Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Base / MS / Internal / IO / Packaging / Certificate.cs / 1 / Certificate.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Handles serialization to/from X509 Certificate part (X509v3 = ASN.1 DER format) // // History: // 03/22/2004: [....]: Initial Implementation // //----------------------------------------------------------------------------- using System; using System.Diagnostics; // for Assert using System.Security.Cryptography.X509Certificates; using System.Windows; // For Exception strings - SRID using System.IO.Packaging; using System.IO; // for Stream using MS.Internal; // For ContentType namespace MS.Internal.IO.Packaging { ////// CertificatePart /// internal class CertificatePart { #region Internal Members //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- ////// Type of relationship to a Certificate Part /// static internal string RelationshipType { get { return _certificatePartRelationshipType; } } ////// Prefix of auto-generated Certificate Part names /// static internal string PartNamePrefix { get { return _certificatePartNamePrefix; } } ////// Extension of Certificate Part file names /// static internal string PartNameExtension { get { return _certificatePartNameExtension; } } ////// ContentType of Certificate Parts /// static internal ContentType ContentType { get { return _certificatePartContentType; } } internal Uri Uri { get { return _part.Uri; } } //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- ////// Certificate to associate with this Certificate Part /// ////// stream is too large internal X509Certificate2 GetCertificate() { // lazy init if (_certificate == null) { // obtain from the part using (Stream s = _part.GetStream()) { if (s.Length > 0) { // throw if stream is beyond any reasonable length if (s.Length > _maximumCertificateStreamLength) throw new FileFormatException(SR.Get(SRID.CorruptedData)); // X509Certificate constructor desires a byte array Byte[] byteArray = new Byte[s.Length]; PackagingUtilities.ReliableRead(s, byteArray, 0, (int)s.Length); _certificate = new X509Certificate2(byteArray); } } } return _certificate; } internal void SetCertificate(X509Certificate2 certificate) { if (certificate == null) throw new ArgumentNullException("certificate"); _certificate = certificate; // persist to the part Byte[] byteArray = _certificate.GetRawCertData(); // FileMode.Create will ensure that the stream will shrink if overwritten using (Stream s = _part.GetStream(FileMode.Create, FileAccess.Write)) { s.Write(byteArray, 0, byteArray.Length); } } ////// CertificatePart constructor /// internal CertificatePart(Package container, Uri partName) { if (container == null) throw new ArgumentNullException("container"); if (partName == null) throw new ArgumentNullException("partName"); partName = PackUriHelper.ValidatePartUri(partName); // create if not found if (container.PartExists(partName)) { // open the part _part = container.GetPart(partName); // ensure the part is of the expected type if (_part.ValidatedContentType.AreTypeAndSubTypeEqual(_certificatePartContentType) == false) throw new FileFormatException(SR.Get(SRID.CertificatePartContentTypeMismatch)); } else { // create the part _part = container.CreatePart(partName, _certificatePartContentType.ToString()); } } //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ private PackagePart _part; // part that houses the certificate private X509Certificate2 _certificate; // certificate itself // certificate part constants private static readonly ContentType _certificatePartContentType = new ContentType("application/vnd.openxmlformats-package.digital-signature-certificate"); private static readonly string _certificatePartNamePrefix = "/package/services/digital-signature/certificate/"; private static readonly string _certificatePartNameExtension = ".cer"; private static readonly string _certificatePartRelationshipType = "http://schemas.openxmlformats.org/package/2006/relationships/digital-signature/certificate"; private static long _maximumCertificateStreamLength = 0x40000; // 4MB #endregion Private Members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
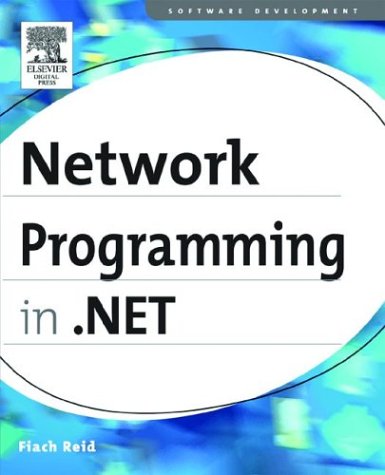
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ButtonStandardAdapter.cs
- PDBReader.cs
- MailSettingsSection.cs
- PEFileReader.cs
- FlowDocumentView.cs
- NumericUpDownAcceleration.cs
- DataGridViewImageColumn.cs
- FromRequest.cs
- ContractsBCL.cs
- ICollection.cs
- RemotingConfiguration.cs
- AutoResizedEvent.cs
- BuildProvider.cs
- AnnotationHelper.cs
- OracleParameterCollection.cs
- FusionWrap.cs
- DirectionalAction.cs
- EventListenerClientSide.cs
- DataGridViewCellConverter.cs
- EventPropertyMap.cs
- ToolStripContainerDesigner.cs
- HiddenField.cs
- Size3D.cs
- AsyncPostBackTrigger.cs
- Tile.cs
- XsltSettings.cs
- TypeConverterAttribute.cs
- WindowsFormsHost.cs
- ToolStripScrollButton.cs
- CultureInfo.cs
- CompatibleComparer.cs
- Pkcs7Signer.cs
- TreeNodeCollectionEditorDialog.cs
- InternalTypeHelper.cs
- ObjectManager.cs
- DummyDataSource.cs
- SqlFlattener.cs
- CodeAttachEventStatement.cs
- DataRowCollection.cs
- WindowsGraphics2.cs
- ConfigurationException.cs
- SurrogateEncoder.cs
- GuidelineCollection.cs
- _emptywebproxy.cs
- HttpAsyncResult.cs
- LocalizationComments.cs
- CreateRefExpr.cs
- SqlClientFactory.cs
- CodeSnippetExpression.cs
- XmlSchemaNotation.cs
- ReadOnlyCollectionBase.cs
- DesignerLinkAdapter.cs
- Bidi.cs
- CodeDelegateInvokeExpression.cs
- HTTPNotFoundHandler.cs
- handlecollector.cs
- Lease.cs
- WebHttpSecurity.cs
- Calendar.cs
- XmlNodeWriter.cs
- DocumentsTrace.cs
- _OSSOCK.cs
- BindStream.cs
- WsiProfilesElement.cs
- RemotingSurrogateSelector.cs
- MemberHolder.cs
- AmbiguousMatchException.cs
- PathGradientBrush.cs
- SchemaSetCompiler.cs
- WebPartConnectVerb.cs
- RepeaterDesigner.cs
- SecurityState.cs
- Camera.cs
- InvokeHandlers.cs
- Util.cs
- DiagnosticTraceSource.cs
- AdapterDictionary.cs
- CodeNamespace.cs
- AsyncResult.cs
- InstanceBehavior.cs
- LifetimeServices.cs
- EventlogProvider.cs
- WebReferencesBuildProvider.cs
- ButtonStandardAdapter.cs
- ValidatorCompatibilityHelper.cs
- EntityDesignerUtils.cs
- WebServiceResponse.cs
- SubMenuStyle.cs
- CriticalFinalizerObject.cs
- DefaultValidator.cs
- CharConverter.cs
- XmlTextEncoder.cs
- ModelPerspective.cs
- SamlSubjectStatement.cs
- ToolStripOverflow.cs
- Maps.cs
- OleDbErrorCollection.cs
- RectangleGeometry.cs
- EmbeddedMailObject.cs
- OperationPickerDialog.cs