Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / Layout / LayoutEngine.cs / 1 / LayoutEngine.cs
//#define LAYOUT_PERFWATCH //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.Layout { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Drawing; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Security.Permissions; ///public abstract class LayoutEngine { internal IArrangedElement CastToArrangedElement(object obj) { IArrangedElement element = obj as IArrangedElement; if(obj == null) { throw new NotSupportedException(SR.GetString(SR.LayoutEngineUnsupportedType, obj.GetType())); } return element; } internal virtual Size GetPreferredSize(IArrangedElement container, Size proposedConstraints) { return Size.Empty; } /// public virtual void InitLayout(object child, BoundsSpecified specified) { InitLayoutCore(CastToArrangedElement(child), specified); } internal virtual void InitLayoutCore(IArrangedElement element, BoundsSpecified bounds) {} internal virtual void ProcessSuspendedLayoutEventArgs(IArrangedElement container, LayoutEventArgs args) {} #if LAYOUT_PERFWATCH private static int LayoutWatch = 100; #endif /// [SuppressMessage("Microsoft.Security", "CA2109:ReviewVisibleEventHandlers")] public virtual bool Layout(object container, LayoutEventArgs layoutEventArgs) { #if LAYOUT_PERFWATCH Debug.WriteLine(container.GetType().Name + "::Layout(" + (layoutEventArgs.AffectedControl != null ? layoutEventArgs.AffectedControl.Name : "null") + ", " + layoutEventArgs.AffectedProperty + ")"); Debug.Indent(); Stopwatch sw = new Stopwatch(); sw.Start(); #endif bool parentNeedsLayout = LayoutCore(CastToArrangedElement(container), layoutEventArgs); #if LAYOUT_PERFWATCH sw.Stop(); if (sw.ElapsedMilliseconds > LayoutWatch && Debugger.IsAttached) { Debugger.Break(); } Debug.Unindent(); Debug.WriteLine(container.GetType().Name + "::Layout elapsed " + sw.ElapsedMilliseconds.ToString() + " returned: " + parentNeedsLayout); #endif return parentNeedsLayout; } internal virtual bool LayoutCore(IArrangedElement container, LayoutEventArgs layoutEventArgs) { return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //#define LAYOUT_PERFWATCH //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.Layout { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Drawing; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Security.Permissions; ///public abstract class LayoutEngine { internal IArrangedElement CastToArrangedElement(object obj) { IArrangedElement element = obj as IArrangedElement; if(obj == null) { throw new NotSupportedException(SR.GetString(SR.LayoutEngineUnsupportedType, obj.GetType())); } return element; } internal virtual Size GetPreferredSize(IArrangedElement container, Size proposedConstraints) { return Size.Empty; } /// public virtual void InitLayout(object child, BoundsSpecified specified) { InitLayoutCore(CastToArrangedElement(child), specified); } internal virtual void InitLayoutCore(IArrangedElement element, BoundsSpecified bounds) {} internal virtual void ProcessSuspendedLayoutEventArgs(IArrangedElement container, LayoutEventArgs args) {} #if LAYOUT_PERFWATCH private static int LayoutWatch = 100; #endif /// [SuppressMessage("Microsoft.Security", "CA2109:ReviewVisibleEventHandlers")] public virtual bool Layout(object container, LayoutEventArgs