Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Markup / Localizer / BamlLocalizableResourceKey.cs / 1 / BamlLocalizableResourceKey.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: BamlLocalizableResourceKey class // // History: // 03/25/2005 garyyang - created the file // //--------------------------------------------------------------------------- using System; namespace System.Windows.Markup.Localizer { ////// Key to BamlLocalizableResource /// public class BamlLocalizableResourceKey { //------------------------------- // Constructor //------------------------------- internal BamlLocalizableResourceKey( string uid, string className, string propertyName, string assemblyName ) { if (uid == null) { throw new ArgumentNullException("uid"); } if (className == null) { throw new ArgumentNullException("className"); } if (propertyName == null) { throw new ArgumentNullException("propertyName"); } _uid = uid; _className = className; _propertyName = propertyName; _assemblyName = assemblyName; } ////// Construct a key to the BamlLocalizableResource. The key /// consists of name, class name and property name, which will be used to /// identify a localizable resource in Baml. /// /// The unique id of the element that has the localizable resource. It is equivalent of x:Uid in XAML file. /// class name of localizable resource in Baml. /// property name of the localizable resource in Baml public BamlLocalizableResourceKey( string uid, string className, string propertyName ) : this (uid, className, propertyName, null) { } //------------------------------- // Public properties //------------------------------- ////// Id of the element that has the localizable resource /// public string Uid { get { return _uid; } } ////// Class name of the localizable resource /// public string ClassName { get { return _className; } } ////// Property name of the localizable resource /// public string PropertyName { get { return _propertyName; } } ////// The name of the assembly that defines the type of the localizable resource. /// ////// Assembly name is not required for uniquely identifying a resource in Baml. It is /// popluated when extracting resources from Baml so that users can find the type information /// of the localizable resource. /// public string AssemblyName { get { return _assemblyName; } } ////// Compare two BamlLocalizableResourceKey objects /// /// The other BamlLocalizableResourceKey object to be compared against ///True if they are equal. False otherwise public bool Equals(BamlLocalizableResourceKey other) { if (other == null) { return false; } return _uid == other._uid && _className == other._className && _propertyName == other._propertyName; } ////// Compare two BamlLocalizableResourceKey objects /// /// The other BamlLocalizableResourceKey object to be compared against ///True if they are equal. False otherwise public override bool Equals(object other) { return Equals(other as BamlLocalizableResourceKey); } ////// Get the hashcode of this object /// ///Hash code public override int GetHashCode() { return _uid.GetHashCode() ^ _className.GetHashCode() ^ _propertyName.GetHashCode(); } //------------------------------- // Private members //------------------------------- private string _uid; private string _className; private string _propertyName; private string _assemblyName; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: BamlLocalizableResourceKey class // // History: // 03/25/2005 garyyang - created the file // //--------------------------------------------------------------------------- using System; namespace System.Windows.Markup.Localizer { ////// Key to BamlLocalizableResource /// public class BamlLocalizableResourceKey { //------------------------------- // Constructor //------------------------------- internal BamlLocalizableResourceKey( string uid, string className, string propertyName, string assemblyName ) { if (uid == null) { throw new ArgumentNullException("uid"); } if (className == null) { throw new ArgumentNullException("className"); } if (propertyName == null) { throw new ArgumentNullException("propertyName"); } _uid = uid; _className = className; _propertyName = propertyName; _assemblyName = assemblyName; } ////// Construct a key to the BamlLocalizableResource. The key /// consists of name, class name and property name, which will be used to /// identify a localizable resource in Baml. /// /// The unique id of the element that has the localizable resource. It is equivalent of x:Uid in XAML file. /// class name of localizable resource