Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / ColorTransformHelper.cs / 1 / ColorTransformHelper.cs
//------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2001, 2002, 2003 // // File: ColorTransformHelper.cs //----------------------------------------------------------------------------- using System; using System.IO; using System.Runtime.InteropServices; using System.Windows.Media; using System.Windows.Media.Imaging; using MS.Internal; using MS.Win32; using System.Security; using System.Security.Permissions; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Diagnostics; using System.Globalization; using Microsoft.Win32.SafeHandles; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods; namespace System.Windows.Media { #region ColorTransformHandle internal class ColorTransformHandle : SafeHandleZeroOrMinusOneIsInvalid { ////// Use this constructor if the handle exists at construction time. /// ////// Critical: The ctor of the base class requires SecurityPermission /// [SecurityCritical] internal ColorTransformHandle() : base(true) { } ////// Use this constructor if the handle exists at construction time. /// ////// Critical: The ctor of the base class requires SecurityPermission /// This code calls SetHandle /// [SecurityCritical] internal ColorTransformHandle(IntPtr profile) : base(true) { SetHandle(profile); } ////// Critical - calls unmanaged code, not treat as safe because you must /// validate that handle is a valid color transform handle. /// [SecurityCritical] protected override bool ReleaseHandle() { if (!UnsafeNativeMethods.Mscms.DeleteColorTransform(handle)) { HRESULT.Check(Marshal.GetHRForLastWin32Error()); } return true; } } #endregion #region ColorTransformHelper ////// Class to call into MSCMS color transform APIs /// internal class ColorTransformHelper { /// Constructor internal ColorTransformHelper() { } /// Creates an ICM Profile Transform /// Retrieves a standard color space profile ////// SecurityCritical: Calls unmanaged code, accepts SafeHandles as input. /// [SecurityCritical] internal void CreateTransform(SafeProfileHandle sourceProfile, SafeProfileHandle destinationProfile) { if (sourceProfile == null || sourceProfile.IsInvalid) { throw new ArgumentNullException("sourceProfile"); } if (destinationProfile == null || destinationProfile.IsInvalid) { throw new ArgumentNullException("destinationProfile"); } IntPtr[] handles = new IntPtr[2]; bool success = true; sourceProfile.DangerousAddRef(ref success); Debug.Assert(success); destinationProfile.DangerousAddRef(ref success); Debug.Assert(success); try { handles[0] = sourceProfile.DangerousGetHandle(); handles[1] = destinationProfile.DangerousGetHandle(); UInt32[] dwIntents = new UInt32[2] {INTENT_PERCEPTUAL, INTENT_PERCEPTUAL}; // No need to get rid of the old handle as it will get GC'ed _transformHandle = UnsafeNativeMethods.Mscms.CreateMultiProfileTransform( handles, (UInt32)handles.Length, dwIntents, (UInt32)dwIntents.Length, BEST_MODE | USE_RELATIVE_COLORIMETRIC, 0 ); } finally { sourceProfile.DangerousRelease(); destinationProfile.DangerousRelease(); } if (_transformHandle == null || _transformHandle.IsInvalid) { HRESULT.Check(Marshal.GetHRForLastWin32Error()); } } /// Translates the colors /// Retrieves a standard color space profile ////// SecurityCritical: Calls unmanaged code, accepts IntPtr/unverified data. /// [SecurityCritical] internal void TranslateColors(IntPtr paInputColors, UInt32 numColors, UInt32 inputColorType, IntPtr paOutputColors, UInt32 outputColorType) { if (_transformHandle == null || _transformHandle.IsInvalid) { throw new InvalidOperationException(SR.Get(SRID.Image_ColorTransformInvalid)); } HRESULT.Check(UnsafeNativeMethods.Mscms.TranslateColors( _transformHandle, paInputColors, numColors, inputColorType, paOutputColors, outputColorType)); } #region Data members /// Handle for the ICM Color Transform private ColorTransformHandle _transformHandle; /// Intents private const UInt32 INTENT_PERCEPTUAL = 0; private const UInt32 INTENT_RELATIVE_COLORIMETRIC = 1; private const UInt32 INTENT_SATURATION = 2; private const UInt32 INTENT_ABSOLUTE_COLORIMETRIC = 3; /// Flags for create color transform private const UInt32 PROOF_MODE = 0x00000001; private const UInt32 NORMAL_MODE = 0x00000002; private const UInt32 BEST_MODE = 0x00000003; private const UInt32 ENABLE_GAMUT_CHECKING = 0x00010000; private const UInt32 USE_RELATIVE_COLORIMETRIC = 0x00020000; private const UInt32 FAST_TRANSLATE = 0x00040000; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
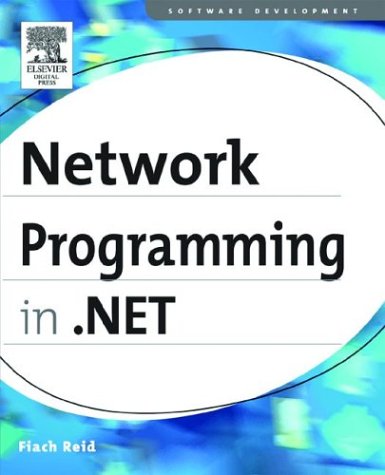
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TransformerTypeCollection.cs
- LogSwitch.cs
- ToolStripManager.cs
- SvcMapFileLoader.cs
- elementinformation.cs
- SaveFileDialog.cs
- DataGridClipboardCellContent.cs
- FrameworkElementFactoryMarkupObject.cs
- HttpPostClientProtocol.cs
- EntityDataSource.cs
- DockProviderWrapper.cs
- HttpClientCertificate.cs
- DefaultAssemblyResolver.cs
- PatternMatchRules.cs
- NullRuntimeConfig.cs
- ClientUtils.cs
- HopperCache.cs
- DiagnosticTrace.cs
- ContentDesigner.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- HelpInfo.cs
- EventBuilder.cs
- FixedSOMImage.cs
- DispatcherProcessingDisabled.cs
- RegisteredExpandoAttribute.cs
- Certificate.cs
- FunctionImportMapping.cs
- Int32Storage.cs
- NotifyParentPropertyAttribute.cs
- FixedDocumentSequencePaginator.cs
- EmptyStringExpandableObjectConverter.cs
- WebPartMenuStyle.cs
- HttpStreamMessage.cs
- CompressEmulationStream.cs
- CodeCompiler.cs
- SafeEventLogWriteHandle.cs
- SafeHandle.cs
- MetadataArtifactLoaderComposite.cs
- CoTaskMemHandle.cs
- ReferenceService.cs
- BasicExpandProvider.cs
- RemoteWebConfigurationHostStream.cs
- HttpRequestCacheValidator.cs
- Win32.cs
- QueryOutputWriter.cs
- WindowsFormsEditorServiceHelper.cs
- PathGradientBrush.cs
- _ListenerResponseStream.cs
- OleDbStruct.cs
- EventMetadata.cs
- CrossContextChannel.cs
- StatusBarItem.cs
- FileClassifier.cs
- TabRenderer.cs
- MdImport.cs
- CssTextWriter.cs
- IIS7UserPrincipal.cs
- AdvancedBindingEditor.cs
- Typography.cs
- SqlServices.cs
- uribuilder.cs
- ImageUrlEditor.cs
- ComponentDispatcher.cs
- WorkflowServiceBuildProvider.cs
- Color.cs
- IFormattable.cs
- AppDomainProtocolHandler.cs
- TextEndOfParagraph.cs
- EncoderFallback.cs
- EventListener.cs
- HostingEnvironmentException.cs
- Soap.cs
- JsonObjectDataContract.cs
- GroupByExpressionRewriter.cs
- DataTableReader.cs
- Bits.cs
- SafeCloseHandleCritical.cs
- DataGridToolTip.cs
- DbConnectionPoolOptions.cs
- TextParagraphCache.cs
- SqlMethodTransformer.cs
- RuntimeHandles.cs
- IdentityNotMappedException.cs
- ResourceDisplayNameAttribute.cs
- XPathDocumentNavigator.cs
- CharacterBufferReference.cs
- DataTransferEventArgs.cs
- SEHException.cs
- EntityDataSourceContextCreatedEventArgs.cs
- OdbcRowUpdatingEvent.cs
- DBProviderConfigurationHandler.cs
- DataDocumentXPathNavigator.cs
- CngAlgorithm.cs
- Stacktrace.cs
- UIElementParagraph.cs
- DataRowExtensions.cs
- ObjectListFieldCollection.cs
- Nodes.cs
- SRGSCompiler.cs
- ProgressBarHighlightConverter.cs