Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Data / System / Data / Common / DBAsyncResult.cs / 1305376 / DBAsyncResult.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.Common { using System; using System.Data.ProviderBase; using System.Diagnostics; using System.Threading; internal sealed class DbAsyncResult : IAsyncResult { private readonly AsyncCallback _callback = null; private bool _fCompleted = false; private bool _fCompletedSynchronously = false; private readonly ManualResetEvent _manualResetEvent = null; private object _owner = null; private readonly object _stateObject = null; private readonly string _endMethodName; private ExecutionContext _execContext = null; static private ContextCallback _contextCallback = new ContextCallback(AsyncCallback_Context); // Used for SqlClient Open async private DbConnectionInternal _connectionInternal = null; internal DbAsyncResult(object owner, string endMethodName, AsyncCallback callback, object stateObject, ExecutionContext execContext) { _owner = owner; _endMethodName = endMethodName; _callback = callback; _stateObject = stateObject; _manualResetEvent = new ManualResetEvent(false); _execContext = execContext; } object IAsyncResult.AsyncState { get { return _stateObject; } } WaitHandle IAsyncResult.AsyncWaitHandle { get { return _manualResetEvent; } } bool IAsyncResult.CompletedSynchronously { get { return _fCompletedSynchronously; } } internal DbConnectionInternal ConnectionInternal { get { return _connectionInternal; } set { _connectionInternal = value; } } bool IAsyncResult.IsCompleted { get { return _fCompleted; } } internal string EndMethodName { get { return _endMethodName; } } internal void CompareExchangeOwner(object owner, string method) { object prior = Interlocked.CompareExchange(ref _owner, null, owner); if (prior != owner) { if (null != prior) { throw ADP.IncorrectAsyncResult(); } throw ADP.MethodCalledTwice(method); } } internal void Reset() { _fCompleted = false; _fCompletedSynchronously = false; _manualResetEvent.Reset(); } internal void SetCompleted() { _fCompleted = true; _manualResetEvent.Set(); if (_callback != null) { // QueueUserWorkItem only accepts WaitCallback - which requires a signature of Foo(object state). // Must call function on this object with that signature - and then call user AsyncCallback. // AsyncCallback signature is Foo(IAsyncResult result). ThreadPool.QueueUserWorkItem(new WaitCallback(ExecuteCallback), this); } } internal void SetCompletedSynchronously() { _fCompletedSynchronously = true; } static private void AsyncCallback_Context(Object state) { DbAsyncResult result = (DbAsyncResult) state; if (result._callback != null) { result._callback(result); } } private void ExecuteCallback(object asyncResult) { DbAsyncResult result = (DbAsyncResult) asyncResult; if (null != result._callback) { if (result._execContext != null) { ExecutionContext.Run(result._execContext, DbAsyncResult._contextCallback, result); } else { result._callback(this); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
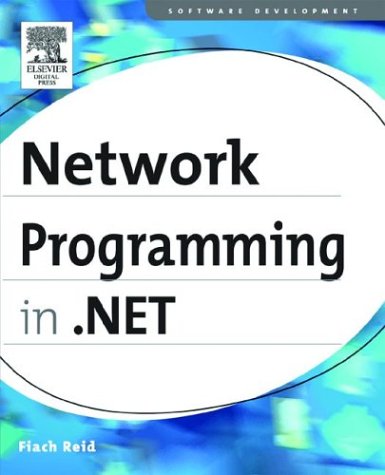
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartManagerDesigner.cs
- RemoveStoryboard.cs
- Ops.cs
- SharedRuntimeState.cs
- PageRanges.cs
- Compress.cs
- ListControlDataBindingHandler.cs
- WebContext.cs
- EventProviderWriter.cs
- ItemCheckEvent.cs
- QilInvoke.cs
- XmlMemberMapping.cs
- BaseCollection.cs
- FixedSOMPageConstructor.cs
- DocumentViewerAutomationPeer.cs
- Int32EqualityComparer.cs
- OLEDB_Enum.cs
- AddToCollection.cs
- ThreadPool.cs
- AppModelKnownContentFactory.cs
- UnaryNode.cs
- CompositeFontFamily.cs
- PickBranchDesigner.xaml.cs
- CFStream.cs
- DecoratedNameAttribute.cs
- CompositeControlDesigner.cs
- TableNameAttribute.cs
- ObjRef.cs
- XhtmlStyleClass.cs
- AbstractDataSvcMapFileLoader.cs
- SortableBindingList.cs
- SHA1CryptoServiceProvider.cs
- SqlBooleanMismatchVisitor.cs
- ScrollItemProviderWrapper.cs
- ZoneLinkButton.cs
- CodeValidator.cs
- SharedStatics.cs
- HttpModule.cs
- CharAnimationUsingKeyFrames.cs
- SessionPageStateSection.cs
- precedingquery.cs
- SQLInt64Storage.cs
- GZipStream.cs
- xml.cs
- XmlReflectionMember.cs
- XmlSequenceWriter.cs
- Separator.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- MergeFailedEvent.cs
- CurrentChangingEventManager.cs
- HashCodeCombiner.cs
- CompositeActivityTypeDescriptorProvider.cs
- HttpException.cs
- EarlyBoundInfo.cs
- IResourceProvider.cs
- LambdaCompiler.Expressions.cs
- JoinElimination.cs
- ActivityDesigner.cs
- RegexReplacement.cs
- AuthenticationService.cs
- QueueTransferProtocol.cs
- MultipleFilterMatchesException.cs
- DataGridViewRowsRemovedEventArgs.cs
- DataGridLength.cs
- ButtonBaseAutomationPeer.cs
- NullableConverter.cs
- ServerIdentity.cs
- _HelperAsyncResults.cs
- basecomparevalidator.cs
- InputManager.cs
- DiagnosticTrace.cs
- CellParaClient.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- SqlCacheDependency.cs
- DataContractSet.cs
- CultureInfo.cs
- JoinGraph.cs
- Baml6ConstructorInfo.cs
- Serializer.cs
- ParsedAttributeCollection.cs
- ConditionalAttribute.cs
- EnvironmentPermission.cs
- ListViewHitTestInfo.cs
- PermissionListSet.cs
- WindowsFormsHostAutomationPeer.cs
- EpmContentSerializerBase.cs
- RequestQueue.cs
- TextSelectionHighlightLayer.cs
- XmlSerializer.cs
- DecimalSumAggregationOperator.cs
- FixedSOMLineRanges.cs
- ReliableDuplexSessionChannel.cs
- EventLogInformation.cs
- NamedPipeTransportManager.cs
- TransactionOptions.cs
- TrackingStringDictionary.cs
- ProgramPublisher.cs
- CompilationRelaxations.cs
- DependencyPropertyHelper.cs
- LayoutEngine.cs