Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / Utils / Pair.cs / 1305376 / Pair.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Linq; namespace System.Data.Common.Utils { internal class Pair: InternalBase { #region Fields private readonly TFirst first; private readonly TSecond second; #endregion #region Constructor internal Pair(TFirst first, TSecond second) { this.first = first; this.second = second; } #endregion #region Properties internal TFirst First { get { return first; } } internal TSecond Second { get { return second; } } #endregion #region Methods public override int GetHashCode() { return (first.GetHashCode()<<5) ^ second.GetHashCode(); } public bool Equals(Pair other) { return first.Equals(other.first) && second.Equals(other.second); } public override bool Equals(object other) { Pair otherPair = other as Pair ; return (otherPair != null && Equals(otherPair)); } #endregion #region InternalBase internal override void ToCompactString(StringBuilder builder) { builder.Append("<"); builder.Append(first.ToString()); builder.Append(", "+second.ToString()); builder.Append(">"); } #endregion internal class PairComparer : IEqualityComparer > { private PairComparer() { } internal static readonly PairComparer Instance = new PairComparer(); private static readonly EqualityComparer firstComparer = EqualityComparer .Default; private static readonly EqualityComparer secondComparer = EqualityComparer .Default; public bool Equals(Pair x, Pair y) { return firstComparer.Equals(x.First, y.First) && secondComparer.Equals(x.Second, y.Second); } public int GetHashCode(Pair source) { return source.GetHashCode(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Linq; namespace System.Data.Common.Utils { internal class Pair: InternalBase { #region Fields private readonly TFirst first; private readonly TSecond second; #endregion #region Constructor internal Pair(TFirst first, TSecond second) { this.first = first; this.second = second; } #endregion #region Properties internal TFirst First { get { return first; } } internal TSecond Second { get { return second; } } #endregion #region Methods public override int GetHashCode() { return (first.GetHashCode()<<5) ^ second.GetHashCode(); } public bool Equals(Pair other) { return first.Equals(other.first) && second.Equals(other.second); } public override bool Equals(object other) { Pair otherPair = other as Pair ; return (otherPair != null && Equals(otherPair)); } #endregion #region InternalBase internal override void ToCompactString(StringBuilder builder) { builder.Append("<"); builder.Append(first.ToString()); builder.Append(", "+second.ToString()); builder.Append(">"); } #endregion internal class PairComparer : IEqualityComparer > { private PairComparer() { } internal static readonly PairComparer Instance = new PairComparer(); private static readonly EqualityComparer firstComparer = EqualityComparer .Default; private static readonly EqualityComparer secondComparer = EqualityComparer .Default; public bool Equals(Pair x, Pair y) { return firstComparer.Equals(x.First, y.First) && secondComparer.Equals(x.Second, y.Second); } public int GetHashCode(Pair source) { return source.GetHashCode(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
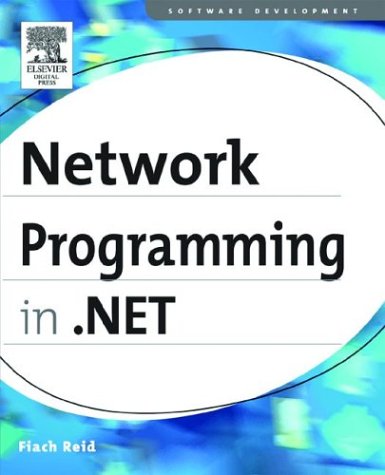
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BindUriHelper.cs
- ExtendedPropertyCollection.cs
- TabControlDesigner.cs
- ECDiffieHellmanPublicKey.cs
- Attributes.cs
- NativeMethods.cs
- LinkTarget.cs
- StaticContext.cs
- UnsafeNativeMethodsPenimc.cs
- XmlLanguage.cs
- DataException.cs
- SystemBrushes.cs
- WindowsScrollBar.cs
- mda.cs
- SrgsSemanticInterpretationTag.cs
- StringFreezingAttribute.cs
- TextEffectResolver.cs
- XmlSecureResolver.cs
- TypeResolver.cs
- RawStylusInputReport.cs
- DiscriminatorMap.cs
- RTTrackingProfile.cs
- RelationshipEntry.cs
- SqlDataSourceCache.cs
- XmlReflectionImporter.cs
- WindowsAuthenticationEventArgs.cs
- GeneralTransform3D.cs
- FontInfo.cs
- XmlExceptionHelper.cs
- HtmlControlPersistable.cs
- DataGridComboBoxColumn.cs
- GridViewRow.cs
- WindowPattern.cs
- PageRanges.cs
- RsaSecurityKey.cs
- ImmutableObjectAttribute.cs
- ToolboxItem.cs
- XamlSerializerUtil.cs
- EncryptedData.cs
- SafeFileMapViewHandle.cs
- Win32Interop.cs
- HttpCapabilitiesBase.cs
- InvalidAsynchronousStateException.cs
- MobileDeviceCapabilitiesSectionHandler.cs
- _CacheStreams.cs
- _SpnDictionary.cs
- SspiWrapper.cs
- InputLanguageEventArgs.cs
- ToolStripItemEventArgs.cs
- BitmapEffectGeneralTransform.cs
- DefaultAuthorizationContext.cs
- ButtonBase.cs
- Section.cs
- UserControl.cs
- RepeaterItemEventArgs.cs
- Compilation.cs
- DataServices.cs
- KeyPullup.cs
- XmlDataSourceView.cs
- SystemIPGlobalProperties.cs
- RemoteWebConfigurationHostServer.cs
- DescendentsWalker.cs
- BaseAppDomainProtocolHandler.cs
- FragmentNavigationEventArgs.cs
- MetadataCacheItem.cs
- CapiHashAlgorithm.cs
- GlobalizationAssembly.cs
- EUCJPEncoding.cs
- PropertyMap.cs
- RawUIStateInputReport.cs
- D3DImage.cs
- TileModeValidation.cs
- ToolStripLabel.cs
- DockPattern.cs
- XmlSchemaInclude.cs
- TransformGroup.cs
- Token.cs
- VectorKeyFrameCollection.cs
- BlockUIContainer.cs
- AuthorizationRule.cs
- WasEndpointConfigContainer.cs
- Canvas.cs
- OwnerDrawPropertyBag.cs
- DoubleLinkList.cs
- MultipleViewPatternIdentifiers.cs
- SiteMapDataSource.cs
- GridViewCancelEditEventArgs.cs
- FormsAuthenticationUserCollection.cs
- ClockGroup.cs
- MenuItemStyle.cs
- ObjectReaderCompiler.cs
- MD5CryptoServiceProvider.cs
- DeobfuscatingStream.cs
- UdpTransportSettingsElement.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- SafeHandles.cs
- DataServiceStreamResponse.cs
- propertytag.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- Encoder.cs