Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / PrimitiveType.cs / 2 / PrimitiveType.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Collections.ObjectModel; using System.Data.Common; using System.Data.Common.Utils; using System.Diagnostics; using System.Linq; namespace System.Data.Metadata.Edm { ////// Class representing a primitive type /// public sealed class PrimitiveType : SimpleType { #region constructors ////// Initializes a new instance of PrimitiveType /// internal PrimitiveType() { // No initialization of item attributes in here, it's used as a pass thru in the case for delay population // of item attributes } ////// The constructor for PrimitiveType. It takes the required information to identify this type. /// /// The name of this type /// The namespace name of this type /// The version of this type /// dataSpace in which this primitive type belongs to /// The primitive type that this type is derived from /// The ProviderManifest of the provider of this type ///Thrown if name, namespaceName, version, baseType or providerManifest arguments are null internal PrimitiveType(string name, string namespaceName, DataSpace dataSpace, PrimitiveType baseType, DbProviderManifest providerManifest) : base(name, namespaceName, dataSpace) { EntityUtil.GenericCheckArgumentNull(baseType, "baseType"); EntityUtil.GenericCheckArgumentNull(providerManifest, "providerManifest"); this.BaseType = baseType; Initialize(this, baseType.PrimitiveTypeKind, false, // isDefault providerManifest); } ////// The constructor for PrimitiveType, it takes in a CLR type containing the identity information /// /// The CLR type object for this primitive type /// The base type for this primitive type /// The ProviderManifest of the provider of this type internal PrimitiveType(Type clrType, PrimitiveType baseType, DbProviderManifest providerManifest) : this(EntityUtil.GenericCheckArgumentNull(clrType, "clrType").Name, clrType.Namespace, DataSpace.OSpace, baseType, providerManifest) { Debug.Assert(clrType == ClrEquivalentType, "not equivalent to ClrEquivalentType"); } #endregion #region Fields private PrimitiveTypeKind _primitiveTypeKind; private DbProviderManifest _providerManifest; #endregion #region Properties ////// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.PrimitiveType; } } ////// internal override System.Type ClrType { get { return ClrEquivalentType; } } ////// Returns the PrimitiveTypeKind enumeration value indicating what kind of primitive type this is /// ///A PrimitiveTypeKind value [MetadataProperty(BuiltInTypeKind.PrimitiveTypeKind, false)] public PrimitiveTypeKind PrimitiveTypeKind { get { return _primitiveTypeKind; } internal set { _primitiveTypeKind = value; } } ////// Returns the ProviderManifest giving access to the Manifest that this type came from /// ///The types ProviderManifest value internal DbProviderManifest ProviderManifest { get { Debug.Assert(_providerManifest != null, "This primitive type should have been added to a manifest, which should have set this"); return _providerManifest; } set { Debug.Assert(value != null, "This primitive type should have been added to a manifest, which should have set this"); _providerManifest = value; } } ////// Gets the FacetDescriptions for this type /// ///The FacetDescritions for this type. public System.Collections.ObjectModel.ReadOnlyCollectionFacetDescriptions { get { return ProviderManifest.GetFacetDescriptions(this); } } /// /// Returns an equivalent CLR type representing this primitive type /// public Type ClrEquivalentType { get { switch (PrimitiveTypeKind) { case PrimitiveTypeKind.Binary: return typeof(byte[]); case PrimitiveTypeKind.Boolean: