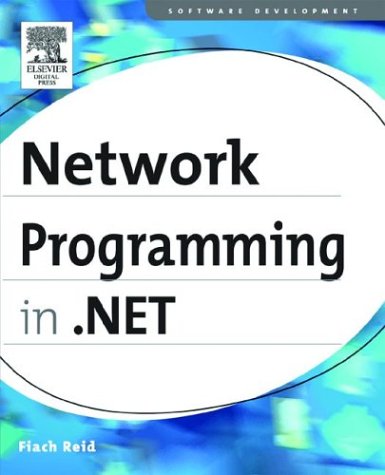
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ContentValidator.cs
- ServiceBusyException.cs
- TimeManager.cs
- KernelTypeValidation.cs
- PerformanceCounterLib.cs
- TrackingStringDictionary.cs
- FixedPosition.cs
- AsyncStreamReader.cs
- XmlUtf8RawTextWriter.cs
- GridLength.cs
- SoapExtensionImporter.cs
- ResourceSetExpression.cs
- OleDbDataReader.cs
- HostingEnvironmentException.cs
- ThemeInfoAttribute.cs
- ControlEvent.cs
- ReadOnlyDictionary.cs
- ObjectListComponentEditor.cs
- SemanticValue.cs
- XamlHostingSection.cs
- DecodeHelper.cs
- ToolStripButton.cs
- HMACMD5.cs
- EnumerableCollectionView.cs
- DropDownList.cs
- SystemIPv6InterfaceProperties.cs
- ProjectionNode.cs
- DelegatingTypeDescriptionProvider.cs
- WindowsStreamSecurityElement.cs
- AnnotationResourceCollection.cs
- RegexCode.cs
- EnumConverter.cs
- References.cs
- SHA256Cng.cs
- ChildTable.cs
- DebugInfoGenerator.cs
- MarkupCompilePass1.cs
- odbcmetadatacolumnnames.cs
- ClientRolePrincipal.cs
- RowCache.cs
- ObjRef.cs
- SynchronizationContext.cs
- ConstraintConverter.cs
- DesignerActionUIService.cs
- XamlLoadErrorInfo.cs
- AssemblyResourceLoader.cs
- ListItemParagraph.cs
- ClientBuildManagerCallback.cs
- Floater.cs
- BitmapInitialize.cs
- ResizingMessageFilter.cs
- SecurityContextTokenValidationException.cs
- BuildProvider.cs
- DrawingContextDrawingContextWalker.cs
- ComMethodElement.cs
- SelectedDatesCollection.cs
- EditorPart.cs
- OrderedDictionaryStateHelper.cs
- InvalidCastException.cs
- MouseCaptureWithinProperty.cs
- HttpCapabilitiesBase.cs
- RadioButtonPopupAdapter.cs
- ImageInfo.cs
- LookupNode.cs
- LineServicesCallbacks.cs
- ReflectionHelper.cs
- StylusDevice.cs
- TTSEvent.cs
- UnsafeNativeMethods.cs
- SettingsPropertyIsReadOnlyException.cs
- EventProxy.cs
- COM2IPerPropertyBrowsingHandler.cs
- NotificationContext.cs
- OracleDataAdapter.cs
- FilteredReadOnlyMetadataCollection.cs
- Journaling.cs
- XmlReaderDelegator.cs
- SafeNativeMethods.cs
- CodePageEncoding.cs
- IntermediatePolicyValidator.cs
- DataKeyArray.cs
- DragStartedEventArgs.cs
- OdbcConnectionOpen.cs
- NetworkCredential.cs
- ImageAttributes.cs
- OdbcUtils.cs
- AnnotationAdorner.cs
- Exception.cs
- SemaphoreFullException.cs
- CookielessHelper.cs
- TextChange.cs
- ObjectParameterCollection.cs
- ServiceDescription.cs
- DoubleCollection.cs
- PageCatalogPart.cs
- AsyncPostBackErrorEventArgs.cs
- ReadOnlyNameValueCollection.cs
- ToolStripControlHost.cs
- safelinkcollection.cs
- ScriptManagerProxy.cs