Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Documents / InlineUIContainer.cs / 1 / InlineUIContainer.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: InlineUIContainer - a wrapper for embedded UIElements in text // flow content inline collections // //--------------------------------------------------------------------------- using System.ComponentModel; // DesignerSerializationVisibility using System.Windows.Markup; // XamlDesignerSerializationManager using MS.Internal; using MS.Internal.Documents; namespace System.Windows.Documents { ////// InlineUIContainer - a wrapper for embedded UIElements in text /// flow content inline collections /// [ContentProperty("Child")] [TextElementEditingBehaviorAttribute(IsMergeable = false)] public class InlineUIContainer : Inline { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Initializes a new instance of InlineUIContainer element. /// ////// The purpose of this element is to be a wrapper for UIElements /// when they are embedded into text flow - as items of /// InlineCollections. /// public InlineUIContainer() { } ////// Initializes an InlineBox specifying its child UIElement /// /// /// UIElement set as a child of this inline item /// public InlineUIContainer(UIElement childUIElement) : this(childUIElement, null) { } ////// Creates a new InlineUIContainer instance. /// /// /// Optional child of the new InlineUIContainer, may be null. /// /// /// Optional position at which to insert the new InlineUIContainer. May /// be null. /// public InlineUIContainer(UIElement childUIElement, TextPointer insertionPosition) { if (insertionPosition != null) { insertionPosition.TextContainer.BeginChange(); } try { if (insertionPosition != null) { // This will throw InvalidOperationException if schema validity is violated. insertionPosition.InsertInline(this); } this.Child = childUIElement; } finally { if (insertionPosition != null) { insertionPosition.TextContainer.EndChange(); } } } #endregion Constructors //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// The content spanned by this TextElement. /// public UIElement Child { get { return this.ContentStart.GetAdjacentElement(LogicalDirection.Forward) as UIElement; } set { TextContainer textContainer = this.TextContainer; textContainer.BeginChange(); try { TextPointer contentStart = this.ContentStart; UIElement child = Child; if (child != null) { textContainer.DeleteContentInternal(contentStart, this.ContentEnd); ContainerTextElementField.ClearValue(child); } if (value != null) { ContainerTextElementField.SetValue(value, this); contentStart.InsertUIElement(value); } } finally { textContainer.EndChange(); } } } #endregion Public Properties #region Internal Properties ////// UIElementIsland representing embedded Element Layout island within content world. /// internal UIElementIsland UIElementIsland { get { UpdateUIElementIsland(); return _uiElementIsland; } } #endregion Internal Properties #region Private Methods ////// Ensures the _uiElementIsland variable is up to date /// private void UpdateUIElementIsland() { UIElement childElement = this.Child; if(_uiElementIsland == null || _uiElementIsland.Root != childElement) { if(_uiElementIsland != null) { _uiElementIsland.Dispose(); _uiElementIsland = null; } if(childElement != null) { _uiElementIsland = new UIElementIsland(childElement); } } } #endregion Private Methods #region Private Data private UIElementIsland _uiElementIsland; #endregion Private Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: InlineUIContainer - a wrapper for embedded UIElements in text // flow content inline collections // //--------------------------------------------------------------------------- using System.ComponentModel; // DesignerSerializationVisibility using System.Windows.Markup; // XamlDesignerSerializationManager using MS.Internal; using MS.Internal.Documents; namespace System.Windows.Documents { ////// InlineUIContainer - a wrapper for embedded UIElements in text /// flow content inline collections /// [ContentProperty("Child")] [TextElementEditingBehaviorAttribute(IsMergeable = false)] public class InlineUIContainer : Inline { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Initializes a new instance of InlineUIContainer element. /// ////// The purpose of this element is to be a wrapper for UIElements /// when they are embedded into text flow - as items of /// InlineCollections. /// public InlineUIContainer() { } ////// Initializes an InlineBox specifying its child UIElement /// /// /// UIElement set as a child of this inline item /// public InlineUIContainer(UIElement childUIElement) : this(childUIElement, null) { } ////// Creates a new InlineUIContainer instance. /// /// /// Optional child of the