Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CompMod / System / Collections / Specialized / StringDictionary.cs / 1305376 / StringDictionary.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Collections.Specialized { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Collections; using System.ComponentModel.Design.Serialization; using System.Globalization; ////// [Serializable] [DesignerSerializer("System.Diagnostics.Design.StringDictionaryCodeDomSerializer, " + AssemblyRef.SystemDesign, "System.ComponentModel.Design.Serialization.CodeDomSerializer, " + AssemblyRef.SystemDesign)] // @ public class StringDictionary : IEnumerable { // For compatibility, we want the Keys property to return values in lower-case. // That means using ToLower in each property on this type. Also for backwards // compatibility, we will be converting strings to lower-case, which has a // problem for some Georgian alphabets. Can't really fix it now though... internal Hashtable contents = new Hashtable(); ///Implements a hashtable with the key strongly typed to be /// a string rather than an object. ///Consider this class obsolete - use Dictionary<String, String> instead /// with a proper StringComparer instance. ////// public StringDictionary() { } ///Initializes a new instance of the StringDictionary class. ///If you're using file names, registry keys, etc, you want to use /// a Dictionary<String, Object> and use /// StringComparer.OrdinalIgnoreCase. ////// public virtual int Count { get { return contents.Count; } } ///Gets the number of key-and-value pairs in the StringDictionary. ////// public virtual bool IsSynchronized { get { return contents.IsSynchronized; } } ///Indicates whether access to the StringDictionary is synchronized (thread-safe). This property is /// read-only. ////// public virtual string this[string key] { get { if( key == null ) { throw new ArgumentNullException("key"); } return (string) contents[key.ToLower(CultureInfo.InvariantCulture)]; } set { if( key == null ) { throw new ArgumentNullException("key"); } contents[key.ToLower(CultureInfo.InvariantCulture)] = value; } } ///Gets or sets the value associated with the specified key. ////// public virtual ICollection Keys { get { return contents.Keys; } } ///Gets a collection of keys in the StringDictionary. ////// public virtual object SyncRoot { get { return contents.SyncRoot; } } ///Gets an object that can be used to synchronize access to the StringDictionary. ////// public virtual ICollection Values { get { return contents.Values; } } ///Gets a collection of values in the StringDictionary. ////// public virtual void Add(string key, string value) { if( key == null ) { throw new ArgumentNullException("key"); } contents.Add(key.ToLower(CultureInfo.InvariantCulture), value); } ///Adds an entry with the specified key and value into the StringDictionary. ////// public virtual void Clear() { contents.Clear(); } ///Removes all entries from the StringDictionary. ////// public virtual bool ContainsKey(string key) { if( key == null ) { throw new ArgumentNullException("key"); } return contents.ContainsKey(key.ToLower(CultureInfo.InvariantCulture)); } ///Determines if the string dictionary contains a specific key ////// public virtual bool ContainsValue(string value) { return contents.ContainsValue(value); } ///Determines if the StringDictionary contains a specific value. ////// public virtual void CopyTo(Array array, int index) { contents.CopyTo(array, index); } ///Copies the string dictionary values to a one-dimensional ///instance at the /// specified index. /// public virtual IEnumerator GetEnumerator() { return contents.GetEnumerator(); } ///Returns an enumerator that can iterate through the string dictionary. ////// public virtual void Remove(string key) { if( key == null ) { throw new ArgumentNullException("key"); } contents.Remove(key.ToLower(CultureInfo.InvariantCulture)); } ///Removes the entry with the