return typeof(bool); case PrimitiveTypeKind.Byte: return typeof(byte); case PrimitiveTypeKind.DateTime: return typeof(DateTime); case PrimitiveTypeKind.Time: return typeof(TimeSpan); case PrimitiveTypeKind.DateTimeOffset: return typeof(DateTimeOffset); case PrimitiveTypeKind.Decimal: return typeof(decimal); case PrimitiveTypeKind.Double: return typeof(double); case PrimitiveTypeKind.Guid: return typeof(Guid); case PrimitiveTypeKind.Single: return typeof(Single); case PrimitiveTypeKind.SByte: return typeof(sbyte); case PrimitiveTypeKind.Int16: return typeof(short); case PrimitiveTypeKind.Int32: return typeof(int); case PrimitiveTypeKind.Int64: return typeof(long); case PrimitiveTypeKind.String: return typeof(string); } return null; } } #endregion #region Methods internal override IEnumerableGetAssociatedFacetDescriptions() { // return all general facets and facets associated with this type return base.GetAssociatedFacetDescriptions().Concat(this.FacetDescriptions); } /// /// Perform initialization that's common across all constructors /// /// The primitive type to initialize /// The primitive type kind of this primitive type /// When true this is the default type to return when a type is asked for by PrimitiveTypeKind /// The ProviderManifest of the provider of this type internal static void Initialize(PrimitiveType primitiveType, PrimitiveTypeKind primitiveTypeKind, bool isDefaultType, DbProviderManifest providerManifest) { primitiveType._primitiveTypeKind = primitiveTypeKind; primitiveType._providerManifest = providerManifest; } ////// return the model equivalent type for this type, /// for example if this instance is nvarchar and it's /// base type is Edm String then the return type is Edm String. /// If the type is actually already a model type then the /// return type is "this". /// ///[System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public EdmType GetEdmPrimitiveType() { return MetadataItem.EdmProviderManifest.GetPrimitiveType(PrimitiveTypeKind); } /// /// Returns the list of EDM primitive types /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public static System.Collections.ObjectModel.ReadOnlyCollectionGetEdmPrimitiveTypes() { return EdmProviderManifest.GetStoreTypes(); } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public static PrimitiveType GetEdmPrimitiveType(PrimitiveTypeKind primitiveTypeKind) { return MetadataItem.EdmProviderManifest.GetPrimitiveType(primitiveTypeKind); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Collections.ObjectModel; using System.Data.Common; using System.Data.Common.Utils; using System.Diagnostics; using System.Linq; namespace System.Data.Metadata.Edm { ////// Class representing a primitive type /// public sealed class PrimitiveType : SimpleType { #region constructors ////// Initializes a new instance of PrimitiveType /// internal PrimitiveType() { // No initialization of item attributes in here, it's used as a pass thru in the case for delay population // of item attributes } ////// The constructor for PrimitiveType. It takes the required information to identify this type. /// /// The name of this type /// The namespace name of this type /// The version of this type /// dataSpace in which this primitive type belongs to /// The primitive type that this type is derived from /// The ProviderManifest of the provider of this type ///Thrown if name, namespaceName, version, baseType or providerManifest arguments are null internal PrimitiveType(string name, string namespaceName, DataSpace dataSpace, PrimitiveType baseType, DbProviderManifest