Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / Structures / ConstantProjectedSlot.cs / 1305376 / ConstantProjectedSlot.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Mapping.ViewGeneration.CqlGeneration; using System.Collections.Generic; using System.Text; using System.Diagnostics; namespace System.Data.Mapping.ViewGeneration.Structures { // A constant that can be projected in a cell query internal class ConstantProjectedSlot : ProjectedSlot { #region Constructors // effects: Creates a slot with constant value being "value" internal ConstantProjectedSlot(Constant value) { Debug.Assert(value != null); m_constant = value; Debug.Assert(value.IsNotNull() == false, "Cannot store NotNull in a slot - NotNull is only for conditions"); } #endregion #region Fields private Constant m_constant; // The actual value #endregion #region Properties // effects: Returns the value stored in this constant internal Constant CellConstant { get { return m_constant; } } #endregion #region ProjectedSlot Members internal override ProjectedSlot MakeAliasedSlot(CqlBlock block, MemberPath memberPath, int slotNum) { return this; // Nothing to create } internal override StringBuilder AsCql(StringBuilder builder, MemberPath outputMember, string blockAlias, int indentLevel) { return m_constant.AsCql(builder, outputMember, blockAlias); } protected override bool IsEqualTo(ProjectedSlot right) { ConstantProjectedSlot rightSlot = right as ConstantProjectedSlot; if (rightSlot == null) { return false; } return Constant.EqualityComparer.Equals(m_constant, rightSlot.m_constant); } protected override int GetHash() { return Constant.EqualityComparer.GetHashCode(m_constant); } #endregion internal override void ToCompactString(StringBuilder builder) { m_constant.ToCompactString(builder); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
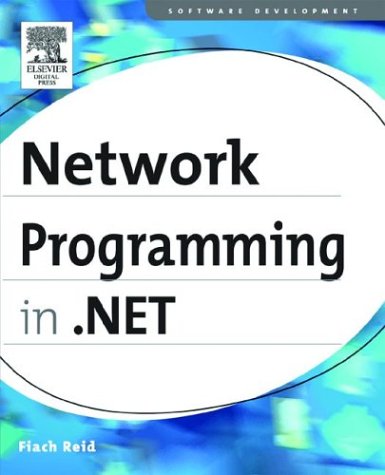
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSortKey.cs
- Duration.cs
- XD.cs
- HtmlUtf8RawTextWriter.cs
- GregorianCalendar.cs
- EditorPart.cs
- Pair.cs
- Control.cs
- AuthenticationConfig.cs
- ServiceNameCollection.cs
- ExecutionEngineException.cs
- AssociationSet.cs
- ControlUtil.cs
- ForAllOperator.cs
- GenericAuthenticationEventArgs.cs
- SQLConvert.cs
- Error.cs
- SHA1Managed.cs
- Schema.cs
- ConnectionStringsExpressionBuilder.cs
- EntityKey.cs
- IsolatedStorage.cs
- DiscoveryProxy.cs
- Group.cs
- XmlSequenceWriter.cs
- DataGridViewRowPrePaintEventArgs.cs
- ForceCopyBuildProvider.cs
- IDQuery.cs
- ConnectionManagementElementCollection.cs
- CommandField.cs
- ContextMenu.cs
- X509CertificateTokenFactoryCredential.cs
- UnmanagedHandle.cs
- CustomGrammar.cs
- SendKeys.cs
- StdValidatorsAndConverters.cs
- VBIdentifierNameEditor.cs
- PersonalizableAttribute.cs
- TypeElement.cs
- NotifyInputEventArgs.cs
- SelectionRangeConverter.cs
- Convert.cs
- ScriptMethodAttribute.cs
- InvalidCommandTreeException.cs
- TreeIterator.cs
- PointAnimationClockResource.cs
- StringReader.cs
- MenuItemCollectionEditor.cs
- ContextMenuStripActionList.cs
- ProxyElement.cs
- storagemappingitemcollection.viewdictionary.cs
- SQLDateTime.cs
- Imaging.cs
- HtmlControlPersistable.cs
- TextCompositionEventArgs.cs
- Maps.cs
- DBNull.cs
- MenuCommands.cs
- SocketPermission.cs
- HostedBindingBehavior.cs
- ResourceDescriptionAttribute.cs
- CollectionDataContract.cs
- ControlAdapter.cs
- ParenthesizePropertyNameAttribute.cs
- _Events.cs
- XamlStream.cs
- SHA512.cs
- CustomAttributeBuilder.cs
- FormsAuthenticationUser.cs
- ConfigurationManager.cs
- HyperlinkAutomationPeer.cs
- ComboBoxItem.cs
- ToolStripDropDown.cs
- WindowsNonControl.cs
- ErrorHandler.cs
- PeerNameRecord.cs
- ManagedWndProcTracker.cs
- DoubleStorage.cs
- NumericPagerField.cs
- WorkflowTimerService.cs
- HGlobalSafeHandle.cs
- FirstMatchCodeGroup.cs
- AuthorizationBehavior.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- PersonalizationEntry.cs
- DrawingAttributes.cs
- MeasureData.cs
- ModelPropertyDescriptor.cs
- HttpAsyncResult.cs
- Propagator.Evaluator.cs
- FieldToken.cs
- ProcessHostConfigUtils.cs
- Unit.cs
- DataDocumentXPathNavigator.cs
- DataViewManager.cs
- RegexBoyerMoore.cs
- Models.cs
- GridViewRowPresenter.cs
- TypeLoadException.cs
- SqlCommandBuilder.cs