in Baml. /// property name of the localizable resource in Baml public BamlLocalizableResourceKey( string uid, string className, string propertyName ) : this (uid, className, propertyName, null) { } //------------------------------- // Public properties //------------------------------- ////// Id of the element that has the localizable resource /// public string Uid { get { return _uid; } } ////// Class name of the localizable resource /// public string ClassName { get { return _className; } } ////// Property name of the localizable resource /// public string PropertyName { get { return _propertyName; } } ////// The name of the assembly that defines the type of the localizable resource. /// ////// Assembly name is not required for uniquely identifying a resource in Baml. It is /// popluated when extracting resources from Baml so that users can find the type information /// of the localizable resource. /// public string AssemblyName { get { return _assemblyName; } } ////// Compare two BamlLocalizableResourceKey objects /// /// The other BamlLocalizableResourceKey object to be compared against ///True if they are equal. False otherwise public bool Equals(BamlLocalizableResourceKey other) { if (other == null) { return false; } return _uid == other._uid && _className == other._className && _propertyName == other._propertyName; } ////// Compare two BamlLocalizableResourceKey objects /// /// The other BamlLocalizableResourceKey object to be compared against ///True if they are equal. False otherwise public override bool Equals(object other) { return Equals(other as BamlLocalizableResourceKey); } ////// Get the hashcode of this object /// ///Hash code public override int GetHashCode() { return _uid.GetHashCode() ^ _className.GetHashCode() ^ _propertyName.GetHashCode(); } //------------------------------- // Private members //------------------------------- private string _uid; private string _className; private string _propertyName; private string _assemblyName; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
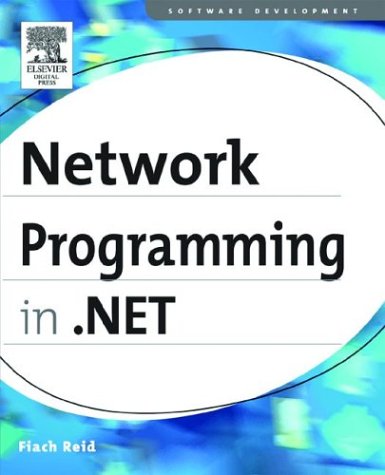
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Profiler.cs
- QilScopedVisitor.cs
- WaitHandleCannotBeOpenedException.cs
- StrongNameMembershipCondition.cs
- MenuAdapter.cs
- EntityType.cs
- DependsOnAttribute.cs
- XamlFilter.cs
- DataPagerFieldCollection.cs
- ImageCodecInfoPrivate.cs
- PTConverter.cs
- DataSourceSelectArguments.cs
- ScriptingScriptResourceHandlerSection.cs
- OletxDependentTransaction.cs
- WindowHideOrCloseTracker.cs
- CommandCollectionEditor.cs
- TypeSource.cs
- DataTableNewRowEvent.cs
- MultiSelectRootGridEntry.cs
- StructuredType.cs
- MasterPageParser.cs
- TableRowCollection.cs
- Utilities.cs
- WebPartConnectionsCancelVerb.cs
- QilInvoke.cs
- FrameworkElementAutomationPeer.cs
- dsa.cs
- HighlightVisual.cs
- DefaultValueAttribute.cs
- TargetControlTypeCache.cs
- ShadowGlyph.cs
- ToolStripContentPanel.cs
- SQLStringStorage.cs
- NullableBoolConverter.cs
- IdentitySection.cs
- UnhandledExceptionEventArgs.cs
- NamespaceCollection.cs
- ImportCatalogPart.cs
- CommandManager.cs
- RegexCaptureCollection.cs
- ColumnResizeUndoUnit.cs
- XhtmlStyleClass.cs
- FixedSOMContainer.cs
- MetadataHelper.cs
- CompiledRegexRunner.cs
- StagingAreaInputItem.cs
- StagingAreaInputItem.cs
- DataGridViewRowPrePaintEventArgs.cs
- DiscreteKeyFrames.cs
- Compiler.cs
- BuildDependencySet.cs
- TextCompositionEventArgs.cs
- ScrollProperties.cs
- TableProviderWrapper.cs
- RestClientProxyHandler.cs
- DiagnosticsConfigurationHandler.cs
- TrustLevelCollection.cs
- xsdvalidator.cs
- SafeProcessHandle.cs
- Validator.cs
- BitmapEffectDrawingContent.cs
- NoneExcludedImageIndexConverter.cs
- UpdateCommandGenerator.cs
- ThicknessAnimationUsingKeyFrames.cs
- ListControl.cs
- AVElementHelper.cs
- TreeView.cs
- PeerInvitationResponse.cs
- SelectionHighlightInfo.cs
- Converter.cs
- UpdatePanelControlTrigger.cs
- ListViewItem.cs
- ParamArrayAttribute.cs
- PrefixQName.cs
- OdbcParameter.cs
- ProfileModule.cs
- _CookieModule.cs
- ScriptMethodAttribute.cs
- QueryContinueDragEvent.cs
- EventItfInfo.cs
- MonikerBuilder.cs
- OpenFileDialog.cs
- ExternalException.cs
- FixUpCollection.cs
- LazyTextWriterCreator.cs
- ChannelServices.cs
- MemberPath.cs
- HMAC.cs
- HostVisual.cs
- SiteMapSection.cs
- GiveFeedbackEvent.cs
- ObjectListCommandCollection.cs
- SynchronizingStream.cs
- BitmapImage.cs
- RelationshipDetailsRow.cs
- ActiveDocumentEvent.cs
- ExpressionList.cs
- ObjectDataSourceSelectingEventArgs.cs
- TabControlCancelEvent.cs
- PageFunction.cs