Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media3D / GeneralTransform2DTo3D.cs / 1305600 / GeneralTransform2DTo3D.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Declaration of the GeneralTransform2DTo3D class. // //--------------------------------------------------------------------------- using System.Diagnostics; using System.Windows.Media; using System.Windows.Media.Animation; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Media3D { ////// TransformTo3D class provides services to transform points from 2D in to 3D /// public class GeneralTransform2DTo3D : Freezable { internal GeneralTransform2DTo3D() { } internal GeneralTransform2DTo3D(GeneralTransform transform2D, Viewport2DVisual3D containingVisual3D, GeneralTransform3D transform3D) { Visual child = containingVisual3D.Visual; Debug.Assert(child != null, "Going from 2D to 3D containingVisual3D.Visual should not be null"); _transform3D = (GeneralTransform3D)transform3D.GetCurrentValueAsFrozen(); // we also need to go one more level up to handle a transform being placed // on the Viewport2DVisual3D's child GeneralTransformGroup transformGroup = new GeneralTransformGroup(); transformGroup.Children.Add((GeneralTransform)transform2D.GetCurrentValueAsFrozen()); transformGroup.Children.Add((GeneralTransform)child.TransformToOuterSpace().GetCurrentValueAsFrozen()); transformGroup.Freeze(); _transform2D = transformGroup; _positions = containingVisual3D.InternalPositionsCache; _textureCoords = containingVisual3D.InternalTextureCoordinatesCache; _triIndices = containingVisual3D.InternalTriangleIndicesCache; _childBounds = child.CalculateSubgraphRenderBoundsOuterSpace(); } ////// Transform a point /// /// Input point /// Output point ///True if the point was transformed successfuly, false otherwise public bool TryTransform(Point inPoint, out Point3D result) { Point final2DPoint; // assign this now so that we can return false if needed result = new Point3D(); if (!_transform2D.TryTransform(inPoint, out final2DPoint)) { return false; } Point texCoord = Viewport2DVisual3D.VisualCoordsToTextureCoords(final2DPoint, _childBounds); // need to walk the texture coordinates on the Viewport2DVisual3D // and look for where this point intersects one of them Point3D coordPoint; if (!Viewport2DVisual3D.Get3DPointFor2DCoordinate(texCoord, out coordPoint, _positions, _textureCoords, _triIndices)) { return false; } if (!_transform3D.TryTransform(coordPoint, out result)) { return false; } return true; } ////// Transform a point from 2D in to 3D /// /// If the transformation does not succeed, this will throw an InvalidOperationException. /// If you don't want to try/catch, call TryTransform instead and check the boolean it /// returns. /// /// /// Input point ///The transformed point public Point3D Transform(Point point) { Point3D transformedPoint; if (!TryTransform(point, out transformedPoint)) { throw new InvalidOperationException(SR.Get(SRID.GeneralTransform_TransformFailed, null)); } return transformedPoint; } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new GeneralTransform2DTo3D(); } ////// Implementation of /// protected override void CloneCore(Freezable sourceFreezable) { GeneralTransform2DTo3D transform = (GeneralTransform2DTo3D)sourceFreezable; base.CloneCore(sourceFreezable); CopyCommon(transform); } ///Freezable.CloneCore . ////// Implementation of /// protected override void CloneCurrentValueCore(Freezable sourceFreezable) { GeneralTransform2DTo3D transform = (GeneralTransform2DTo3D)sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); CopyCommon(transform); } ///Freezable.CloneCurrentValueCore . ////// Implementation of /// protected override void GetAsFrozenCore(Freezable sourceFreezable) { GeneralTransform2DTo3D transform = (GeneralTransform2DTo3D)sourceFreezable; base.GetAsFrozenCore(sourceFreezable); CopyCommon(transform); } ///Freezable.GetAsFrozenCore . ////// Implementation of /// protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { GeneralTransform2DTo3D transform = (GeneralTransform2DTo3D)sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); CopyCommon(transform); } ///Freezable.GetCurrentValueAsFrozenCore . ////// Clones values that do not have corresponding DPs /// /// private void CopyCommon(GeneralTransform2DTo3D transform) { _transform2D = transform._transform2D; _transform3D = transform._transform3D; _positions = transform._positions; _textureCoords = transform._textureCoords; _triIndices = transform._triIndices; _childBounds = transform._childBounds; } private GeneralTransform _transform2D; private GeneralTransform3D _transform3D; private Point3DCollection _positions; private PointCollection _textureCoords; private Int32Collection _triIndices; private Rect _childBounds; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
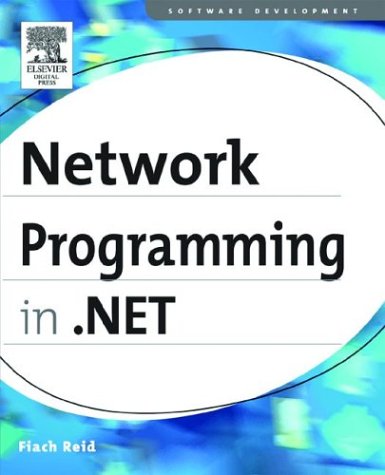
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StorageEntityTypeMapping.cs
- GZipDecoder.cs
- SamlAuthenticationClaimResource.cs
- ListSourceHelper.cs
- KeyValuePairs.cs
- SubMenuStyleCollection.cs
- LinearGradientBrush.cs
- LinqDataSourceUpdateEventArgs.cs
- VoiceChangeEventArgs.cs
- CellCreator.cs
- HttpListenerRequest.cs
- MessagePropertyFilter.cs
- EndPoint.cs
- SortedSet.cs
- MenuCommands.cs
- PropertyItem.cs
- autovalidator.cs
- SoapAttributeAttribute.cs
- HttpListenerContext.cs
- Item.cs
- ClientUrlResolverWrapper.cs
- RegexCode.cs
- XPathNodeHelper.cs
- HiddenField.cs
- ToolStripDropDownDesigner.cs
- DataServiceStreamResponse.cs
- WizardDesigner.cs
- PreloadedPackages.cs
- HeaderLabel.cs
- DefaultHttpHandler.cs
- FrameworkElementFactory.cs
- ArrayConverter.cs
- QuestionEventArgs.cs
- FixedHighlight.cs
- DateTimeParse.cs
- StylusPlugin.cs
- DataGridViewToolTip.cs
- BamlResourceContent.cs
- PanelStyle.cs
- NativeMethods.cs
- PageThemeBuildProvider.cs
- UpnEndpointIdentityExtension.cs
- Pair.cs
- Query.cs
- MappingMetadataHelper.cs
- RequestQueue.cs
- AttributeCollection.cs
- PieceDirectory.cs
- Bold.cs
- OleDbException.cs
- FontWeight.cs
- LinkClickEvent.cs
- Control.cs
- StringAnimationUsingKeyFrames.cs
- SqlMultiplexer.cs
- XamlFilter.cs
- DataIdProcessor.cs
- LogWriteRestartAreaState.cs
- DiagnosticTraceSource.cs
- TextWriterTraceListener.cs
- Odbc32.cs
- UniqueConstraint.cs
- StateMachineAction.cs
- DependencyObjectPropertyDescriptor.cs
- DataTableNameHandler.cs
- ArglessEventHandlerProxy.cs
- XdrBuilder.cs
- SpeechSeg.cs
- ThumbButtonInfoCollection.cs
- HandledEventArgs.cs
- NumericUpDownAccelerationCollection.cs
- AddingNewEventArgs.cs
- ApplyImportsAction.cs
- OleDbReferenceCollection.cs
- Validator.cs
- DropTarget.cs
- SrgsElementFactory.cs
- RequestContext.cs
- RadioButtonAutomationPeer.cs
- ParentUndoUnit.cs
- SectionUpdates.cs
- SchemaCreator.cs
- TableCell.cs
- ChangeInterceptorAttribute.cs
- ActivityScheduledQuery.cs
- Header.cs
- BitmapCache.cs
- DetailsViewInsertedEventArgs.cs
- Completion.cs
- ResourceDescriptionAttribute.cs
- TreeNodeMouseHoverEvent.cs
- HttpListener.cs
- DynamicValidator.cs
- _NegotiateClient.cs
- SpotLight.cs
- Line.cs
- XmlIlGenerator.cs
- GradientSpreadMethodValidation.cs
- ProgressBarHighlightConverter.cs
- FontStretches.cs