Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / ContainerVisual.cs / 1 / ContainerVisual.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: ContainerVisual.cs // // History: GSchneid: 05/28/2003 // Created it. //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Windows.Threading; using System.Windows.Media; using System.Windows.Media.Effects; using System.Diagnostics; using System.Collections; using MS.Internal; using System.Resources; using System.Runtime.InteropServices; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// A ContainerVisual is a Container for other Visuals. /// public class ContainerVisual : Visual { ////// Ctor ContainerVisual. /// public ContainerVisual() { _children = new VisualCollection(this); } // ----------------------------------------------------------------------------------------- // Publicly re-exposed VisualTreeHelper interfaces. // ----------------------------------------------------------------------------------------- ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public VisualCollection Children { get { VerifyAPIReadOnly(); return _children; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public DependencyObject Parent { get { return base.VisualParent; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public Geometry Clip { get { return base.VisualClip; } set { base.VisualClip = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public double Opacity { get { return base.VisualOpacity; } set { base.VisualOpacity = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public Brush OpacityMask { get { return base.VisualOpacityMask; } set { base.VisualOpacityMask = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// [Obsolete(MS.Internal.Media.VisualTreeUtils.BitmapEffectObsoleteMessage)] public BitmapEffect BitmapEffect { get { return base.VisualBitmapEffect; } set { base.VisualBitmapEffect = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// [Obsolete(MS.Internal.Media.VisualTreeUtils.BitmapEffectObsoleteMessage)] public BitmapEffectInput BitmapEffectInput { get { return base.VisualBitmapEffectInput; } set { base.VisualBitmapEffectInput = value; } } ////// Gets or sets X- (vertical) guidelines on this Visual. /// [DefaultValue(null)] public DoubleCollection XSnappingGuidelines { get { return base.VisualXSnappingGuidelines; } set { base.VisualXSnappingGuidelines = value; } } ////// Gets or sets Y- (vertical) guidelines on this Visual. /// [DefaultValue(null)] public DoubleCollection YSnappingGuidelines { get { return base.VisualYSnappingGuidelines; } set { base.VisualYSnappingGuidelines = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// new public HitTestResult HitTest(Point point) { return base.HitTest(point); } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// new public void HitTest(HitTestFilterCallback filterCallback, HitTestResultCallback resultCallback, HitTestParameters hitTestParameters) { base.HitTest(filterCallback, resultCallback, hitTestParameters); } ////// VisualContentBounds returns the bounding box for the contents of this Visual. /// public Rect ContentBounds { get { return base.VisualContentBounds; } } ////// Gets or sets the Transform property. /// public Transform Transform { get { return base.VisualTransform; } set { base.VisualTransform = value; } } ////// Gets or sets the Offset property. /// public Vector Offset { get { return base.VisualOffset; } set { base.VisualOffset = value; } } ////// DescendantBounds returns the union of all of the content bounding /// boxes for all of the descendants of the current visual, but not including /// the contents of the current visual. /// public Rect DescendantBounds { get { return base.VisualDescendantBounds; } } // ------------------------------------------------------------------------------------------ // Protected methods // ----------------------------------------------------------------------------------------- ////// Derived class must implement to support Visual children. The method must return /// the child at the specified index. Index must be between 0 and GetVisualChildrenCount-1. /// /// By default a Visual does not have any children. /// /// Remark: /// During this virtual call it