Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Animation / Generated / VectorKeyFrameCollection.cs / 1305600 / VectorKeyFrameCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; namespace System.Windows.Media.Animation { ////// This collection is used in conjunction with a KeyFrameVectorAnimation /// to animate a Vector property value along a set of key frames. /// public class VectorKeyFrameCollection : Freezable, IList { #region Data private List_keyFrames; private static VectorKeyFrameCollection s_emptyCollection; #endregion #region Constructors /// /// Creates a new VectorKeyFrameCollection. /// public VectorKeyFrameCollection() : base() { _keyFrames = new List< VectorKeyFrame>(2); } #endregion #region Static Methods ////// An empty VectorKeyFrameCollection. /// public static VectorKeyFrameCollection Empty { get { if (s_emptyCollection == null) { VectorKeyFrameCollection emptyCollection = new VectorKeyFrameCollection(); emptyCollection._keyFrames = new List< VectorKeyFrame>(0); emptyCollection.Freeze(); s_emptyCollection = emptyCollection; } return s_emptyCollection; } } #endregion #region Freezable ////// Creates a freezable copy of this VectorKeyFrameCollection. /// ///The copy public new VectorKeyFrameCollection Clone() { return (VectorKeyFrameCollection)base.Clone(); } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new VectorKeyFrameCollection(); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { VectorKeyFrameCollection sourceCollection = (VectorKeyFrameCollection) sourceFreezable; base.CloneCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< VectorKeyFrame>(count); for (int i = 0; i < count; i++) { VectorKeyFrame keyFrame = (VectorKeyFrame)sourceCollection._keyFrames[i].Clone(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { VectorKeyFrameCollection sourceCollection = (VectorKeyFrameCollection) sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< VectorKeyFrame>(count); for (int i = 0; i < count; i++) { VectorKeyFrame keyFrame = (VectorKeyFrame)sourceCollection._keyFrames[i].CloneCurrentValue(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { VectorKeyFrameCollection sourceCollection = (VectorKeyFrameCollection) sourceFreezable; base.GetAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< VectorKeyFrame>(count); for (int i = 0; i < count; i++) { VectorKeyFrame keyFrame = (VectorKeyFrame)sourceCollection._keyFrames[i].GetAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { VectorKeyFrameCollection sourceCollection = (VectorKeyFrameCollection) sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< VectorKeyFrame>(count); for (int i = 0; i < count; i++) { VectorKeyFrame keyFrame = (VectorKeyFrame)sourceCollection._keyFrames[i].GetCurrentValueAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetCurrentValueAsFrozenCore . ////// /// protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); for (int i = 0; i < _keyFrames.Count && canFreeze; i++) { canFreeze &= Freezable.Freeze(_keyFrames[i], isChecking); } return canFreeze; } #endregion #region IEnumerable ////// Returns an enumerator of the VectorKeyFrames in the collection. /// public IEnumerator GetEnumerator() { ReadPreamble(); return _keyFrames.GetEnumerator(); } #endregion #region ICollection ////// Returns the number of VectorKeyFrames in the collection. /// public int Count { get { ReadPreamble(); return _keyFrames.Count; } } ////// See public bool IsSynchronized { get { ReadPreamble(); return (IsFrozen || Dispatcher != null); } } ///ICollection.IsSynchronized . ////// See public object SyncRoot { get { ReadPreamble(); return ((ICollection)_keyFrames).SyncRoot; } } ///ICollection.SyncRoot . ////// Copies all of the VectorKeyFrames in the collection to an /// array. /// void ICollection.CopyTo(Array array, int index) { ReadPreamble(); ((ICollection)_keyFrames).CopyTo(array, index); } ////// Copies all of the VectorKeyFrames in the collection to an /// array of VectorKeyFrames. /// public void CopyTo(VectorKeyFrame[] array, int index) { ReadPreamble(); _keyFrames.CopyTo(array, index); } #endregion #region IList ////// Adds a VectorKeyFrame to the collection. /// int IList.Add(object keyFrame) { return Add((VectorKeyFrame)keyFrame); } ////// Adds a