Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / MS / Internal / Shaping / ShapeTypeface.cs / 1305600 / ShapeTypeface.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2002 // // File: ShapeTypeface.cs // // Contents: GlyphTypeface with shaping capability // // Created: 10-13-2003 Worachai Chaoweeraprasit (wchao) // //----------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Collections; using System.Collections.Generic; using System.Globalization; using System.Diagnostics; using System.Security; using System.Windows; using System.Windows.Media; using System.Windows.Media.TextFormatting; using MS.Utility; using MS.Internal; using MS.Internal.FontCache; using MS.Internal.FontFace; using MS.Internal.TextFormatting; using FontFace = MS.Internal.FontFace; namespace MS.Internal.Shaping { ////// Typeface that is capable of shaping character string. Shaping is done /// thru shaping engines. /// internal class ShapeTypeface { private GlyphTypeface _glyphTypeface; private IDeviceFont _deviceFont; internal ShapeTypeface( GlyphTypeface glyphTypeface, IDeviceFont deviceFont ) { Invariant.Assert(glyphTypeface != null); _glyphTypeface = glyphTypeface; _deviceFont = deviceFont; } public override int GetHashCode() { return HashFn.HashMultiply(_glyphTypeface.GetHashCode()) + (_deviceFont == null ? 0 : _deviceFont.Name.GetHashCode()); } public override bool Equals(object o) { ShapeTypeface t = o as ShapeTypeface; if(t == null) return false; if (_deviceFont == null) { if (t._deviceFont != null) return false; } else { if (t._deviceFont == null || t._deviceFont.Name != _deviceFont.Name) return false; } return _glyphTypeface.Equals(t._glyphTypeface); } internal IDeviceFont DeviceFont { get { return _deviceFont; } } ////// Get physical font face /// internal GlyphTypeface GlyphTypeface { get { return _glyphTypeface; } } } ////// Scaled shape typeface /// internal class ScaledShapeTypeface { private ShapeTypeface _shapeTypeface; private double _scaleInEm; private bool _nullShape; internal ScaledShapeTypeface( GlyphTypeface glyphTypeface, IDeviceFont deviceFont, double scaleInEm, bool nullShape ) { _shapeTypeface = new ShapeTypeface(glyphTypeface, deviceFont); _scaleInEm = scaleInEm; _nullShape = nullShape; } internal ShapeTypeface ShapeTypeface { get { return _shapeTypeface; } } internal double ScaleInEm { get { return _scaleInEm; } } internal bool NullShape { get { return _nullShape; } } public override int GetHashCode() { int hash = _shapeTypeface.GetHashCode(); unsafe { hash = HashFn.HashMultiply(hash) + (int)(_nullShape ? 1 : 0); hash = HashFn.HashMultiply(hash) + _scaleInEm.GetHashCode(); return HashFn.HashScramble(hash); } } public override bool Equals(object o) { ScaledShapeTypeface t = o as ScaledShapeTypeface; if (t == null) return false; return _shapeTypeface.Equals(t._shapeTypeface) && _scaleInEm == t._scaleInEm && _nullShape == t._nullShape; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2002 // // File: ShapeTypeface.cs // // Contents: GlyphTypeface with shaping capability // // Created: 10-13-2003 Worachai Chaoweeraprasit (wchao) // //----------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Collections; using System.Collections.Generic; using System.Globalization; using System.Diagnostics; using System.Security; using System.Windows; using System.Windows.Media; using System.Windows.Media.TextFormatting; using MS.Utility; using MS.Internal; using MS.Internal.FontCache; using MS.Internal.FontFace; using MS.Internal.TextFormatting; using FontFace = MS.Internal.FontFace; namespace MS.Internal.Shaping { ////// Typeface that is capable of shaping character string. Shaping is done /// thru shaping engines. /// internal class ShapeTypeface { private GlyphTypeface _glyphTypeface; private IDeviceFont _deviceFont; internal ShapeTypeface( GlyphTypeface glyphTypeface, IDeviceFont deviceFont ) { Invariant.Assert(glyphTypeface != null); _glyphTypeface = glyphTypeface; _deviceFont = deviceFont; } public override int GetHashCode() { return HashFn.HashMultiply(_glyphTypeface.GetHashCode()) + (_deviceFont == null ? 