is not valid to modify the Visual tree. /// protected sealed override Visual GetVisualChild(int index) { //VisualCollection does the range check for index return _children[index]; } ////// Derived classes override this property to enable the Visual code to enumerate /// the Visual children. Derived classes need to return the number of children /// from this method. /// /// By default a Visual does not have any children. /// /// Remark: During this virtual method the Visual tree must not be modified. /// protected sealed override int VisualChildrenCount { get { return _children.Count; } } // ------------------------------------------------------------------------------------------ // Private fields // ------------------------------------------------------------------------------------------ private readonly VisualCollection _children; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: ContainerVisual.cs // // History: GSchneid: 05/28/2003 // Created it. //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Windows.Threading; using System.Windows.Media; using System.Windows.Media.Effects; using System.Diagnostics; using System.Collections; using MS.Internal; using System.Resources; using System.Runtime.InteropServices; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// A ContainerVisual is a Container for other Visuals. /// public class ContainerVisual : Visual { ////// Ctor ContainerVisual. /// public ContainerVisual() { _children = new VisualCollection(this); } // ----------------------------------------------------------------------------------------- // Publicly re-exposed VisualTreeHelper interfaces. // ----------------------------------------------------------------------------------------- ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public VisualCollection Children { get { VerifyAPIReadOnly(); return _children; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public DependencyObject Parent { get { return base.VisualParent; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public Geometry Clip { get { return base.VisualClip; } set { base.VisualClip = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public double Opacity { get { return base.VisualOpacity; } set { base.VisualOpacity = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// public Brush OpacityMask { get { return base.VisualOpacityMask; } set { base.VisualOpacityMask = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// [Obsolete(MS.Internal.Media.VisualTreeUtils.BitmapEffectObsoleteMessage)] public BitmapEffect BitmapEffect { get { return base.VisualBitmapEffect; } set { base.VisualBitmapEffect = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// [Obsolete(MS.Internal.Media.VisualTreeUtils.BitmapEffectObsoleteMessage)] public BitmapEffectInput BitmapEffectInput { get { return base.VisualBitmapEffectInput; } set { base.VisualBitmapEffectInput = value; } } ////// Gets or sets X- (vertical) guidelines on this Visual. /// [DefaultValue(null)] public DoubleCollection XSnappingGuidelines { get { return base.VisualXSnappingGuidelines; } set { base.VisualXSnappingGuidelines = value; } } ////// Gets or sets Y- (vertical) guidelines on this Visual. /// [DefaultValue(null)] public DoubleCollection YSnappingGuidelines { get { return base.VisualYSnappingGuidelines; } set { base.VisualYSnappingGuidelines = value; } } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// new public HitTestResult HitTest(Point point) { return base.HitTest(point); } ////// Re-exposes the Visual base class's corresponding VisualTreeHelper implementation as public method. /// new public void HitTest(HitTestFilterCallback filterCallback, HitTestResultCallback resultCallback, HitTestParameters hitTestParameters) { base.HitTest(filterCallback, resultCallback, hitTestParameters); } ////// VisualContentBounds returns the bounding box for the contents of this Visual. /// public Rect ContentBounds { get { return base.VisualContentBounds; } } ////// Gets or sets the Transform property. /// public Transform Transform { get { return base.VisualTransform; } set { base.VisualTransform = value; } } ////// Gets or sets the Offset property. /// public Vector Offset { get { return