layoutEventArgs) { #if LAYOUT_PERFWATCH Debug.WriteLine(container.GetType().Name + "::Layout(" + (layoutEventArgs.AffectedControl != null ? layoutEventArgs.AffectedControl.Name : "null") + ", " + layoutEventArgs.AffectedProperty + ")"); Debug.Indent(); Stopwatch sw = new Stopwatch(); sw.Start(); #endif bool parentNeedsLayout = LayoutCore(CastToArrangedElement(container), layoutEventArgs); #if LAYOUT_PERFWATCH sw.Stop(); if (sw.ElapsedMilliseconds > LayoutWatch && Debugger.IsAttached) { Debugger.Break(); } Debug.Unindent(); Debug.WriteLine(container.GetType().Name + "::Layout elapsed " + sw.ElapsedMilliseconds.ToString() + " returned: " + parentNeedsLayout); #endif return parentNeedsLayout; } internal virtual bool LayoutCore(IArrangedElement container, LayoutEventArgs layoutEventArgs) { return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
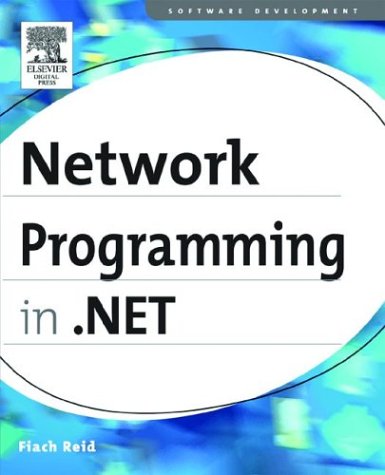
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Rule.cs
- GraphicsContext.cs
- FlowDocumentView.cs
- ErrorEventArgs.cs
- LifetimeServices.cs
- DataSourceControlBuilder.cs
- XmlWrappingReader.cs
- SafeCryptoKeyHandle.cs
- XmlDownloadManager.cs
- GeneralTransform3DGroup.cs
- ButtonField.cs
- ProviderIncompatibleException.cs
- StringOutput.cs
- CopyEncoder.cs
- ContentElement.cs
- XmlNode.cs
- ScaleTransform3D.cs
- DBSqlParserTableCollection.cs
- QilSortKey.cs
- XPathEmptyIterator.cs
- hresults.cs
- TypeUtils.cs
- PropertyIDSet.cs
- __ComObject.cs
- XamlValidatingReader.cs
- HtmlTableRow.cs
- ControlAdapter.cs
- KeyMatchBuilder.cs
- DoubleLink.cs
- CodeCommentStatementCollection.cs
- ConfigurationFileMap.cs
- PropertyFilterAttribute.cs
- ObjectPersistData.cs
- ConfigurationValidatorAttribute.cs
- SystemMulticastIPAddressInformation.cs
- MatrixTransform.cs
- COM2Properties.cs
- DataGridViewElement.cs
- TreeWalker.cs
- Events.cs
- RuntimeComponentFilter.cs
- CollectionBuilder.cs
- XPathMessageContext.cs
- SpeechEvent.cs
- CodeParameterDeclarationExpressionCollection.cs
- XmlDocumentSerializer.cs
- Activation.cs
- XmlSchemaDatatype.cs
- OdbcTransaction.cs
- QilValidationVisitor.cs
- OptionalMessageQuery.cs
- TabItemAutomationPeer.cs
- TextTrailingCharacterEllipsis.cs
- VectorAnimation.cs
- NavigationProgressEventArgs.cs
- DesignerAttribute.cs
- SubtreeProcessor.cs
- BuildProvider.cs
- CopyOnWriteList.cs
- QilScopedVisitor.cs
- BitmapEffectGeneralTransform.cs
- XmlEventCache.cs
- InlineUIContainer.cs
- Wizard.cs
- InvalidAsynchronousStateException.cs
- ProfilePropertyNameValidator.cs
- HelpEvent.cs
- SmiRequestExecutor.cs
- EventMappingSettingsCollection.cs
- ObjectListFieldCollection.cs
- SQLGuid.cs
- SecurityManager.cs
- WSSecurityOneDotOneSendSecurityHeader.cs
- StringInfo.cs
- TextEmbeddedObject.cs
- WindowsHyperlink.cs
- ListBindableAttribute.cs
- OleDbRowUpdatedEvent.cs
- unitconverter.cs
- FileDataSourceCache.cs
- HtmlControlPersistable.cs
- LateBoundBitmapDecoder.cs
- SqlCharStream.cs
- DLinqAssociationProvider.cs
- StickyNote.cs
- TextServicesContext.cs
- ZipIORawDataFileBlock.cs
- TargetPerspective.cs
- SByteStorage.cs
- List.cs
- Pair.cs
- ListSortDescription.cs
- LogReserveAndAppendState.cs
- BindableAttribute.cs
- NetPeerTcpBindingElement.cs
- CollectionMarkupSerializer.cs
- WsdlWriter.cs
- SessionPageStatePersister.cs
- StrokeCollection.cs
- WindowsFont.cs