Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Routing / UrlRoutingModule.cs / 1305376 / UrlRoutingModule.cs
namespace System.Web.Routing { using System.Diagnostics; using System.Globalization; using System.Runtime.CompilerServices; using System.Web.Security; [TypeForwardedFrom("System.Web.Routing, Version=3.5.0.0, Culture=Neutral, PublicKeyToken=31bf3856ad364e35")] public class UrlRoutingModule : IHttpModule { private static readonly object _contextKey = new Object(); private static readonly object _requestDataKey = new Object(); private RouteCollection _routeCollection; [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2227:CollectionPropertiesShouldBeReadOnly", Justification = "This needs to be settable for unit tests.")] public RouteCollection RouteCollection { get { if (_routeCollection == null) { _routeCollection = RouteTable.Routes; } return _routeCollection; } set { _routeCollection = value; } } protected virtual void Dispose() { } protected virtual void Init(HttpApplication application) { ////////////////////////////////////////////////////////////////// // Check if this module has been already addded if (application.Context.Items[_contextKey] != null) { return; // already added to the pipeline } application.Context.Items[_contextKey] = _contextKey; // Ideally we would use the MapRequestHandler event. However, MapRequestHandler is not available // in II6 or IIS7 ISAPI Mode. Instead, we use PostResolveRequestCache, which is the event immediately // before MapRequestHandler. This allows use to use one common codepath for all versions of IIS. application.PostResolveRequestCache += OnApplicationPostResolveRequestCache; } private void OnApplicationPostResolveRequestCache(object sender, EventArgs e) { HttpContextBase context = new HttpContextWrapper(((HttpApplication)sender).Context); PostResolveRequestCache(context); } [Obsolete("This method is obsolete. Override the Init method to use the PostMapRequestHandler event.")] public virtual void PostMapRequestHandler(HttpContextBase context) { // Backwards compat with 3.5 which used to have code here to Rewrite the URL } public virtual void PostResolveRequestCache(HttpContextBase context) { // Match the incoming URL against the route table RouteData routeData = RouteCollection.GetRouteData(context); // Do nothing if no route found if (routeData == null) { return; } // If a route was found, get an IHttpHandler from the route's RouteHandler IRouteHandler routeHandler = routeData.RouteHandler; if (routeHandler == null) { throw new InvalidOperationException( String.Format( CultureInfo.CurrentUICulture, SR.GetString(SR.UrlRoutingModule_NoRouteHandler))); } // This is a special IRouteHandler that tells the routing module to stop processing // routes and to let the fallback handler handle the request. if (routeHandler is StopRoutingHandler) { return; } RequestContext requestContext = new RequestContext(context, routeData); // Dev10 766875 Adding RouteData to HttpContext context.Request.RequestContext = requestContext; IHttpHandler httpHandler = routeHandler.GetHttpHandler(requestContext); if (httpHandler == null) { throw new InvalidOperationException( String.Format( CultureInfo.CurrentUICulture, SR.GetString(SR.UrlRoutingModule_NoHttpHandler), routeHandler.GetType())); } if (httpHandler is UrlAuthFailureHandler) { if (FormsAuthenticationModule.FormsAuthRequired) { UrlAuthorizationModule.ReportUrlAuthorizationFailure(HttpContext.Current, this); return; } else { throw new HttpException(401, SR.GetString(SR.Assess_Denied_Description3)); } } // Remap IIS7 to our handler context.RemapHandler(httpHandler); } #region IHttpModule Members void IHttpModule.Dispose() { Dispose(); } void IHttpModule.Init(HttpApplication application) { Init(application); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Web.Routing { using System.Diagnostics; using System.Globalization; using System.Runtime.CompilerServices; using System.Web.Security; [TypeForwardedFrom("System.Web.Routing, Version=3.5.0.0, Culture=Neutral, PublicKeyToken=31bf3856ad364e35")] public class UrlRoutingModule : IHttpModule { private static readonly object _contextKey = new Object(); private static readonly object _requestDataKey = new Object(); private RouteCollection _routeCollection; [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2227:CollectionPropertiesShouldBeReadOnly", Justification = "This needs to be settable for unit tests.")] public RouteCollection RouteCollection { get { if (_routeCollection == null) { _routeCollection = RouteTable.Routes; } return _routeCollection; } set { _routeCollection = value; } } protected virtual void Dispose() { } protected virtual void Init(HttpApplication application) { ////////////////////////////////////////////////////////////////// // Check if this module has been already addded if (application.Context.Items[_contextKey] != null) { return; // already added to the pipeline } application.Context.Items[_contextKey] = _contextKey; // Ideally