base.VisualOffset; } set { base.VisualOffset = value; } } ////// DescendantBounds returns the union of all of the content bounding /// boxes for all of the descendants of the current visual, but not including /// the contents of the current visual. /// public Rect DescendantBounds { get { return base.VisualDescendantBounds; } } // ------------------------------------------------------------------------------------------ // Protected methods // ----------------------------------------------------------------------------------------- ////// Derived class must implement to support Visual children. The method must return /// the child at the specified index. Index must be between 0 and GetVisualChildrenCount-1. /// /// By default a Visual does not have any children. /// /// Remark: /// During this virtual call it is not valid to modify the Visual tree. /// protected sealed override Visual GetVisualChild(int index) { //VisualCollection does the range check for index return _children[index]; } ////// Derived classes override this property to enable the Visual code to enumerate /// the Visual children. Derived classes need to return the number of children /// from this method. /// /// By default a Visual does not have any children. /// /// Remark: During this virtual method the Visual tree must not be modified. /// protected sealed override int VisualChildrenCount { get { return _children.Count; } } // ------------------------------------------------------------------------------------------ // Private fields // ------------------------------------------------------------------------------------------ private readonly VisualCollection _children; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
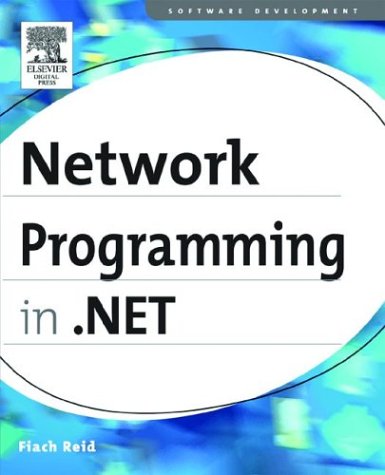
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SemaphoreFullException.cs
- OleDbPermission.cs
- TdsParserStateObject.cs
- TraceListeners.cs
- OleCmdHelper.cs
- XmlDataDocument.cs
- DataColumnChangeEvent.cs
- AsyncStreamReader.cs
- PrimitiveSchema.cs
- XXXOnTypeBuilderInstantiation.cs
- CqlLexer.cs
- BaseTreeIterator.cs
- SqlNode.cs
- ZoneLinkButton.cs
- BroadcastEventHelper.cs
- BuildProviderCollection.cs
- GenericEnumConverter.cs
- LogAppendAsyncResult.cs
- ConfigViewGenerator.cs
- ViewCellRelation.cs
- StringStorage.cs
- SiteMap.cs
- PropertyMetadata.cs
- ListInitExpression.cs
- DesignParameter.cs
- recordstatefactory.cs
- ConstructorBuilder.cs
- ImagingCache.cs
- ServiceBehaviorAttribute.cs
- XmlIncludeAttribute.cs
- typedescriptorpermissionattribute.cs
- XmlSchemaValidator.cs
- BinarySerializer.cs
- RemoteArgument.cs
- DataGridViewHitTestInfo.cs
- Animatable.cs
- PriorityQueue.cs
- DetailsViewModeEventArgs.cs
- HtmlTableCellCollection.cs
- PresentationAppDomainManager.cs
- IIS7UserPrincipal.cs
- X509IssuerSerialKeyIdentifierClause.cs
- NavigationService.cs
- EntityDataSourceWrapperCollection.cs
- TagNameToTypeMapper.cs
- LinqDataSourceContextEventArgs.cs
- SourceElementsCollection.cs
- EventRouteFactory.cs
- ChangeNode.cs
- CaseStatementSlot.cs
- XmlImplementation.cs
- DES.cs
- PackageRelationshipSelector.cs
- FrameSecurityDescriptor.cs
- XhtmlBasicSelectionListAdapter.cs
- RestHandler.cs
- TextLine.cs
- AuthorizationRule.cs
- SymDocumentType.cs
- CommandValueSerializer.cs
- TextProviderWrapper.cs
- ViewBase.cs
- AssemblyNameProxy.cs
- ApplicationActivator.cs
- ChangeDirector.cs
- MediaTimeline.cs
- initElementDictionary.cs
- PreviewPageInfo.cs
- _DigestClient.cs
- TransactionFlowBindingElement.cs
- DataGridColumnCollection.cs
- ReadOnlyTernaryTree.cs
- bidPrivateBase.cs
- sqlser.cs
- BufferedGraphics.cs
- XmlObjectSerializerWriteContext.cs
- DocumentGridContextMenu.cs
- _NestedSingleAsyncResult.cs
- WmlTextBoxAdapter.cs
- WeakEventTable.cs
- OrderedEnumerableRowCollection.cs
- OracleSqlParser.cs
- ListenerSessionConnection.cs
- DataSourceHelper.cs
- ActivityMarkupSerializer.cs
- Attribute.cs
- GridViewEditEventArgs.cs
- SamlAssertion.cs
- RequestCachePolicy.cs
- ListViewItem.cs
- UrlPropertyAttribute.cs
- SetState.cs
- XPathParser.cs
- Int32Converter.cs
- OracleDateTime.cs
- OuterGlowBitmapEffect.cs
- TextComposition.cs
- HttpServerUtilityBase.cs
- SingleObjectCollection.cs
- UriParserTemplates.cs