new InlineUIContainer, may be null. /// /// /// Optional position at which to insert the new InlineUIContainer. May /// be null. /// public InlineUIContainer(UIElement childUIElement, TextPointer insertionPosition) { if (insertionPosition != null) { insertionPosition.TextContainer.BeginChange(); } try { if (insertionPosition != null) { // This will throw InvalidOperationException if schema validity is violated. insertionPosition.InsertInline(this); } this.Child = childUIElement; } finally { if (insertionPosition != null) { insertionPosition.TextContainer.EndChange(); } } } #endregion Constructors //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// The content spanned by this TextElement. /// public UIElement Child { get { return this.ContentStart.GetAdjacentElement(LogicalDirection.Forward) as UIElement; } set { TextContainer textContainer = this.TextContainer; textContainer.BeginChange(); try { TextPointer contentStart = this.ContentStart; UIElement child = Child; if (child != null) { textContainer.DeleteContentInternal(contentStart, this.ContentEnd); ContainerTextElementField.ClearValue(child); } if (value != null) { ContainerTextElementField.SetValue(value, this); contentStart.InsertUIElement(value); } } finally { textContainer.EndChange(); } } } #endregion Public Properties #region Internal Properties ////// UIElementIsland representing embedded Element Layout island within content world. /// internal UIElementIsland UIElementIsland { get { UpdateUIElementIsland(); return _uiElementIsland; } } #endregion Internal Properties #region Private Methods ////// Ensures the _uiElementIsland variable is up to date /// private void UpdateUIElementIsland() { UIElement childElement = this.Child; if(_uiElementIsland == null || _uiElementIsland.Root != childElement) { if(_uiElementIsland != null) { _uiElementIsland.Dispose(); _uiElementIsland = null; } if(childElement != null) { _uiElementIsland = new UIElementIsland(childElement); } } } #endregion Private Methods #region Private Data private UIElementIsland _uiElementIsland; #endregion Private Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
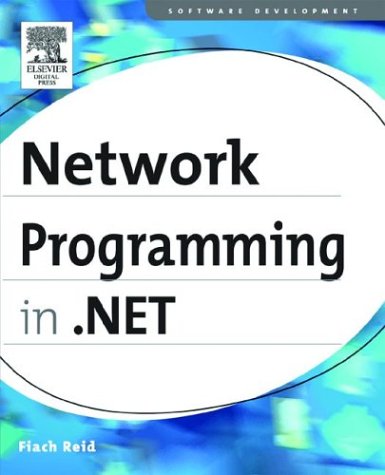
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TdsEnums.cs
- IBuiltInEvidence.cs
- UnionCqlBlock.cs
- TimeManager.cs
- InheritanceContextHelper.cs
- FlowNode.cs
- TextEncodedRawTextWriter.cs
- ConstraintEnumerator.cs
- Rotation3DAnimationBase.cs
- MembershipValidatePasswordEventArgs.cs
- EnumMemberAttribute.cs
- WorkflowPrinting.cs
- WindowsSolidBrush.cs
- InternalConfigRoot.cs
- DataObject.cs
- RealizationDrawingContextWalker.cs
- IsolatedStorageException.cs
- SmtpFailedRecipientException.cs
- CommandEventArgs.cs
- ListDataHelper.cs
- Permission.cs
- PerspectiveCamera.cs
- CodeAttributeArgument.cs
- OutputScopeManager.cs
- EventManager.cs
- DynamicMethod.cs
- AutomationFocusChangedEventArgs.cs
- ADRoleFactory.cs
- TextTrailingWordEllipsis.cs
- HttpTransportSecurityElement.cs
- DesignerListAdapter.cs
- ParserOptions.cs
- PropertyPathWorker.cs
- Stack.cs
- FrameworkTemplate.cs
- NeutralResourcesLanguageAttribute.cs
- MediaCommands.cs
- StickyNote.cs
- BooleanProjectedSlot.cs
- RegexFCD.cs
- DependencyPropertyConverter.cs
- SecurityDocument.cs
- RenderData.cs
- SystemIPAddressInformation.cs
- SafeNativeMethodsOther.cs
- CroppedBitmap.cs
- AstTree.cs
- ConfigXmlText.cs
- SHA256Cng.cs
- SyndicationElementExtensionCollection.cs
- SiteMembershipCondition.cs
- AddInAttribute.cs
- PersonalizationProviderHelper.cs
- RequestSecurityToken.cs
- SimplePropertyEntry.cs
- validation.cs
- EdgeModeValidation.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- HGlobalSafeHandle.cs
- WebFaultClientMessageInspector.cs
- SqlFunctions.cs
- Flowchart.cs
- SqlBulkCopyColumnMappingCollection.cs
- PropertyGeneratedEventArgs.cs
- WebPartUserCapability.cs
- PenLineJoinValidation.cs
- HostSecurityManager.cs
- CryptographicAttribute.cs
- SelectionItemProviderWrapper.cs
- _NegoStream.cs
- XmlCDATASection.cs
- WorkflowApplicationUnhandledExceptionEventArgs.cs
- invalidudtexception.cs
- UrlAuthFailureHandler.cs
- EntitySet.cs
- ClientConfigPaths.cs
- TreeChangeInfo.cs
- EntitySqlQueryState.cs
- RealProxy.cs
- ColorConvertedBitmap.cs
- VarRemapper.cs
- XmlObjectSerializer.cs
- _NTAuthentication.cs
- UnknownBitmapDecoder.cs
- datacache.cs
- SspiHelper.cs
- basemetadatamappingvisitor.cs
- TransformCollection.cs
- PathTooLongException.cs
- RewritingPass.cs
- PerfProviderCollection.cs
- EffectiveValueEntry.cs
- NativeMethods.cs
- ResXResourceSet.cs
- XmlSchemaValidationException.cs
- ProfilePropertySettings.cs
- InkCanvasFeedbackAdorner.cs
- BaseInfoTable.cs
- StorageConditionPropertyMapping.cs
- ContentTypeSettingDispatchMessageFormatter.cs