Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CompMod / System / CodeDOM / Compiler / CompilerParameters.cs / 1305376 / CompilerParameters.cs
//------------------------------------------------------------------------------ //// // //----------------------------------------------------------------------------- namespace System.CodeDom.Compiler { using System; using System.CodeDom; using System.Collections; using System.Collections.Specialized; using Microsoft.Win32; using Microsoft.Win32.SafeHandles; using System.Runtime.InteropServices; using System.Security.Permissions; using System.Security.Policy; using System.Runtime.Serialization; using System.Runtime.Versioning; ///[....] // Copyright (c) Microsoft Corporation. All rights reserved. ///// [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] [PermissionSet(SecurityAction.InheritanceDemand, Name="FullTrust")] [Serializable] public class CompilerParameters { private StringCollection assemblyNames = new StringCollection(); [OptionalField] private StringCollection embeddedResources = new StringCollection(); [OptionalField] private StringCollection linkedResources = new StringCollection(); private string outputName; private string mainClass; private bool generateInMemory = false; private bool includeDebugInformation = false; private int warningLevel = -1; // -1 means not set (use compiler default) private string compilerOptions; private string win32Resource; private bool treatWarningsAsErrors = false; private bool generateExecutable = false; private TempFileCollection tempFiles; [NonSerializedAttribute] private SafeUserTokenHandle userToken; private Evidence evidence = null; ////// Represents the parameters used in to invoke the compiler. /// ////// [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] public CompilerParameters() : this(null, null) { } ////// Initializes a new instance of ///. /// /// [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] public CompilerParameters(string[] assemblyNames) : this(assemblyNames, null, false) { } ////// Initializes a new instance of ///using the specified /// assembly names. /// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public CompilerParameters(string[] assemblyNames, string outputName) : this(assemblyNames, outputName, false) { } ////// Initializes a new instance of ///using the specified /// assembly names and output name. /// /// [ResourceExposure(ResourceScope.Machine)] public CompilerParameters(string[] assemblyNames, string outputName, bool includeDebugInformation) { if (assemblyNames != null) { ReferencedAssemblies.AddRange(assemblyNames); } this.outputName = outputName; this.includeDebugInformation = includeDebugInformation; } ////// Initializes a new instance of ///using the specified /// assembly names, output name and a whether to include debug information flag. /// /// public bool GenerateExecutable { get { return generateExecutable; } set { generateExecutable = value; } } ////// Gets or sets whether to generate an executable. /// ////// public bool GenerateInMemory { get { return generateInMemory; } set { generateInMemory = value; } } ////// Gets or sets whether to generate in memory. /// ////// public StringCollection ReferencedAssemblies { get { return assemblyNames; } } ////// Gets or sets the assemblies referenced by the source to compile. /// ////// public string MainClass { get { return mainClass; } set { mainClass = value; } } ////// Gets or sets the main class. /// ////// public string OutputAssembly { [ResourceExposure(ResourceScope.Machine)] get { return outputName; } [ResourceExposure(ResourceScope.Machine)] set { outputName = value; } } ////// Gets or sets the output assembly. /// ////// public TempFileCollection TempFiles { get { if (tempFiles == null) tempFiles = new TempFileCollection(); return tempFiles; } set { tempFiles = value; } } ////// Gets or sets the temp files. /// ////// public bool IncludeDebugInformation { get { return includeDebugInformation; } set { includeDebugInformation = value; } } ////// Gets or sets whether to include debug information in the compiled /// executable. /// ////// public bool TreatWarningsAsErrors { get { return treatWarningsAsErrors; } set { treatWarningsAsErrors = value; } } ///[To be supplied.] ////// public int WarningLevel { get { return warningLevel; } set { warningLevel = value; } } ///[To be supplied.] ////// public string CompilerOptions { get { return compilerOptions; } set { compilerOptions = value; } } ///[To be supplied.] ////// public string Win32Resource { get { return win32Resource; } set { win32Resource = value; } } ///[To be supplied.] ////// [System.Runtime.InteropServices.ComVisible(false)] public StringCollection EmbeddedResources { get { return embeddedResources; } } ////// Gets or sets the resources to be compiled into the target /// ////// [System.Runtime.InteropServices.ComVisible(false)] public StringCollection LinkedResources { get { return linkedResources; } } ////// Gets or sets the linked resources /// ////// public IntPtr UserToken { get { if (userToken != null) return userToken.DangerousGetHandle(); else return IntPtr.Zero; } set { if (userToken != null) userToken.Close(); userToken = new SafeUserTokenHandle(value, false); } } internal SafeUserTokenHandle SafeUserToken { get { return userToken; } } ////// Gets or sets user token to be employed when creating the compiler process. /// ////// [Obsolete("CAS policy is obsolete and will be removed in a future release of the .NET Framework. Please see http://go2.microsoft.com/fwlink/?LinkId=131738 for more information.")] public Evidence Evidence { get { Evidence e = null; if (evidence != null) e = evidence.Clone(); return e; } [SecurityPermissionAttribute( SecurityAction.Demand, ControlEvidence = true )] set { if (value != null) evidence = value.Clone(); else evidence = null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Set the evidence for partially trusted scenarios. /// ///
Link Menu
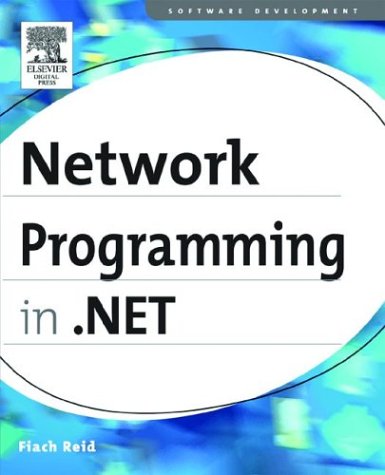
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Visual.cs
- QueryGenerator.cs
- ObjRef.cs
- FlowNode.cs
- ReadOnlyObservableCollection.cs
- SiteMapSection.cs
- GridViewDeleteEventArgs.cs
- RemoteWebConfigurationHostStream.cs
- XmlDesignerDataSourceView.cs
- SerTrace.cs
- DispatcherHooks.cs
- Helper.cs
- ProxyWebPartConnectionCollection.cs
- SqlCommandBuilder.cs
- LinkUtilities.cs
- FrameDimension.cs
- Compensate.cs
- FacetChecker.cs
- SystemIcmpV6Statistics.cs
- TextComposition.cs
- SecurityHelper.cs
- EditingScopeUndoUnit.cs
- CommonXSendMessage.cs
- StylusEventArgs.cs
- PageOutputColor.cs
- TableHeaderCell.cs
- BamlLocalizabilityResolver.cs
- AutomationPropertyInfo.cs
- Visitors.cs
- versioninfo.cs
- FacetDescription.cs
- HighlightVisual.cs
- ContainsRowNumberChecker.cs
- DomainUpDown.cs
- Bidi.cs
- BufferedReadStream.cs
- TextShapeableCharacters.cs
- _NetworkingPerfCounters.cs
- PlainXmlSerializer.cs
- Point3DAnimationBase.cs
- Serializer.cs
- BamlLocalizableResourceKey.cs
- ReaderWriterLock.cs
- DesignerToolStripControlHost.cs
- RepeaterDesigner.cs
- FormCollection.cs
- ActivationArguments.cs
- AssemblyBuilder.cs
- StorageConditionPropertyMapping.cs
- EffectiveValueEntry.cs
- commandenforcer.cs
- GridViewRowEventArgs.cs
- IconBitmapDecoder.cs
- SessionEndedEventArgs.cs
- CancellationHandlerDesigner.cs
- HttpPostedFileWrapper.cs
- infer.cs
- SmiContext.cs
- WorkflowStateRollbackService.cs
- FaultException.cs
- MimeBasePart.cs
- DBCommandBuilder.cs
- MissingFieldException.cs
- MimePart.cs
- ResourceManager.cs
- PbrsForward.cs
- PieceNameHelper.cs
- EventWaitHandle.cs
- LockedBorderGlyph.cs
- ConfigurationSectionGroupCollection.cs
- Parsers.cs
- UniqueIdentifierService.cs
- SystemInfo.cs
- SqlVersion.cs
- FormConverter.cs
- Function.cs
- DisplayMemberTemplateSelector.cs
- ComponentCollection.cs
- OperatingSystem.cs
- NetStream.cs
- VerificationException.cs
- Cursor.cs
- Msec.cs
- StdValidatorsAndConverters.cs
- DataGridViewImageCell.cs
- VisualProxy.cs
- NativeMethods.cs
- FileDialogPermission.cs
- ErrorInfoXmlDocument.cs
- MessageFilter.cs
- CapabilitiesRule.cs
- PublisherIdentityPermission.cs
- Model3DCollection.cs
- NewArrayExpression.cs
- CollectionBuilder.cs
- AttachmentCollection.cs
- PathNode.cs
- SerializationInfoEnumerator.cs
- XmlEntity.cs
- SpeakCompletedEventArgs.cs