VectorKeyFrame to the collection. /// public int Add(VectorKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Add(keyFrame); WritePostscript(); return _keyFrames.Count - 1; } ////// Removes all VectorKeyFrames from the collection. /// public void Clear() { WritePreamble(); if (_keyFrames.Count > 0) { for (int i = 0; i < _keyFrames.Count; i++) { OnFreezablePropertyChanged(_keyFrames[i], null); } _keyFrames.Clear(); WritePostscript(); } } ////// Returns true of the collection contains the given VectorKeyFrame. /// bool IList.Contains(object keyFrame) { return Contains((VectorKeyFrame)keyFrame); } ////// Returns true of the collection contains the given VectorKeyFrame. /// public bool Contains(VectorKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.Contains(keyFrame); } ////// Returns the index of a given VectorKeyFrame in the collection. /// int IList.IndexOf(object keyFrame) { return IndexOf((VectorKeyFrame)keyFrame); } ////// Returns the index of a given VectorKeyFrame in the collection. /// public int IndexOf(VectorKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.IndexOf(keyFrame); } ////// Inserts a VectorKeyFrame into a specific location in the collection. /// void IList.Insert(int index, object keyFrame) { Insert(index, (VectorKeyFrame)keyFrame); } ////// Inserts a VectorKeyFrame into a specific location in the collection. /// public void Insert(int index, VectorKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Insert(index, keyFrame); WritePostscript(); } ////// Returns true if the collection is frozen. /// public bool IsFixedSize { get { ReadPreamble(); return IsFrozen; } } ////// Returns true if the collection is frozen. /// public bool IsReadOnly { get { ReadPreamble(); return IsFrozen; } } ////// Removes a VectorKeyFrame from the collection. /// void IList.Remove(object keyFrame) { Remove((VectorKeyFrame)keyFrame); } ////// Removes a VectorKeyFrame from the collection. /// public void Remove(VectorKeyFrame keyFrame) { WritePreamble(); if (_keyFrames.Contains(keyFrame)) { OnFreezablePropertyChanged(keyFrame, null); _keyFrames.Remove(keyFrame); WritePostscript(); } } ////// Removes the VectorKeyFrame at the specified index from the collection. /// public void RemoveAt(int index) { WritePreamble(); OnFreezablePropertyChanged(_keyFrames[index], null); _keyFrames.RemoveAt(index); WritePostscript(); } ////// Gets or sets the VectorKeyFrame at a given index. /// object IList.this[int index] { get { return this[index]; } set { this[index] = (VectorKeyFrame)value; } } ////// Gets or sets the VectorKeyFrame at a given index. /// public VectorKeyFrame this[int index] { get { ReadPreamble(); return _keyFrames[index]; } set { if (value == null) { throw new ArgumentNullException(String.Format(CultureInfo.InvariantCulture, "VectorKeyFrameCollection[{0}]", index)); } WritePreamble(); if (value != _keyFrames[index]) { OnFreezablePropertyChanged(_keyFrames[index], value); _keyFrames[index] = value; Debug.Assert(_keyFrames[index] != null); WritePostscript(); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; namespace System.Windows.Media.Animation { ////// This collection is used in conjunction with a KeyFrameVectorAnimation /// to animate a Vector property value along a set of key frames. /// public class VectorKeyFrameCollection : Freezable, IList { #region Data private List_keyFrames; private static VectorKeyFrameCollection s_emptyCollection; #endregion #region Constructors /// /// Creates a new VectorKeyFrameCollection. /// public VectorKeyFrameCollection() : base() { _keyFrames = new List< VectorKeyFrame>(2); } #endregion #region Static Methods ////// An empty VectorKeyFrameCollection. /// public static VectorKeyFrameCollection Empty { get { if (s_emptyCollection == null) { VectorKeyFrameCollection emptyCollection = new VectorKeyFrameCollection(); emptyCollection._keyFrames = new List< VectorKeyFrame>(0); emptyCollection.Freeze(); s_emptyCollection = emptyCollection; } return s_emptyCollection; } } #endregion #region Freezable ////// Creates a freezable copy of this VectorKeyFrameCollection. /// ///The copy public new VectorKeyFrameCollection Clone() { return (VectorKeyFrameCollection)base.Clone(); } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new