Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Query / PlanCompiler / TypeUtils.cs / 1305376 / TypeUtils.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; //using System.Diagnostics; // Please use PlanCompiler.Assert instead of Debug.Assert in this class... using System.Globalization; using System.Data.Common; using md = System.Data.Metadata.Edm; // // This module contains a few utility functions that make it easier to operate // with type metadata // namespace System.Data.Query.PlanCompiler { ////// This class is used as a Comparer for Types all through the PlanCompiler. /// It has a pretty strict definition of type equality - which pretty much devolves /// to equality of the "Identity" of the Type (not the TypeUsage). /// /// NOTE: Unlike other parts of the query pipeline, record types follow /// a much stricter equality condition here - the field names must be the same, and /// the field types must be equal. /// /// NOTE: Primitive types are considered equal, if their Identities are equal. This doesn't /// take into account any of the facets that are represented external to the type (size, for instance). /// Again, this is different from other parts of the query pipeline; and we're much stricter here /// /// sealed internal class TypeUsageEqualityComparer : IEqualityComparer{ private TypeUsageEqualityComparer() { } internal static readonly TypeUsageEqualityComparer Instance = new TypeUsageEqualityComparer(); #region IEqualityComparer Members public bool Equals(System.Data.Metadata.Edm.TypeUsage x, System.Data.Metadata.Edm.TypeUsage y) { if (x == null || y == null) { return false; } return TypeUsageEqualityComparer.Equals(x.EdmType, y.EdmType); } public int GetHashCode(System.Data.Metadata.Edm.TypeUsage obj) { return obj.EdmType.Identity.GetHashCode(); } #endregion internal static bool Equals(md.EdmType x, md.EdmType y) { return x.Identity.Equals(y.Identity); } } internal static class TypeUtils { /// /// Is this type a UDT? (ie) a structural type supported by the store /// /// the type in question ///true, if the type was a UDT internal static bool IsUdt(md.TypeUsage type) { return IsUdt(type.EdmType); } ////// Is this type a UDT? (ie) a structural type supported by the store /// /// the type in question ///true, if the type was a UDT internal static bool IsUdt(md.EdmType type) { #if UDT_SUPPORT // Ideally this should be as simple as: // return TypeUsage.HasExtendedAttribute(type, MetadataConstants.UdtAttribute); // The definition below is 'Type is a ComplexType defined in the store'. return (BuiltInTypeKind.ComplexType == type.BuiltInTypeKind && TypeHelpers.HasExtendedAttribute(type, MetadataConstants.TargetAttribute)); #else return false; #endif } ////// Is this a structured type? /// Note: Structured, in this context means structured outside the server. /// UDTs for instance, are considered to be scalar types - all WinFS types, /// would by this argument, be scalar types. /// /// The type to check ///true, if the type is a structured type internal static bool IsStructuredType(md.TypeUsage type) { return (md.TypeSemantics.IsReferenceType(type) || md.TypeSemantics.IsRowType(type) || md.TypeSemantics.IsEntityType(type) || md.TypeSemantics.IsRelationshipType(type) || (md.TypeSemantics.IsComplexType(type) && !IsUdt(type))); } ////// Is this type a collection type? /// /// the current type ///true, if this is a collection type internal static bool IsCollectionType(md.TypeUsage type) { return md.TypeSemantics.IsCollectionType(type); } ////// Create a new collection type based on the supplied element type /// /// element type of the collection ///the new collection type internal static md.TypeUsage CreateCollectionType(md.TypeUsage elementType) { return TypeHelpers.CreateCollectionTypeUsage(elementType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
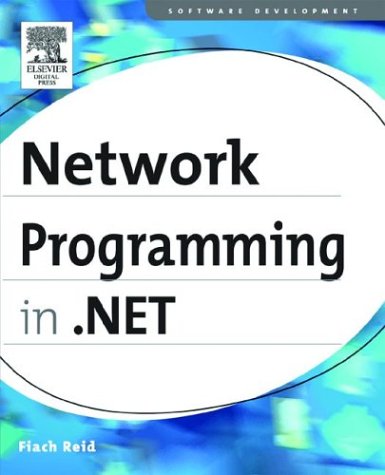
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FileAuthorizationModule.cs
- DataSourceHelper.cs
- EditorPart.cs
- SignatureToken.cs
- AuthorizationSection.cs
- HttpPostClientProtocol.cs
- FeatureAttribute.cs
- ITextView.cs
- CounterNameConverter.cs
- CompilerParameters.cs
- UnauthorizedAccessException.cs
- Icon.cs
- ClientUtils.cs
- DependencyPropertyValueSerializer.cs
- FileChangesMonitor.cs
- NetPeerTcpBinding.cs
- ContainerParagraph.cs
- RevocationPoint.cs
- ZipIOModeEnforcingStream.cs
- PositiveTimeSpanValidator.cs
- OutputCacheProfile.cs
- AdapterDictionary.cs
- CommunicationObjectAbortedException.cs
- TextInfo.cs
- ToolStripItemGlyph.cs
- PasswordTextNavigator.cs
- ParameterCollectionEditorForm.cs
- ContractNamespaceAttribute.cs
- PropertyManager.cs
- MethodBody.cs
- RetrieveVirtualItemEventArgs.cs
- RightNameExpirationInfoPair.cs
- SimpleRecyclingCache.cs
- Transform.cs
- SelectedPathEditor.cs
- GeneratedCodeAttribute.cs
- MemberJoinTreeNode.cs
- ListInitExpression.cs
- EDesignUtil.cs
- IsolatedStorageFilePermission.cs
- ClientConvert.cs
- DataMemberFieldConverter.cs
- HashHelper.cs
- TemplateParser.cs
- WebPartConnectionCollection.cs
- ReflectEventDescriptor.cs
- XamlStyleSerializer.cs
- SkinBuilder.cs
- AuditLogLocation.cs
- PassportPrincipal.cs
- ReadOnlyCollection.cs
- ConvertersCollection.cs
- SqlConnectionManager.cs
- XmlSchemaType.cs
- JsonReader.cs
- ExternalCalls.cs
- columnmapkeybuilder.cs
- PeerNameRecord.cs
- TreeNodeClickEventArgs.cs
- FixUpCollection.cs
- EntityDataSourceValidationException.cs
- TemplateControlBuildProvider.cs
- AssemblyNameProxy.cs
- ComplexBindingPropertiesAttribute.cs
- HandlerBase.cs
- FacetChecker.cs
- IPCCacheManager.cs
- XPathEmptyIterator.cs
- FactoryMaker.cs
- InsufficientMemoryException.cs
- BinaryObjectWriter.cs
- QueryStringParameter.cs
- LogRestartAreaEnumerator.cs
- Pkcs7Recipient.cs
- SoapSchemaExporter.cs
- NetNamedPipeSecurity.cs
- NotSupportedException.cs
- QueueProcessor.cs
- MethodToken.cs
- UpdatePanelTriggerCollection.cs
- GridViewDeletedEventArgs.cs
- CellLabel.cs
- CompiledScopeCriteria.cs
- Animatable.cs
- ParseHttpDate.cs
- WebPartChrome.cs
- SkewTransform.cs
- PortCache.cs
- ThemeInfoAttribute.cs
- DocumentApplicationJournalEntry.cs
- ColorInterpolationModeValidation.cs
- FilteredAttributeCollection.cs
- UnknownBitmapEncoder.cs
- SimpleTypesSurrogate.cs
- EdmValidator.cs
- CodeDOMUtility.cs
- UserNamePasswordValidationMode.cs
- XmlMemberMapping.cs
- Light.cs
- SHA384Managed.cs