providerManifest) : base(name, namespaceName, dataSpace) { EntityUtil.GenericCheckArgumentNull(baseType, "baseType"); EntityUtil.GenericCheckArgumentNull(providerManifest, "providerManifest"); this.BaseType = baseType; Initialize(this, baseType.PrimitiveTypeKind, false, // isDefault providerManifest); } ////// The constructor for PrimitiveType, it takes in a CLR type containing the identity information /// /// The CLR type object for this primitive type /// The base type for this primitive type /// The ProviderManifest of the provider of this type internal PrimitiveType(Type clrType, PrimitiveType baseType, DbProviderManifest providerManifest) : this(EntityUtil.GenericCheckArgumentNull(clrType, "clrType").Name, clrType.Namespace, DataSpace.OSpace, baseType, providerManifest) { Debug.Assert(clrType == ClrEquivalentType, "not equivalent to ClrEquivalentType"); } #endregion #region Fields private PrimitiveTypeKind _primitiveTypeKind; private DbProviderManifest _providerManifest; #endregion #region Properties ////// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.PrimitiveType; } } ////// internal override System.Type ClrType { get { return ClrEquivalentType; } } ////// Returns the PrimitiveTypeKind enumeration value indicating what kind of primitive type this is /// ///A PrimitiveTypeKind value [MetadataProperty(BuiltInTypeKind.PrimitiveTypeKind, false)] public PrimitiveTypeKind PrimitiveTypeKind { get { return _primitiveTypeKind; } internal set { _primitiveTypeKind = value; } } ////// Returns the ProviderManifest giving access to the Manifest that this type came from /// ///The types ProviderManifest value internal DbProviderManifest ProviderManifest { get { Debug.Assert(_providerManifest != null, "This primitive type should have been added to a manifest, which should have set this"); return _providerManifest; } set { Debug.Assert(value != null, "This primitive type should have been added to a manifest, which should have set this"); _providerManifest = value; } } ////// Gets the FacetDescriptions for this type /// ///The FacetDescritions for this type. public System.Collections.ObjectModel.ReadOnlyCollectionFacetDescriptions { get { return ProviderManifest.GetFacetDescriptions(this); } } /// /// Returns an equivalent CLR type representing this primitive type /// public Type ClrEquivalentType { get { switch (PrimitiveTypeKind) { case PrimitiveTypeKind.Binary: return typeof(byte[]); case PrimitiveTypeKind.Boolean: return typeof(bool); case PrimitiveTypeKind.Byte: return typeof(byte); case PrimitiveTypeKind.DateTime: return typeof(DateTime); case PrimitiveTypeKind.Time: return typeof(TimeSpan); case PrimitiveTypeKind.DateTimeOffset: return typeof(DateTimeOffset); case PrimitiveTypeKind.Decimal: return typeof(decimal); case PrimitiveTypeKind.Double: return typeof(double); case PrimitiveTypeKind.Guid: return typeof(Guid); case PrimitiveTypeKind.Single: return typeof(Single); case PrimitiveTypeKind.SByte: return typeof(sbyte); case PrimitiveTypeKind.Int16: return typeof(short); case PrimitiveTypeKind.Int32: return typeof(int); case PrimitiveTypeKind.Int64: return typeof(long); case PrimitiveTypeKind.String: return typeof(string); } return null; } } #endregion #region Methods internal override IEnumerableGetAssociatedFacetDescriptions() { // return all general facets and facets associated with this type return base.GetAssociatedFacetDescriptions().Concat(this.FacetDescriptions); } /// /// Perform initialization that's common across all constructors /// /// The primitive type to initialize /// The primitive type kind of this primitive type /// When true this is the default type to return when a type is asked for by PrimitiveTypeKind /// The ProviderManifest of the provider of this type internal