VectorKeyFrameCollection(); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { VectorKeyFrameCollection sourceCollection = (VectorKeyFrameCollection) sourceFreezable; base.CloneCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< VectorKeyFrame>(count); for (int i = 0; i < count; i++) { VectorKeyFrame keyFrame = (VectorKeyFrame)sourceCollection._keyFrames[i].Clone(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { VectorKeyFrameCollection sourceCollection = (VectorKeyFrameCollection) sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< VectorKeyFrame>(count); for (int i = 0; i < count; i++) { VectorKeyFrame keyFrame = (VectorKeyFrame)sourceCollection._keyFrames[i].CloneCurrentValue(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { VectorKeyFrameCollection sourceCollection = (VectorKeyFrameCollection) sourceFreezable; base.GetAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< VectorKeyFrame>(count); for (int i = 0; i < count; i++) { VectorKeyFrame keyFrame = (VectorKeyFrame)sourceCollection._keyFrames[i].GetAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { VectorKeyFrameCollection sourceCollection = (VectorKeyFrameCollection) sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< VectorKeyFrame>(count); for (int i = 0; i < count; i++) { VectorKeyFrame keyFrame = (VectorKeyFrame)sourceCollection._keyFrames[i].GetCurrentValueAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetCurrentValueAsFrozenCore . ////// /// protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); for (int i = 0; i < _keyFrames.Count && canFreeze; i++) { canFreeze &= Freezable.Freeze(_keyFrames[i], isChecking); } return canFreeze; } #endregion #region IEnumerable ////// Returns an enumerator of the VectorKeyFrames in the collection. /// public IEnumerator GetEnumerator() { ReadPreamble(); return _keyFrames.GetEnumerator(); } #endregion #region ICollection ////// Returns the number of VectorKeyFrames in the collection. /// public int Count { get { ReadPreamble(); return _keyFrames.Count; } } ////// See public bool IsSynchronized { get { ReadPreamble(); return (IsFrozen || Dispatcher != null); } } ///ICollection.IsSynchronized . ////// See public object SyncRoot { get { ReadPreamble(); return ((ICollection)_keyFrames).SyncRoot; } } ///ICollection.SyncRoot . ////// Copies all of the VectorKeyFrames in the collection to an /// array. /// void ICollection.CopyTo(Array array, int index) { ReadPreamble(); ((ICollection)_keyFrames).CopyTo(array, index); } ////// Copies all of the VectorKeyFrames in the collection to an /// array of VectorKeyFrames. /// public void CopyTo(VectorKeyFrame[] array, int index) { ReadPreamble(); _keyFrames.CopyTo(array, index); } #endregion #region IList ////// Adds a VectorKeyFrame to the collection. /// int IList.Add(object keyFrame) { return Add((VectorKeyFrame)keyFrame); } ////// Adds a VectorKeyFrame to the collection. /// public int Add(VectorKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Add(keyFrame); WritePostscript(); return _keyFrames.Count - 1; } ////// Removes all VectorKeyFrames from the collection. /// public void Clear() { WritePreamble(); if (_keyFrames.Count > 0) { for (int i = 0; i < _keyFrames.Count; i++) { OnFreezablePropertyChanged(_keyFrames[i], null); } _keyFrames.Clear(); WritePostscript(); } } ////// Returns true of the collection contains the given VectorKeyFrame. /// bool IList.Contains(object keyFrame) { return Contains((VectorKeyFrame)keyFrame); } ////// Returns true of the collection contains the given VectorKeyFrame. /// public bool Contains(VectorKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.Contains(keyFrame); } ////// Returns the index of a given VectorKeyFrame in the collection. /// int IList.IndexOf(object keyFrame) { return IndexOf((VectorKeyFrame)keyFrame); } ////// Returns the index of a given VectorKeyFrame in the collection. /// public int IndexOf(VectorKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.IndexOf(keyFrame); } ////// Inserts a VectorKeyFrame into a specific location in the collection. /// void IList.Insert(int index, object keyFrame) { Insert(index, (VectorKeyFrame)keyFrame); } ////// Inserts a VectorKeyFrame into a specific location in