0 : _deviceFont.Name.GetHashCode()); } public override bool Equals(object o) { ShapeTypeface t = o as ShapeTypeface; if(t == null) return false; if (_deviceFont == null) { if (t._deviceFont != null) return false; } else { if (t._deviceFont == null || t._deviceFont.Name != _deviceFont.Name) return false; } return _glyphTypeface.Equals(t._glyphTypeface); } internal IDeviceFont DeviceFont { get { return _deviceFont; } } ////// Get physical font face /// internal GlyphTypeface GlyphTypeface { get { return _glyphTypeface; } } } ////// Scaled shape typeface /// internal class ScaledShapeTypeface { private ShapeTypeface _shapeTypeface; private double _scaleInEm; private bool _nullShape; internal ScaledShapeTypeface( GlyphTypeface glyphTypeface, IDeviceFont deviceFont, double scaleInEm, bool nullShape ) { _shapeTypeface = new ShapeTypeface(glyphTypeface, deviceFont); _scaleInEm = scaleInEm; _nullShape = nullShape; } internal ShapeTypeface ShapeTypeface { get { return _shapeTypeface; } } internal double ScaleInEm { get { return _scaleInEm; } } internal bool NullShape { get { return _nullShape; } } public override int GetHashCode() { int hash = _shapeTypeface.GetHashCode(); unsafe { hash = HashFn.HashMultiply(hash) + (int)(_nullShape ? 1 : 0); hash = HashFn.HashMultiply(hash) + _scaleInEm.GetHashCode(); return HashFn.HashScramble(hash); } } public override bool Equals(object o) { ScaledShapeTypeface t = o as ScaledShapeTypeface; if (t == null) return false; return _shapeTypeface.Equals(t._shapeTypeface) && _scaleInEm == t._scaleInEm && _nullShape == t._nullShape; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
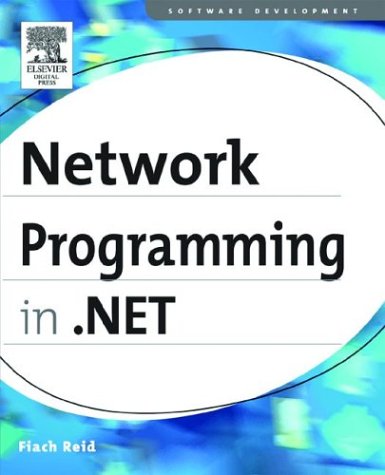
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- VectorAnimationUsingKeyFrames.cs
- VoiceChangeEventArgs.cs
- TextTreeTextElementNode.cs
- TextLineBreak.cs
- RepeaterItemEventArgs.cs
- BindingNavigator.cs
- CssTextWriter.cs
- QueryAsyncResult.cs
- HttpInputStream.cs
- HttpResponseHeader.cs
- EventRouteFactory.cs
- XmlDataCollection.cs
- SQLInt64Storage.cs
- AesCryptoServiceProvider.cs
- WrapPanel.cs
- PolyBezierSegment.cs
- VectorValueSerializer.cs
- SqlNotificationRequest.cs
- PeerApplication.cs
- DataObject.cs
- AuthorizationSection.cs
- TextDecorationLocationValidation.cs
- Guid.cs
- Helper.cs
- Source.cs
- TypeResolver.cs
- SelectedDatesCollection.cs
- TextEncodedRawTextWriter.cs
- ITreeGenerator.cs
- AspNetSynchronizationContext.cs
- WorkflowValidationFailedException.cs
- SeekStoryboard.cs
- MailWriter.cs
- TextBoxBase.cs
- SQLUtility.cs
- Matrix3DStack.cs
- WebRequestModuleElement.cs
- DataTemplate.cs
- TextBoxBase.cs
- ListSurrogate.cs
- XmlValidatingReader.cs
- X509Utils.cs
- AspNetSynchronizationContext.cs
- InputBuffer.cs
- LoggedException.cs
- xml.cs
- XmlSchemaExternal.cs
- BuildProviderUtils.cs
- DataGridViewColumnStateChangedEventArgs.cs
- BindValidator.cs
- Invariant.cs
- _StreamFramer.cs
- LocatorGroup.cs
- Symbol.cs
- ApplicationContext.cs
- TraceData.cs
- CompareValidator.cs
- XmlSchema.cs
- SafeFileMappingHandle.cs
- BindUriHelper.cs
- UriParserTemplates.cs
- RotationValidation.cs
- UriTemplateVariablePathSegment.cs
- ConfigXmlWhitespace.cs
- NominalTypeEliminator.cs
- MarshalByValueComponent.cs
- CodeTypeReferenceExpression.cs
- QilLiteral.cs
- TextTreeText.cs
- ImportOptions.cs
- ConsoleKeyInfo.cs
- StickyNote.cs
- NamespaceDecl.cs
- ObjectDataSource.cs
- ConfigurationManagerInternalFactory.cs
- ManagementOperationWatcher.cs
- Bold.cs
- RootAction.cs
- ContextStaticAttribute.cs
- GeneralTransform3D.cs
- ResourcePermissionBase.cs
- LinkedList.cs
- DrawingState.cs
- DbProviderFactoriesConfigurationHandler.cs
- TextDecorationCollectionConverter.cs
- WebBrowserProgressChangedEventHandler.cs
- SqlConnectionString.cs
- MessageEncoder.cs
- ViewGenerator.cs
- LogFlushAsyncResult.cs
- mda.cs
- parserscommon.cs
- printdlgexmarshaler.cs
- XmlILModule.cs
- OleDbErrorCollection.cs
- WebPartManagerInternals.cs
- AuthenticationException.cs
- HexParser.cs
- SqlColumnizer.cs
- UserControl.cs