static void Initialize(PrimitiveType primitiveType, PrimitiveTypeKind primitiveTypeKind, bool isDefaultType, DbProviderManifest providerManifest) { primitiveType._primitiveTypeKind = primitiveTypeKind; primitiveType._providerManifest = providerManifest; } ////// return the model equivalent type for this type, /// for example if this instance is nvarchar and it's /// base type is Edm String then the return type is Edm String. /// If the type is actually already a model type then the /// return type is "this". /// ///[System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public EdmType GetEdmPrimitiveType() { return MetadataItem.EdmProviderManifest.GetPrimitiveType(PrimitiveTypeKind); } /// /// Returns the list of EDM primitive types /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public static System.Collections.ObjectModel.ReadOnlyCollectionGetEdmPrimitiveTypes() { return EdmProviderManifest.GetStoreTypes(); } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public static PrimitiveType GetEdmPrimitiveType(PrimitiveTypeKind primitiveTypeKind) { return MetadataItem.EdmProviderManifest.GetPrimitiveType(primitiveTypeKind); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
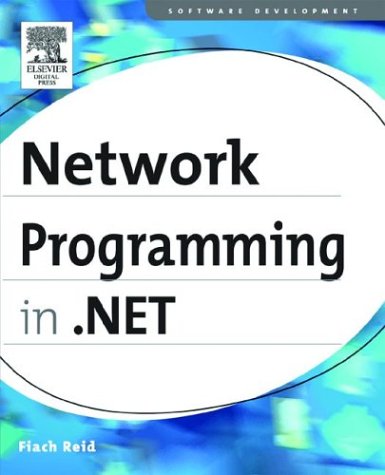
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Cell.cs
- DesignerHelpers.cs
- SoapElementAttribute.cs
- LoadMessageLogger.cs
- TextEncodedRawTextWriter.cs
- TextShapeableCharacters.cs
- FactoryGenerator.cs
- DataGridViewHitTestInfo.cs
- AsyncDataRequest.cs
- ExpandSegmentCollection.cs
- TabControlEvent.cs
- ReferenceConverter.cs
- AmbientProperties.cs
- TreeNodeConverter.cs
- MessageDecoder.cs
- AuthorizationRule.cs
- ReverseComparer.cs
- MultiByteCodec.cs
- ReflectTypeDescriptionProvider.cs
- SmiXetterAccessMap.cs
- RoleGroupCollection.cs
- Path.cs
- LingerOption.cs
- Tablet.cs
- PropertyGeneratedEventArgs.cs
- ForeignKeyConstraint.cs
- ZipIOLocalFileHeader.cs
- TrailingSpaceComparer.cs
- NavigationProperty.cs
- ColumnHeaderConverter.cs
- XmlConvert.cs
- OleDbReferenceCollection.cs
- DBAsyncResult.cs
- FontUnit.cs
- LinearGradientBrush.cs
- CodeConstructor.cs
- InputLanguageProfileNotifySink.cs
- StoreItemCollection.Loader.cs
- SetUserPreferenceRequest.cs
- ThemeDirectoryCompiler.cs
- TextEndOfLine.cs
- COM2IDispatchConverter.cs
- CodeCompiler.cs
- InvalidAsynchronousStateException.cs
- Mapping.cs
- XmlWriter.cs
- RenderingEventArgs.cs
- OrderedParallelQuery.cs
- TextElementCollection.cs
- WebControlsSection.cs
- TimeStampChecker.cs
- M3DUtil.cs
- control.ime.cs
- ValidatedControlConverter.cs
- ComponentRenameEvent.cs
- WizardForm.cs
- Descriptor.cs
- TransformGroup.cs
- BatchStream.cs
- TouchDevice.cs
- PartManifestEntry.cs
- TableRowsCollectionEditor.cs
- UserControlParser.cs
- StreamWriter.cs
- DataGridColumnReorderingEventArgs.cs
- AutomationEvent.cs
- ChildrenQuery.cs
- IdentifierCreationService.cs
- XmlSchemaObjectTable.cs
- glyphs.cs
- BatchParser.cs
- XmlExpressionDumper.cs
- SelectionPattern.cs
- PersonalizationEntry.cs
- SmtpSection.cs
- dsa.cs
- FormViewRow.cs
- StatusBarItemAutomationPeer.cs
- ToolStripSplitStackLayout.cs
- ParameterCollectionEditorForm.cs
- QilIterator.cs
- XmlDownloadManager.cs
- CodeSubDirectory.cs
- UpdatePanelTriggerCollection.cs
- BitmapCodecInfo.cs
- IntSumAggregationOperator.cs
- GridViewColumnCollectionChangedEventArgs.cs
- precedingsibling.cs
- RectValueSerializer.cs
- TextWriter.cs
- TransformerInfo.cs
- StateManagedCollection.cs
- SubMenuStyle.cs
- HwndKeyboardInputProvider.cs
- CompiledRegexRunner.cs
- Propagator.JoinPropagator.cs
- WindowsStatic.cs
- DependencyPropertyKey.cs
- DotNetATv1WindowsLogEntrySerializer.cs
- EntityObject.cs