we would use the MapRequestHandler event. However, MapRequestHandler is not available // in II6 or IIS7 ISAPI Mode. Instead, we use PostResolveRequestCache, which is the event immediately // before MapRequestHandler. This allows use to use one common codepath for all versions of IIS. application.PostResolveRequestCache += OnApplicationPostResolveRequestCache; } private void OnApplicationPostResolveRequestCache(object sender, EventArgs e) { HttpContextBase context = new HttpContextWrapper(((HttpApplication)sender).Context); PostResolveRequestCache(context); } [Obsolete("This method is obsolete. Override the Init method to use the PostMapRequestHandler event.")] public virtual void PostMapRequestHandler(HttpContextBase context) { // Backwards compat with 3.5 which used to have code here to Rewrite the URL } public virtual void PostResolveRequestCache(HttpContextBase context) { // Match the incoming URL against the route table RouteData routeData = RouteCollection.GetRouteData(context); // Do nothing if no route found if (routeData == null) { return; } // If a route was found, get an IHttpHandler from the route's RouteHandler IRouteHandler routeHandler = routeData.RouteHandler; if (routeHandler == null) { throw new InvalidOperationException( String.Format( CultureInfo.CurrentUICulture, SR.GetString(SR.UrlRoutingModule_NoRouteHandler))); } // This is a special IRouteHandler that tells the routing module to stop processing // routes and to let the fallback handler handle the request. if (routeHandler is StopRoutingHandler) { return; } RequestContext requestContext = new RequestContext(context, routeData); // Dev10 766875 Adding RouteData to HttpContext context.Request.RequestContext = requestContext; IHttpHandler httpHandler = routeHandler.GetHttpHandler(requestContext); if (httpHandler == null) { throw new InvalidOperationException( String.Format( CultureInfo.CurrentUICulture, SR.GetString(SR.UrlRoutingModule_NoHttpHandler), routeHandler.GetType())); } if (httpHandler is UrlAuthFailureHandler) { if (FormsAuthenticationModule.FormsAuthRequired) { UrlAuthorizationModule.ReportUrlAuthorizationFailure(HttpContext.Current, this); return; } else { throw new HttpException(401, SR.GetString(SR.Assess_Denied_Description3)); } } // Remap IIS7 to our handler context.RemapHandler(httpHandler); } #region IHttpModule Members void IHttpModule.Dispose() { Dispose(); } void IHttpModule.Init(HttpApplication application) { Init(application); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
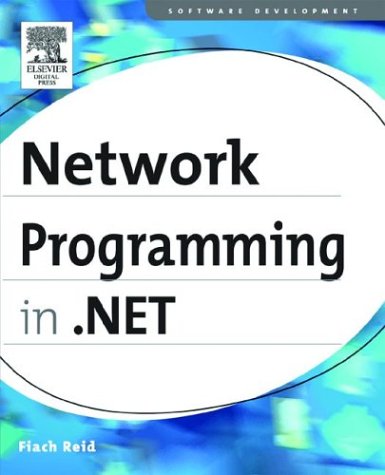
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PenThreadPool.cs
- CompressionTracing.cs
- GZipStream.cs
- HandledMouseEvent.cs
- ProcessModuleCollection.cs
- MouseActionValueSerializer.cs
- TypeSystem.cs
- ConstructorBuilder.cs
- SecurityElement.cs
- DataGridDesigner.cs
- DataMisalignedException.cs
- InheritedPropertyChangedEventArgs.cs
- OutputCacheSettingsSection.cs
- EventMetadata.cs
- LinqToSqlWrapper.cs
- CustomCredentialPolicy.cs
- TreeNodeBindingCollection.cs
- LateBoundBitmapDecoder.cs
- PenLineCapValidation.cs
- DuplicateMessageDetector.cs
- XmlValidatingReader.cs
- DynamicILGenerator.cs
- CFGGrammar.cs
- StateWorkerRequest.cs
- EventListener.cs
- HtmlFormWrapper.cs
- CodeEntryPointMethod.cs
- CompositeActivityValidator.cs
- StateChangeEvent.cs
- Msec.cs
- CompoundFileIOPermission.cs
- WebPartHelpVerb.cs
- HwndAppCommandInputProvider.cs
- WorkflowTimerService.cs
- ThemeDictionaryExtension.cs
- CatalogPartCollection.cs
- MouseBinding.cs
- mediaeventargs.cs
- SchemaTypeEmitter.cs
- DataGridPagerStyle.cs
- ServerIdentity.cs
- ZipIOModeEnforcingStream.cs
- CapabilitiesSection.cs
- SqlDataAdapter.cs
- IODescriptionAttribute.cs
- ServiceBehaviorElementCollection.cs
- BorderGapMaskConverter.cs
- GraphicsState.cs
- JsonSerializer.cs
- PauseStoryboard.cs
- RouteItem.cs
- GeneralTransform2DTo3D.cs
- ValidatorUtils.cs
- ZoneMembershipCondition.cs
- ToolBarButton.cs
- DesignerActionUIStateChangeEventArgs.cs
- TabControl.cs
- WindowsListViewGroupHelper.cs
- CollectionViewGroupRoot.cs
- URLString.cs
- CodeParameterDeclarationExpression.cs
- TableCellCollection.cs
- ToolBar.cs
- Encoder.cs
- XmlUtil.cs
- Int16.cs
- FaultConverter.cs
- ObjectHandle.cs
- ImageAnimator.cs
- XmlILModule.cs
- ObjectDataSourceView.cs
- CompressionTransform.cs
- UriParserTemplates.cs
- SmtpMail.cs
- SortFieldComparer.cs
- OdbcConnectionHandle.cs
- ProfileSettings.cs
- DragEventArgs.cs
- Ref.cs
- XdrBuilder.cs
- DataList.cs
- MediaScriptCommandRoutedEventArgs.cs
- PublishLicense.cs
- ListViewSelectEventArgs.cs
- ServiceReference.cs
- DataServiceOperationContext.cs
- PriorityBindingExpression.cs
- AuthenticationManager.cs
- TextPointer.cs
- SQLBytes.cs
- RecordBuilder.cs
- DataGridViewColumnEventArgs.cs
- GridViewRowCollection.cs
- MessagePropertyVariants.cs
- CompilerHelpers.cs
- SurrogateChar.cs
- PerfCounterSection.cs
- TickBar.cs
- CellNormalizer.cs
- SiteMapNode.cs