Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / CodeDOM / CodeStatementCollection.cs / 1 / CodeStatementCollection.cs
// ------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // ----------------------------------------------------------------------------- // namespace System.CodeDom { using System; using System.Collections; using System.Runtime.InteropServices; ////// [ ClassInterface(ClassInterfaceType.AutoDispatch), ComVisible(true), Serializable, ] public class CodeStatementCollection : CollectionBase { ////// A collection that stores ///objects. /// /// public CodeStatementCollection() { } ////// Initializes a new instance of ///. /// /// public CodeStatementCollection(CodeStatementCollection value) { this.AddRange(value); } ////// Initializes a new instance of ///based on another . /// /// public CodeStatementCollection(CodeStatement[] value) { this.AddRange(value); } ////// Initializes a new instance of ///containing any array of objects. /// /// public CodeStatement this[int index] { get { return ((CodeStatement)(List[index])); } set { List[index] = value; } } ///Represents the entry at the specified index of the ///. /// public int Add(CodeStatement value) { return List.Add(value); } ///Adds a ///with the specified value to the /// . /// public int Add(CodeExpression value) { return Add(new CodeExpressionStatement(value)); } ///[To be supplied.] ////// public void AddRange(CodeStatement[] value) { if (value == null) { throw new ArgumentNullException("value"); } for (int i = 0; ((i) < (value.Length)); i = ((i) + (1))) { this.Add(value[i]); } } ///Copies the elements of an array to the end of the ///. /// public void AddRange(CodeStatementCollection value) { if (value == null) { throw new ArgumentNullException("value"); } int currentCount = value.Count; for (int i = 0; i < currentCount; i = ((i) + (1))) { this.Add(value[i]); } } ////// Adds the contents of another ///to the end of the collection. /// /// public bool Contains(CodeStatement value) { return List.Contains(value); } ///Gets a value indicating whether the /// ///contains the specified . /// public void CopyTo(CodeStatement[] array, int index) { List.CopyTo(array, index); } ///Copies the ///values to a one-dimensional instance at the /// specified index. /// public int IndexOf(CodeStatement value) { return List.IndexOf(value); } ///Returns the index of a ///in /// the . /// public void Insert(int index, CodeStatement value) { List.Insert(index, value); } ///Inserts a ///into the at the specified index. /// public void Remove(CodeStatement value) { List.Remove(value); } } }Removes a specific ///from the /// .
Link Menu
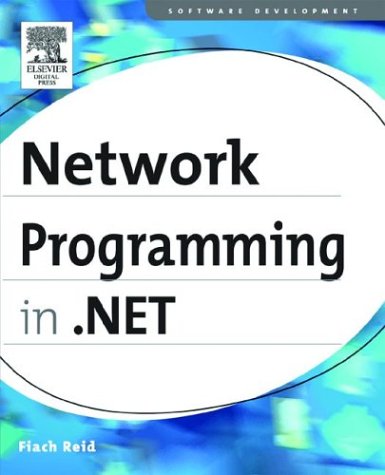
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartZoneBaseDesigner.cs
- ToolStripLabel.cs
- DbConnectionInternal.cs
- CqlIdentifiers.cs
- DispatcherProcessingDisabled.cs
- ACL.cs
- HttpListener.cs
- ByteViewer.cs
- ContentFileHelper.cs
- TagPrefixInfo.cs
- PrintController.cs
- ChangeInterceptorAttribute.cs
- DateTime.cs
- HostedElements.cs
- WebCodeGenerator.cs
- DeclarationUpdate.cs
- Events.cs
- GetPageNumberCompletedEventArgs.cs
- HtmlWindow.cs
- RadialGradientBrush.cs
- SecUtil.cs
- ObjectCacheHost.cs
- XslNumber.cs
- GlyphsSerializer.cs
- HttpVersion.cs
- PersonalizationProviderCollection.cs
- WorkflowRuntimeServiceElementCollection.cs
- MatrixStack.cs
- HotCommands.cs
- Vector3DAnimationBase.cs
- XmlSchemaCompilationSettings.cs
- UnauthorizedWebPart.cs
- TypedTableHandler.cs
- DataGridViewColumnEventArgs.cs
- ModuleElement.cs
- TextParagraph.cs
- ActivityWithResultConverter.cs
- TextElementEditingBehaviorAttribute.cs
- ContainerVisual.cs
- MouseEvent.cs
- ContainerUIElement3D.cs
- BaseTemplateParser.cs
- XmlDocumentSchema.cs
- DeviceOverridableAttribute.cs
- NameTable.cs
- AssemblyUtil.cs
- DateTimeConverter.cs
- SecurityUtils.cs
- RenderOptions.cs
- httpstaticobjectscollection.cs
- JournalEntryListConverter.cs
- ToolStripItemDesigner.cs
- ObjectStateFormatter.cs
- TrustSection.cs
- AssemblyBuilder.cs
- SQLBytes.cs
- ColorConverter.cs
- ContainerUIElement3D.cs
- printdlgexmarshaler.cs
- OperationFormatStyle.cs
- ExpressionBuilderCollection.cs
- TextTrailingCharacterEllipsis.cs
- DateTimeConverter2.cs
- DataKeyCollection.cs
- ListControlConvertEventArgs.cs
- ExecutorLocksHeldException.cs
- EntityDataSourceDesignerHelper.cs
- _ChunkParse.cs
- ClientTarget.cs
- InvokePattern.cs
- PassportAuthenticationModule.cs
- WebFaultClientMessageInspector.cs
- RemotingServices.cs
- SkinBuilder.cs
- StyleCollection.cs
- COM2ExtendedUITypeEditor.cs
- XmlSchemaAttributeGroup.cs
- ProtocolsConfiguration.cs
- BrowserDefinitionCollection.cs
- SessionSwitchEventArgs.cs
- _LocalDataStore.cs
- KeyToListMap.cs
- DbConnectionClosed.cs
- DataBoundControlAdapter.cs
- ServicePoint.cs
- DocumentApplicationJournalEntry.cs
- ColorTransformHelper.cs
- ConfigXmlComment.cs
- SqlXmlStorage.cs
- PagesSection.cs
- ChannelServices.cs
- RequestResponse.cs
- EntityWrapper.cs
- TypeConstant.cs
- IImplicitResourceProvider.cs
- ListViewGroupConverter.cs
- EntityConnectionStringBuilderItem.cs
- SmtpTransport.cs
- PrePrepareMethodAttribute.cs
- SendActivityDesignerTheme.cs