specified key from the string dictionary. ////// Make this StringDictionary subservient to some other collection. /// /// Replaces the backing store with another, possibly aliased Hashtable. internal void ReplaceHashtable(Hashtable useThisHashtableInstead) { contents = useThisHashtableInstead; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Some code was replacing the contents field with a Hashtable created elsewhere. /// While it may not have been incorrect, we don't want to encourage that pattern, because /// it will replace the IEqualityComparer in the Hashtable, and creates a possibly-undesirable /// link between two seemingly different collections. Let's discourage that somewhat by /// making this an explicit method call instead of an internal field assignment. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Collections.Specialized { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Collections; using System.ComponentModel.Design.Serialization; using System.Globalization; ////// [Serializable] [DesignerSerializer("System.Diagnostics.Design.StringDictionaryCodeDomSerializer, " + AssemblyRef.SystemDesign, "System.ComponentModel.Design.Serialization.CodeDomSerializer, " + AssemblyRef.SystemDesign)] // @ public class StringDictionary : IEnumerable { // For compatibility, we want the Keys property to return values in lower-case. // That means using ToLower in each property on this type. Also for backwards // compatibility, we will be converting strings to lower-case, which has a // problem for some Georgian alphabets. Can't really fix it now though... internal Hashtable contents = new Hashtable(); ///Implements a hashtable with the key strongly typed to be /// a string rather than an object. ///Consider this class obsolete - use Dictionary<String, String> instead /// with a proper StringComparer instance. ////// public StringDictionary() { } ///Initializes a new instance of the StringDictionary class. ///If you're using file names, registry keys, etc, you want to use /// a Dictionary<String, Object> and use /// StringComparer.OrdinalIgnoreCase. ////// public virtual int Count { get { return contents.Count; } } ///Gets the number of key-and-value pairs in the StringDictionary. ////// public virtual bool IsSynchronized { get { return contents.IsSynchronized; } } ///Indicates whether access to the StringDictionary is synchronized (thread-safe). This property is /// read-only. ////// public virtual string this[string key] { get { if( key == null ) { throw new ArgumentNullException("key"); } return (string) contents[key.ToLower(CultureInfo.InvariantCulture)]; } set { if( key == null ) { throw new ArgumentNullException("key"); } contents[key.ToLower(CultureInfo.InvariantCulture)] = value; } } ///Gets or sets the value associated with the specified key. ////// public virtual ICollection Keys { get { return contents.Keys; } } ///Gets a collection of keys in the StringDictionary. ////// public virtual object SyncRoot { get { return contents.SyncRoot; } } ///Gets an object that can be used to synchronize access to the StringDictionary. ////// public virtual ICollection Values { get { return contents.Values; } } ///Gets a collection of values in the StringDictionary. ////// public virtual void Add(string key, string value) { if( key == null ) { throw new ArgumentNullException("key"); } contents.Add(key.ToLower(CultureInfo.InvariantCulture), value); } ///Adds an entry with the specified key and value into the StringDictionary. ////// public virtual void Clear() { contents.Clear(); } ///Removes all entries from the StringDictionary. ////// public virtual bool ContainsKey(string key) { if( key == null ) { throw new ArgumentNullException("key"); } return contents.ContainsKey(key.ToLower(CultureInfo.InvariantCulture)); } ///Determines if the string dictionary contains a specific key ////// public virtual bool ContainsValue(string value) { return