Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Mapping / StorageTypeMapping.cs / 1 / StorageTypeMapping.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Text; using System.Data.Metadata.Edm; namespace System.Data.Mapping { ////// Represents the Mapping metadata for a type map in CS space. /// ////// For Example if conceptually you could represent the CS MSL file as following /// --Mapping /// --EntityContainerMapping ( CNorthwind-->SNorthwind ) /// --EntitySetMapping /// --EntityTypeMapping /// --TableMappingFragment /// --EntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --EntityTypeMapping /// --TableMappingFragment /// --EntityKey /// --ScalarPropertyMap /// --ComplexPropertyMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --ScalarPropertyMap /// --AssociationSetMapping /// --AssociationTypeMapping /// --TableMappingFragment /// --EndPropertyMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --EndPropertyMap /// --ScalarPropertyMap /// --EntityContainerMapping ( CMyDatabase-->SMyDatabase ) /// --CompositionSetMapping /// --CompositionTypeMapping /// --TableMappingFragment /// --ParentEntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --EntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --ComplexPropertyMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --ScalarPropertyMap /// This class represents the metadata for all the Type map elements in the /// above example namely EntityTypeMapping, AssociationTypeMapping and CompositionTypeMapping. /// The TypeMapping elements contain TableMappingFragments which in turn contain the property maps. /// internal abstract class StorageTypeMapping { #region Constructors ////// Construct the new CSTypeMapping object. /// /// SetMapping that contains this type mapping internal StorageTypeMapping(StorageSetMapping setMapping) { this.m_fragments = new List(); this.m_setMapping = setMapping; } #endregion #region Fields StorageSetMapping m_setMapping; //ExtentMap that contains this type mapping List m_fragments; //Set of fragments that make up the type Mapping #endregion #region Properties /// /// Mapping fragments that make up this set type /// internal ReadOnlyCollectionMappingFragments { get { return this.m_fragments.AsReadOnly(); } } internal StorageSetMapping SetMapping { get { return m_setMapping; } } /// /// a list of TypeMetadata that this mapping holds true for. /// internal abstract ReadOnlyCollectionTypes { get;} /// /// a list of TypeMetadatas for which the mapping holds true for /// not only the type specified but the sub-types of that type as well. /// internal abstract ReadOnlyCollectionIsOfTypes { get;} #endregion #region Methods /// /// Add a fragment mapping as child of this type mapping /// /// internal void AddFragment(StorageMappingFragment fragment) { this.m_fragments.Add(fragment); } ////// This method is primarily for debugging purposes. /// Will be removed shortly. /// /// internal abstract void Print(int index); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Text; using System.Data.Metadata.Edm; namespace System.Data.Mapping { ////// Represents the Mapping metadata for a type map in CS space. /// ////// For Example if conceptually you could represent the CS MSL file as following /// --Mapping /// --EntityContainerMapping ( CNorthwind-->SNorthwind ) /// --EntitySetMapping /// --EntityTypeMapping /// --TableMappingFragment /// --EntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --EntityTypeMapping /// --TableMappingFragment /// --EntityKey /// --ScalarPropertyMap /// --ComplexPropertyMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --ScalarPropertyMap /// --AssociationSetMapping /// --AssociationTypeMapping /// --TableMappingFragment /// --EndPropertyMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --EndPropertyMap /// --ScalarPropertyMap /// --EntityContainerMapping ( CMyDatabase-->SMyDatabase ) /// --CompositionSetMapping /// --CompositionTypeMapping /// --TableMappingFragment /// --ParentEntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --EntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --ComplexPropertyMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --ScalarPropertyMap /// This class represents the metadata for all the Type map elements in the /// above example namely EntityTypeMapping, AssociationTypeMapping and CompositionTypeMapping. /// The TypeMapping elements contain TableMappingFragments which in turn contain the property maps. /// internal abstract class StorageTypeMapping { #region Constructors ////// Construct the new CSTypeMapping object. /// /// SetMapping that contains this type mapping internal StorageTypeMapping(StorageSetMapping setMapping) { this.m_fragments = new List(); this.m_setMapping = setMapping; } #endregion #region Fields StorageSetMapping m_setMapping; //ExtentMap that contains this type mapping List m_fragments; //Set of fragments that make up the type Mapping #endregion #region Properties /// /// Mapping fragments that make up this set type /// internal ReadOnlyCollectionMappingFragments { get { return this.m_fragments.AsReadOnly(); } } internal StorageSetMapping SetMapping { get { return m_setMapping; } } /// /// a list of TypeMetadata that this mapping holds true for. /// internal abstract ReadOnlyCollectionTypes { get;} /// /// a list of TypeMetadatas for which the mapping holds true for /// not only the type specified but the sub-types of that type as well. /// internal abstract ReadOnlyCollectionIsOfTypes { get;} #endregion #region Methods /// /// Add a fragment mapping as child of this type mapping /// /// internal void AddFragment(StorageMappingFragment fragment) { this.m_fragments.Add(fragment); } ////// This method is primarily for debugging purposes. /// Will be removed shortly. /// /// internal abstract void Print(int index); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
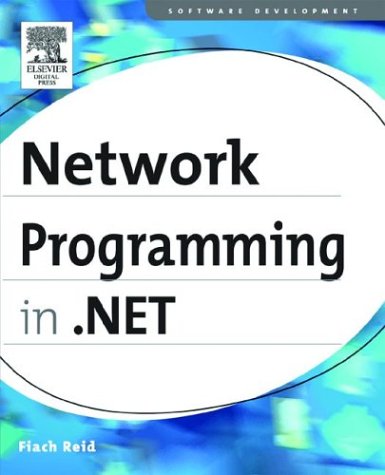
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AddInAdapter.cs
- TransformedBitmap.cs
- FileDataSourceCache.cs
- _ListenerAsyncResult.cs
- _KerberosClient.cs
- XmlSerializer.cs
- SvcMapFileLoader.cs
- VisualTreeUtils.cs
- SchemaTypeEmitter.cs
- SelectionEditingBehavior.cs
- NeutralResourcesLanguageAttribute.cs
- LocalizationComments.cs
- SqlCharStream.cs
- WebException.cs
- HttpStreamXmlDictionaryWriter.cs
- SQLBinary.cs
- CodeSubDirectory.cs
- MatrixCamera.cs
- DiagnosticStrings.cs
- DurableOperationContext.cs
- BulletDecorator.cs
- HttpSocketManager.cs
- mil_sdk_version.cs
- ModuleElement.cs
- TableCellAutomationPeer.cs
- DbDataAdapter.cs
- MgmtConfigurationRecord.cs
- DistinctQueryOperator.cs
- DateTimeOffsetAdapter.cs
- PagerSettings.cs
- WebPartVerbCollection.cs
- HtmlTableRowCollection.cs
- ProfilePropertyNameValidator.cs
- HwndAppCommandInputProvider.cs
- Constraint.cs
- SqlFileStream.cs
- MasterPage.cs
- MulticastDelegate.cs
- MdbDataFileEditor.cs
- LinkedList.cs
- ViewStateModeByIdAttribute.cs
- TypeForwardedToAttribute.cs
- ExtensionDataObject.cs
- CookieProtection.cs
- ListItemCollection.cs
- SafeEventLogWriteHandle.cs
- FastEncoderWindow.cs
- TemplateManager.cs
- XamlToRtfParser.cs
- ControllableStoryboardAction.cs
- ListViewItemEventArgs.cs
- ToolStripDropDown.cs
- SqlNodeTypeOperators.cs
- DataKey.cs
- XmlILModule.cs
- Rss20ItemFormatter.cs
- WsrmMessageInfo.cs
- XmlDataSourceView.cs
- PasswordTextNavigator.cs
- EntitySetBase.cs
- DesignerDataRelationship.cs
- ComEventsHelper.cs
- unitconverter.cs
- MsmqDiagnostics.cs
- TreeViewHitTestInfo.cs
- SelectionWordBreaker.cs
- DocumentSchemaValidator.cs
- StoreContentChangedEventArgs.cs
- ResourcePermissionBase.cs
- _SslSessionsCache.cs
- ResourceDictionary.cs
- DataGridViewCellParsingEventArgs.cs
- DomainUpDown.cs
- NavigateEvent.cs
- GridViewSortEventArgs.cs
- CustomPopupPlacement.cs
- ActivationArguments.cs
- HwndSourceParameters.cs
- HtmlElementCollection.cs
- ModifierKeysConverter.cs
- TemplatedAdorner.cs
- CutCopyPasteHelper.cs
- HttpListenerException.cs
- ImportCatalogPart.cs
- ContainerParaClient.cs
- ParameterToken.cs
- SoapSchemaImporter.cs
- ActivityDesigner.cs
- GPPOINT.cs
- SqlTriggerContext.cs
- StringDictionary.cs
- TransformGroup.cs
- TransportBindingElementImporter.cs
- DatasetMethodGenerator.cs
- Base64WriteStateInfo.cs
- Tag.cs
- AlphaSortedEnumConverter.cs
- BookmarkNameHelper.cs
- XamlTreeBuilder.cs
- ElapsedEventArgs.cs