Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / clr / src / BCL / System / Reflection / Cache / InternalCache.cs / 1 / InternalCache.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: InternalCache ** ** ** Purpose: A high-performance internal caching class. All key ** lookups are done on the basis of the CacheObjType enum. The ** cache is limited to one object of any particular type per ** instance. ** ** ============================================================*/ using System; using System.Threading; using System.Diagnostics; using System.Runtime.CompilerServices; using System.Runtime.Remoting.Metadata; namespace System.Reflection.Cache { [Serializable] internal enum CacheAction { AllocateCache = 1, AddItem = 2, ClearCache = 3, LookupItemHit = 4, LookupItemMiss = 5, GrowCache = 6, SetItemReplace = 7, ReplaceFailed = 8 } [Serializable] internal class InternalCache { private const int MinCacheSize = 2; //We'll start the cache as null and only grow it as we need it. private InternalCacheItem[] m_cache=null; private int m_numItems = 0; // private bool m_copying = false; //Knowing the name of the cache is very useful for debugging, but we don't //want to take the working-set hit in the debug build. We'll only include //the field in that build. #if _LOGGING private String m_cacheName; #endif internal InternalCache(String cacheName) { #if _LOGGING m_cacheName = cacheName; #endif //We won't allocate any items until the first time that they're requested. } internal Object this[CacheObjType cacheType] { get { //Let's snapshot a reference to the array up front so that //we don't have to worry about any writers. It's important //to grab the cache first and then numItems. In the event that //the cache gets cleared, m_numItems will be set to 0 before //we actually release the cache. Getting it second will cause //us to walk only 0 elements, but not to fault. InternalCacheItem[] cache = m_cache; int numItems = m_numItems; int position = FindObjectPosition(cache, numItems, cacheType, false); if (position>=0) { if (!BCLDebug.m_loggingNotEnabled) LogAction(CacheAction.LookupItemHit, cacheType, cache[position].Value); return cache[position].Value; } //Couldn't find it -- oh, well. if (!BCLDebug.m_loggingNotEnabled) LogAction(CacheAction.LookupItemMiss, cacheType); return null; } set { int position; if (!BCLDebug.m_loggingNotEnabled) LogAction(CacheAction.AddItem, cacheType, value); lock(this) { position = FindObjectPosition(m_cache, m_numItems, cacheType, true); if (position>0) { m_cache[position].Value = value; m_cache[position].Key = cacheType; if (position==m_numItems) { m_numItems++; } return; } if (m_cache==null) { if (!BCLDebug.m_loggingNotEnabled) LogAction(CacheAction.AllocateCache, cacheType); // m_copying = true; m_cache = new InternalCacheItem[MinCacheSize]; m_cache[0].Value = value; m_cache[0].Key = cacheType; m_numItems = 1; // m_copying = false; } else { if (!BCLDebug.m_loggingNotEnabled) LogAction(CacheAction.GrowCache, cacheType); // m_copying = true; InternalCacheItem[] tempCache = new InternalCacheItem[m_numItems * 2]; for (int i=0; icache.Length) { itemCount = cache.Length; } for (int i=0; i =0) { if (!BCLDebug.m_loggingNotEnabled) LogAction(CacheAction.LookupItemHit, cacheType, cache[position].Value); return cache[position].Value; } //Couldn't find it -- oh, well. if (!BCLDebug.m_loggingNotEnabled) LogAction(CacheAction.LookupItemMiss, cacheType); return null; } set { int position; if (!BCLDebug.m_loggingNotEnabled) LogAction(CacheAction.AddItem, cacheType, value); lock(this) { position = FindObjectPosition(m_cache, m_numItems, cacheType, true); if (position>0) { m_cache[position].Value = value; m_cache[position].Key = cacheType; if (position==m_numItems) { m_numItems++; } return; } if (m_cache==null) { if (!BCLDebug.m_loggingNotEnabled) LogAction(CacheAction.AllocateCache, cacheType); // m_copying = true; m_cache = new InternalCacheItem[MinCacheSize]; m_cache[0].Value = value; m_cache[0].Key = cacheType; m_numItems = 1; // m_copying = false; } else { if (!BCLDebug.m_loggingNotEnabled) LogAction(CacheAction.GrowCache, cacheType); // m_copying = true; InternalCacheItem[] tempCache = new InternalCacheItem[m_numItems * 2]; for (int i=0; i cache.Length) { itemCount = cache.Length; } for (int i=0; i
Link Menu
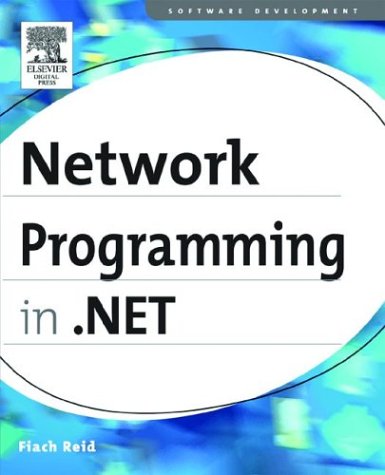
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CollectionTypeElement.cs
- configsystem.cs
- UpDownBase.cs
- ActivityInterfaces.cs
- CodeMemberField.cs
- PerformanceCounterLib.cs
- ClientSideProviderDescription.cs
- EditableRegion.cs
- WindowsScrollBarBits.cs
- QueryTask.cs
- AnimationStorage.cs
- DesignerLinkAdapter.cs
- MediaScriptCommandRoutedEventArgs.cs
- EditorZone.cs
- IdentityModelDictionary.cs
- ColumnBinding.cs
- Transaction.cs
- DataGridViewCell.cs
- VisualBrush.cs
- Point3D.cs
- MemberHolder.cs
- SerializableAttribute.cs
- VectorKeyFrameCollection.cs
- ListDictionary.cs
- ArrangedElement.cs
- CodeIdentifiers.cs
- OutOfProcStateClientManager.cs
- PrivilegeNotHeldException.cs
- SoapAttributes.cs
- Marshal.cs
- Button.cs
- RSAOAEPKeyExchangeDeformatter.cs
- VoiceObjectToken.cs
- ExtensionsSection.cs
- OdbcEnvironmentHandle.cs
- MemoryRecordBuffer.cs
- SmiContextFactory.cs
- XmlSchemaSimpleTypeUnion.cs
- EmbeddedMailObject.cs
- HttpRequestWrapper.cs
- CalendarAutoFormatDialog.cs
- SpeechEvent.cs
- SerializationAttributes.cs
- TypeConverterValueSerializer.cs
- PackageProperties.cs
- UrlPath.cs
- TextSelectionProcessor.cs
- ConfigurationManagerHelperFactory.cs
- RegistryExceptionHelper.cs
- ReadOnlyDictionary.cs
- SchemaName.cs
- TextParaLineResult.cs
- DataGridTableCollection.cs
- SmiContext.cs
- StandardTransformFactory.cs
- TabControlDesigner.cs
- XmlDictionaryString.cs
- TextTreeNode.cs
- SelectedDatesCollection.cs
- OptimalBreakSession.cs
- dsa.cs
- XsltSettings.cs
- XamlPathDataSerializer.cs
- TypeLibConverter.cs
- ColorInterpolationModeValidation.cs
- BlurEffect.cs
- PathNode.cs
- DynamicRendererThreadManager.cs
- ToolboxItem.cs
- HtmlShim.cs
- KnowledgeBase.cs
- BindingNavigator.cs
- ControlCommandSet.cs
- PrivateFontCollection.cs
- Win32.cs
- ObjectItemNoOpAssemblyLoader.cs
- WindowsUserNameSecurityTokenAuthenticator.cs
- DbDataAdapter.cs
- XmlReflectionMember.cs
- CompilationUtil.cs
- PageAsyncTask.cs
- XmlNamespaceMappingCollection.cs
- OracleCommandSet.cs
- StrongNameIdentityPermission.cs
- BrowserTree.cs
- xmlfixedPageInfo.cs
- ImageKeyConverter.cs
- SspiSafeHandles.cs
- RequestReplyCorrelator.cs
- StringToken.cs
- Material.cs
- ComponentChangedEvent.cs
- SourceFileBuildProvider.cs
- RootBrowserWindow.cs
- NetworkStream.cs
- DropDownButton.cs
- IImplicitResourceProvider.cs
- XmlHierarchicalEnumerable.cs
- InternalBufferOverflowException.cs
- HttpException.cs