the collection. /// public void Insert(int index, VectorKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Insert(index, keyFrame); WritePostscript(); } ////// Returns true if the collection is frozen. /// public bool IsFixedSize { get { ReadPreamble(); return IsFrozen; } } ////// Returns true if the collection is frozen. /// public bool IsReadOnly { get { ReadPreamble(); return IsFrozen; } } ////// Removes a VectorKeyFrame from the collection. /// void IList.Remove(object keyFrame) { Remove((VectorKeyFrame)keyFrame); } ////// Removes a VectorKeyFrame from the collection. /// public void Remove(VectorKeyFrame keyFrame) { WritePreamble(); if (_keyFrames.Contains(keyFrame)) { OnFreezablePropertyChanged(keyFrame, null); _keyFrames.Remove(keyFrame); WritePostscript(); } } ////// Removes the VectorKeyFrame at the specified index from the collection. /// public void RemoveAt(int index) { WritePreamble(); OnFreezablePropertyChanged(_keyFrames[index], null); _keyFrames.RemoveAt(index); WritePostscript(); } ////// Gets or sets the VectorKeyFrame at a given index. /// object IList.this[int index] { get { return this[index]; } set { this[index] = (VectorKeyFrame)value; } } ////// Gets or sets the VectorKeyFrame at a given index. /// public VectorKeyFrame this[int index] { get { ReadPreamble(); return _keyFrames[index]; } set { if (value == null) { throw new ArgumentNullException(String.Format(CultureInfo.InvariantCulture, "VectorKeyFrameCollection[{0}]", index)); } WritePreamble(); if (value != _keyFrames[index]) { OnFreezablePropertyChanged(_keyFrames[index], value); _keyFrames[index] = value; Debug.Assert(_keyFrames[index] != null); WritePostscript(); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
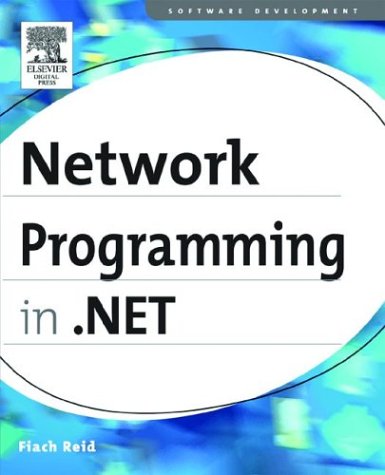
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RepeaterItemEventArgs.cs
- SmtpNegotiateAuthenticationModule.cs
- BlobPersonalizationState.cs
- CompilationRelaxations.cs
- IteratorFilter.cs
- XamlDesignerSerializationManager.cs
- Pair.cs
- ProcessThread.cs
- CommonXSendMessage.cs
- TextTreeExtractElementUndoUnit.cs
- Sql8ConformanceChecker.cs
- DesignerDataSchemaClass.cs
- JoinQueryOperator.cs
- ControlPager.cs
- Setter.cs
- MSAAWinEventWrap.cs
- BitmapData.cs
- BounceEase.cs
- LabelAutomationPeer.cs
- DataGridColumnStyleMappingNameEditor.cs
- OutOfMemoryException.cs
- ColumnResizeAdorner.cs
- Helpers.cs
- DecimalAnimationBase.cs
- CompositeScriptReferenceEventArgs.cs
- ToolboxItem.cs
- InvalidCastException.cs
- SqlDataSourceStatusEventArgs.cs
- Section.cs
- VerticalAlignConverter.cs
- InternalControlCollection.cs
- Int16AnimationUsingKeyFrames.cs
- Int64AnimationBase.cs
- RuleValidation.cs
- ButtonBase.cs
- AutomationEventArgs.cs
- PrefixQName.cs
- RandomNumberGenerator.cs
- DotAtomReader.cs
- ServiceInfo.cs
- SqlFacetAttribute.cs
- ProjectionPlanCompiler.cs
- ContentDisposition.cs
- ProcessProtocolHandler.cs
- TypeDescriptor.cs
- ConsoleKeyInfo.cs
- Input.cs
- HttpDictionary.cs
- PngBitmapDecoder.cs
- SerializationInfoEnumerator.cs
- Dictionary.cs
- AppSecurityManager.cs
- FrameworkElement.cs
- HeaderCollection.cs
- SqlConnectionStringBuilder.cs
- TypeConverterAttribute.cs
- Facet.cs
- PropertyGridView.cs
- LookupNode.cs
- StringHelper.cs
- ControlCachePolicy.cs
- RelationshipNavigation.cs
- IconConverter.cs
- DataControlPagerLinkButton.cs
- HtmlLiteralTextAdapter.cs
- XmlReflectionImporter.cs
- OptimizerPatterns.cs
- ProbeDuplex11AsyncResult.cs
- RubberbandSelector.cs
- X509CertificateChain.cs
- SourceElementsCollection.cs
- SamlAttributeStatement.cs
- AsyncResult.cs
- GradientStopCollection.cs
- XmlConverter.cs
- OdbcConnectionOpen.cs
- wmiutil.cs
- HtmlInputReset.cs
- NoneExcludedImageIndexConverter.cs
- WorkflowInstanceProvider.cs
- ElementAction.cs
- WebPartHeaderCloseVerb.cs
- ThreadSafeList.cs
- SecurityException.cs
- MouseOverProperty.cs
- ByteStreamGeometryContext.cs
- XmlDictionaryString.cs
- RepeaterItemCollection.cs
- OracleString.cs
- DataGridViewRowHeaderCell.cs
- ConfigurationValidatorAttribute.cs
- IPEndPointCollection.cs
- LineInfo.cs
- Options.cs
- FullTrustAssembliesSection.cs
- DispatcherTimer.cs
- ApplicationCommands.cs
- UserPersonalizationStateInfo.cs
- MetadataCollection.cs
- _NegoStream.cs