contents.ContainsValue(value); } ///Determines if the StringDictionary contains a specific value. ////// public virtual void CopyTo(Array array, int index) { contents.CopyTo(array, index); } ///Copies the string dictionary values to a one-dimensional ///instance at the /// specified index. /// public virtual IEnumerator GetEnumerator() { return contents.GetEnumerator(); } ///Returns an enumerator that can iterate through the string dictionary. ////// public virtual void Remove(string key) { if( key == null ) { throw new ArgumentNullException("key"); } contents.Remove(key.ToLower(CultureInfo.InvariantCulture)); } ///Removes the entry with the specified key from the string dictionary. ////// Make this StringDictionary subservient to some other collection. /// /// Replaces the backing store with another, possibly aliased Hashtable. internal void ReplaceHashtable(Hashtable useThisHashtableInstead) { contents = useThisHashtableInstead; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Some code was replacing the contents field with a Hashtable created elsewhere. /// While it may not have been incorrect, we don't want to encourage that pattern, because /// it will replace the IEqualityComparer in the Hashtable, and creates a possibly-undesirable /// link between two seemingly different collections. Let's discourage that somewhat by /// making this an explicit method call instead of an internal field assignment. ///
Link Menu
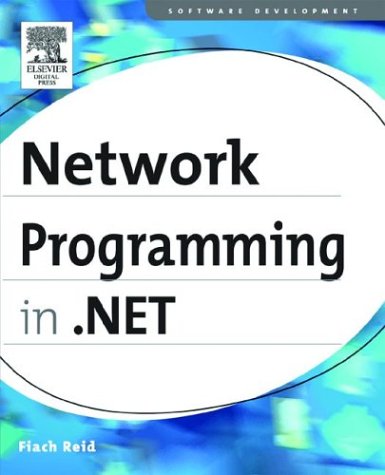
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TrackingStringDictionary.cs
- CodeStatementCollection.cs
- AccessibleObject.cs
- fixedPageContentExtractor.cs
- HostingEnvironmentWrapper.cs
- ProtocolElement.cs
- IgnoreSectionHandler.cs
- DataSourceCacheDurationConverter.cs
- SHA512.cs
- BaseServiceProvider.cs
- GeometryCollection.cs
- XmlSchemaGroupRef.cs
- ComplexPropertyEntry.cs
- Tokenizer.cs
- controlskin.cs
- ResourceDescriptionAttribute.cs
- PackWebRequestFactory.cs
- TextSchema.cs
- Errors.cs
- CodeIndexerExpression.cs
- PolicyStatement.cs
- StorageAssociationTypeMapping.cs
- ToolStripPanelCell.cs
- CharUnicodeInfo.cs
- ImportCatalogPart.cs
- SmuggledIUnknown.cs
- XmlSchemaSimpleTypeUnion.cs
- DataServiceHostFactory.cs
- EncoderFallback.cs
- DataBoundControlAdapter.cs
- ControlCachePolicy.cs
- CreateUserWizardStep.cs
- StorageModelBuildProvider.cs
- KeyEvent.cs
- ArrangedElementCollection.cs
- StopStoryboard.cs
- VirtualDirectoryMapping.cs
- MaskedTextProvider.cs
- Sql8ExpressionRewriter.cs
- ConfigPathUtility.cs
- PerformanceCounterCategory.cs
- PasswordTextNavigator.cs
- TypeSystem.cs
- CommonGetThemePartSize.cs
- Trigger.cs
- OleDragDropHandler.cs
- XNodeNavigator.cs
- MulticastIPAddressInformationCollection.cs
- GridItem.cs
- UriPrefixTable.cs
- PostBackOptions.cs
- UndoManager.cs
- ActivitySurrogateSelector.cs
- ContextStack.cs
- EnumBuilder.cs
- HttpBufferlessInputStream.cs
- TabControl.cs
- OLEDB_Util.cs
- RoleServiceManager.cs
- HashStream.cs
- DataPagerCommandEventArgs.cs
- HasRunnableWorkflowEvent.cs
- ProxyGenerator.cs
- Pkcs7Recipient.cs
- LocatorPartList.cs
- QueryExtender.cs
- TextTreeExtractElementUndoUnit.cs
- BinaryNode.cs
- CapabilitiesAssignment.cs
- TraceShell.cs
- UiaCoreTypesApi.cs
- ASCIIEncoding.cs
- ScriptRegistrationManager.cs
- GridViewColumnCollectionChangedEventArgs.cs
- SchemaNamespaceManager.cs
- ExecutionEngineException.cs
- DataList.cs
- DbConnectionPoolGroup.cs
- EnumDataContract.cs
- DataGridViewColumnDesigner.cs
- StrokeNodeData.cs
- AccessDataSource.cs
- ValueTypeIndexerReference.cs
- SkipStoryboardToFill.cs
- OleDbRowUpdatedEvent.cs
- CodeFieldReferenceExpression.cs
- EntityDataSourceSelectedEventArgs.cs
- TypeBuilderInstantiation.cs
- CustomCategoryAttribute.cs
- XmlNodeList.cs
- InfoCardConstants.cs
- PolygonHotSpot.cs
- TypeDelegator.cs
- StoreItemCollection.cs
- TypeDescriptionProvider.cs
- EDesignUtil.cs
- UserThread.cs
- MultiView.cs
- SplitterEvent.cs
- Contracts.cs